如何在Java中实现分布式系统的数据复制和数据同步
随着分布式系统的兴起,数据复制和数据同步成为保障数据一致性和可靠性的重要手段。在Java中,我们可以利用一些常见的框架和技术来实现分布式系统的数据复制和数据同步。本文将详细介绍如何利用Java实现分布式系统中的数据复制和数据同步,并给出具体的代码示例。
一、数据复制
数据复制是将数据从一个节点复制到另一个节点的过程,旨在提高数据的可靠性和容灾能力。在Java中,我们可以利用一些常见的技术来实现数据的复制。
- 数据库复制
数据库是实现数据复制的常用手段之一。在大多数分布式系统中,数据通常存储在数据库中,并通过数据库的复制机制实现数据的复制。Java中有很多数据库管理系统(DBMS)可供选择,包括MySQL、Oracle等。这些DBMS提供了复制机制,可以将数据从一个节点复制到其他节点。
以下是一个使用MySQL数据库进行数据复制的示例代码:
import java.sql.*; public class DataReplication { public static void main(String[] args) { try { Class.forName("com.mysql.jdbc.Driver"); Connection sourceConn = DriverManager.getConnection( "jdbc:mysql://sourceDBIP/sourceDB?user=root&password=123456"); Connection targetConn = DriverManager.getConnection( "jdbc:mysql://targetDBIP/targetDB?user=root&password=123456"); Statement sourceStatement = sourceConn.createStatement(); Statement targetStatement = targetConn.createStatement(); ResultSet rs = sourceStatement.executeQuery("SELECT * FROM data"); while (rs.next()) { int id = rs.getInt("id"); String name = rs.getString("name"); targetStatement.executeUpdate("INSERT INTO data (id, name) VALUES (" + id + ", '" + name + "')"); } rs.close(); sourceStatement.close(); targetStatement.close(); sourceConn.close(); targetConn.close(); System.out.println("数据复制完成!"); } catch (Exception e) { e.printStackTrace(); } } }
- 文件复制
另一种常见的数据复制方式是文件复制。可以通过Java中的文件操作相关API来实现文件的复制。在分布式系统中,可以将数据以文件的形式存储在不同节点上,并通过文件复制的方式实现数据的复制。
以下是一个使用Java进行文件复制的示例代码:
import java.io.*; public class DataReplication { public static void main(String[] args) { try { File sourceFile = new File("source.txt"); File targetFile = new File("target.txt"); FileInputStream fis = new FileInputStream(sourceFile); FileOutputStream fos = new FileOutputStream(targetFile); byte[] buffer = new byte[1024]; int len; while ((len = fis.read(buffer)) != -1) { fos.write(buffer, 0, len); } fis.close(); fos.close(); System.out.println("数据复制完成!"); } catch (IOException e) { e.printStackTrace(); } } }
二、数据同步
数据同步是指将不同节点中的数据保持一致的过程。在分布式系统中,由于各个节点之间的并发操作,数据不可避免地会出现不一致的情况。为了解决这个问题,可以利用一些技术来实现数据的同步。
- ZooKeeper
ZooKeeper是一个分布式协调服务,可以用于实现数据的同步。它提供了多种特性,如临时节点、监听机制等,可以帮助我们实现分布式系统中的数据同步。
以下是一个使用ZooKeeper实现数据同步的示例代码:
import org.apache.zookeeper.*; import java.util.concurrent.CountDownLatch; public class DataSynchronization { private static final String ZK_ADDRESS = "127.0.0.1:2181"; private static final String ZK_PATH = "/data"; public static void main(String[] args) { try { CountDownLatch connectedSemaphore = new CountDownLatch(1); ZooKeeper zk = new ZooKeeper(ZK_ADDRESS, 5000, new Watcher() { @Override public void process(WatchedEvent event) { if (event.getType() == Event.EventType.None && event.getState() == Event.KeeperState.SyncConnected) { connectedSemaphore.countDown(); } } }); connectedSemaphore.await(); byte[] data = zk.getData(ZK_PATH, true, null); String strData = new String(data); System.out.println("获取到的数据:" + strData); zk.close(); System.out.println("数据同步完成!"); } catch (Exception e) { e.printStackTrace(); } } }
- Redis
Redis是一个开源的内存数据结构存储系统,它可以用作分布式系统中数据的缓存和同步。Redis提供了publish/subscribe机制,可以帮助我们实现数据的同步。
以下是一个使用Redis实现数据同步的示例代码:
import redis.clients.jedis.Jedis; import redis.clients.jedis.JedisPubSub; public class DataSynchronization { private static final String CHANNEL_NAME = "dataChannel"; public static void main(String[] args) { Jedis jedis = new Jedis("localhost"); Thread subscriberThread = new Thread(() -> { Jedis jedisSubscriber = new Jedis("localhost"); jedisSubscriber.subscribe(new JedisPubSub() { @Override public void onMessage(String channel, String message) { System.out.println("收到的数据:" + message); } }, CHANNEL_NAME); }); subscriberThread.start(); Thread publisherThread = new Thread(() -> { for (int i = 0; i < 10; i++) { jedis.publish(CHANNEL_NAME, "data" + i); } }); publisherThread.start(); } }
通过上述代码示例,我们可以看到如何利用Java实现分布式系统中的数据复制和数据同步。无论是数据库复制还是文件复制,还是通过ZooKeeper或Redis等工具实现数据同步,都可以根据具体的需求选择合适的方法。希望这篇文章对你理解分布式系统中的数据复制和数据同步有所帮助。
以上是如何在Java中实现分布式系统的数据复制和数据同步的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具
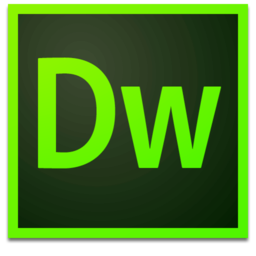
Dreamweaver Mac版
视觉化网页开发工具

记事本++7.3.1
好用且免费的代码编辑器

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。