一、游戏解说
“走四儿”大部分活跃在山东济南、聊城、菏泽等地,是一种棋类游戏,特别适合儿童试玩。
在一个4×4的棋盘上,双方各有4子,分别摆放在棋盘两个最上面的两端线的四个位置上。下图
就是“走四儿”开局的样子。
二、游戏规则
“走四儿”的游戏规则是:
1.双方轮流走,每一步只能在上下左右中的一个无子的方向上走一个格,不能斜走。如果一方无法移动,则由另一方走。
2.当甲方的一个子移动到一条线上之后,这条线上只有甲方的两个子和乙方的一个子,且甲方的这两子相连,乙方的子与甲方那两子中的一个子相连,那么乙方的这个子就被吃掉。
下图是可以吃子的样式例举:
3.少于2个子的一方为输者。双方都无法胜对方就可以认为是和棋。
三、环境安装
1)素材(图片)
2)运行环境 小编使用的环境:Python3、Pycharm社区版、Pygame、 numpy模块部分自带就不一一 展示啦。
模块安装:pip install -i https://pypi.douban.com/simple/+模块名
四、代码展示
import pygame as pg from pygame.locals import * import sys import time import numpy as np pg.init() size = width, height = 600, 400 screen = pg.display.set_mode(size) f_clock = pg.time.Clock() fps = 30 pg.display.set_caption("走四棋儿") background = pg.image.load("background.png").convert_alpha() glb_pos = [[(90, 40), (190, 40), (290, 40), (390, 40)], [(90, 140), (190, 140), (290, 140), (390, 140)], [(90, 240), (190, 240), (290, 240), (390, 240)], [(90, 340), (190, 340), (290, 340), (390, 340)]] class ChessPieces(): def __init__(self, img_name): self.name = img_name self.id = None if self.name == 'heart': self.id = 2 elif self.name == 'spade': self.id = 3 self.img = pg.image.load(img_name + ".png").convert_alpha() self.rect = self.img.get_rect() self.pos_x, self.pos_y = 0, 0 self.alive_state = True def get_rect(self): return (self.rect[0], self.rect[1]) def get_pos(self): return (self.pos_x, self.pos_y) def update(self): if self.alive_state == True: self.rect[0] = glb_pos[self.pos_y][self.pos_x][0] self.rect[1] = glb_pos[self.pos_y][self.pos_x][1] screen.blit(self.img, self.rect) class Pointer(): def __init__(self): self.img = pg.image.load("pointer.png").convert_alpha() self.rect = self.img.get_rect() self.show = False self.selecting_item = False def point_to(self, Heart_Blade_class): if Heart_Blade_class.alive_state: self.pointing_to_item = Heart_Blade_class self.item_pos = Heart_Blade_class.get_rect() self.rect[0], self.rect[1] = self.item_pos[0], self.item_pos[1] - 24 def update(self): screen.blit(self.img, self.rect) class GlobalSituation(): def __init__(self): self.glb_situation = np.array([[2, 2, 2, 2], [0, 0, 0, 0], [0, 0, 0, 0], [3, 3, 3, 3]], dtype=np.uint8) self.spade_turn = None def refresh_situation(self): self.glb_situation = np.zeros([4, 4], np.uint8) for i in range(4): if heart[i].alive_state: self.glb_situation[heart[i].pos_y, heart[i].pos_x] = heart[i].id for i in range(4): if spade[i].alive_state: self.glb_situation[spade[i].pos_y, spade[i].pos_x] = spade[i].id for i in range(4): print(self.glb_situation[i][:]) print('=' * 12) if self.spade_turn != None: self.spade_turn = not self.spade_turn def check_situation(self, moved_item): curr_pos_x, curr_pos_y = moved_item.get_pos() curr_pos_col = self.glb_situation[:, curr_pos_x] curr_pos_raw = self.glb_situation[curr_pos_y, :] enemy_die = False if moved_item.id == 2: if np.sum(curr_pos_col) == 7: if (curr_pos_col == np.array([0, 2, 2, 3])).all(): enemy_die = True self.glb_situation[3, curr_pos_x] = 0 for spade_i in spade: if spade_i.alive_state and spade_i.pos_x == curr_pos_x and spade_i.pos_y == 3: spade_i.alive_state = False elif (curr_pos_col == np.array([2, 2, 3, 0])).all(): enemy_die = True self.glb_situation[2, curr_pos_x] = 0 for spade_i in spade: if spade_i.alive_state and spade_i.pos_x == curr_pos_x and spade_i.pos_y == 2: spade_i.alive_state = False elif (curr_pos_col == np.array([0, 3, 2, 2])).all(): enemy_die = True self.glb_situation[1, curr_pos_x] = 0 