在本文中,我们将向您展示什么是稀疏矩阵以及如何在 python 中创建稀疏矩阵。
什么是稀疏矩阵?
稀疏矩阵是大多数元素为0的矩阵。也就是说,矩阵仅包含少数位置的数据。稀疏矩阵消耗的大部分内存都是由零组成的。
例如 -
M = [ [1, 0, 0, 0], [0, 0, 3, 0], [0, 0, 0, 0], [0, 0, 0, 2] ]
使用二维数组来表示稀疏矩阵会浪费大量内存,因为矩阵中的零在大多数情况下都是无用的。因此,我们不是将零与非零元素一起保存,而是只存储非零元素。这涉及使用三元组来存储非零元素(行、列、值)。
自然语言处理(NLP)和数据编码都大量使用稀疏矩阵。如果大多数矩阵元素为 0,则存储所有矩阵元素的存储成本会很高。
这是因为我们只有几个数据点,并且大部分存储空间都被冗余零占用。
稀疏矩阵的优点
以下是使用稀疏矩阵而不是简单矩阵的两个主要优点 -
存储 - 因为非零元素比零少,所以可以使用更少的内存来单独存储这些元素。
计算时间 - 通过逻辑创建仅遍历非零元素的数据结构可以节省计算时间。
如何在Python中创建稀疏矩阵?
Python 中的 SciPy 提供了使用各种数据结构创建稀疏矩阵以及将稠密矩阵转换为稀疏矩阵的工具。
在Python中,我们可以使用以下函数创建稀疏矩阵 -
csr_matrix() 函数 - 以压缩稀疏行格式创建稀疏矩阵,
csc_matrix() 函数 - 以压缩稀疏列格式创建稀疏矩阵。,,
方法1.使用csr_matrix()函数创建稀疏矩阵
它以压缩稀疏行格式创建稀疏矩阵。
语法
scipy.sparse.csr_matrix(shape=None, dtype=None)
参数
shape - 它是矩阵的形状
dtype - 它是矩阵的数据类型
算法(步骤)
以下是执行所需任务所需遵循的算法/步骤 -
使用 import 关键字,导入带有别名 (np) 的 numpy 模块。
使用 import 关键字,从 scipy 模块导入 csr_matrix 函数。
使用csr_matrix()函数创建int数据类型的3 * 3稀疏矩阵(row格式)并使用toarray转换为数组() 函数。
打印生成的稀疏矩阵。
示例
以下程序使用 csr_matrix() 函数返回稀疏矩阵 (3x3) -
# importing numpy module with an alias name import numpy as np # importing csr_matrix function from scipy module from scipy.sparse import csr_matrix # Using csr_matrix function to create a 3 * 3 sparse matrix of int datatype # and converting into array sparse_matrix = csr_matrix((3, 3), dtype = np.int8).toarray() # printing the resultant sparse matrix print("The resultant sparse matrix:\n", sparse_matrix)
输出
执行时,上述程序将生成以下输出 -
The resultant sparse matrix: [[0 0 0] [0 0 0] [0 0 0]]
方法 2. 使用给定 Numpy 数组的 csr_matrix() 函数创建稀疏矩阵
算法(步骤)
以下是执行所需任务所需遵循的算法/步骤 -
使用 import 关键字,导入带有别名 (np) 的 numpy 模块。
使用 import 关键字,从 scipy 模块导入 csr_matrix 函数。
使用numpy.array()函数创建数组(返回一个ndarray。ndarray是满足给定要求的数组对象)
示例
# importing numpy module with alias name import numpy as np # importing csr_matrix function from scipy module from scipy.sparse import csr_matrix # Giving rows and columns values rows = np.array([0, 1, 0, 2, 1, 1]) columns = np.array([1, 0, 0, 2, 1, 2]) # Giving array data arrayData = np.array([1, 3, 2, 5, 7, 6]) # Using csr_matrix function to create a 3x3 sparse matrix sparse_matrix = csr_matrix((arrayData, (rows, columns)), shape = (3, 3)).toarray() # print the resultant sparse matrix print("The resultant sparse matrix:\n", sparse_matrix)
输出
执行时,上述程序将生成以下输出 -
The resultant sparse matrix: [[2 1 0] [3 7 6] [0 0 5]]
方法 3.使用 csc_matrix() 函数创建稀疏矩阵
它以压缩稀疏列格式创建稀疏矩阵。
语法
scipy.sparse.csc_matrix(shape=None, dtype=None)
参数
shape - 它是矩阵的形状
dtype - 它是矩阵的数据类型
算法
以下是执行所需任务所需遵循的算法/步骤 -
使用 import 关键字,导入带有别名 (np) 的 numpy 模块。
使用 import 关键字,从 scipy 模块导入 csc_matrix 函数。
使用csc_matrix()函数创建int数据类型的3 * 3稀疏矩阵(列格式)并使用toarray转换为数组() 函数。
打印生成的稀疏矩阵。
示例
以下程序使用 csc_matrix() 函数以列格式返回稀疏矩阵 (3x3) -
# importing numpy module with an alias name import numpy as np # importing csc_matrix function from scipy module from scipy.sparse import csc_matrix # Using csc_matrix function to create a 3 * 3 sparse matrix of int datatype # and converting into array sparse_matrix = csc_matrix((3, 3), dtype = np.int8).toarray() # printing the resultant sparse matrix print("The resultant sparse matrix:\n", sparse_matrix)
输出
执行时,上述程序将生成以下输出 -
The resultant sparse matrix: [[0 0 0] [0 0 0] [0 0 0]]
方法 4. 使用给定 Numpy 数组的 csc_matrix() 函数创建稀疏矩阵
