生成树是连接所有顶点的有向无向图子图。图中可以存在许多生成树。每个图上的最小生成树(MST)的权重相同或小于所有其他生成树。权重被分配给生成树的边,总和是分配给每个边的权重。由于 V 是图中的顶点数,因此最小生成树的边数为 (V - 1),其中 V 是边数。
使用 Kruskal 算法查找最小生成树
所有边应按权重非降序排列。
选择最小的边。如果未形成环,则包含该边。
应执行步骤 2,直到生成树具有 (V-1) 条边。
在这种情况下,我们被告知要使用贪婪方法。贪心选项是选择权重最小的边。举例来说:该图的最小生成树为 (9-1)= 8 条边。
After sorting: Weight Src Dest 21 27 26 22 28 22 22 26 25 24 20 21 24 22 25 26 28 26 27 22 23 27 27 28 28 20 27 28 21 22 29 23 24 30 25 24 31 21 27 34 23 25
现在我们需要根据排序选取所有边。
包含边 26-27->,因为没有形成环
边包括 28-22->,因为没有形成环路
包括边缘 26-25->,因为没有形成环路。
包括边缘 20-21->,因为没有形成环路
边 22-25-> 被包含,因为没有形成环路。
边 28-26-> 因环路形成而被丢弃
边 22-23-> > 包括,因为没有形成环路
边 27-28-> 因环路形成而被丢弃
边线 20-27-> 包括,因为没有形成环路
边21-22->因形成环而被丢弃
边23-24->因未形成环而被包括
由于边的数量为(V-1),所以算法到此结束。
示例
#include <stdio.h> #include <stdlib.h> #include <string.h> struct Edge { int src, dest, weight; }; struct Graph { int V, E; struct Edge* edge; }; struct Graph* createGraph(int V, int E){ struct Graph* graph = (struct Graph*)(malloc(sizeof(struct Graph))); graph->V = V; graph->E = E; graph->edge = (struct Edge*)malloc(sizeof( struct Edge)*E); return graph; } struct subset { int parent; int rank; }; int find(struct subset subsets[], int i){ if (subsets[i].parent != i) subsets[i].parent = find(subsets, subsets[i].parent); return subsets[i].parent; } void Union(struct subset subsets[], int x, int y){ int xroot = find(subsets, x); int yroot = find(subsets, y); if (subsets[xroot].rank < subsets[yroot].rank) subsets[xroot].parent = yroot; else if (subsets[xroot].rank > subsets[yroot].rank) subsets[yroot].parent = xroot; else{ subsets[yroot].parent = xroot; subsets[xroot].rank++; } } int myComp(const void* a, const void* b){ struct Edge* a1 = (struct Edge*)a; struct Edge* b1 = (struct Edge*)b; return a1->weight > b1->weight; } void KruskalMST(struct Graph* graph){ int V = graph->V; struct Edge result[V]; int e = 0; int i = 0; qsort(graph->edge, graph->E, sizeof(graph->edge[0]), myComp); struct subset* subsets = (struct subset*)malloc(V * sizeof(struct subset)); for (int v = 0; v < V; ++v) { subsets[v].parent = v; subsets[v].rank = 0; } while (e < V - 1 && i < graph->E) { struct Edge next_edge = graph->edge[i++]; int x = find(subsets, next_edge.src); int y = find(subsets, next_edge.dest); if (x != y) { result[e++] = next_edge; Union(subsets, x, y); } } printf("Following are the edges in the constructed MST\n"); int minimumCost = 0; for (i = 0; i < e; ++i){ printf("%d -- %d == %d\n", result[i].src, result[i].dest, result[i].weight); minimumCost += result[i].weight; } printf("Minimum Cost Spanning tree : %d",minimumCost); return; } int main(){ /* Let us create the following weighted graph 30 0--------1 | \ | 26| 25\ |15 | \ | 22--------23 24 */ int V = 24; int E = 25; struct Graph* graph = createGraph(V, E); graph->edge[0].src = 20; graph->edge[0].dest = 21; graph->edge[0].weight = 30; graph->edge[1].src = 20; graph->edge[1].dest = 22; graph->edge[1].weight = 26; graph->edge[2].src = 20; graph->edge[2].dest = 23; graph->edge[2].weight = 25; graph->edge[3].src = 21; graph->edge[3].dest = 23; graph->edge[3].weight = 35; graph->edge[4].src = 22; graph->edge[4].dest = 23; graph->edge[4].weight = 24; KruskalMST(graph); return 0; }
输出
Following are the edges in the constructed MST 22 -- 23 == 24 20 -- 23 == 25 20 -- 21 == 30 Minimum Cost Spanning tree : 79
结论
本教程演示了如何使用 Kruskal 的最小生成树算法-贪心法和 C++ 代码来解决此问题。我们还可以用java、python和其他语言编写这段代码。它是根据克鲁斯卡尔的概念建模的。该程序查找给定图中的最短生成树。我们希望本教程对您有所帮助。
以上是Kruskal的最小生成树算法-贪婪算法在C++中的详细内容。更多信息请关注PHP中文网其他相关文章!
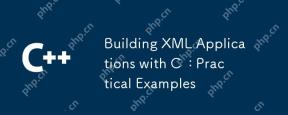
在C 中处理XML数据可以使用TinyXML、Pugixml或libxml2库。1)解析XML文件:使用DOM或SAX方法,DOM适合小文件,SAX适合大文件。2)生成XML文件:将数据结构转换为XML格式并写入文件。通过这些步骤,可以有效地管理和操作XML数据。
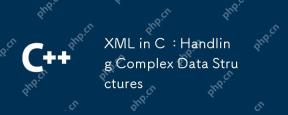
在C 中处理XML数据结构可以使用TinyXML或pugixml库。1)使用pugixml库解析和生成XML文件。2)处理复杂的嵌套XML元素,如书籍信息。3)优化XML处理代码,建议使用高效库和流式解析。通过这些步骤,可以高效处理XML数据。
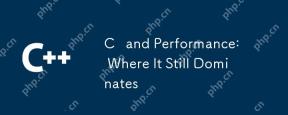
C 在性能优化方面仍然占据主导地位,因为其低级内存管理和高效执行能力使其在游戏开发、金融交易系统和嵌入式系统中不可或缺。具体表现为:1)在游戏开发中,C 的低级内存管理和高效执行能力使得它成为游戏引擎开发的首选语言;2)在金融交易系统中,C 的性能优势确保了极低的延迟和高吞吐量;3)在嵌入式系统中,C 的低级内存管理和高效执行能力使得它在资源有限的环境中非常受欢迎。
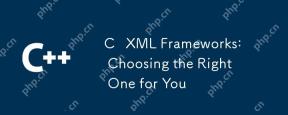
C XML框架的选择应基于项目需求。1)TinyXML适合资源受限环境,2)pugixml适用于高性能需求,3)Xerces-C 支持复杂的XMLSchema验证,选择时需考虑性能、易用性和许可证。
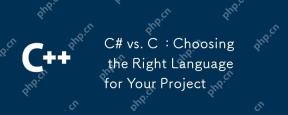
C#适合需要开发效率和类型安全的项目,而C 适合需要高性能和硬件控制的项目。 1)C#提供垃圾回收和LINQ,适用于企业应用和Windows开发。 2)C 以高性能和底层控制着称,广泛用于游戏和系统编程。
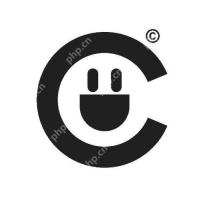
C 代码优化可以通过以下策略实现:1.手动管理内存以优化使用;2.编写符合编译器优化规则的代码;3.选择合适的算法和数据结构;4.使用内联函数减少调用开销;5.应用模板元编程在编译时优化;6.避免不必要的拷贝,使用移动语义和引用参数;7.正确使用const帮助编译器优化;8.选择合适的数据结构,如std::vector。
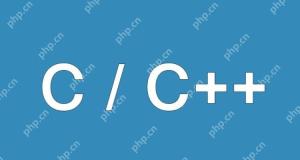
C 中的volatile关键字用于告知编译器变量值可能在代码控制之外被改变,因此不能对其进行优化。1)它常用于读取可能被硬件或中断服务程序修改的变量,如传感器状态。2)volatile不能保证多线程安全,应使用互斥锁或原子操作。3)使用volatile可能导致性能slight下降,但确保程序正确性。
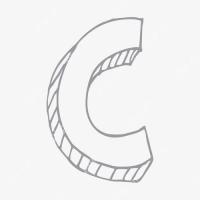
在C 中测量线程性能可以使用标准库中的计时工具、性能分析工具和自定义计时器。1.使用库测量执行时间。2.使用gprof进行性能分析,步骤包括编译时添加-pg选项、运行程序生成gmon.out文件、生成性能报告。3.使用Valgrind的Callgrind模块进行更详细的分析,步骤包括运行程序生成callgrind.out文件、使用kcachegrind查看结果。4.自定义计时器可灵活测量特定代码段的执行时间。这些方法帮助全面了解线程性能,并优化代码。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

SublimeText3汉化版
中文版,非常好用

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),

Atom编辑器mac版下载
最流行的的开源编辑器