1. 容器属性
编号 |
属性 |
描述 |
1 |
flex-flow |
主轴方向与换行方式 |
2 |
justify-conten |
项目在主轴上的对齐方式 |
3 |
aling-items |
项目在交叉轴上的对齐方式 |
4 |
align-content |
项目在多行容器中的对齐方式 |
代码示例
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>容器属性</title>
<style>
.container {
display: flex;
height: 40em;
padding: 1em;
margin: 1em;
border: limegreen 1px solid;
}
.item {
border: magenta solid 1px;
background-color: mediumslateblue;
width: 4em;
height: 3em;
}
/* 单行容器属性 */
.container {
/* flex-direction: row; 这个是默认属性 */
/* flex-wrap: nowrap; 这个是控制换行 */
/* 一般使用简写 */
/* flex-flow: nowrap row;默认值 */
/* 多行容器:一行显示不行,允许换行显示 */
/* flex-flow: row wrap; */
/* 换为列方向 主轴垂直方向*/
/* flex-flow: nowrap column; */
/* flex-flow: wrap column; */
}
/* 设置项目在单行容器上轴上的对齐前提:主轴上存在剩余空间 */
/* 空间分配的两个方案: */
.container {
/* 1.将所有项目视为一个整体,在项目组两边进行分配 */
/* 起始边对齐 */
justify-content: flex-start;
/* 终止边对齐 */
justify-content: flex-end;
/* 居中对齐 */
justify-content: center;
/* 2.将所有项目视为独立个体,在项目之间进行分配 */
/* 两端对齐 */
justify-content: space-between;
/*分散对齐:每个项目中间平均分配*/
justify-content: space-around;
/* 平均对齐 */
justify-content: space-evenly;
}
/* 交叉轴上的对齐方式: */
.container {
/* 默认拉伸 */
align-items: stretch;
align-items: flex-start;
align-items: flex-end;
align-items: center;
}
</style>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
</div>
</body>
</html>
效果:
- 起始边对齐

- 终止边对齐

- 居中对齐

- 两端对齐

- 分散对齐

- 平均对齐

1.4 align-content
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>容器属性</title>
<style>
.container {
display: flex;
height: 40em;
padding: 1em;
margin: 1em;
border: limegreen 1px solid;
}
.item {
border: magenta solid 1px;
background-color: mediumslateblue;
width: 4em;
height: 3em;
}
/* 多行容器属性 */
.container {
/* 多行容器 */
flex-flow: row wrap;
/* 默认值 */
align-content: stretch;
align-content: flex-start;
align-content: flex-end;
align-content: center;
/* 两端对齐 */
align-content: space-between;
/* 分散对齐 */
align-content: space-around;
/*平均对齐*/
align-content: space-evenly;
}
</style>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
</div>
</body>
</html>
效果:

2. 项目属性
编号 |
属性 |
描述 |
1 |
flex |
项目的缩放比例与基准宽度 |
2 |
align-self |
单个项目在交叉轴上的对齐方式 |
4 |
order |
项目在主轴上排列顺序 |
2.1 flex
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>flex</title>
<style>
.container {
display: flex;
height: 40em;
padding: 1em;
margin: 1em;
border: limegreen 1px solid;
}
.item {
border: magenta solid 1px;
background-color: mediumslateblue;
width: 8em;
height: 3em;
/* flex: flex-grow flex-shrink flex-basis */
/* flex: 放大因子 收缩因子 项目在主轴上的基准宽度 */
/* 默认属性,不放大可以收缩 */
flex: 0 1 auto;
flex: initial;
/* 放大和收缩 */
flex: 1 1 auto;
flex: auto;
/* 禁止放大收缩 */
flex: 0 0 auto;
/* 简写 */
flex: none;
/* 如果只有一个数字,省掉后面二个参数,表示的放大因子 */
flex: 1;
/* 等于 flex: 1 1 auto; */
/* flex一般不会用来设置所有项目的默认选项,通常用来设置某一个项目的特征 */
}
.container > .item:last-of-type {
flex: 2;
}
.container > .item:nth-of-type(2),
.container > .item:first-of-type {
flex: 1;
}
</style>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
</div>
</body>
</html>

2.2 align-self
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>容器属性</title>
<style>
.container {
display: flex;
height: 20em;
padding: 1em;
margin: 1em;
border: limegreen 1px solid;
}
.item {
border: magenta solid 1px;
background-color: mediumslateblue;
width: 4em;
height: 3em;
}
/* 设置一个项目与其它项目对齐方式不一样 */
.item:first-of-type {
align-self: stretch;
align-self: flex-start;
align-self: flex-end;
align-self: center;
}
</style>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
</div>
</body>
</html>
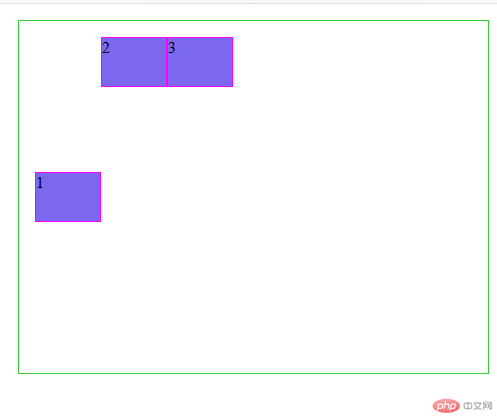
2.3 order
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>容器属性</title>
<style>
.container {
display: flex;
height: 40em;
padding: 1em;
margin: 1em;
border: limegreen 1px solid;
}
.item {
border: magenta solid 1px;
position: relative;
width: 4em;
height: 3em;
}
/* 设置一个项目与其它项目对齐方式不一样 */
.container > .item:first-of-type {
background-color: mediumslateblue;
order: 2;
}
.container > .item:nth-of-type(2) {
background-color: rgb(186, 211, 96);
order: 1;
}
.container > .item:last-of-type {
background-color: rgb(155, 238, 87);
order: 3;
}
</style>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
</div>
</body>
</html>
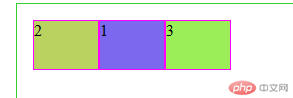