示例
以下程序使用 csc_matrix() 函数以整数列格式返回稀疏矩阵 (3x3) -
import numpy as np # importing csc_matrix function from scipy module from scipy.sparse import csc_matrix # Giving rows and columns values rows = np.array([0, 1, 0, 2, 1, 1]) columns = np.array([1, 0, 0, 2, 1, 2]) # Giving array data arrayData = np.array([1, 3, 2, 5, 7, 6]) # Using csc_matrix function to create a 3x3 sparse matrix in column format sparse_matrix = csc_matrix((arrayData, (rows, columns)), shape = (3, 3)).toarray() # print the resultant sparse matrix print("The resultant sparse matrix:\n", sparse_matrix)
输出
执行时,上述程序将生成以下输出 -
The resultant sparse matrix: [[2 1 0] [3 7 6] [0 0 5]]
结论
在本教程中,我们学习了四种在 Python 中生成稀疏矩阵的不同方法。我们还学习了如何从 numpy 数组生成稀疏矩阵。
以上是如何在Python中创建稀疏矩阵?的详细内容。更多信息请关注PHP中文网其他相关文章!
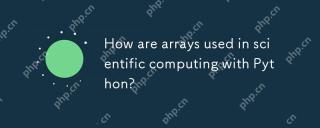
Arraysinpython,尤其是Vianumpy,ArecrucialInsCientificComputingfortheireftheireffertheireffertheirefferthe.1)Heasuedfornumerericalicerationalation,dataAnalysis和Machinelearning.2)Numpy'Simpy'Simpy'simplementIncressionSressirestrionsfasteroperoperoperationspasterationspasterationspasterationspasterationspasterationsthanpythonlists.3)inthanypythonlists.3)andAreseNableAblequick
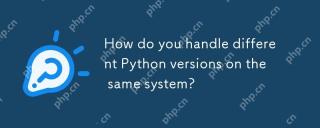
你可以通过使用pyenv、venv和Anaconda来管理不同的Python版本。1)使用pyenv管理多个Python版本:安装pyenv,设置全局和本地版本。2)使用venv创建虚拟环境以隔离项目依赖。3)使用Anaconda管理数据科学项目中的Python版本。4)保留系统Python用于系统级任务。通过这些工具和策略,你可以有效地管理不同版本的Python,确保项目顺利运行。
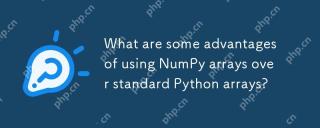
numpyarrayshaveseveraladagesoverandastardandpythonarrays:1)基于基于duetoc的iMplation,2)2)他们的aremoremoremorymorymoremorymoremorymoremorymoremoremory,尤其是WithlargedAtasets和3)效率化,效率化,矢量化函数函数函数函数构成和稳定性构成和稳定性的操作,制造
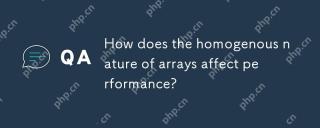
数组的同质性对性能的影响是双重的:1)同质性允许编译器优化内存访问,提高性能;2)但限制了类型多样性,可能导致效率低下。总之,选择合适的数据结构至关重要。
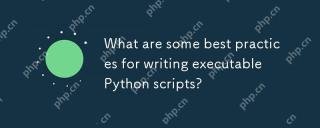
到CraftCraftExecutablePythcripts,lollow TheSebestPractices:1)Addashebangline(#!/usr/usr/bin/envpython3)tomakethescriptexecutable.2)setpermissionswithchmodwithchmod xyour_script.3)
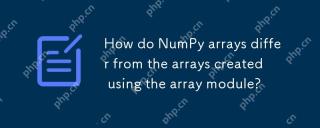
numpyArraysareAreBetterFornumericalialoperations andmulti-demensionaldata,而learthearrayModuleSutableforbasic,内存效率段
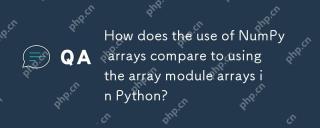
numpyArraySareAreBetterForHeAvyNumericalComputing,而lelethearRayModulesiutable-usemoblemory-connerage-inderabledsswithSimpleDatateTypes.1)NumpyArsofferVerverVerverVerverVersAtility andPerformanceForlargedForlargedAtatasetSetsAtsAndAtasEndCompleXoper.2)
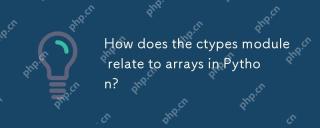
ctypesallowscreatingingangandmanipulatingc-stylarraysinpython.1)usectypestoInterfacewithClibrariesForperfermance.2)createc-stylec-stylec-stylarraysfornumericalcomputations.3)passarraystocfunctions foreforfunctionsforeffortions.however.however,However,HoweverofiousofmemoryManageManiverage,Pressiveo,Pressivero


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具
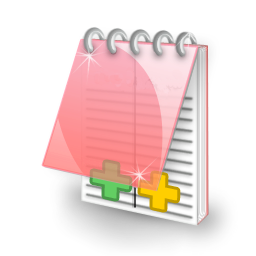
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能
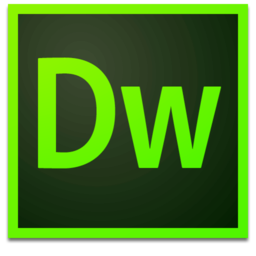
Dreamweaver Mac版
视觉化网页开发工具

记事本++7.3.1
好用且免费的代码编辑器

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。
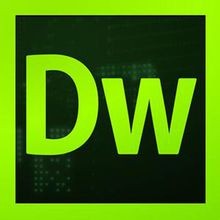
Dreamweaver CS6
视觉化网页开发工具