一、表格:超市购物清单
1、效果图:
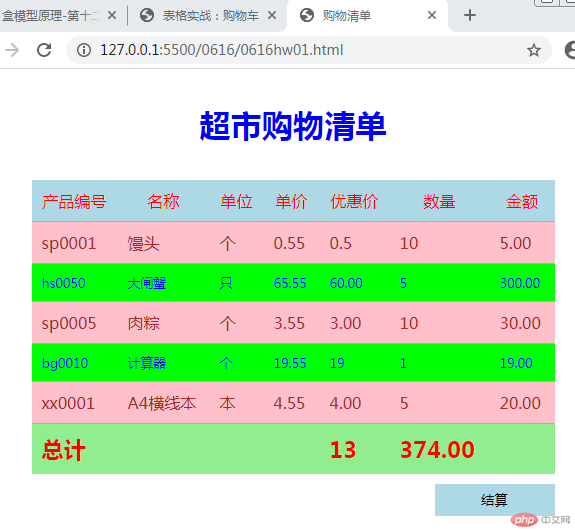
2、源码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>购物清单</title>
<style>
table{
/* 将单元格之间的间隙去除 */
border-collapse: collapse;
width:80%;
/* auto:左右居中 */
margin:auto;
color:black;
background-color: lightgreen;
}
/* 表格样式: */
table thead th,
table td{
/* 表格去掉上,右,左边线,只留底线:border-bottom: */
border-bottom:1px solid violet;
padding: 10px;
}
/* 标题样式: */
table caption{
font-size:1.3rem;
margin-bottom:10px;
color:blue;
}
table th{
/* 首行字体本身是粗体,变成细体如下: */
font-weight:lighter;
color:red;
background-color: lightblue;
}
/* 隔行变色 ,even:偶数行,奇数:odd*/
table tbody tr:nth-of-type(odd){
background-color: pink;
color:brown;
font-size: 1rem;
}
table tbody tr:nth-of-type(even) {
background-color: lime;
color:blue;
font-size: 0.8rem;
}
/* 鼠标悬停效果 */
table tbody tr:hover{
background-color: yellow;
}
/* 表尾单元格样式 */
table tfoot td{
border-bottom: none;
color:red;
font-size: 1.4rem;
/* 字体加粗 */
font-weight:bolder;
}
/* 结算样式 */
div:first-of-type{
/* div:first-of-type也是div:last-of-type */
width:80%;
margin:10px auto;
/* color:coral; */
background-color: darkgoldenrod;
}
div:first-of-type button{
float:right;
width:120px;
height:32px;
background-color:lightblue;
/* 去掉按钮框线 */
border:none;
/* 设置鼠标样式,鼠标移到按钮上时变成手 */
cursor:pointer;
}
div:first-of-type button:hover{
background-color:coral;
font-size: 1.2rem;
font-weight:bolder;
}
</style>
</head>
<body>
<!-- 表单结构 -->
<!-- <table>
表头:
<thead>
<tr>
<th></th>
<th></th>
</tr>
</thead>
主体:
<tbody>
<tr>
<td></td>
<td></td>
</tr>
</tbody>
表尾:
<tfoot>
<tr>
<td></td>
</tr>
</tfoot>
</table> -->
<table>
<caption><h2>超市购物清单</h2></caption>
<!-- tr-行,td-列,th-首行加粗+居中 -->
<thead>
<tr>
<!-- th*7 -->
<th>产品编号</th>
<th>名称</th>
<th>单位</th>
<th>单价</th>
<th>优惠价</th>
<th>数量</th>
<th>金额</th>
</tr>
</thead>
<tbody>
<tr>
<td>sp0001</td>
<td>馒头</td>
<td>个</td>
<td>0.55</td>
<td>0.5</td>
<td>10</td>
<td>5.00</td>
</tr>
<tr>
<td>hs0050</td>
<td>大闸蟹</td>
<td>只</td>
<td>65.55</td>
<td>60.00</td>
<td>5</td>
<td>300.00</td>
</tr>
<tr>
<td>sp0005</td>
<td>肉粽</td>
<td>个</td>
<td>3.55</td>
<td>3.00</td>
<td>10</td>
<td>30.00</td>
</tr>
<tr>
<td>bg0010</td>
<td>计算器</td>
<td>个</td>
<td>19.55</td>
<td>19</td>
<td>1</td>
<td>19.00</td>
</tr>
<tr>
<td>xx0001</td>
<td>A4横线本</td>
<td>本</td>
<td>4.55</td>
<td>4.00</td>
<td>5</td>
<td>20.00</td>
</tr>
</tbody>
<tfoot>
<tr>
<!-- column span=colspan -->
<td colspan="4"> 总计 </td>
<td>13</td>
<td>374.00</td>
</tr>
</tfoot>
</table >
<!-- 结算按钮 -->
<div>
<button>结算</button>
</div>
</body>
</html>
二、表格:个人简历
