Test Paragraph.
// prevent execution of jQuery if included more than once if(typeof window.jQuery == "undefined") { /* * jQuery 1.1.3a - New Wave Javascript * * Copyright (c) 2007 John Resig (jquery.com) * Dual licensed under the MIT (MIT-LICENSE.txt) * a
// prevent execution of jQuery if included more than once
if(typeof window.jQuery == "undefined") {
/*
* jQuery 1.1.3a - New Wave Javascript
*
* Copyright (c) 2007 John Resig (jquery.com)
* Dual licensed under the MIT (MIT-LICENSE.txt)
* and GPL (GPL-LICENSE.txt) licenses.
*
* $Date: 2007-05-23 08:48:15 -0400 (Wed, 23 May 2007) $
* $Rev: 1961 $
*/
// Global undefined variable
window.undefined = window.undefined;
/**
* Create a new jQuery Object
*
* @constructor
* @private
* @name jQuery
* @param String|Function|Element|Array
* @param jQuery|Element|Array
* @cat Core
*/
var jQuery = function(a,c) {
// If the context is global, return a new object
if ( window == this )
return new jQuery(a,c);
return this.init(a,c);
};
// Map over the $ in case of overwrite
if ( typeof $ != "undefined" )
jQuery._$ = $;
// Map the jQuery namespace to the '$' one
var $ = jQuery;
/**
* This function accepts a string containing a CSS or
* basic XPath selector which is then used to match a set of elements.
*
* The core functionality of jQuery centers around this function.
* Everything in jQuery is based upon this, or uses this in some way.
* The most basic use of this function is to pass in an expression
* (usually consisting of CSS or XPath), which then finds all matching
* elements.
*
* By default, if no context is specified, $() looks for DOM elements within the context of the
* current HTML document. If you do specify a context, such as a DOM
* element or jQuery object, the expression will be matched against
* the contents of that context.
*
* See [[DOM/Traversing/Selectors]] for the allowed CSS/XPath syntax for expressions.
*
* @example $("div > p")
* @desc Finds all p elements that are children of a div element.
* @before
one
two
three
* @result [
two
]*
* @example $("input:radio", document.forms[0])
* @desc Searches for all inputs of type radio within the first form in the document
*
* @example $("div", xml.responseXML)
* @desc This finds all div elements within the specified XML document.
*
* @name $
* @param String expr An expression to search with
* @param Element|jQuery context (optional) A DOM Element, Document or jQuery to use as context
* @cat Core
* @type jQuery
* @see $(Element)
* @see $(Element
*/
/**
* Create DOM elements on-the-fly from the provided String of raw HTML.
*
* @example $("
Hello
* @desc Creates a div element (and all of its contents) dynamically,
* and appends it to the body element. Internally, an
* element is created and its innerHTML property set to the given markup.
* It is therefore both quite flexible and limited.
*
* @name $
* @param String html A string of HTML to create on the fly.
* @cat Core
* @type jQuery
* @see appendTo(String)
*/
/**
* Wrap jQuery functionality around a single or multiple DOM Element(s).
*
* This function also accepts XML Documents and Window objects
* as valid arguments (even though they are not DOM Elements).
*
* @example $(document.body).css( "background", "black" );
* @desc Sets the background color of the page to black.
*
* @example $( myForm.elements ).hide()
* @desc Hides all the input elements within a form
*
* @name $
* @param Element|Array
* @cat Core
* @type jQuery
*/
/**
* A shorthand for $(document).ready(), allowing you to bind a function
* to be executed when the DOM document has finished loading. This function
* behaves just like $(document).ready(), in that it should be used to wrap
* other $() operations on your page that depend on the DOM being ready to be
* operated on. While this function is, technically, chainable - there really
* isn't much use for chaining against it.
*
* You can have as many $(document).ready events on your page as you like.
*
* See ready(Function) for details about the ready event.
*
* @example $(function(){
* // Document is ready
* });
* @desc Executes the function when the DOM is ready to be used.
*
* @example jQuery(function($) {
* // Your code using failsafe $ alias here...
* });
* @desc Uses both the shortcut for $(document).ready() and the argument
* to write failsafe jQuery code using the $ alias, without relying on the
* global alias.
*
* @name $
* @param Function fn The function to execute when the DOM is ready.
* @cat Core
* @type jQuery
* @see ready(Function)
*/
jQuery.fn = jQuery.prototype = {
/**
* Initialize a new jQuery object
*
* @private
* @name init
* @param String|Function|Element|Array
* @param jQuery|Element|Array
* @cat Core
*/
init: function(a,c) {
// Make sure that a selection was provided
a = a || document;
// HANDLE: $(function)
// Shortcut for document ready
if ( jQuery.isFunction(a) )
return new jQuery(document)[ jQuery.fn.ready ? "ready" : "load" ]( a );
// Handle HTML strings
if ( typeof a == "string" ) {
// HANDLE: $(html) -> $(array)
var m = /^[^)[^>]*$/.exec(a);
if ( m )
a = jQuery.clean( [ m[1] ] );
// HANDLE: $(expr)
else
return new jQuery( c ).find( a );
}
return this.setArray(
// HANDLE: $(array)
a.constructor == Array && a ||
// HANDLE: $(arraylike)
// Watch for when an array-like object is passed as the selector
(a.jquery || a.length && a != window && !a.nodeType && a[0] != undefined && a[0].nodeType) && jQuery.makeArray( a ) ||
// HANDLE: $(*)
[ a ] );
},
/**
* The current version of jQuery.
