intermediary pattern
The Mediator Pattern is used to reduce the complexity of communication between multiple objects and classes. This pattern provides an intermediary class that typically handles communication between different classes and supports loose coupling, making the code easier to maintain. The mediator model is a behavioral model.
Introduction
Intent:Use an intermediary object to encapsulate a series of object interactions. The intermediary allows each object to couple without explicitly referencing each other. loose, and can independently change their interactions.
Main solution: There are a large number of relationships between objects, which will inevitably cause the system structure to become very complicated. At the same time, if an object changes, we also need to track and associated objects and perform corresponding processing at the same time.
When to use: Multiple classes are coupled to each other, forming a network structure.
How to solve: Separate the above mesh structure into a star structure.
Key code: The communication between object Colleague is encapsulated into a class and handled separately.
Application examples: 1. Before China joined the WTO, various countries traded with each other, and the structure was complex. Now, various countries trade with each other through the WTO. 2. Airport dispatch system. 3. MVC framework, in which C (controller) is the mediator between M (model) and V (view).
Advantages: 1. Reduce the complexity of the class and convert one-to-many into one-to-one. 2. Decoupling between various classes. 3. Comply with the Dimit principle.
Disadvantages: The intermediary will be huge and become complex and difficult to maintain.
Usage scenarios: 1. There are relatively complex reference relationships between objects in the system, resulting in a confusing dependency structure between them and making it difficult to reuse the object. 2. I want to use an intermediate class to encapsulate the behaviors in multiple classes without generating too many subclasses.
Note: should not be used when responsibilities are confusing.
Implementation
We demonstrate the mediator pattern through a chat room instance. In this example, multiple users can send messages to the chat room, and the chat room displays the messages to all users. We will create two classes ChatRoom and User. User objects use the ChatRoom methods to share their messages.
MediatorPatternDemo, our demo class uses the User object to show the communication between them.
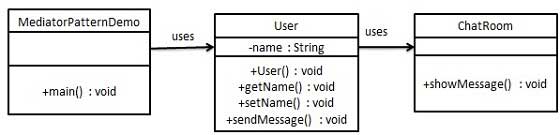
Step 1
Create an intermediary class.
ChatRoom.java
import java.util.Date; public class ChatRoom { public static void showMessage(User user, String message){ System.out.println(new Date().toString() + " [" + user.getName() +"] : " + message); } }
Step 2
Create the user class.
User.java
public class User { private String name; public String getName() { return name; } public void setName(String name) { this.name = name; } public User(String name){ this.name = name; } public void sendMessage(String message){ ChatRoom.showMessage(this,message); } }
Step 3
Use the User object to display the communication between them.
MediatorPatternDemo.java
public class MediatorPatternDemo { public static void main(String[] args) { User robert = new User("Robert"); User john = new User("John"); robert.sendMessage("Hi! John!"); john.sendMessage("Hello! Robert!"); } }
Step 4
Verify the output.
Thu Jan 31 16:05:46 IST 2013 [Robert] : Hi! John! Thu Jan 31 16:05:46 IST 2013 [John] : Hello! Robert!