PHP和Vue开发脑图功能实战指南
引言:
脑图是一种流行的信息组织方式,可以将复杂的思维或知识关系以图形化的形式呈现出来。在现代互联网应用开发中,添加脑图功能能够提升用户体验和数据可视化效果。本文将介绍如何使用PHP和Vue开发脑图功能,并提供实战指南和代码示例。
技术介绍:
- PHP:PHP是一种用于开发Web应用的服务器端脚本语言。在本文中,我们将使用PHP来处理数据库交互和API请求。
- Vue:Vue是一种流行的JavaScript框架,用于构建前端用户界面。在本文中,我们将使用Vue来实现脑图的前端组件。
开发准备:
在开始之前,我们需要确保已经安装了PHP和Vue的开发环境。可以在官方网站上下载和安装所需的软件包。
步骤一:数据库设计
首先,我们需要设计数据库以存储脑图的相关数据。以下是一个简化的数据库表设计示例:
CREATE TABLE `nodes` ( `id` int(11) NOT NULL AUTO_INCREMENT, `title` varchar(255) NOT NULL, `parent_id` int(11) DEFAULT NULL, PRIMARY KEY (`id`), FOREIGN KEY (`parent_id`) REFERENCES `nodes` (`id`) ); CREATE TABLE `edges` ( `id` int(11) NOT NULL AUTO_INCREMENT, `from_node_id` int(11) NOT NULL, `to_node_id` int(11) NOT NULL, PRIMARY KEY (`id`), FOREIGN KEY (`from_node_id`) REFERENCES `nodes` (`id`), FOREIGN KEY (`to_node_id`) REFERENCES `nodes` (`id`) );
步骤二:后端开发
接下来,我们开始开发后端代码。首先,创建一个PHP文件,命名为“api.php”。在该文件中,我们将实现服务器端的API接口。
<?php // 连接数据库 $db = new mysqli('localhost', 'username', 'password', 'database'); // 获取所有节点 function getAllNodes() { global $db; $result = $db->query("SELECT * FROM `nodes`"); $nodes = array(); while ($row = $result->fetch_assoc()) { $nodes[] = $row; } return $nodes; } // 获取所有边 function getAllEdges() { global $db; $result = $db->query("SELECT * FROM `edges`"); $edges = array(); while ($row = $result->fetch_assoc()) { $edges[] = $row; } return $edges; } // 设置HTTP响应标头 header('Content-Type: application/json'); // 处理API请求 $method = $_SERVER['REQUEST_METHOD']; $path = $_SERVER['REQUEST_URI']; if ($method === 'GET' && $path === '/api/nodes') { echo json_encode(getAllNodes()); } else if ($method === 'GET' && $path === '/api/edges') { echo json_encode(getAllEdges()); } else { http_response_code(404); echo json_encode(array('error' => 'Not Found')); } ?>
步骤三:前端开发
现在,我们开始开发前端代码。创建一个Vue组件,命名为“MindMap.vue”。在该组件中,我们将使用Vue的数据绑定和组件化技术来实现脑图的交互功能。
<template> <div> <div id="mindmap"></div> </div> </template> <script> export default { mounted() { // 从API获取数据 fetch('/api/nodes') .then(response => response.json()) .then(nodes => { // 使用数据来绘制脑图 const mindmap = new d3.Mindmap('#mindmap') .nodes(nodes) .edges(this.edges) .render(); }); }, computed: { edges() { // 从API获取边 return fetch('/api/edges') .then(response => response.json()) .then(edges => edges); } } } </script> <style> #mindmap { width: 800px; height: 600px; } </style>