*
* @private
* @property
* @name jquery
* @type String
* @cat Core
*/
jquery: "1.1.3a",
/**
* The number of elements currently matched. The size function will return the same value.
*
* @example $("img").length;
* @before
* @result 2
*
* @property
* @name length
* @type Number
* @cat Core
*/
/**
* Get the number of elements currently matched. This returns the same
* number as the 'length' property of the jQuery object.
*
* @example $("img").size();
* @before
* @result 2
*
* @name size
* @type Number
* @cat Core
*/
size: function() {
return this.length;
},
length: 0,
/**
* Access all matched DOM elements. This serves as a backwards-compatible
* way of accessing all matched elements (other than the jQuery object
* itself, which is, in fact, an array of elements).
*
* It is useful if you need to operate on the DOM elements themselves instead of using built-in jQuery functions.
*
* @example $("img").get();
* @before
* @result [
]
* @desc Selects all images in the document and returns the DOM Elements as an Array
*
* @name get
* @type Array
* @cat Core
*/
/**
* Access a single matched DOM element at a specified index in the matched set.
* This allows you to extract the actual DOM element and operate on it
* directly without necessarily using jQuery functionality on it.
*
* @example $("img").get(0);
* @before
* @result
* @desc Selects all images in the document and returns the first one
*
* @name get
* @type Element
* @param Number num Access the element in the Nth position.
* @cat Core
*/
get: function( num ) {
return num == undefined ?
// Return a 'clean' array
jQuery.makeArray( this ) :
// Return just the object
this[num];
},
/**
* Set the jQuery object to an array of elements, while maintaining
* the stack.
*
* @example $("img").pushStack([ document.body ]);
* @result $("img").pushStack() == [ document.body ]
*
* @private
* @name pushStack
* @type jQuery
* @param Elements elems An array of elements
* @cat Core
*/
pushStack: function( a ) {
var ret = jQuery(a);
ret.prevObject = this;
return ret;
},
/**
* Set the jQuery object to an array of elements. This operation is
* completely destructive - be sure to use .pushStack() if you wish to maintain
* the jQuery stack.
*
* @example $("img").setArray([ document.body ]);
* @result $("img").setArray() == [ document.body ]
*
* @private
* @name setArray
* @type jQuery
* @param Elements elems An array of elements
* @cat Core
*/
setArray: function( a ) {
this.length = 0;
[].push.apply( this, a );
return this;
},
/**
* Execute a function within the context of every matched element.
* This means that every time the passed-in function is executed
* (which is once for every element matched) the 'this' keyword
* points to the specific DOM element.
*
* Additionally, the function, when executed, is passed a single
* argument representing the position of the element in the matched
* set (integer, zero-index).
*
* @example $("img").each(function(i){
* this.src = "test" + i + ".jpg";
* });
* @before
* @result
* @desc Iterates over two images and sets their src property
*
* @name each
* @type jQuery
* @param Function fn A function to execute
* @cat Core
*/
each: function( fn, args ) {
return jQuery.each( this, fn, args );
},
/**
* Searches every matched element for the object and returns
* the index of the element, if found, starting with zero.
* Returns -1 if the object wasn't found.
*
* @example $("*").index( $('#foobar')[0] )
* @before
* @result 0
* @desc Returns the index for the element with ID foobar
*
* @example $("*").index( $('#foo')[0] )
* @before
* @result 2
* @desc Returns the index for the element with ID foo within another element
*
* @example $("*").index( $('#bar')[0] )
* @before
* @result -1
* @desc Returns -1, as there is no element with ID bar
*
* @name index
* @type Number
* @param Element subject Object to search for
* @cat Core
*/
index: function( obj ) {
var pos = -1;
this.each(function(i){
if ( this == obj ) pos = i;
});
return pos;
},
/**
* Access a property on the first matched element.
* This method makes it easy to retrieve a property value
* from the first matched element.
*
* If the element does not have an attribute with such a
* name, undefined is returned.
*
* @example $("img").attr("src");
* @before
* @result test.jpg
* @desc Returns the src attribute from the first image in the document.
*
* @name attr
* @type Object
* @param String name The name of the property to access.
* @cat DOM/Attributes
*/
/**
* Set a key/value object as properties to all matched elements.
*
* This serves as the best way to set a large number of properties
* on all matched elements.
*
* @example $("img").attr({ src: "test.jpg", alt: "Test Image" });
* @before
* @result
* @desc Sets src and alt attributes to all images.
*
* @name attr
* @type jQuery
* @param Map properties Key/value pairs to set as object properties.
