CSS选择器
1.简单选择器与ID选择器,类选择器
序号 |
选择器 |
描述 |
举例 |
1 |
元素选择器 |
根据元素标签名称进行匹配 |
div {...} |
2 |
群组选择器 |
同时选择多个不同类型的元素 |
h1,h2,h3{...} |
3 |
通配选择器 |
选择全部元素,不区分类型 |
* {...} |
4 |
属性选择器 |
根据元素属性进行匹配 |
*[...] |
5 |
类选择器 |
根据元素 class 属性进行匹配 |
*.active {...} |
6 |
id 选择器 |
根据元素 id 属性进行匹配 |
*#top {...} |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>简单选择器</title>
</head>
<style>
.container {
width: 300px;
height: 300px;
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 5px;
}
.item {
font-size: 2rem;
background-color: lightblue;
color: white;
display: flex;
justify-content: center;
align-items: center;
}
#first {
background-color: slateblue;
}
.item[title="hello"] {
background-color: red;
}
.item[title="php"] {
background-color: sienna;
}
</style>
<body>
<div class="container">
<div class="item" id="first">1</div>
<div class="item">2</div>
<div class="item" title="hello">3</div>
<div class="item">4</div>
<div class="item" title="php">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
效果图
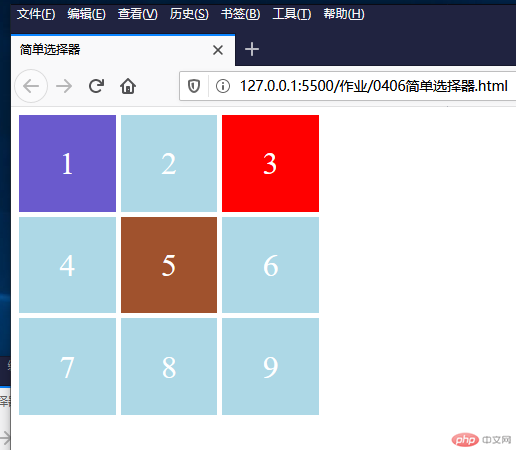
2.上下文选择器
序号 |
选择器 |
操作符 |
描述 |
举例 |
1 |
后代选择器 |
空格 |
选择当前元素的所有后代元素 |
div p , body * |
2 |
父子选择器 |
> |
选择当前元素的所有子元素 |
div > h2 |
3 |
同级相邻选择器 |
+ |
选择拥有共同父级且相邻的元素 |
li.red + li |
4 |
同级所有选择器 |
~ |
选择拥有共同父级的后续所有元素 |
li.red ~ li |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>上下文选择器</title>
</head>
<style>
.container {
width: 300px;
height: 300px;
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 5px;
}
.item {
font-size: 2rem;
background-color: lightskyblue;
display: flex;
justify-content: center;
align-items: center;
}
.container div {
border: 2px solid brown;
}
body > div {
border: 2px solid red;
}
.item.center ~ .item {
background-color: yellow;
}
</style>
<body>
<div class="container">
<div class="item" id="first">1</div>
<div class="item">2</div>
<div class="item" title="hello">3</div>
<div class="item center">4</div>
<div class="item" title="php">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
效果图
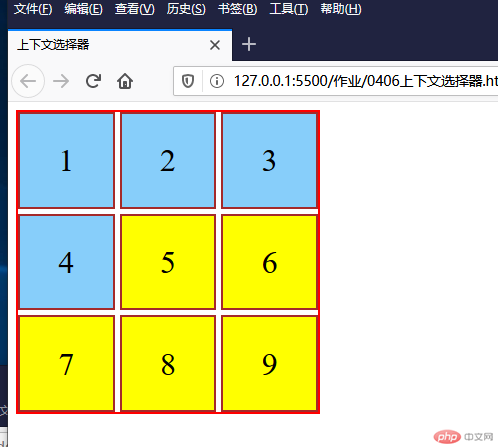
3.伪类选择器
3.1 伪类不分组
序号 |
选择器 |
描述 |
举例 |
1 |
:first-child |
匹配第一个子元素 |
div :first-child |
2 |
:last-child |
匹配最后一个子元素 |
div :last-child |
3 |
:only-child |
选择元素的唯一子元素 |
div :only-child |
4 |
:nth-child(n) |
匹配任意位置的子元素 |
div :nth-child(n) |
5 |
:nth-last-child(n) |
匹配倒数任意位置的子元素 |
div :nth-last-child(n) |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>伪类选择器:不分组</title>
<style>
.container {
width: 300px;