1、部分效果图:
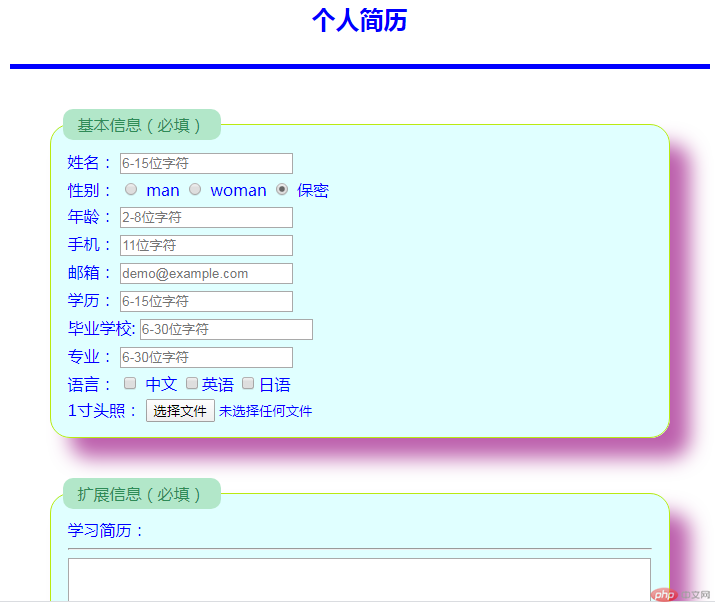
2、网页链接:
个人简历
3、源码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>个人简历</title>
<style>
body {
color:blue;
}
h2 {
text-align: center;
}
form {
width: 700px;
margin: 30px auto;
border-top: 5px solid blue;
}
form fieldset {
border: 1px solid rgb(186, 233, 16);
background-color: lightcyan;
box-shadow: 20px 20px 20px rgb(189, 96, 173);
/* 边框四角圆形弧度 */
border-radius: 20px;
margin: 40px;
/* margin: 0px;比较效果差 */
}
form fieldset legend {
background-color: rgb(178, 231, 201);
border-radius: 10px;
color: seagreen;
padding: 5px 15px;
}
form div {
margin: 5px;
}
form p {
text-align: center;
}
form .btn {
font-size: 1.2rem;
width: 100px;
height: 40px;
border: none;
background-color: seagreen;
color: #ddd;
}
form .btn:hover {
background-color: coral;
color: blue;
cursor: pointer;
}
input:focus {
background-color: rgb(39, 214, 62);
}
</style>
</head>
<body>
<h2>个人简历</h2>
<form action="" method="POST">
<fieldset>
<legend>基本信息(必填)</legend>
<div>
<label for="myname">姓名:</label>
<input
type="text"
id="myname"
name="name"
placeholder="6-15位字符"
autofocus
required
/>
</div>
<div>
<label for="secret">性别:</label>
<input
type="radio"
name="gender"
value="male"
id="male"/>
<label for="male">man</label>
<input
type="radio"
name="gender"
value="female"
id="female"/>
<label for="female">woman</label>
<input
type="radio"
name="gender"
value="secret"
id="secret"
checked
/>
<label for="secret">保密</label>
</div>
<div>
<label for="age">年龄:</label>
<input
type="text"
id="age"
name="age"
placeholder="2-8位字符"
autofocus
required
/>
</div>
<div>
<label for="cellphone">手机:</label>
<input
type="text"
id="cellphone"
name="phone"
placeholder="11位字符"
autofocus
required
/>
</div>
<div>
<label for="">邮箱:</label>
<input
type="email"
name="email"
id="email-id"
placeholder="demo@example.com"
required
/>
</div>
<div>
<label for="education">学历:</label>
<input
type="text"
id="education"
name="education"
placeholder="6-15位字符"
autofocus
required
/>
</div>
<div>
<label for="college">毕业学校:</label>
<input
type="text"
id="college"
name="college"
placeholder="6-30位字符"
autofocus
required
/>
</div>
<div>
<label for="main courses">专业:</label>
<input
type="text"
id="main courses"
name="main courses"
placeholder="6-30位字符"
autofocus
required
/>
</div>
<div>
<label for="language">语言:</label>
<input type="checkbox" name="language[]" id="chinese"
value="chinese"
/> <label for="chinese" >中文</label >
<input
type="checkbox"
name="language[]"
value="english"
id="english"
/><label for="english">英语</label>