* @cat DOM/Attributes
*/
/**
* Set a single property to a value, on all matched elements.
*
* Note that you can't set the name property of input elements in IE.
* Use $(html) or .append(html) or .html(html) to create elements
* on the fly including the name property.
*
* @example $("img").attr("src","test.jpg");
* @before
* @result
* @desc Sets src attribute to all images.
*
* @name attr
* @type jQuery
* @param String key The name of the property to set.
* @param Object value The value to set the property to.
* @cat DOM/Attributes
*/
/**
* Set a single property to a computed value, on all matched elements.
*
* Instead of supplying a string value as described
* [[DOM/Attributes#attr.28_key.2C_value_.29|above]],
* a function is provided that computes the value.
*
* @example $("img").attr("title", function() { return this.src });
* @before
* @result
* @desc Sets title attribute from src attribute.
*
* @example $("img").attr("title", function(index) { return this.title + (i + 1); });
* @before
* @result
* @desc Enumerate title attribute.
*
* @name attr
* @type jQuery
* @param String key The name of the property to set.
* @param Function value A function returning the value to set.
* Scope: Current element, argument: Index of current element
* @cat DOM/Attributes
*/
attr: function( key, value, type ) {
var obj = key;
// Look for the case where we're accessing a style value
if ( key.constructor == String )
if ( value == undefined )
return this.length && jQuery[ type || "attr" ]( this[0], key ) || undefined;
else {
obj = {};
obj[ key ] = value;
}
// Check to see if we're setting style values
return this.each(function(index){
// Set all the styles
for ( var prop in obj )
jQuery.attr(
type ? this.style : this,
prop, jQuery.prop(this, obj[prop], type, index, prop)
);
});
},
/**
* Access a style property on the first matched element.
* This method makes it easy to retrieve a style property value
* from the first matched element.
*
* @example $("p").css("color");
* @before
Test Paragraph.
* @result "red"
* @desc Retrieves the color style of the first paragraph
*
* @example $("p").css("font-weight");
* @before
Test Paragraph.
* @result "bold"
* @desc Retrieves the font-weight style of the first paragraph.
*
* @name css
* @type String
* @param String name The name of the property to access.
* @cat CSS
*/
/**
* Set a key/value object as style properties to all matched elements.
*
* This serves as the best way to set a large number of style properties
* on all matched elements.
*
* @example $("p").css({ color: "red", background: "blue" });
* @before
Test Paragraph.
* @result
Test Paragraph.
* @desc Sets color and background styles to all p elements.
*
* @name css
* @type jQuery
* @param Map properties Key/value pairs to set as style properties.
* @cat CSS
*/
/**
* Set a single style property to a value, on all matched elements.
* If a number is provided, it is automatically converted into a pixel value.
*
* @example $("p").css("color","red");
* @before
Test Paragraph.
* @result
Test Paragraph.
* @desc Changes the color of all paragraphs to red
*
* @example $("p").css("left",30);
* @before
Test Paragraph.
* @result
Test Paragraph.
* @desc Changes the left of all paragraphs to "30px"
*
* @name css
* @type jQuery
* @param String key The name of the property to set.
* @param String|Number value The value to set the property to.
* @cat CSS
*/
css: function( key, value ) {
return this.attr( key, value, "curCSS" );
},
/**
* Get the text contents of all matched elements. The result is
* a string that contains the combined text contents of all matched
* elements. This method works on both HTML and XML documents.
*
* @example $("p").text();
* @before
Test Paragraph.
Paraparagraph
* @result Test Paragraph.Paraparagraph
* @desc Gets the concatenated text of all paragraphs
*
* @name text
* @type String
* @cat DOM/Attributes
*/
/**
* Set the text contents of all matched elements.
*
* Similar to html(), but escapes HTML (replace "" with their
* HTML entities).
*
* @example $("p").text("Some new text.");
* @before
Test Paragraph.
* @result
Some new text.
* @desc Sets the text of all paragraphs.
*
* @example $("p").text("Some new text.", true);
* @before
Test Paragraph.
* @result
Some new text.
* @desc Sets the text of all paragraphs.
*
* @name text
* @type String
* @param String val The text value to set the contents of the element to.
* @cat DOM/Attributes
*/
text: function(e) {
if ( typeof e == "string" )
return this.empty().append( document.createTextNode( e ) );
var t = "";
jQuery.each( e || this, function(){
jQuery.each( this.childNodes, function(){
if ( this.nodeType != 8 )
t += this.nodeType != 1 ?
this.nodeValue : jQuery.fn.text([ this ]);
});
});
return t;
},
/**
* Wrap all matched elements with a structure of other elements.
* This wrapping process is most useful for injecting additional
* stucture into a document, without ruining the original semantic
* qualities of a document.
*
* This works by going through the first element
* provided (which is generated, on the fly, from the provided HTML)
* and finds the deepest ancestor element within its
* structure - it is that element that will en-wrap everything else.
*
* This does not work with elements that contain text. Any necessary text
* must be added after the wrapping is done.