height: 300px;
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 5px;
}
.item {
font-size: 2rem;
background-color: lightskyblue;
display: flex;
justify-content: center;
align-items: center;
}
* .container :first-child {
background-color: red;
}
.container :nth-child(3) {
background-color: salmon;
}
.container :last-child {
background-color: lightgreen;
}
.container :nth-child(even) {
background-color: lime;
}
.container :nth-last-child(3) {
background-color: mediumblue;
}
</style>
</head>
<body>
<div class="container">
<div class="item first">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item center">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
效果图
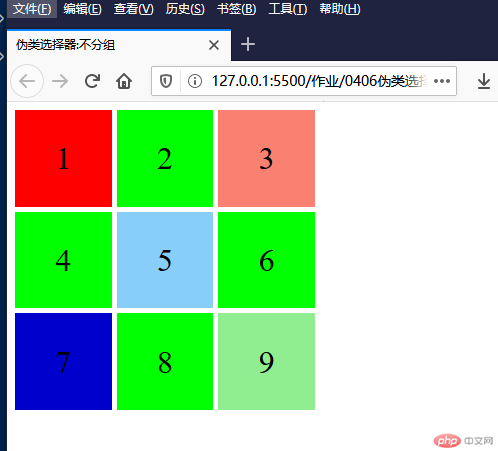
3.2伪类分组
序号 |
选择器 |
描述 |
举例 |
1 |
:first-of-type |
匹配按类型分组后的第一个子元素 |
div :first-of-type |
2 |
:last-of-type |
匹配按类型分组后的最后一个子元素 |
div :last-of-type |
3 |
:only-of-type |
匹配按类型分组后的唯一子元素 |
div :only-of-type |
4 |
:nth-of-type() |
匹配按类型分组后的任意位置的子元素 |
div :nth-of-type(n) |
5 |
:nth-last-of-type() |
匹配按类型分组后倒数任意位置的子元素 |
div :nth-last-of-type(n) |
- 允许使用表达式来匹配一组元素,表达式中的”n”是从”0”开始计数,且必须写到前面
- “-n”表示获取前面一组元素,正数表示从指定位置获取余下元素
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>伪类选择器分组</title>
<style>
.container {
width: 300px;
height: 300px;
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 5px;
}
.item {
font-size: 2rem;
background-color: lightskyblue;
display: flex;
justify-content: center;
align-items: center;
}
.container span:first-of-type {
background-color: blueviolet;
}
.container div:first-of-type {
background-color: brown;
}
.container span:nth-last-of-type(3) {
background-color: chocolate;
}
.container div:nth-last-of-type(2) {
background-color: darkcyan;
}
</style>
</head>
<body>
<div class="container">
<div class="item first">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<span class="item center">5</span>
<span class="item">6</span>
<span class="item">7</span>
<span class="item">8</span>
<span class="item">9</span>
</div>
</body>
</html>
效果图
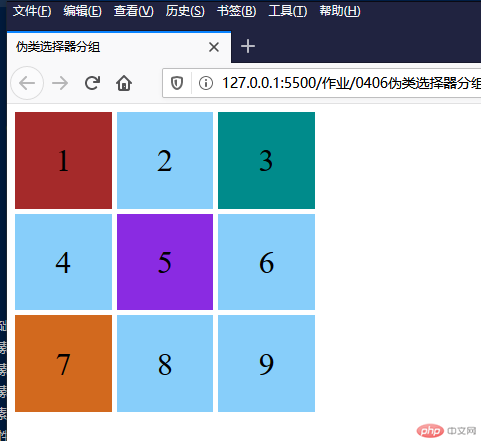
3.2表单伪类
序号 |
选择器 |
描述 |
1 |
:active |
向被激活的元素添加样式 |
2 |
:focus |
向拥有键盘输入焦点的元素添加样式 |
3 |
:hover |
当鼠标悬浮在元素上方时,向元素添加样式 |
4 |
:link |
向未被访问的链接添加样式 |
5 |
:visited |
向已被访问的链接添加样式 |
5 |
:root |
根元素,通常是html |
5 |
:empty |
选择没有任何子元素的元素(含文本节点) |
5 |
:not() |
排除与选择器参数匹配的元素 |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<style>
:root {
background-color: darkkhaki;
}
input:required {