<input
type="checkbox"
name="language[]"
value="japanses"
id="japanses"
/><label for="japanses">日语</label>
</div>
<div>
<label for="uploads">1寸头照:</label>
<input type="file" name="pic" id="uploads" accept="image/png, image/jpeg,image/gif" />
</div>
</fieldset>
<fieldset>
<legend>扩展信息(必填)</legend>
<div>
<label for="Study resume">学习简历:</label>
<hr>
<textarea name="study" id="Study resume" cols="80" rows="5" placeholder="不超过200字">
</textarea>
</div>
<div>
<label for="work experience">工作简历:</label>
<hr>
<textarea name="work" id="work experience" cols="80" rows="5" placeholder="不超过200字">
</textarea>
</div>
<label for="training">培训简历:</label>
<hr>
<textarea name="training" id="training" cols="80" rows="5" placeholder="不超过200字">
</textarea>
<div>
<label for="Certificate">资格证:</label>
<hr>
<textarea name="Certificate" id="Certificate" cols="80" rows="5" placeholder="不超过200字">
</textarea>
</div>
<div>
<label for="skills">技能获得:</label>
<hr>
<textarea name="skills" id="skills" cols="80" rows="5" placeholder="不超过200字">
</textarea>
</div>
</fieldset>
<fieldset>
<legend>其他信息(选填)</legend>
<div>
<label for="hobby">爱好:</label>
<input type="checkbox" name="hobby[]" id="moive"
value="moive"
/> <label for="moive" >看电影</label >
<input
type="checkbox"
name="hobby[]"
value="shoot"
id="shoot"
/><label for="shoot">摄影</label>
<input
type="checkbox"
name="hobby[]"
value="jogging"
id="jogging"
/><label for="jogging">慢跑</label>
<input
type="checkbox"
name="hobby[]"
value="travel"
id="travel"
/><label for="travel">旅行</label >
</div>
<div>
<label for="constellation">星座:</label>
<input
type="text"
id="constellation"
name="constellation"
placeholder="6-15位字符"
autofocus
required
/>
</div>
<div>
<label for="Btype">血型:</label>
<input type="checkbox" name="Btype[]" id="A"
value="A"
/> <label for="B" >A</label >
<input
type="checkbox"
name="Btype[]"
value="B"
id="B"
/><label for="B">B</label>
<input
type="checkbox"
name="Btype[]"
value="O"
id="O"
/><label for="O">O</label>
</div>
<div>
<label for="awards">获奖:</label>
<hr>
<textarea name="skills" id="skills" cols="80" rows="5" placeholder="不超过200字">
</textarea>
</div>
<div>
<label for="schoolarships">奖学金:</label>
<hr>
<textarea name="skills" id="skills" cols="80" rows="5" placeholder="不超过200字">
</textarea>
</div>
<div>
<label for=" Self-evaluation">自我评价:</label>
<hr>
<textarea name="Self-evaluation" id="Self-evaluation" cols="80" rows="5" placeholder="不超过200字">
</textarea>
</div>
</fieldset>
<p>
<button class="btn">提交</button>
</p>
</form>
</body>
</html>