设计与实现Golang中链表的数据结构
引言:
链表是一种常见的数据结构,用于存储一系列的节点。每个节点包含数据和指向下一个节点的指针。在Golang中,我们可以通过使用结构体和指针来实现链表。
- 链表的设计与结构定义
在Golang中,我们可以使用结构体和指针来定义链表的节点和链表本身的结构。节点结构体包含一个数据字段和一个指向下一个节点的指针。
type Node struct { data interface{} // 存储数据 next *Node // 指向下一个节点的指针 } type LinkedList struct { head *Node // 链表头节点的指针 }
- 链表的初始化
在创建链表时,我们需要初始化一个空链表。初始化链表时,链表头节点指针为空。
func NewLinkedList() *LinkedList { return &LinkedList{} }
- 链表的插入
链表的插入操作将节点添加到链表的尾部。首先,我们需要创建一个新节点,并将数据赋值给它。然后,我们找到链表的最后一个节点,并将其next
指针指向新节点。next
指针指向新节点。
func (list *LinkedList) Insert(data interface{}) { newNode := &Node{data: data} // 创建新节点 if list.head == nil { // 链表为空 list.head = newNode // 直接将新节点设为头节点 } else { current := list.head for current.next != nil { current = current.next // 找到链表的最后一个节点 } current.next = newNode // 将新节点链接到最后一个节点的next指针 } }
- 链表的删除
链表的删除操作将找到并删除链表中特定节点。首先,我们需要找到要删除的节点的前一个节点,并将其next
指针设置为被删除节点的next
-
func (list *LinkedList) Traverse() { if list.head == nil { return // 链表为空 } current := list.head for current != nil { fmt.Println(current.data) current = current.next } }
func main() { list := NewLinkedList() // 创建一个新链表 list.Insert(1) // 插入节点1 list.Insert(2) // 插入节点2 list.Insert(3) // 插入节点3 list.Traverse() // 遍历链表,输出: 1 2 3 list.Delete(2) // 删除节点2 list.Traverse() // 遍历链表,输出: 1 3 }
func (list *LinkedList) Delete(data interface{}) { if list.head == nil { return // 链表为空,无需删除 } if list.head.data == data { // 头节点需要删除 list.head = list.head.next return } current := list.head for current.next != nil { if current.next.data == data { // 找到要删除节点的前一个节点 current.next = current.next.next return } current = current.next } }
- 链表的删除操作将找到并删除链表中特定节点。首先,我们需要找到要删除的节点的前一个节点,并将其
next
指针设置为被删除节点的next
指针。- 链表的遍历操作将打印链表中的所有节点。
链表的使用示例
以上是设计与实现Golang中链表的数据结构的详细内容。更多信息请关注PHP中文网其他相关文章!
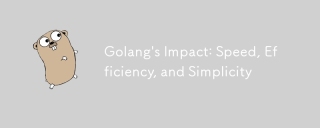
GoimpactsdevelopmentPositationalityThroughSpeed,效率和模拟性。1)速度:gocompilesquicklyandrunseff,ifealforlargeprojects.2)效率:效率:ITScomprehenSevestAndArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdEcceSteral Depentencies,增强开发的简单性:3)SimpleflovelmentIcties:3)简单性。
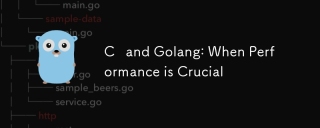
C 更适合需要直接控制硬件资源和高性能优化的场景,而Golang更适合需要快速开发和高并发处理的场景。1.C 的优势在于其接近硬件的特性和高度的优化能力,适合游戏开发等高性能需求。2.Golang的优势在于其简洁的语法和天然的并发支持,适合高并发服务开发。
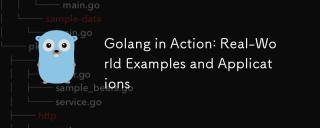
Golang在实际应用中表现出色,以简洁、高效和并发性着称。 1)通过Goroutines和Channels实现并发编程,2)利用接口和多态编写灵活代码,3)使用net/http包简化网络编程,4)构建高效并发爬虫,5)通过工具和最佳实践进行调试和优化。
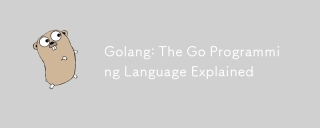
Go语言的核心特性包括垃圾回收、静态链接和并发支持。1.Go语言的并发模型通过goroutine和channel实现高效并发编程。2.接口和多态性通过实现接口方法,使得不同类型可以统一处理。3.基本用法展示了函数定义和调用的高效性。4.高级用法中,切片提供了动态调整大小的强大功能。5.常见错误如竞态条件可以通过gotest-race检测并解决。6.性能优化通过sync.Pool重用对象,减少垃圾回收压力。
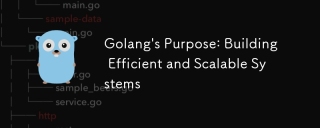
Go语言在构建高效且可扩展的系统中表现出色,其优势包括:1.高性能:编译成机器码,运行速度快;2.并发编程:通过goroutines和channels简化多任务处理;3.简洁性:语法简洁,降低学习和维护成本;4.跨平台:支持跨平台编译,方便部署。
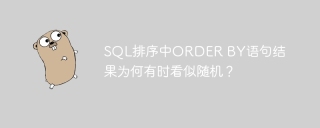
关于SQL查询结果排序的疑惑学习SQL的过程中,常常会遇到一些令人困惑的问题。最近,笔者在阅读《MICK-SQL基础�...
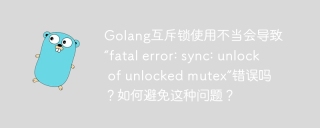
golang ...


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

SublimeText3 Linux新版
SublimeText3 Linux最新版
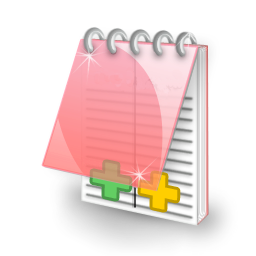
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

PhpStorm Mac 版本
最新(2018.2.1 )专业的PHP集成开发工具
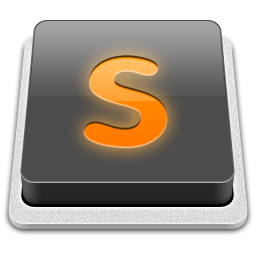
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

记事本++7.3.1
好用且免费的代码编辑器