*
* @example $("p").wrap("
* @before
Test Paragraph.
* @result
Test Paragraph.
*
* @name wrap
* @type jQuery
* @param String html A string of HTML, that will be created on the fly and wrapped around the target.
* @cat DOM/Manipulation
*/
/**
* Wrap all matched elements with a structure of other elements.
* This wrapping process is most useful for injecting additional
* stucture into a document, without ruining the original semantic
* qualities of a document.
*
* This works by going through the first element
* provided and finding the deepest ancestor element within its
* structure - it is that element that will en-wrap everything else.
*
* This does not work with elements that contain text. Any necessary text
* must be added after the wrapping is done.
*
* @example $("p").wrap( document.getElementById('content') );
* @before
Test Paragraph.
* @result
*
* @name wrap
* @type jQuery
* @param Element elem A DOM element that will be wrapped around the target.
* @cat DOM/Manipulation
*/
wrap: function() {
// The elements to wrap the target around
var a, args = arguments;
// Wrap each of the matched elements individually
return this.each(function(){
if ( !a )
a = jQuery.clean(args, this.ownerDocument);
// Clone the structure that we're using to wrap
var b = a[0].cloneNode(true);
// Insert it before the element to be wrapped
this.parentNode.insertBefore( b, this );
// Find the deepest point in the wrap structure
while ( b.firstChild )
b = b.firstChild;
// Move the matched element to within the wrap structure
b.appendChild( this );
});
},
/**
* Append content to the inside of every matched element.
*
* This operation is similar to doing an appendChild to all the
* specified elements, adding them into the document.
*
* @example $("p").append("Hello");
* @before
I would like to say:
* @result
I would like to say: Hello
* @desc Appends some HTML to all paragraphs.
*
* @example $("p").append( $("#foo")[0] );
* @before
I would like to say:
Hello* @result
I would like to say: Hello
* @desc Appends an Element to all paragraphs.
*
* @example $("p").append( $("b") );
* @before
I would like to say:
Hello* @result
I would like to say: Hello
* @desc Appends a jQuery object (similar to an Array of DOM Elements) to all paragraphs.
*
* @name append
* @type jQuery
* @param
* @cat DOM/Manipulation
* @see prepend(
* @see before(
* @see after(
*/
append: function() {
return this.domManip(arguments, true, 1, function(a){
this.appendChild( a );
});
},
/**
* Prepend content to the inside of every matched element.
*
* This operation is the best way to insert elements
* inside, at the beginning, of all matched elements.
*
* @example $("p").prepend("Hello");
* @before
I would like to say:
* @result
HelloI would like to say:
* @desc Prepends some HTML to all paragraphs.
*
* @example $("p").prepend( $("#foo")[0] );
* @before
I would like to say:
Hello* @result
HelloI would like to say:
* @desc Prepends an Element to all paragraphs.
*
* @example $("p").prepend( $("b") );
* @before
I would like to say:
Hello* @result
HelloI would like to say:
* @desc Prepends a jQuery object (similar to an Array of DOM Elements) to all paragraphs.
*
* @name prepend
* @type jQuery
* @param
* @cat DOM/Manipulation
* @see append(
* @see before(
* @see after(
*/
prepend: function() {
return this.domManip(arguments, true, -1, function(a){
this.insertBefore( a, this.firstChild );
});
},
/**
* Insert content before each of the matched elements.
*
* @example $("p").before("Hello");
* @before
I would like to say:
* @result Hello
I would like to say:
* @desc Inserts some HTML before all paragraphs.
*
* @example $("p").before( $("#foo")[0] );
* @before
I would like to say:
Hello* @result Hello
I would like to say:
* @desc Inserts an Element before all paragraphs.
*
* @example $("p").before( $("b") );
* @before
I would like to say:
Hello* @result Hello
I would like to say:
* @desc Inserts a jQuery object (similar to an Array of DOM Elements) before all paragraphs.
*
* @name before
* @type jQuery
* @param
* @cat DOM/Manipulation
* @see append(
* @see prepend(
* @see after(
*/
before: function() {
return this.domManip(arguments, false, 1, function(a){
this.parentNode.insertBefore( a, this );
});
},
/**
* Insert content after each of the matched elements.
*
* @example $("p").after("Hello");
* @before
I would like to say:
* @result
I would like to say:
Hello* @desc Inserts some HTML after all paragraphs.
*
* @example $("p").after( $("#foo")[0] );
* @before Hello
I would like to say:
* @result
I would like to say:
Hello* @desc Inserts an Element after all paragraphs.
*
* @example $("p").after( $("b") );
* @before Hello
I would like to say:
* @result
I would like to say:
Hello* @desc Inserts a jQuery object (similar to an Array of DOM Elements) after all paragraphs.
*
* @name after
* @type jQuery
* @param
* @cat DOM/Manipulation
* @see append(
* @see prepend(
* @see before(
*/
after: function() {
return this.domManip(arguments, false, -1, function(a){
this.parentNode.insertBefore( a, this.nextSibling );
});
},
/**
* Revert the most recent 'destructive' operation, changing the set of matched elements
* to its previous state (right before the destructive operation).