步骤四:运行应用程序
现在,我们已经完成了后端代码和前端代码的开发。可以在命令行中运行以下命令来启动本地服务器,并查看应用程序的运行效果:
php -S localhost:8000
打开浏览器并访问“http://localhost:8000”以查看应用程序的脑图功能。
结论:
本文介绍了如何使用PHP和Vue开发脑图功能的实战指南,并提供了相关的代码示例。通过学习本文,您可以了解到如何利用PHP和Vue来实现脑图的数据库交互和前端组件化功能。希望本文能对您的开发工作有所帮助,祝您开发愉快!
以上是PHP和Vue开发脑图功能实战指南的详细内容。更多信息请关注PHP中文网其他相关文章!
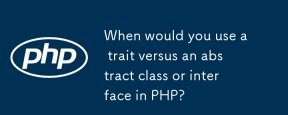
在PHP中,trait适用于需要方法复用但不适合使用继承的情况。1)trait允许在类中复用方法,避免多重继承复杂性。2)使用trait时需注意方法冲突,可通过insteadof和as关键字解决。3)应避免过度使用trait,保持其单一职责,以优化性能和提高代码可维护性。
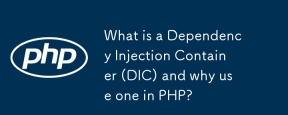
依赖注入容器(DIC)是一种管理和提供对象依赖关系的工具,用于PHP项目中。DIC的主要好处包括:1.解耦,使组件独立,代码易维护和测试;2.灵活性,易替换或修改依赖关系;3.可测试性,方便注入mock对象进行单元测试。
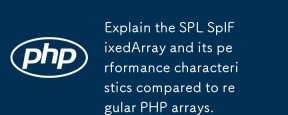
SplFixedArray在PHP中是一种固定大小的数组,适用于需要高性能和低内存使用量的场景。1)它在创建时需指定大小,避免动态调整带来的开销。2)基于C语言数组,直接操作内存,访问速度快。3)适合大规模数据处理和内存敏感环境,但需谨慎使用,因其大小固定。
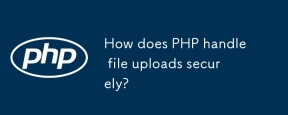
PHP通过$\_FILES变量处理文件上传,确保安全性的方法包括:1.检查上传错误,2.验证文件类型和大小,3.防止文件覆盖,4.移动文件到永久存储位置。
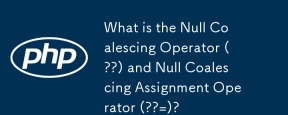
JavaScript中处理空值可以使用NullCoalescingOperator(??)和NullCoalescingAssignmentOperator(??=)。1.??返回第一个非null或非undefined的操作数。2.??=将变量赋值为右操作数的值,但前提是该变量为null或undefined。这些操作符简化了代码逻辑,提高了可读性和性能。
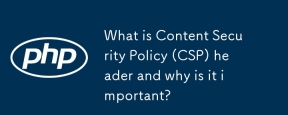
CSP重要因为它能防范XSS攻击和限制资源加载,提升网站安全性。1.CSP是HTTP响应头的一部分,通过严格策略限制恶意行为。2.基本用法是只允许从同源加载资源。3.高级用法可设置更细粒度的策略,如允许特定域名加载脚本和样式。4.使用Content-Security-Policy-Report-Only头部可调试和优化CSP策略。
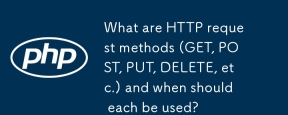
HTTP请求方法包括GET、POST、PUT和DELETE,分别用于获取、提交、更新和删除资源。1.GET方法用于获取资源,适用于读取操作。2.POST方法用于提交数据,常用于创建新资源。3.PUT方法用于更新资源,适用于完整更新。4.DELETE方法用于删除资源,适用于删除操作。
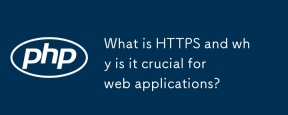
HTTPS是一种在HTTP基础上增加安全层的协议,主要通过加密数据保护用户隐私和数据安全。其工作原理包括TLS握手、证书验证和加密通信。实现HTTPS时需注意证书管理、性能影响和混合内容问题。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),

SublimeText3 Linux新版
SublimeText3 Linux最新版

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。

SublimeText3汉化版
中文版,非常好用

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。