MongoDB 是一个著名的 NoSQL 数据库,它提供了一种可扩展且灵活的方法来存储和检索数据,还可以通过 Python(一种多功能编程语言)访问数据库集合。将 MongoDB 与 Python 集成使开发人员能够轻松地与其数据库集合进行交互。
本文深入解释了如何使用 Python 访问 MongoDB 集合。它涵盖了连接、查询和操作数据所需的步骤、语法和技术。
皮蒙戈
PyMongo 是一个 Python 库,充当官方 MongoDB 驱动程序。它提供了一个简单直观的界面,可以通过 Python 应用程序与 MongoDB 数据库进行交互。
PyMongo 由 MongoDB 组织开发和维护,允许 Python 开发人员无缝连接到 MongoDB 并执行各种数据库操作。它利用了 MongoDB 的强大功能,例如灵活的面向文档的数据模型、高可扩展性和丰富的查询功能。
如何使用 python 访问 MongoDB 中的集合?
按照下面给出的这些步骤,我们可以使用 Python 成功访问 MongoDB 中的集合并对存储在其中的数据执行各种操作 -
安装 MongoDB Python 驱动程序 - 首先安装 pymongo 包,这是 Python 的官方 MongoDB 驱动程序。您可以通过运行命令 pip install pymongo 来使用 pip 包管理器。
导入必要的模块 - 在Python脚本中,导入所需的模块,包括pymongo和MongoClient。 MongoClient 类提供连接 MongoDB 服务器的接口。
建立连接 - 使用 MongoClient 类创建 MongoDB 客户端对象,指定连接详细信息,例如主机名和端口号。如果 MongoDB 服务器在本地计算机上的默认端口 (27017) 上运行,则只需使用 client = MongoClient() 即可。
访问数据库 - 连接后,指定您要使用的数据库。使用客户端对象后跟数据库名称来访问它。例如,db = client.mydatabase 将允许您访问“mydatabase”数据库。
访问集合 - 现在您可以访问数据库,您可以通过使用 db 对象调用它来检索特定的集合。例如,collection = db.mycollection 将允许您在所选数据库中使用“mycollection”集合。
对集合执行操作 - 您现在可以使用集合对象提供的各种方法来执行操作,例如插入、更新、删除或查询集合中的文档。这些操作是使用 insert_one()、update_one()、delete_one() 或 find() 等函数完成的。
关闭连接 - 完成操作后,最好关闭 MongoDB 连接。使用客户端对象上的 close() 方法正常终止连接。
下面是一个示例程序,演示了如何使用 Python 访问 MongoDB 中的集合以及预期输出 -
示例
from pymongo import MongoClient # Establish a connection to the MongoDB server client = MongoClient() # Access the desired database db = client.mydatabase # Access the collection within the database collection = db.mycollection # Insert a document into the collection document = {"name": "John", "age": 30} collection.insert_one(document) print("Document inserted successfully.") # Retrieve documents from the collection documents = collection.find() print("Documents in the collection:") for doc in documents: print(doc) # Update a document in the collection collection.update_one({"name": "John"}, {"$set": {"age": 35}}) print("Document updated successfully.") # Retrieve the updated document updated_doc = collection.find_one({"name": "John"}) print("Updated Document:") print(updated_doc) # Delete a document from the collection collection.delete_one({"name": "John"}) print("Document deleted successfully.") # Verify the deletion by retrieving the document again deleted_doc = collection.find_one({"name": "John"}) print("Deleted Document:") print(deleted_doc) # Close the MongoDB connection client.close()
输出
Document inserted successfully. Documents in the collection: {'_id': ObjectId('646364820b3f42435e3ad5df'), 'name': 'John', 'age': 30} Document updated successfully. Updated Document: {'_id': ObjectId('646364820b3f42435e3ad5df'), 'name': 'John', 'age': 35} Document deleted successfully. Deleted Document: None
在上面的程序中,我们首先从 pymongo 模块导入 MongoClient 类。然后我们使用 client = MongoClient() 建立了到 MongoDB 服务器的连接。默认情况下,这会连接到在默认端口 (27017) 的本地主机上运行的 MongoDB 服务器。
接下来,我们通过将所需的数据库分配给 db 变量来访问它。在此示例中,我们假设数据库名为“mydatabase”。
之后,我们通过将集合分配给集合变量来访问数据库中的集合。在这里,我们假设该集合名为“mycollection”。
然后我们演示如何使用 insert_one() 方法将文档插入到集合中并打印成功消息。
为了从集合中检索文档,我们使用 find() 方法,该方法返回一个游标对象。我们遍历光标并打印每个文档。
我们还展示了使用 update_one() 方法更新集合中的文档并打印成功消息。
为了检索更新的文档,我们使用 find_one() 方法以及指定更新的文档字段的查询。我们打印更新后的文档。
我们演示了使用delete_one()方法从集合中删除文档并打印成功消息。为了验证删除,我们尝试使用 find_one() 方法再次检索文档并打印结果。
最后,我们使用 client.close() 关闭了 MongoDB 连接,以优雅地释放资源。
结论
总之,在 PyMongo 库的帮助下,使用 Python 访问 MongoDB 中的集合变得简单而高效。通过遵循本文中概述的步骤,开发人员可以无缝连接、查询和操作数据,从而在其 Python 项目中释放 MongoDB 的强大功能。
以上是如何使用Python访问MongoDB中的集合?的详细内容。更多信息请关注PHP中文网其他相关文章!
