砍价活动是流行于电商和社交平台的一种促销形式,参与者可以在一定时间内通过砍价来获得商品的优惠价格。然而,砍价的逻辑实现并不简单,需要考虑到参与者之间的关系以及价格的控制等问题。
本文将介绍如何使用Golang实现砍价的逻辑。
一、砍价的基本逻辑
砍价的基本逻辑可以概括如下:
- 创建砍价活动:活动发起者选择一个商品,设定原价和砍价周期,并邀请其他人参与砍价。
- 参与砍价:活动参与者通过发起砍价来降低商品价格。砍价幅度由系统随机确定,但不会低于一个最小值。
- 分享砍价:参与者可以通过分享砍价链接来邀请更多人参与砍价,并增加自己的砍价机会。
- 砍价成功:当商品价格降到一定程度时,砍价视为成功,用户可以获得相应优惠。
二、Golang实现砍价逻辑
下面我们来介绍如何使用Golang实现砍价逻辑。首先我们需要定义一些数据结构:
- 商品信息
type Product struct {
ID int // 商品ID Name string // 商品名称 OriginalPrice float32 // 商品原价 CurrentPrice float32 // 当前价格 MinPriceDelta float32 // 最小砍价幅度 MinPrice float32 // 最低价格 Participants map[int]*Participant // 参与者列表 ChoppedLogs map[int]float32 // 砍价日志 StartTime time.Time // 开始时间 EndTime time.Time // 结束时间
}
其中,Participants表示参与者的列表,ChoppedLogs记录用户每次砍价的幅度,StartTime和EndTime表示砍价周期。
- 参与者信息
type Participant struct {
ID int // 参与者ID Name string // 参与者名称 AmountChopped float32 // 已砍金额 JoinedTime time.Time // 加入时间 InviterID int // 邀请者ID ProductID int // 商品ID Invited []*Participant // 被邀请人列表
}
在参与者信息中,AmountChopped表示参与者在当前商品中已经砍下的金额,InviterID记录邀请者的ID,Invited记录被邀请者的列表。
- 砍价日志
type ChoppedLog struct {
ParticipantID int // 砍价者ID ChoppedAmount float32 // 砍价金额 ProductID int // 商品ID CreatedTime time.Time // 砍价时间
}
在砍价日志中,记录了砍价者的ID、砍价金额、商品ID以及砍价时间。
通过上述定义,我们可以写出如下的砍价逻辑:
- 创建砍价活动
func NewProduct(name string, originalPrice, minPriceDelta, minPrice float32, startTime, endTime time.Time) *Product {
return &Product{ Name: name, OriginalPrice: originalPrice, CurrentPrice: originalPrice, MinPriceDelta: minPriceDelta, MinPrice: minPrice, Participants: make(map[int]*Participant), ChoppedLogs: make(map[int]float32), StartTime: startTime, EndTime: endTime, }
}
- 参与砍价
func (p Product) Join(participant Participant) error {
if participant.JoinedTime.Before(p.StartTime) || participant.JoinedTime.After(p.EndTime) { return fmt.Errorf("参与时间错误") } if p.CurrentPrice <= p.MinPrice { return fmt.Errorf("价格已经到达最低价,不能再砍价了。") } id := len(p.Participants) + 1 participant.ID = id participant.ProductID = p.ID p.Participants[id] = participant return nil
}
- 分享砍价
func (p *Product) Invite(participantID, invitedID int) error {