for spade_i in spade: if spade_i.alive_state and spade_i.pos_x == curr_pos_x and spade_i.pos_y == 1: spade_i.alive_state = False elif (curr_pos_col == np.array([3, 2, 2, 0])).all(): enemy_die = True self.glb_situation[0, curr_pos_x] = 0 for spade_i in spade: if spade_i.alive_state and spade_i.pos_x == curr_pos_x and spade_i.pos_y == 0: spade_i.alive_state = False if np.sum(curr_pos_raw) == 7: if (curr_pos_raw == np.array([0, 2, 2, 3])).all(): enemy_die = True self.glb_situation[curr_pos_y, 3] = 0 for spade_i in spade: if spade_i.alive_state and spade_i.pos_x == 3 and spade_i.pos_y == curr_pos_y: spade_i.alive_state = False elif (curr_pos_raw == np.array([2, 2, 3, 0])).all(): enemy_die = True self.glb_situation[curr_pos_y, 2] = 0 for spade_i in spade: if spade_i.alive_state and spade_i.pos_x == 2 and spade_i.pos_y == curr_pos_y: spade_i.alive_state = False elif (curr_pos_raw == np.array([0, 3, 2, 2])).all(): enemy_die = True self.glb_situation[curr_pos_y, 1] = 0 for spade_i in spade: if spade_i.alive_state and spade_i.pos_x == 1 and spade_i.pos_y == curr_pos_y: spade_i.alive_state = False elif (curr_pos_raw == np.array([3, 2, 2, 0])).all(): enemy_die = True self.glb_situation[curr_pos_y, 0] = 0 for spade_i in spade: if spade_i.alive_state and spade_i.pos_x == 0 and spade_i.pos_y == curr_pos_y: spade_i.alive_state = False elif moved_item.id == 3: if np.sum(curr_pos_col) == 8: if (curr_pos_col == np.array([0, 3, 3, 2])).all(): enemy_die = True self.glb_situation[3, curr_pos_x] = 0 for heart_i in heart: if heart_i.alive_state and heart_i.pos_x == curr_pos_x and heart_i.pos_y == 3: heart_i.alive_state = False elif (curr_pos_col == np.array([3, 3, 2, 0])).all(): enemy_die = True self.glb_situation[2, curr_pos_x] = 0 for heart_i in heart: if heart_i.alive_state and heart_i.pos_x == curr_pos_x and heart_i.pos_y == 2: heart_i.alive_state = False elif (curr_pos_col == np.array([0, 2, 3, 3])).all(): enemy_die = True self.glb_situation[1, curr_pos_x] = 0 for heart_i in heart: if heart_i.alive_state and heart_i.pos_x == curr_pos_x and heart_i.pos_y == 1: heart_i.alive_state = False elif (curr_pos_col == np.array([2, 3, 3, 0])).all(): enemy_die = True self.glb_situation[0, curr_pos_x] = 0 for heart_i in heart: if heart_i.alive_state and heart_i.pos_x == curr_pos_x and heart_i.pos_y == 0: heart_i.alive_state = False if np.sum(curr_pos_raw) == 8: if (curr_pos_raw == np.array([0, 3, 3, 2])).all(): enemy_die = True self.glb_situation[curr_pos_y, 3] = 0 for heart_i in heart: if heart_i.alive_state and heart_i.pos_x == 3 and heart_i.pos_y == curr_pos_y: heart_i.alive_state = False elif (curr_pos_raw == np.array([3, 3, 2, 0])).all(): enemy_die = True self.glb_situation[curr_pos_y, 2] = 0 for heart_i in heart: if heart_i.alive_state and heart_i.pos_x == 2 and heart_i.pos_y == curr_pos_y: heart_i.alive_state = False elif (curr_pos_raw == np.array([0, 2, 3, 3])).all(): enemy_die = True self.glb_situation[curr_pos_y, 1] = 0 for heart_i in heart: if heart_i.alive_state and heart_i.pos_x == 1 and heart_i.pos_y == curr_pos_y: heart_i.alive_state = False elif (curr_pos_raw == np.array([2, 3, 3, 0])).all(): enemy_die = True self.glb_situation[curr_pos_y, 0] = 0 for heart_i in heart: if heart_i.alive_state and heart_i.pos_x == 0 and heart_i.pos_y == curr_pos_y: heart_i.alive_state = False if enemy_die == True: self.glb_situation = np.zeros([4, 4], np.uint8) for i in range(4): if heart[i].alive_state: self.glb_situation[heart[i].pos_y, heart[i].pos_x] = heart[i].id for i in range(4): if spade[i].alive_state: self.glb_situation[spade[i].pos_y, spade[i].pos_x] = spade[i].id for i in range(4): print(self.glb_situation[i][:]) print('=' * 12) def check_game_over(self): heart_alive_num, spade_alive_num = 0, 0 for heart_i in heart: if heart_i.alive_state: heart_alive_num += 1 for spade_i in spade: if spade_i.alive_state: spade_alive_num += 1 if heart_alive_num <= 1: print('Spades win!') GlobalSituation.