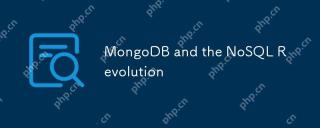
MongoDB是一种文档型NoSQL数据库,旨在提供高性能、易扩展和灵活的数据存储解决方案。1)它使用BSON格式存储数据,适合处理半结构化或非结构化数据。2)通过分片技术实现水平扩展,支持复杂查询和数据处理。3)在使用时需注意索引优化、数据建模和性能监控,以发挥其优势。
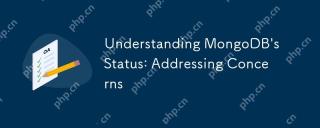
MongoDB适合项目需求,但需优化使用。1)性能:优化索引策略和使用分片技术。2)安全性:启用身份验证和数据加密。3)可扩展性:使用副本集和分片技术。
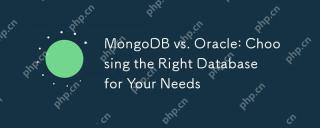
MongoDB适合非结构化数据和高扩展性需求,Oracle适合需要严格数据一致性的场景。1.MongoDB灵活存储不同结构数据,适合社交媒体和物联网。2.Oracle结构化数据模型确保数据完整性,适用于金融交易。3.MongoDB通过分片横向扩展,Oracle通过RAC纵向扩展。4.MongoDB维护成本低,Oracle维护成本高但支持完善。
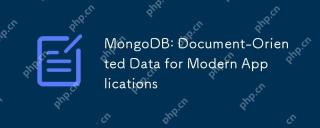
MongoDB通过其灵活的文档模型和高性能的存储引擎改变了开发方式。其优势包括:1.无模式设计,允许快速迭代;2.文档模型支持嵌套和数组,增强数据结构灵活性;3.自动分片功能支持水平扩展,适用于大规模数据处理。
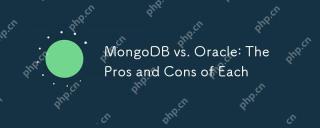
MongoDB适合快速迭代和处理大规模非结构化数据的项目,而Oracle适合需要高可靠性和复杂事务处理的企业级应用。 MongoDB以其灵活的文档存储和高效的读写操作着称,适用于现代web应用和大数据分析;Oracle则以其强大的数据管理能力和SQL支持着称,广泛应用于金融和电信等行业。
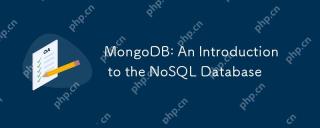
MongoDB是一种文档型NoSQL数据库,使用BSON格式存储数据,适合处理复杂和非结构化数据。1)其文档模型灵活,适用于变化频繁的数据结构。2)MongoDB使用WiredTiger存储引擎和查询优化器,支持高效的数据操作和查询。3)基本操作包括插入、查询、更新和删除文档。4)高级用法包括使用聚合框架进行复杂数据分析。5)常见错误包括连接问题、查询性能问题和数据一致性问题。6)性能优化和最佳实践包括索引优化、数据建模、分片、缓存和监控与调优。
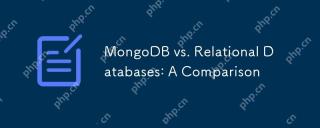
MongoDB适合需要灵活数据模型和高扩展性的场景,而关系型数据库更适合复杂查询和事务处理的应用。1)MongoDB的文档模型适应快速迭代的现代应用开发。2)关系型数据库通过表结构和SQL支持复杂查询和金融系统等事务处理。3)MongoDB通过分片实现水平扩展,适合大规模数据处理。4)关系型数据库依赖垂直扩展,适用于需要优化查询和索引的场景。
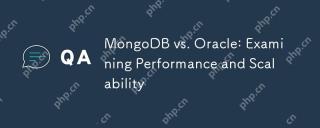
MongoDB在性能和可扩展性上表现出色,适合高扩展性和灵活性需求;Oracle则在需要严格事务控制和复杂查询时表现优异。1.MongoDB通过分片技术实现高扩展性,适合大规模数据和高并发场景。2.Oracle依赖优化器和并行处理提高性能,适合结构化数据和事务控制需求。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具
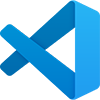
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器
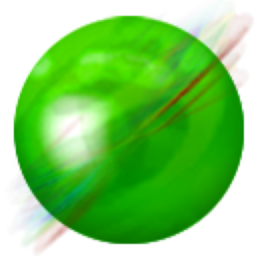
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。

记事本++7.3.1
好用且免费的代码编辑器

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),