*
* If there was no destructive operation before, an empty set is returned.
*
* A 'destructive' operation is any operation that changes the set of
* matched jQuery elements. These functions are: add
,
* children
, clone
, filter
,
* find
, not
, next
,
* parent
, parents
, prev
and siblings
.
*
* @example $("p").find("span").end();
* @before
Hello, how are you?
* @result [
...
]* @desc Selects all paragraphs, finds span elements inside these, and reverts the
* selection back to the paragraphs.
*
* @name end
* @type jQuery
* @cat DOM/Traversing
*/
end: function() {
return this.prevObject || jQuery([]);
},
/**
* Searches for all elements that match the specified expression.
* This method is a good way to find additional descendant
* elements with which to process.
*
* All searching is done using a jQuery expression. The expression can be
* written using CSS 1-3 Selector syntax, or basic XPath.
*
* @example $("p").find("span");
* @before
Hello, how are you?
* @result [ Hello ]
* @desc Starts with all paragraphs and searches for descendant span
* elements, same as $("p span")
*
* @name find
* @type jQuery
* @param String expr An expression to search with.
* @cat DOM/Traversing
*/
find: function(t) {
return this.pushStack( jQuery.unique( jQuery.map( this, function(a){
return jQuery.find(t,a);
}) ), t );
},
/**
* Clone matched DOM Elements and select the clones.
*
* This is useful for moving copies of the elements to another
* location in the DOM.
*
* @example $("b").clone().prependTo("p");
* @before Hello
, how are you?
* @result Hello
Hello, how are you?
* @desc Clones all b elements (and selects the clones) and prepends them to all paragraphs.
*
* @name clone
* @type jQuery
* @param Boolean deep (Optional) Set to false if you don't want to clone all descendant nodes, in addition to the element itself.
* @cat DOM/Manipulation
*/
clone: function(deep) {
// Need to remove events on the element and its descendants
var $this = this.add(this.find("*"));
$this.each(function() {
this._$events = {};
for (var type in this.$events)
this._$events[type] = jQuery.extend({},this.$events[type]);
}).unbind();
// Do the clone
var r = this.pushStack( jQuery.map( this, function(a){
return a.cloneNode( deep != undefined ? deep : true );
}) );
// Add the events back to the original and its descendants
$this.each(function() {
var events = this._$events;
for (var type in events)
for (var handler in events[type])
jQuery.event.add(this, type, events[type][handler], events[type][handler].data);
this._$events = null;
});
// Return the cloned set
return r;
},
/**
* Removes all elements from the set of matched elements that do not
* match the specified expression(s). This method is used to narrow down
* the results of a search.
*
* Provide a comma-separated list of expressions to apply multiple filters at once.
*
* @example $("p").filter(".selected")
* @before
Hello
How are you?
* @result [
Hello
]* @desc Selects all paragraphs and removes those without a class "selected".
*
* @example $("p").filter(".selected, :first")
* @before
Hello
Hello Again
And Again
* @result [
Hello
,And Again
]* @desc Selects all paragraphs and removes those without class "selected" and being the first one.
*
* @name filter
* @type jQuery
* @param String expression Expression(s) to search with.
* @cat DOM/Traversing
*/
/**
* Removes all elements from the set of matched elements that do not
* pass the specified filter. This method is used to narrow down
* the results of a search.
*
* @example $("p").filter(function(index) {
* return $("ol", this).length == 0;
* })
* @before
- Hello
How are you?
* @result [
How are you?
]* @desc Remove all elements that have a child ol element
*
* @name filter
* @type jQuery
* @param Function filter A function to use for filtering
* @cat DOM/Traversing
*/
filter: function(t) {
return this.pushStack(
jQuery.isFunction( t ) &&
jQuery.grep(this, function(el, index){
return t.apply(el, [index])
}) ||
jQuery.multiFilter(t,this) );
},
/**
* Removes the specified Element from the set of matched elements. This
* method is used to remove a single Element from a jQuery object.
*
* @example $("p").not( $("#selected")[0] )
* @before
Hello
Hello Again
* @result [
Hello
]* @desc Removes the element with the ID "selected" from the set of all paragraphs.
*
* @name not
* @type jQuery
* @param Element el An element to remove from the set
* @cat DOM/Traversing
*/
/**
* Removes elements matching the specified expression from the set
* of matched elements. This method is used to remove one or more
* elements from a jQuery object.
*
* @example $("p").not("#selected")
* @before
Hello
Hello Again
* @result [
Hello
]* @desc Removes the element with the ID "selected" from the set of all paragraphs.
*
* @name not
* @type jQuery
* @param String expr An expression with which to remove matching elements
* @cat DOM/Traversing
*/
/**
* Removes any elements inside the array of elements from the set
* of matched elements. This method is used to remove one or more
* elements from a jQuery object.
*
* Please note: the expression cannot use a reference to the
* element name. See the two examples below.