__init__(self) Pointer.__init__(self) chess_pieces_init() if spade_alive_num <= 1: print('Hearts win!') GlobalSituation.__init__(self) Pointer.__init__(self) chess_pieces_init() heart, spade = [None] * 4, [None] * 4 for i in range(4): heart[i] = ChessPieces('heart') spade[i] = ChessPieces('spade') def chess_pieces_init(): for i in range(4): heart[i].pos_y, heart[i].pos_x = 0, i spade[i].pos_y, spade[i].pos_x = 3, i heart[i].alive_state = True spade[i].alive_state = True chess_pieces_init() pointer = Pointer() situation = GlobalSituation() def check_click_item(c_x, c_y): selected_item = None if situation.spade_turn==None: for heart_i in heart: if heart_i.alive_state and heart_i.rect.collidepoint(c_x, c_y): situation.spade_turn = False selected_item = heart_i for spade_i in spade: if spade_i.alive_state and spade_i.rect.collidepoint(c_x, c_y): situation.spade_turn = True selected_item = spade_i else: if situation.spade_turn: for spade_i in spade: if spade_i.alive_state and spade_i.rect.collidepoint(c_x, c_y): selected_item = spade_i else: for heart_i in heart: if heart_i.alive_state and heart_i.rect.collidepoint(c_x, c_y): selected_item = heart_i return selected_item def move_to_dst_pos(selected_item, c_x, c_y): update_situation = False enemy_exist = False if selected_item.name == 'heart': for spade_i in spade: if spade_i.rect.collidepoint(c_x, c_y) and spade_i.alive_state: enemy_exist = True elif selected_item.name == 'spade': for heart_i in heart: if heart_i.rect.collidepoint(c_x, c_y) and heart_i.alive_state: enemy_exist = True if enemy_exist == False: delta_y, delta_x = c_y - selected_item.rect[1], c_x - selected_item.rect[0] if 80 <= abs(delta_x) <= 120 and abs(delta_y) <= 20: if delta_x < 0: if selected_item.pos_x > 0: selected_item.pos_x -= 1 else: if selected_item.pos_x < 3: selected_item.pos_x += 1 update_situation = True if 80 <= abs(delta_y) <= 120 and abs(delta_x) <= 20: if delta_y < 0: if selected_item.pos_y > 0: selected_item.pos_y -= 1 else: if selected_item.pos_y < 3: selected_item.pos_y += 1 update_situation = True return update_situation while True: for event in pg.event.get(): if event.type == pg.QUIT: sys.exit() elif event.type == pg.MOUSEBUTTONDOWN: cursor_x, cursor_y = pg.mouse.get_pos() clicked_item = check_click_item(cursor_x, cursor_y) if clicked_item != None: pointer.selecting_item = True pointer.point_to(clicked_item) else: if pointer.selecting_item: update_situation_flag = move_to_dst_pos(pointer.pointing_to_item, cursor_x, cursor_y) if update_situation_flag: situation.refresh_situation() situation.check_situation(pointer.pointing_to_item) situation.check_game_over() pointer.selecting_item = False screen.blit(background, (0, 0)) for heart_i in heart: heart_i.update() for spade_i in spade: spade_i.update() if pointer.selecting_item: pointer.update() f_clock.tick(fps) pg.display.update()
五、效果展示
以上是怎么使用Python+Pygame实现走四棋儿游戏的详细内容。更多信息请关注PHP中文网其他相关文章!