if _, ok := p.Participants[participantID]; !ok { return fmt.Errorf("该用户未参加本次砍价活动") } if _, ok := p.Participants[invitedID]; !ok { return fmt.Errorf("该用户未在砍价活动中") } if participantID == invitedID { return fmt.Errorf("不允许自己邀请自己") } p.Participants[participantID].Invited = append(p.Participants[participantID].Invited, p.Participants[invitedID]) p.Participants[invitedID].InviterID = participantID return nil
}
- 砍价成功
func (p *Product) Chop(participantID int) error {
if _, ok := p.Participants[participantID]; !ok { return fmt.Errorf("该用户未参加本次砍价活动") } if p.CurrentPrice <= p.MinPrice { return fmt.Errorf("提前到达底价,不能再砍价了。") } num := rand.Intn(10) // 随机砍价幅度 chopAmount := p.MinPriceDelta + float32(num) if chopAmount >= p.CurrentPrice-p.MinPrice { chopAmount = p.CurrentPrice - p.MinPrice } p.CurrentPrice -= chopAmount p.Participants[participantID].AmountChopped += chopAmount p.ChoppedLogs[participantID] = chopAmount if p.CurrentPrice <= p.MinPrice { p.CurrentPrice = p.MinPrice } return nil
}
通过上述代码,我们可以实现基本的砍价逻辑,包括创建砍价活动、参与砍价、分享砍价和砍价成功等基本操作。但是,这些代码还远远不能满足实际应用的需求,因为我们还需要考虑到以下问题:
- 如何防止一些用户通过恶意砍价来砍到商品的底价?
- 如何控制砍价幅度,使得价格在一个合理的范围内波动?
- 如何设计砍价规则和邀请机制,使得砍价活动能够吸引更多的用户参与?
对于以上问题,我们也需要根据具体的业务需求加以处理。
三、总结
通过Golang实现砍价逻辑,可以让我们更好地理解砍价活动的实现原理。但是,在实际开发中,我们还需要考虑到其他问题,如并发处理、防止刷单等,这些问题也需要我们针对具体业务场景进行处理。相信在不断的实践中,我们可以逐步掌握砍价活动的实现技巧,为电商和社交平台的发展做出更大的贡献。
以上是砍价逻辑golang实现的详细内容。更多信息请关注PHP中文网其他相关文章!
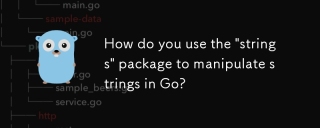
本文讨论了使用GO的“字符串”软件包进行字符串操作,详细介绍了共同的功能和最佳实践,以提高效率并有效地处理Unicode。
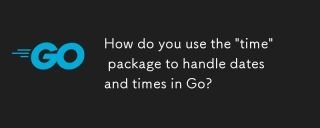
本文详细介绍了GO的“时间”包用于处理日期,时间和时区,包括获得当前时间,创建特定时间,解析字符串以及测量经过的时间。
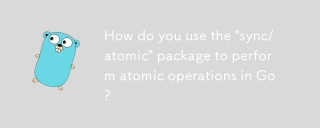
文章讨论了使用GO的“同步/原子”软件包进行并发编程中的原子操作,详细说明了其益处,例如防止比赛条件和提高性能。
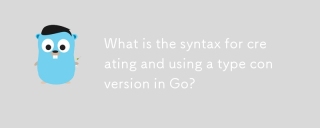
本文讨论了GO中的类型转换,包括语法,安全转换实践,常见的陷阱和学习资源。它强调明确的类型转换和错误处理。[159个字符]
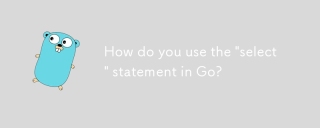
本文解释了在GO中使用“选择”语句来处理多个频道操作的使用,其与“开关”语句的差异以及常见用例,例如处理多个渠道,实现超时,非B


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。
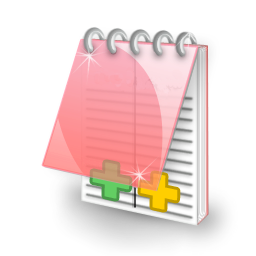
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

SublimeText3 英文版
推荐:为Win版本,支持代码提示!

SublimeText3 Linux新版
SublimeText3 Linux最新版

记事本++7.3.1
好用且免费的代码编辑器