What are Vuex plugins? How can you create custom plugins?
Vuex plugins are essentially functions that are used to extend the behavior of a Vuex store. They can be used to log mutations, persist the state, or even to integrate the store with external APIs and services. Plugins have access to the store's internals and can react to mutations and actions, making them powerful tools for enhancing the functionality of a Vuex store.
To create a custom Vuex plugin, you need to define a function that takes the store as its argument. Inside this function, you can subscribe to the store's mutations and actions, and perform any necessary operations. Here's a basic example of how to create a custom plugin:
const myCustomPlugin = (store) => { // Called when the store is initialized store.subscribe((mutation, state) => { // Log each mutation console.log(mutation.type) console.log(mutation.payload) }) } // To use the plugin, you would pass it to the Vuex store constructor const store = new Vuex.Store({ // ... other store options plugins: [myCustomPlugin] })
In this example, myCustomPlugin
logs every mutation that occurs in the store. You can extend this concept to create more complex plugins that interact with the store in various ways.
What functionality can Vuex plugins add to a Vuex store?
Vuex plugins can add a wide range of functionalities to a Vuex store. Some common functionalities include:
- State Persistence: Plugins can save the state to local storage or another persistent storage mechanism, allowing the application to restore the state when it restarts.
- Logging: Plugins can log mutations and actions, which is useful for debugging and monitoring the application's state changes.
- Integration with External APIs: Plugins can be used to synchronize the store's state with external APIs, ensuring that the application's state remains consistent with external data sources.
- Time Travel Debugging: Plugins can implement time travel debugging, allowing developers to step through the state changes of the application.
- Data Validation: Plugins can validate the state before mutations are committed, ensuring that the state remains in a valid state.
- Performance Monitoring: Plugins can track the performance of mutations and actions, helping to identify bottlenecks in the application.
By leveraging these functionalities, Vuex plugins can significantly enhance the capabilities of a Vuex store, making it more robust and easier to manage.
How do you install and use an existing Vuex plugin in your project?
To install and use an existing Vuex plugin in your project, follow these steps:
-
Install the Plugin: Most Vuex plugins are available as npm packages. You can install them using npm or yarn. For example, to install the
vuex-persistedstate
plugin, you would run:npm install vuex-persistedstate # or yarn add vuex-persistedstate
-
Import the Plugin: In your Vuex store file, import the plugin. For example:
import createPersistedState from 'vuex-persistedstate'
-
Configure and Use the Plugin: Pass the plugin to the Vuex store constructor. You can configure the plugin according to its documentation. For
vuex-persistedstate
, you might do something like this:const store = new Vuex.Store({ // ... other store options plugins: [createPersistedState()] })
- Use the Plugin's Functionality: Depending on the plugin, you might need to configure additional settings or use specific methods provided by the plugin. Refer to the plugin's documentation for detailed usage instructions.
By following these steps, you can easily integrate existing Vuex plugins into your project and leverage their functionalities to enhance your Vuex store.
What are some popular Vuex plugins that can enhance state management?
There are several popular Vuex plugins that can enhance state management in Vue.js applications. Some of the most widely used include:
- vuex-persistedstate: This plugin allows you to persist the Vuex store to local storage, session storage, or cookies. It's useful for maintaining the application's state across page reloads or browser sessions.
- vuex-orm: Vuex ORM is a plugin that provides an Object-Relational Mapping (ORM) system for Vuex. It simplifies the management of complex data models and relationships within the store.
- vuex-shared-mutations: This plugin enables you to share mutations between different tabs or windows of the same application, ensuring that the state remains synchronized across multiple instances.
- vuex-easy-firestore: This plugin integrates Vuex with Firebase Firestore, allowing you to easily synchronize your Vuex store with a Firestore database. It's particularly useful for real-time applications.
- vuex-pathify: Vuex Pathify is a plugin that simplifies the process of accessing and mutating the state in Vuex. It provides a more intuitive and concise syntax for working with the store.
These plugins can significantly enhance the capabilities of your Vuex store, making it easier to manage complex state and integrate with external services. By choosing the right plugins for your project, you can streamline your development process and improve the overall performance and reliability of your application.
The above is the detailed content of What are Vuex plugins? How can you create custom plugins?. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.

Vue.js and React each have their own advantages and disadvantages. When choosing, you need to comprehensively consider team skills, project size and performance requirements. 1) Vue.js is suitable for fast development and small projects, with a low learning curve, but deep nested objects can cause performance problems. 2) React is suitable for large and complex applications, with a rich ecosystem, but frequent updates may lead to performance bottlenecks.

Vue.js is suitable for small to medium-sized projects, while React is suitable for large projects and complex application scenarios. 1) Vue.js is easy to use and is suitable for rapid prototyping and small applications. 2) React has more advantages in handling complex state management and performance optimization, and is suitable for large projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
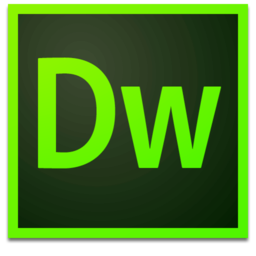
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