*
* @example $("p").not( $("div p.selected") )
* @before
Hello
Hello Again
* @result [
Hello
]* @desc Removes all elements that match "div p.selected" from the total set of all paragraphs.
*
* @name not
* @type jQuery
* @param jQuery elems A set of elements to remove from the jQuery set of matched elements.
* @cat DOM/Traversing
*/
not: function(t) {
return this.pushStack(
t.constructor == String &&
jQuery.multiFilter(t, this, true) ||
jQuery.grep(this, function(a) {
return ( t.constructor == Array || t.jquery )
? jQuery.inArray( a, t ) : a != t;
})
);
},
/**
* Adds more elements, matched by the given expression,
* to the set of matched elements.
*
* @example $("p").add("span")
* @before (HTML)
Hello
Hello Again* @result (jQuery object matching 2 elements) [
Hello
, Hello Again ]* @desc Compare the above result to the result of
$('p')
,* which would just result in
<nowiki>[ <p>Hello</p> ]</nowiki>
.* Using add(), matched elements of
$('span')
are simply* added to the returned jQuery-object.
*
* @name add
* @type jQuery
* @param String expr An expression whose matched elements are added
* @cat DOM/Traversing
*/
/**
* Adds more elements, created on the fly, to the set of
* matched elements.
*
* @example $("p").add("Again")
* @before
Hello
* @result [
Hello
, Again ]*
* @name add
* @type jQuery
* @param String html A string of HTML to create on the fly.
* @cat DOM/Traversing
*/
/**
* Adds one or more Elements to the set of matched elements.
*
* @example $("p").add( document.getElementById("a") )
* @before
Hello
Hello Again
* @result [
Hello
, Hello Again ]*
* @example $("p").add( document.forms[0].elements )
* @before
Hello
* @result [
Hello
, , ]*
* @name add
* @type jQuery
* @param Element|Array
* @cat DOM/Traversing
*/
add: function(t) {
return this.pushStack( jQuery.merge(
this.get(),
t.constructor == String ?
jQuery(t).get() :
t.length != undefined && (!t.nodeName || t.nodeName == "FORM") ?
t : [t] )
);
},
/**
* Checks the current selection against an expression and returns true,
* if at least one element of the selection fits the given expression.
*
* Does return false, if no element fits or the expression is not valid.
*
* filter(String) is used internally, therefore all rules that apply there
* apply here, too.
*
* @example $("input[@type='checkbox']").parent().is("form")
* @before
* @result true
* @desc Returns true, because the parent of the input is a form element
*
* @example $("input[@type='checkbox']").parent().is("form")
* @before
* @result false
* @desc Returns false, because the parent of the input is a p element
*
* @name is
* @type Boolean
* @param String expr The expression with which to filter
* @cat DOM/Traversing
*/
is: function(expr) {
return expr ? jQuery.multiFilter(expr,this).length > 0 : false;
},
/**
* Get the content of the value attribute of the first matched element.
*
* Use caution when relying on this function to check the value of
* multiple-select elements and checkboxes in a form. While it will
* still work as intended, it may not accurately represent the value
* the server will receive because these elements may send an array
* of values. For more robust handling of field values, see the
* [http://www.malsup.com/jquery/form/#fields fieldValue function of the Form Plugin].
*
* @example $("input").val();
* @before
* @result "some text"
*
* @name val
* @type String
* @cat DOM/Attributes
*/
/**
* Set the value attribute of every matched element.
*
* @example $("input").val("test");
* @before
* @result
*
* @name val
* @type jQuery
* @param String val Set the property to the specified value.
* @cat DOM/Attributes
*/
val: function( val ) {
return val == undefined ?
( this.length ? this[0].value : null ) :
this.attr( "value", val );
},
/**
* Get the html contents of the first matched element.
* This property is not available on XML documents.
*
* @example $("div").html();
* @before
* @result
*
* @name html
* @type String
* @cat DOM/Attributes
*/
/**
* Set the html contents of every matched element.
* This property is not available on XML documents.
*
* @example $("div").html("new stuff");
* @before
* @result
*
* @name html
* @type jQuery
* @param String val Set the html contents to the specified value.
* @cat DOM/Attributes
*/
html: function( val ) {
return val == undefined ?
( this.length ? this[0].innerHTML : null ) :
this.empty().append( val );
},
/**
* @private
* @name domManip
* @param Array args
* @param Boolean table Insert TBODY in TABLEs if one is not found.
* @param Number dir If dir * @param Function fn The function doing the DOM manipulation.
* @type jQuery
* @cat Core
*/
domManip: function(args, table, dir, fn){
var clone = this.length > 1, a;
return this.each(function(){
if ( !a ) {
a = jQuery.clean(args, this.ownerDocument);
if ( dir a.reverse();
}
var obj = this;
if ( table && jQuery.nodeName(this, "table") && jQuery.nodeName(a[0], "tr") )
obj = this.getElementsByTagName("tbody")[0] || this.appendChild(document.createElement("tbody"));
jQuery.each( a, function(){
fn.apply( obj, [ clone ? this.cloneNode(true) : this ] );
});
});
}
};
/**
* Extends the jQuery object itself. Can be used to add functions into
* the jQuery namespace and to [[Plugins/Authoring|add plugin methods]] (plugins).
