springboot实现jar运行复制resources文件到指定的目录
1. 需求
在项目开发过程中需要将项目resources/static/目录下所有资源资源复制到指定目录。公司项目中需要下载视频文件,由于下载的有个html页面,对多路视频进行画面加载,用到对应的静态资源文件,如js,css.jwplayer,jquery.js等文件
maven打成的jar和平时发布的项目路径不通,所以在读取路径的时候获取的是jar的路径,无法获取jar里面的文件路径
2. 思路
根据我的需求,复制的思路大概是,先获取到resources/static/blog目录下文件清单,然后通过清单,循环将文件复制到指定位置(相对路径需要确保一致)
因为项目会被打成jar包,所以不能用传统的目录文件复制方式,这里需要用到Spring Resource:
Resource接口,简单说是整个Spring框架对资源的抽象访问接口。它继承于InputStreamSource接口。后续文章会详细的分析。
Resource接口的方法说明:
方法 | 说明 |
---|---|
exists() | 判断资源是否存在,true表示存在。 |
isReadable() | 判断资源的内容是否可读。需要注意的是当其结果为true的时候,其内容未必真的可读,但如果返回false,则其内容必定不可读。 |
isOpen() | 判断当前Resource代表的底层资源是否已经打开,如果返回true,则只能被读取一次然后关闭以避免资源泄露;该方法主要针对于InputStreamResource,实现类中只有它的返回结果为true,其他都为false。 |
getURL() | 返回当前资源对应的URL。如果当前资源不能解析为一个URL则会抛出异常。如ByteArrayResource就不能解析为一个URL。 |
getURI() | 返回当前资源对应的URI。如果当前资源不能解析为一个URI则会抛出异常。 |
getFile() | 返回当前资源对应的File。 |
contentLength() | 返回当前资源内容的长度 |
lastModified() | 返回当前Resource代表的底层资源的最后修改时间。 |
createRelative() | 根据资源的相对路径创建新资源。[默认不支持创建相对路径资源] |
getFilename() | 获取资源的文件名。 |
getDescription() | 返回当前资源底层资源的描述符,通常就是资源的全路径(实际文件名或实际URL地址)。 |
getInputStream() | 获取当前资源代表的输入流。除了InputStreamResource实现类以外,其它Resource实现类每次调用getInputStream()方法都将返回一个全新的InputStream。 |
获取Resource清单,我需要通过ResourceLoader接口获取资源,在这里我选择了
org.springframework.core.io.support.PathMatchingResourcePatternResolver
3. 实现代码
/** * 只复制下载文件中用到的js */ private void copyJwplayer() { //判断指定目录下文件是否存在 ApplicationHome applicationHome = new ApplicationHome(getClass()); String rootpath = applicationHome.getSource().getParentFile().toString(); String realpath=rootpath+"/vod/jwplayer/"; //目标文件 String silderrealpath=rootpath+"/vod/jwplayer/silder/"; //目标文件 String historyrealpath=rootpath+"/vod/jwplayer/history/"; //目标文件 String jwplayerrealpath=rootpath+"/vod/jwplayer/jwplayer/"; //目标文件 String layoutrealpath=rootpath+"/vod/jwplayer/res/layout/"; //目标文件 /** * 此处只能复制目录中的文件,不能多层目录复制(目录中的目录不能复制,如果循环复制目录中的目录则会提示cannot be resolved to URL because it does not exist) */ //不使用getFileFromClassPath()则报[static/jwplayerF:/workspace/VRSH265/target/classes/static/jwplayer/flvjs/] cannot be resolved to URL because it does not exist //jwplayer File fileFromClassPath = FreeMarkerUtil.getFileFromClassPath("/static/jwplayer/"); //复制目录 FreeMarkerUtil.BatCopyFileFromJar(fileFromClassPath.toString(),realpath); //silder File flvjspath = FreeMarkerUtil.getFileFromClassPath("/static/jwplayer/silder/"); //复制目录 FreeMarkerUtil.BatCopyFileFromJar(flvjspath.toString(),silderrealpath); //history File historypath = FreeMarkerUtil.getFileFromClassPath("/static/jwplayer/history/"); //复制目录 FreeMarkerUtil.BatCopyFileFromJar(historypath.toString(),historyrealpath); //jwplayer File jwplayerpath = FreeMarkerUtil.getFileFromClassPath("/static/jwplayer/jwplayer/"); //复制目录 FreeMarkerUtil.BatCopyFileFromJar(jwplayerpath.toString(),jwplayerrealpath); //layout File layoutpath = FreeMarkerUtil.getFileFromClassPath("/static/jwplayer/res/layout/"); //复制目录 FreeMarkerUtil.BatCopyFileFromJar(layoutpath.toString(),layoutrealpath); }
4. 工具类FreeMarkerUtil
package com.aio.util; import org.apache.commons.io.FileUtils; import org.apache.commons.lang3.StringUtils; import org.springframework.core.io.ClassPathResource; import org.springframework.core.io.Resource; import org.springframework.core.io.support.PathMatchingResourcePatternResolver; import org.springframework.core.io.support.ResourcePatternResolver; import java.io.*; /** * @author:hahaha * @creattime:2021-12-02 10:33 */ public class FreeMarkerUtil { /** * 复制path目录下所有文件 * @param path 文件目录 不能以/开头 * @param newpath 新文件目录 */ public static void BatCopyFileFromJar(String path,String newpath) { if (!new File(newpath).exists()){ new File(newpath).mkdir(); } ResourcePatternResolver resolver = new PathMatchingResourcePatternResolver(); try { //获取所有匹配的文件 Resource[] resources = resolver.getResources(path+"/*"); //打印有多少文件 for(int i=0;i<resources.length;i++) { Resource resource=resources[i]; try { //以jar运行时,resource.getFile().isFile() 无法获取文件类型,会报异常,抓取异常后直接生成新的文件即可;以非jar运行时,需要判断文件类型,避免如果是目录会复制错误,将目录写成文件。 if(resource.getFile().isFile()) { makeFile(newpath+"/"+resource.getFilename()); InputStream stream = resource.getInputStream(); write2File(stream, newpath+"/"+resource.getFilename()); } }catch (Exception e) { makeFile(newpath+"/"+resource.getFilename()); InputStream stream = resource.getInputStream(); write2File(stream, newpath+"/"+resource.getFilename()); } } } catch (Exception e) { e.printStackTrace(); } } /** * 创建文件 * @param path 全路径 指向文件 * @return */ public static boolean makeFile(String path) { File file = new File(path); if(file.exists()) { return false; } if (path.endsWith(File.separator)) { return false; } if(!file.getParentFile().exists()) { if(!file.getParentFile().mkdirs()) { return false; } } try { if (file.createNewFile()) { return true; } else { return false; } } catch (IOException e) { e.printStackTrace(); return false; } } /** * 输入流写入文件 * * @param is * 输入流 * @param filePath * 文件保存目录路径 * @throws IOException */ public static void write2File(InputStream is, String filePath) throws IOException { OutputStream os = new FileOutputStream(filePath); int len = 8192; byte[] buffer = new byte[len]; while ((len = is.read(buffer, 0, len)) != -1) { os.write(buffer, 0, len); } os.close(); is.close(); } /** *处理异常报错(springboot读取classpath里的文件,解决打jar包java.io.FileNotFoundException: class path resource cannot be opened) **/ public static File getFileFromClassPath(String path){ File targetFile = new File(path); if(!targetFile.exists()){ if(targetFile.getParent()!=null){ File parent=new File(targetFile.getParent()); if(!parent.exists()){ parent.mkdirs(); } } InputStream initialStream=null; OutputStream outStream =null; try { Resource resource=new ClassPathResource(path); //注意通过getInputStream,不能用getFile initialStream=resource.getInputStream(); byte[] buffer = new byte[initialStream.available()]; initialStream.read(buffer); outStream = new FileOutputStream(targetFile); outStream.write(buffer); } catch (IOException e) { } finally { if (initialStream != null) { try { initialStream.close(); // 关闭流 } catch (IOException e) { } } if (outStream != null) { try { outStream.close(); // 关闭流 } catch (IOException e) { } } } } return targetFile; } }
5.效果
以上是springboot怎么实现jar运行复制resources文件到指定的目录的详细内容。更多信息请关注PHP中文网其他相关文章!

