在 PHP 开发中,我们常常需要判断一个值是否在数组中。如果某个值在数组中,我们可以直接使用 in_array() 函数来判断,但是如果某个值不在数组中,该如何判断呢?本文将介绍多种方法判断某个值在不在数组中。
方法一:使用 in_array() 函数取反
in_array() 函数可以判断一个值是否在数组中,如果不在,返回 false。
我们可以利用此特性来判断某个值不在数组中。
代码示例:
$needle = 'apple'; $fruits = ['banana', 'orange', 'grape']; if (!in_array($needle, $fruits)) { echo $needle . ' is not in the fruits array.'; }
输出结果:
apple is not in the fruits array.
方法二:使用 array_search() 函数
array_search() 函数可以在数组中搜索一个值,并返回该值的键名。如果值不在数组中,返回 false。
我们可以利用此特性来判断某个值不在数组中。
代码示例:
$needle = 'apple'; $fruits = ['banana', 'orange', 'grape']; if (array_search($needle, $fruits) === false) { echo $needle . ' is not in the fruits array.'; }
输出结果:
apple is not in the fruits array.
方法三:使用 array_diff() 函数
array_diff() 函数可以计算出两个或多个数组的差集,也就是说,它可以找出不在数组中的值。
我们可以将要判断的值作为一个只有一个元素的数组与原数组进行差集计算,如果差集的结果为空数组,则说明要判断的值不在原数组中。
代码示例:
$needle = 'apple'; $fruits = ['banana', 'orange', 'grape']; if (empty(array_diff([$needle], $fruits))) { echo $needle . ' is not in the fruits array.'; }
输出结果:
apple is not in the fruits array.
方法四:使用 count() 函数
我们可以使用 count() 函数获取数组中元素的个数,判断要查找的值在原数组中出现的次数,如果次数为 0,则说明该值不在原数组中。
代码示例:
$needle = 'apple'; $fruits = ['banana', 'orange', 'grape']; if (count(array_keys($fruits, $needle)) === 0) { echo $needle . ' is not in the fruits array.'; }
输出结果:
apple is not in the fruits array.
方法五:使用 foreach 循环
我们可以使用 foreach 循环遍历数组,查找要判断的值是否在数组中。如果遍历完数组仍然没有找到要判断的值,则说明该值不在数组中。
代码示例:
$needle = 'apple'; $fruits = ['banana', 'orange', 'grape']; $found = false; foreach ($fruits as $fruit) { if ($fruit === $needle) { $found = true; break; } } if (!$found) { echo $needle . ' is not in the fruits array.'; }
输出结果:
apple is not in the fruits array.
方法六:使用 array_key_exists() 函数
如果数组的键名是字符串,我们可以使用 array_key_exists() 函数判断某个键名是否在数组中存在。
代码示例:
$needle = 'apple'; $fruits = ['banana' => 1, 'orange' => 1, 'grape' => 1]; if (!array_key_exists($needle, $fruits)) { echo $needle . ' is not in the fruits array.'; }
输出结果:
apple is not in the fruits array.
结束语
本文介绍了多种方法判断某个值不在数组中。每种方法都有其优缺点,我们可以根据具体情况选择最适合的方法。在实际开发中,我们也可以结合具体场景,灵活运用这些技巧,提高开发效率和代码质量。
以上是php怎么判断某个值在不在数组中的详细内容。更多信息请关注PHP中文网其他相关文章!
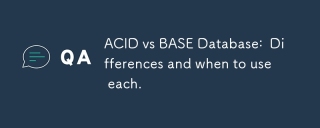
本文比较了酸和基本数据库模型,详细介绍了它们的特征和适当的用例。酸优先确定数据完整性和一致性,适合财务和电子商务应用程序,而基础则侧重于可用性和
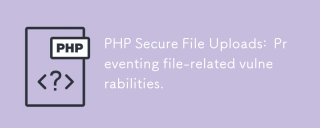
本文讨论了确保PHP文件上传的确保,以防止诸如代码注入之类的漏洞。它专注于文件类型验证,安全存储和错误处理以增强应用程序安全性。
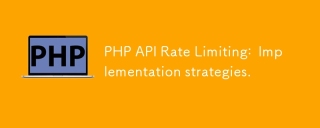
本文讨论了在PHP中实施API速率限制的策略,包括诸如令牌桶和漏水桶等算法,以及使用Symfony/Rate-limimiter之类的库。它还涵盖监视,动态调整速率限制和手
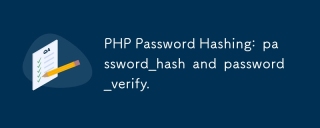
本文讨论了使用password_hash和pyspasswify在PHP中使用密码的好处。主要论点是,这些功能通过自动盐,强大的哈希算法和SECH来增强密码保护
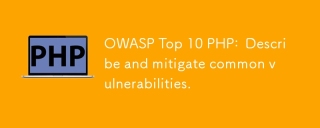
本文讨论了OWASP在PHP和缓解策略中的十大漏洞。关键问题包括注射,验证损坏和XSS,并提供用于监视和保护PHP应用程序的推荐工具。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

SublimeText3汉化版
中文版,非常好用
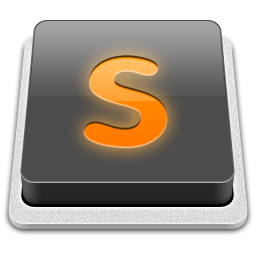
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。
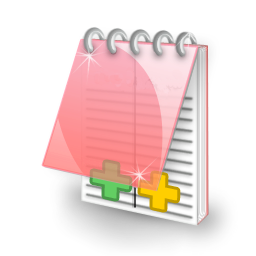
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

DVWA
Damn Vulnerable Web App (DVWA) 是一个PHP/MySQL的Web应用程序,非常容易受到攻击。它的主要目标是成为安全专业人员在合法环境中测试自己的技能和工具的辅助工具,帮助Web开发人员更好地理解保护Web应用程序的过程,并帮助教师/学生在课堂环境中教授/学习Web应用程序安全。DVWA的目标是通过简单直接的界面练习一些最常见的Web漏洞,难度各不相同。请注意,该软件中