*
* @example jQuery.fn.extend({
* check: function() {
* return this.each(function() { this.checked = true; });
* },
* uncheck: function() {
* return this.each(function() { this.checked = false; });
* }
* });
* $("input[@type=checkbox]").check();
* $("input[@type=radio]").uncheck();
* @desc Adds two plugin methods.
*
* @example jQuery.extend({
* min: function(a, b) { return a * max: function(a, b) { return a > b ? a : b; }
* });
* @desc Adds two functions into the jQuery namespace
*
* @name $.extend
* @param Object prop The object that will be merged into the jQuery object
* @type Object
* @cat Core
*/
/**
* Extend one object with one or more others, returning the original,
* modified, object. This is a great utility for simple inheritance.
*
* @example var settings = { validate: false, limit: 5, name: "foo" };
* var options = { validate: true, name: "bar" };
* jQuery.extend(settings, options);
* @result settings == { validate: true, limit: 5, name: "bar" }
* @desc Merge settings and options, modifying settings
*
* @example var defaults = { validate: false, limit: 5, name: "foo" };
* var options = { validate: true, name: "bar" };
* var settings = jQuery.extend({}, defaults, options);
* @result settings == { validate: true, limit: 5, name: "bar" }
* @desc Merge defaults and options, without modifying the defaults
*
* @name $.extend
* @param Object target The object to extend
* @param Object prop1 The object that will be merged into the first.
* @param Object propN (optional) More objects to merge into the first
* @type Object
* @cat JavaScript
*/
jQuery.extend = jQuery.fn.extend = function() {
// copy reference to target object
var target = arguments[0], a = 1;
// extend jQuery itself if only one argument is passed
if ( arguments.length == 1 ) {
target = this;
a = 0;
}
var prop;
while ( (prop = arguments[a++]) != null )
// Extend the base object
for ( var i in prop ) target[i] = prop[i];
// Return the modified object
return target;
};
jQuery.extend({
/**
* Run this function to give control of the $ variable back
* to whichever library first implemented it. This helps to make
* sure that jQuery doesn't conflict with the $ object
* of other libraries.
*
* By using this function, you will only be able to access jQuery
* using the 'jQuery' variable. For example, where you used to do
* $("div p"), you now must do jQuery("div p").
*
* @example jQuery.noConflict();
* // Do something with jQuery
* jQuery("div p").hide();
* // Do something with another library's $()
* $("content").style.display = 'none';
* @desc Maps the original object that was referenced by $ back to $
*
* @example jQuery.noConflict();
* (function($) {
* $(function() {
* // more code using $ as alias to jQuery
* });
* })(jQuery);
* // other code using $ as an alias to the other library
* @desc Reverts the $ alias and then creates and executes a
* function to provide the $ as a jQuery alias inside the functions
* scope. Inside the function the original $ object is not available.
* This works well for most plugins that don't rely on any other library.
*
*
* @name $.noConflict
* @type undefined
* @cat Core
*/
noConflict: function() {
if ( jQuery._$ )
$ = jQuery._$;
return jQuery;
},
// This may seem like some crazy code, but trust me when I say that this
// is the only cross-browser way to do this. --John
isFunction: function( fn ) {
return !!fn && typeof fn != "string" && !fn.nodeName &&
fn.constructor != Array && /function/i.test( fn + "" );
},
// check if an element is in a XML document
isXMLDoc: function(elem) {
return elem.tagName && elem.ownerDocument && !elem.ownerDocument.body;
},
nodeName: function( elem, name ) {
return elem.nodeName && elem.nodeName.toUpperCase() == name.toUpperCase();
},
/**
* A generic iterator function, which can be used to seamlessly
* iterate over both objects and arrays. This function is not the same
* as $().each() - which is used to iterate, exclusively, over a jQuery
* object. This function can be used to iterate over anything.
*
* The callback has two arguments:the key (objects) or index (arrays) as first
* the first, and the value as the second.
*
* @example $.each( [0,1,2], function(i, n){
* alert( "Item #" + i + ": " + n );
* });
* @desc This is an example of iterating over the items in an array,
* accessing both the current item and its index.
*
* @example $.each( { name: "John", lang: "JS" }, function(i, n){
* alert( "Name: " + i + ", Value: " + n );
* });
*
* @desc This is an example of iterating over the properties in an
* Object, accessing both the current item and its key.
*
* @name $.each
* @param Object obj The object, or array, to iterate over.
* @param Function fn The function that will be executed on every object.