本文讨论了使用Maven和Gradle进行Java项目管理,构建自动化和依赖性解决方案,以比较其方法和优化策略。
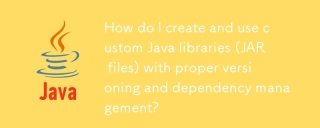
本文使用Maven和Gradle之类的工具讨论了具有适当的版本控制和依赖关系管理的自定义Java库(JAR文件)的创建和使用。
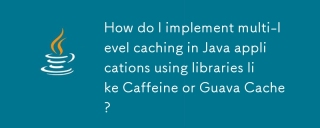
本文讨论了使用咖啡因和Guava缓存在Java中实施多层缓存以提高应用程序性能。它涵盖设置,集成和绩效优势,以及配置和驱逐政策管理最佳PRA

本文讨论了使用JPA进行对象相关映射,并具有高级功能,例如缓存和懒惰加载。它涵盖了设置,实体映射和优化性能的最佳实践,同时突出潜在的陷阱。[159个字符]
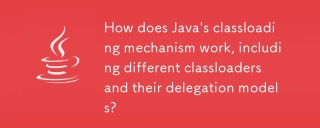
Java的类上载涉及使用带有引导,扩展程序和应用程序类负载器的分层系统加载,链接和初始化类。父代授权模型确保首先加载核心类别,从而影响自定义类LOA


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具
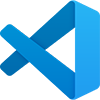
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器

DVWA
Damn Vulnerable Web App (DVWA) 是一个PHP/MySQL的Web应用程序,非常容易受到攻击。它的主要目标是成为安全专业人员在合法环境中测试自己的技能和工具的辅助工具,帮助Web开发人员更好地理解保护Web应用程序的过程,并帮助教师/学生在课堂环境中教授/学习Web应用程序安全。DVWA的目标是通过简单直接的界面练习一些最常见的Web漏洞,难度各不相同。请注意,该软件中

SublimeText3 Linux新版
SublimeText3 Linux最新版
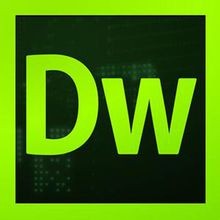
Dreamweaver CS6
视觉化网页开发工具

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。