php代码:
<?php class DES { var $key; var $iv; //偏移量 function DES($key, $iv=0) { $this->key = $key; if($iv == 0) { $this->iv = $key; } else { $this->iv = $iv; } } //加密 function encrypt($str) { $size = mcrypt_get_block_size ( MCRYPT_DES, MCRYPT_MODE_CBC ); $str = $this->pkcs5Pad ( $str, $size ); $data=mcrypt_cbc(MCRYPT_DES, $this->key, $str, MCRYPT_ENCRYPT, $this->iv); //$data=strtoupper(bin2hex($data)); //返回大写十六进制字符串 return base64_encode($data); } //解密 function decrypt($str) { $str = base64_decode ($str); //$strBin = $this->hex2bin( strtolower($str)); $str = mcrypt_cbc(MCRYPT_DES, $this->key, $str, MCRYPT_DECRYPT, $this->iv ); $str = $this->pkcs5Unpad( $str ); return $str; } function hex2bin($hexData) { $binData = ""; for($i = 0; $i < strlen ( $hexData ); $i += 2) { $binData .= chr(hexdec(substr($hexData, $i, 2))); } return $binData; } function pkcs5Pad($text, $blocksize) { $pad = $blocksize - (strlen ( $text ) % $blocksize); return $text . str_repeat ( chr ( $pad ), $pad ); } function pkcs5Unpad($text) { $pad = ord ( $text {strlen ( $text ) - 1} ); if ($pad > strlen ( $text )) return false; if (strspn ( $text, chr ( $pad ), strlen ( $text ) - $pad ) != $pad) return false; return substr ( $text, 0, - 1 * $pad ); } } $str = 'abcd'; $key= 'asdfwef5'; $crypt = new DES($key); $mstr = $crypt->encrypt($str); $str = $crypt->decrypt($mstr); echo $str.' <=> '.$mstr; ?>
java代码:
package com.test; import it.sauronsoftware.base64.Base64; import java.security.Key; import java.security.SecureRandom; import java.security.spec.AlgorithmParameterSpec; import javax.crypto.Cipher; import javax.crypto.SecretKeyFactory; import javax.crypto.spec.DESKeySpec; import javax.crypto.spec.IvParameterSpec; public class Main { public static final String ALGORITHM_DES = "DES/CBC/PKCS5Padding"; /** * DES算法,加密 * * @param data 待加密字符串 * @param key 加密私钥,长度不能够小于8位 * @return 加密后的字节数组,一般结合Base64编码使用 * @throws CryptException 异常 */ public static String encode(String key,String data) throws Exception { return encode(key, data.getBytes()); } /** * DES算法,加密 * * @param data 待加密字符串 * @param key 加密私钥,长度不能够小于8位 * @return 加密后的字节数组,一般结合Base64编码使用 * @throws CryptException 异常 */ public static String encode(String key,byte[] data) throws Exception { try { DESKeySpec dks = new DESKeySpec(key.getBytes()); SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES"); //key的长度不能够小于8位字节 Key secretKey = keyFactory.generateSecret(dks); Cipher cipher = Cipher.getInstance(ALGORITHM_DES); IvParameterSpec iv = new IvParameterSpec(key.getBytes()); AlgorithmParameterSpec paramSpec = iv; cipher.init(Cipher.ENCRYPT_MODE, secretKey,paramSpec); byte[] bytes = cipher.doFinal(data); // return byte2hex(bytes); return new String(Base64.encode(bytes)); } catch (Exception e) { throw new Exception(e); } } /** * DES算法,解密 * * @param data 待解密字符串 * @param key 解密私钥,长度不能够小于8位 * @return 解密后的字节数组 * @throws Exception 异常 */ public static byte[] decode(String key,byte[] data) throws Exception { try { SecureRandom sr = new SecureRandom(); DESKeySpec dks = new DESKeySpec(key.getBytes()); SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DES"); //key的长度不能够小于8位字节 Key secretKey = keyFactory.generateSecret(dks); Cipher cipher = Cipher.getInstance(ALGORITHM_DES); IvParameterSpec iv = new IvParameterSpec(key.getBytes()); AlgorithmParameterSpec paramSpec = iv; cipher.init(Cipher.DECRYPT_MODE, secretKey,paramSpec); return cipher.doFinal(data); } catch (Exception e) { throw new Exception(e); } } /** * 获取编码后的值 * @param key * @param data * @return * @throws Exception */ public static String decodeValue(String key,String data) { byte[] datas; String value = null; try { datas = decode(key, Base64.decode(data.getBytes())); value = new String(datas); } catch (Exception e) { value = ""; } return value; } public static void main(String[] args) throws Exception { System.out.println("明:abcd ;密:" + Main.encode("asdfwef5","abcd")); } }
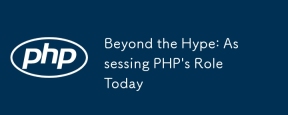
PHP在现代编程中仍然是一个强大且广泛使用的工具,尤其在web开发领域。1)PHP易用且与数据库集成无缝,是许多开发者的首选。2)它支持动态内容生成和面向对象编程,适合快速创建和维护网站。3)PHP的性能可以通过缓存和优化数据库查询来提升,其广泛的社区和丰富生态系统使其在当今技术栈中仍具重要地位。
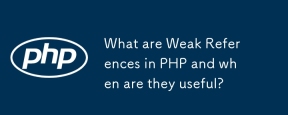
在PHP中,弱引用是通过WeakReference类实现的,不会阻止垃圾回收器回收对象。弱引用适用于缓存系统和事件监听器等场景,需注意其不能保证对象存活,且垃圾回收可能延迟。
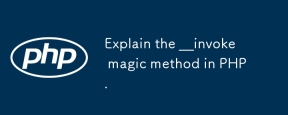
\_\_invoke方法允许对象像函数一样被调用。1.定义\_\_invoke方法使对象可被调用。2.使用$obj(...)语法时,PHP会执行\_\_invoke方法。3.适用于日志记录和计算器等场景,提高代码灵活性和可读性。
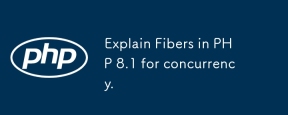
Fibers在PHP8.1中引入,提升了并发处理能力。1)Fibers是一种轻量级的并发模型,类似于协程。2)它们允许开发者手动控制任务的执行流,适合处理I/O密集型任务。3)使用Fibers可以编写更高效、响应性更强的代码。
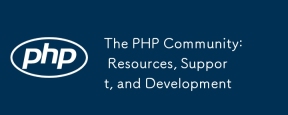
PHP社区提供了丰富的资源和支持,帮助开发者成长。1)资源包括官方文档、教程、博客和开源项目如Laravel和Symfony。2)支持可以通过StackOverflow、Reddit和Slack频道获得。3)开发动态可以通过关注RFC了解。4)融入社区可以通过积极参与、贡献代码和学习分享来实现。
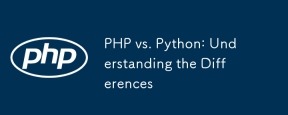
PHP和Python各有优势,选择应基于项目需求。1.PHP适合web开发,语法简单,执行效率高。2.Python适用于数据科学和机器学习,语法简洁,库丰富。
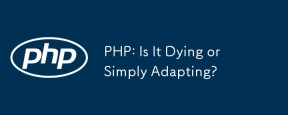
PHP不是在消亡,而是在不断适应和进化。1)PHP从1994年起经历多次版本迭代,适应新技术趋势。2)目前广泛应用于电子商务、内容管理系统等领域。3)PHP8引入JIT编译器等功能,提升性能和现代化。4)使用OPcache和遵循PSR-12标准可优化性能和代码质量。
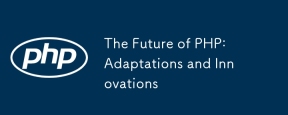
PHP的未来将通过适应新技术趋势和引入创新特性来实现:1)适应云计算、容器化和微服务架构,支持Docker和Kubernetes;2)引入JIT编译器和枚举类型,提升性能和数据处理效率;3)持续优化性能和推广最佳实践。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。

SublimeText3 Linux新版
SublimeText3 Linux最新版

DVWA
Damn Vulnerable Web App (DVWA) 是一个PHP/MySQL的Web应用程序,非常容易受到攻击。它的主要目标是成为安全专业人员在合法环境中测试自己的技能和工具的辅助工具,帮助Web开发人员更好地理解保护Web应用程序的过程,并帮助教师/学生在课堂环境中教授/学习Web应用程序安全。DVWA的目标是通过简单直接的界面练习一些最常见的Web漏洞,难度各不相同。请注意,该软件中

Atom编辑器mac版下载
最流行的的开源编辑器

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。