* @type Object
* @cat JavaScript
*/
// args is for internal usage only
each: function( obj, fn, args ) {
if ( obj.length == undefined )
for ( var i in obj )
fn.apply( obj[i], args || [i, obj[i]] );
else
for ( var i = 0, ol = obj.length; i if ( fn.apply( obj[i], args || [i, obj[i]] ) === false ) break;
return obj;
},
prop: function(elem, value, type, index, prop){
// Handle executable functions
if ( jQuery.isFunction( value ) )
value = value.call( elem, [index] );
// exclude the following css properties to add px
var exclude = /z-?index|font-?weight|opacity|zoom|line-?height/i;
// Handle passing in a number to a CSS property
return value && value.constructor == Number && type == "curCSS" && !exclude.test(prop) ?
value + "px" :
value;
},
className: {
// internal only, use addClass("class")
add: function( elem, c ){
jQuery.each( c.split(//s+/), function(i, cur){
if ( !jQuery.className.has( elem.className, cur ) )
elem.className += ( elem.className ? " " : "" ) + cur;
});
},
// internal only, use removeClass("class")
remove: function( elem, c ){
elem.className = c != undefined ?
jQuery.grep( elem.className.split(//s+/), function(cur){
return !jQuery.className.has( c, cur );
}).join(" ") : "";
},
// internal only, use is(".class")
has: function( t, c ) {
return jQuery.inArray( c, (t.className || t).toString().split(//s+/) ) > -1;
}
},
/**
* Swap in/out style options.
* @private
*/
swap: function(e,o,f) {
for ( var i in o ) {
e.style["old"+i] = e.style[i];
e.style[i] = o[i];
}
f.apply( e, [] );
for ( var i in o )
e.style[i] = e.style["old"+i];
},
css: function(e,p) {
if ( p == "height" || p == "width" ) {
var old = {}, oHeight, oWidth, d = ["Top","Bottom","Right","Left"];
jQuery.each( d, function(){
old["padding" + this] = 0;
old["border" + this + "Width"] = 0;
});
jQuery.swap( e, old, function() {
if ( jQuery(e).is(':visible') ) {
oHeight = e.offsetHeight;
oWidth = e.offsetWidth;
} else {
e = jQuery(e.cloneNode(true))
.find(":radio").removeAttr("checked").end()
.css({
visibility: "hidden", position: "absolute", display: "block", right: "0", left: "0"
}).appendTo(e.parentNode)[0];
var parPos = jQuery.css(e.parentNode,"position") || "static";
if ( parPos == "static" )
e.parentNode.style.position = "relative";
oHeight = e.clientHeight;
oWidth = e.clientWidth;
if ( parPos == "static" )
e.parentNode.style.position = "static";
e.parentNode.removeChild(e);
}
});
return p == "height" ? oHeight : oWidth;
}
return jQuery.curCSS( e, p );
},
curCSS: function(elem, prop, force) {
var ret;
if (prop == "opacity" && jQuery.browser.msie) {
ret = jQuery.attr(elem.style, "opacity");
return ret == "" ? "1" : ret;
}
if (prop.match(/float/i))
prop = jQuery.browser.msie ? "styleFloat" : "cssFloat";
if (!force && elem.style[prop])
ret = elem.style[prop];
else if (document.defaultView && document.defaultView.getComputedStyle) {
if (prop.match(/float/i))
prop = "float";
prop = prop.replace(/([A-Z])/g,"-$1").toLowerCase();
var cur = document.defaultView.getComputedStyle(elem, null);
if ( cur )
ret = cur.getPropertyValue(prop);
else if ( prop == "display" )
ret = "none";
else
jQuery.swap(elem, { display: "block" }, function() {
var c = document.defaultView.getComputedStyle(this, "");
ret = c && c.getPropertyValue(prop) || "";
});
} else if (elem.currentStyle) {
var newProp = prop.replace(//-(/w)/g,function(m,c){return c.toUpperCase();});
ret = elem.currentStyle[prop] || elem.currentStyle[newProp];
}
return ret;
},
clean: function(a, doc) {
var r = [];
doc = doc || document;
jQuery.each( a, function(i,arg){
if ( !arg ) return;
if ( arg.constructor == Number )
arg = arg.toString();
// Convert html string into DOM nodes
 

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

Atom编辑器mac版下载
最流行的的开源编辑器

SublimeText3 英文版
推荐:为Win版本,支持代码提示!
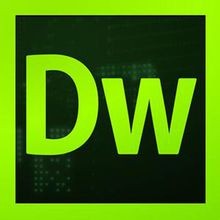
Dreamweaver CS6
视觉化网页开发工具
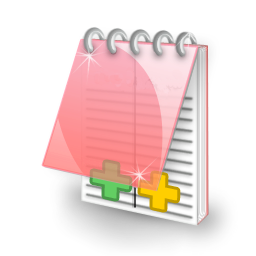
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

DVWA
Damn Vulnerable Web App (DVWA) 是一个PHP/MySQL的Web应用程序,非常容易受到攻击。它的主要目标是成为安全专业人员在合法环境中测试自己的技能和工具的辅助工具,帮助Web开发人员更好地理解保护Web应用程序的过程,并帮助教师/学生在课堂环境中教授/学习Web应用程序安全。DVWA的目标是通过简单直接的界面练习一些最常见的Web漏洞,难度各不相同。请注意,该软件中