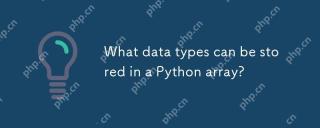
pythonlistscanStoryDatatepe,ArrayModulearRaysStoreOneType,and numpyArraySareSareAraysareSareAraysareSareComputations.1)列出sareversArversAtileButlessMemory-Felide.2)arraymoduleareareMogeMogeNareSaremogeNormogeNoreSoustAta.3)
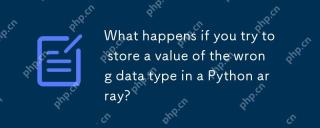
WhenyouattempttostoreavalueofthewrongdatatypeinaPythonarray,you'llencounteraTypeError.Thisisduetothearraymodule'sstricttypeenforcement,whichrequiresallelementstobeofthesametypeasspecifiedbythetypecode.Forperformancereasons,arraysaremoreefficientthanl
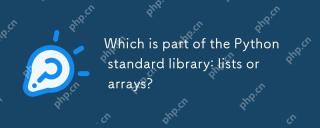
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
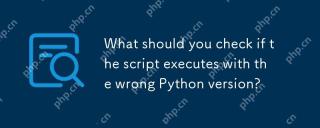
ThescriptisrunningwiththewrongPythonversionduetoincorrectdefaultinterpretersettings.Tofixthis:1)CheckthedefaultPythonversionusingpython--versionorpython3--version.2)Usevirtualenvironmentsbycreatingonewithpython3.9-mvenvmyenv,activatingit,andverifying
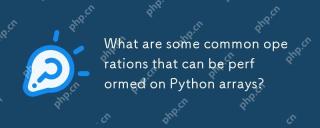
Pythonarrayssupportvariousoperations:1)Slicingextractssubsets,2)Appending/Extendingaddselements,3)Insertingplaceselementsatspecificpositions,4)Removingdeleteselements,5)Sorting/Reversingchangesorder,and6)Listcomprehensionscreatenewlistsbasedonexistin
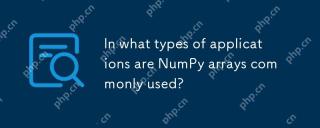
NumPyarraysareessentialforapplicationsrequiringefficientnumericalcomputationsanddatamanipulation.Theyarecrucialindatascience,machinelearning,physics,engineering,andfinanceduetotheirabilitytohandlelarge-scaledataefficiently.Forexample,infinancialanaly
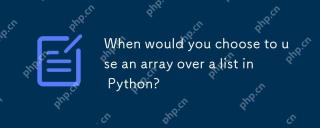
useanArray.ArarayoveralistinpythonwhendeAlingwithHomeSdata,performance-Caliticalcode,orinterFacingWithCcccode.1)同质性data:arrayssavememorywithtypedelements.2)绩效code-performance-clitionalcode-clitadialcode-critical-clitical-clitical-clitical-clitaine code:araysofferferbetterperperperformenterperformanceformanceformancefornalumericalicalialical.3)
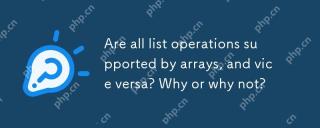
不,notalllistoperationsareSupportedByArrays,andviceversa.1)arraysdonotsupportdynamicoperationslikeappendorinsertwithoutresizing,wheremactssperformance.2)listssdonotguaranteeconeeconeconstanttanttanttanttanttanttanttanttimecomplecomecomecomplecomecomecomecomecomecomplecomectaccesslikearrikearraysodo。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

禅工作室 13.0.1
功能强大的PHP集成开发环境

Atom编辑器mac版下载
最流行的的开源编辑器
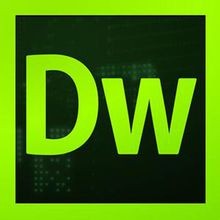
Dreamweaver CS6
视觉化网页开发工具
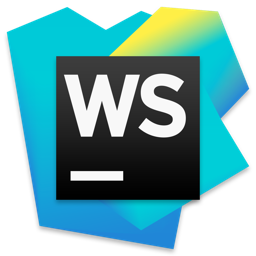
WebStorm Mac版
好用的JavaScript开发工具

DVWA
Damn Vulnerable Web App (DVWA) 是一个PHP/MySQL的Web应用程序,非常容易受到攻击。它的主要目标是成为安全专业人员在合法环境中测试自己的技能和工具的辅助工具,帮助Web开发人员更好地理解保护Web应用程序的过程,并帮助教师/学生在课堂环境中教授/学习Web应用程序安全。DVWA的目标是通过简单直接的界面练习一些最常见的Web漏洞,难度各不相同。请注意,该软件中