background-color: white;
}
input:disabled {
background-color: lightslategray;
color: red;
}
input:hover {
background-color: slateblue;
}
</style>
<body>
<form action="" id="register"></form>
<!-- 第一个表单分组 -->
<fieldset name="base" form="register">
<legend>基本信息</legend>
<section>
<input
type="email"
name="email"
placeholder="您的邮箱"
form="register"
required
autofocus
/>
<input
type="password"
name="psw1"
placeholder="您的密码"
form="register"
required
/>
<input
type="password"
name="psw2"
placeholder="重复密码"
form="register"
/>
</section>
</fieldset>
<fieldset name="base" form="register">
<legend>选填信息</legend>
<section>
<input
type="text"
name="nicheng"
placeholder="您的昵称"
form="register"
autofocus
/>
<input
type="number"
name="nianling"
placeholder="您的年龄"
form="register"
/>
<input
type="text"
name="gexing"
placeholder="个性签名"
form="register"
/>
<input
type="text"
name="tishi"
placeholder="提示:XXXXXXX"
form="register"
disabled
/>
</section>
</fieldset>
<button
type="submit"
form="register"
formenctype="register.php"
formmethod="POST"
formtarget="_blank"
>
提交
</button>
</body>
</html>
效果图
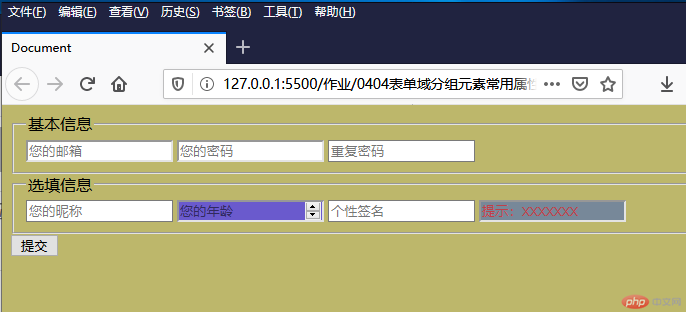
4.总结
4.1 简单选择器
- 最常用的是: 元素选择器, 类选择器, id 选择器
- 当 class,id 选择器不限定被修改的元素类型时, 星号”
*
“可以省略
4.2 上下文选择器
序号 |
选择器 |
操作符 |
描述 |
举例 |
1 |
后代选择器 |
空格 |
选择当前元素的所有后代元素 |
div p , body * |
2 |
父子选择器 |
> |
选择当前元素的所有子元素 |
div > h2 |
3 |
同级相邻选择器 |
+ |
选择拥有共同父级且相邻的元素 |
li.red + li |
4 |
同级所有选择器 |
~ |
选择拥有共同父级的后续所有元素 |
li.red ~ li |
4.3 伪类选择器
4.3.1 伪类选择器-不分组匹配
场景 |
描述 |
结构伪类 |
根据子元素的位置特征进行选择 |
表单伪类 |
根据表单控件状态特征进行选择 |
序号 |
选择器 |
描述 |
举例 |
1 |
:first-child |
匹配第一个子元素 |
div :first-child |
2 |
:last-child |
匹配最后一个子元素 |
div :last-child |
3 |
:only-child |
选择元素的唯一子元素 |
div :only-child |
4 |
:nth-child(n) |
匹配任意位置的子元素 |
div :nth-child(n) |
5 |
:nth-last-child(n) |
匹配倒数任意位置的子元素 |
div :nth-last-child(n) |
4.3.2 伪类选择器-分组匹配
序号 |
选择器 |
描述 |
举例 |
1 |
:first-of-type |
匹配按类型分组后的第一个子元素 |
div :first-of-type |
2 |
:last-of-type |
匹配按类型分组后的最后一个子元素 |
div :last-of-type |
3 |
:only-of-type |
匹配按类型分组后的唯一子元素 |
div :only-of-type |
4 |
:nth-of-type() |
匹配按类型分组后的任意位置的子元素 |
div :nth-of-type(n) |
5 |
:nth-last-of-type() |
匹配按类型分组后倒数任意位置的子元素 |
div :nth-last-of-type(n) |
4.3.3 表单伪类
序号 |
选择器 |
描述 |
1 |
:active |
向被激活的元素添加样式 |
2 |
:focus |
向拥有键盘输入焦点的元素添加样式 |
3 |
:hover |
当鼠标悬浮在元素上方时,向元素添加样式 |
4 |
:link |
向未被访问的链接添加样式 |
5 |
:visited |
向已被访问的链接添加样式 |
5 |
:root |
根元素,通常是html |
5 |
:empty |
选择没有任何子元素的元素(含文本节点) |
5 |
:not() |
排除与选择器参数匹配的元素 |