Node.js是基于JavaScript的任意代码运行时,它广泛应用于服务端开发。在Node.js中,我们经常会遇到复杂的数组操作,因此本文将介绍如何在Node.js中处理数组。
- 创建数组
在Node.js中创建数组有两种方式:使用字面量和使用构造函数。
使用字面量:
const arr = [1, 2, 3, 4, 5];
使用构造函数:
const arr = new Array(1, 2, 3, 4, 5);
- 访问数组元素
要访问数组元素,我们可以使用数组索引。在Node.js中,数组索引从0开始。
const arr = [1, 2, 3, 4, 5]; console.log(arr[0]); //输出1 console.log(arr[2]); //输出3
- 遍历数组
在Node.js中,我们可以使用for循环、forEach()、map()等方法来遍历数组。
使用for循环:
const arr = [1, 2, 3, 4, 5]; for(let i = 0; i < arr.length; i++) { console.log(arr[i]); }
使用forEach():
const arr = [1, 2, 3, 4, 5]; arr.forEach(value => { console.log(value); })
使用map():
const arr = [1, 2, 3, 4, 5]; const newArr = arr.map(value => value * 2); console.log(newArr); //输出[2, 4, 6, 8, 10]
- 添加和删除元素
在Node.js中,可以使用push()和pop()方法添加和删除元素。
使用push()方法添加元素:
const arr = [1, 2, 3, 4, 5]; arr.push(6); console.log(arr); //输出[1, 2, 3, 4, 5, 6]
使用pop()方法删除元素:
const arr = [1, 2, 3, 4, 5]; arr.pop(); console.log(arr); //输出[1, 2, 3, 4]
- 数组排序
在Node.js中,可以使用sort()方法对数组进行排序。
const arr = [5, 2, 1, 4, 3]; arr.sort(); console.log(arr); //输出[1, 2, 3, 4, 5]
- 数组查找
在Node.js中,可以使用indexOf()和includes()方法查找数组元素。
使用indexOf():
const arr = [1, 2, 3, 4, 5]; console.log(arr.indexOf(3)); //输出2 console.log(arr.indexOf(6)); //输出-1
使用includes():
const arr = [1, 2, 3, 4, 5]; console.log(arr.includes(3)); //输出true console.log(arr.includes(6)); //输出false
- 数组过滤
在Node.js中,可以使用filter()方法对数组进行过滤。
const arr = [1, 2, 3, 4, 5]; const newArr = arr.filter(value => value > 3); console.log(newArr); //输出[4, 5]
- 数组拼接
在Node.js中,可以使用concat()方法对数组进行拼接。
const arr1 = [1, 2, 3]; const arr2 = [4, 5, 6]; const newArr = arr1.concat(arr2); console.log(newArr); //输出[1, 2, 3, 4, 5, 6]
总结:
以上就是在Node.js中处理数组的一些基础操作。了解这些操作可以在实际开发中更加方便地进行数组处理。同时,Node.js提供了许多其他的数组操作方法,如reduce()、find()、slice()等,这些高级方法也值得掌握。
以上是nodejs中怎么处理数组的详细内容。更多信息请关注PHP中文网其他相关文章!

Include:1)AsteeplearningCurvedUetoItsVasteCosystem,2)SeochallengesWithClient-SiderEndering,3)潜在的PersperformanceissuesInsuesInlArgeApplications,4)ComplexStateStateManagementAsappsgrow和5)TheneedtokeEedtokeEedtokeEppwithitsrapideDrapidevoltolution.thereedtokeEppectortorservolution.thereedthersrapidevolution.ththesefactorsshesssheou

reactischallengingforbeginnersduetoitssteplearningcurveandparadigmshifttocoment oparchitecent.1)startwithofficialdocumentationforasolidFoundation.2)了解jsxandhowtoembedjavascriptwithinit.3)

ThecorechallengeingeneratingstableanduniquekeysfordynamiclistsinReactisensuringconsistentidentifiersacrossre-rendersforefficientDOMupdates.1)Usenaturalkeyswhenpossible,astheyarereliableifuniqueandstable.2)Generatesynthetickeysbasedonmultipleattribute

javascriptfatigueinrectismanagbaiblewithstrategiesLike just just in-timelearninganning and CuratedInformationsources.1)学习whatyouneedwhenyouneedit

totlecteactComponents通过theusestatehook,使用jestandReaCtteTingLibraryToSigulation Interactions andverifyStatAtaTeChangesInTheUI.1)renderthecomponentAndComponentAndComponentAndCheckInitialState.2)模拟useclicklicksorformsormissionsions.3)

KeysinreactarecrucialforopTimizingPerformanceByingIneFefitedListupDates.1)useKeyStoIndentifyAndTrackListelements.2)避免使用ArrayIndicesasKeystopreventperformansissues.3)ChooSestableIdentifierslikeIdentifierSlikeItem.idtomaintainAinainCommaintOnconMaintOmentStateAteanDimpperperFermerfermperfermerformperfermerformfermerformfermerformfermerment.ChosestopReventPerformissues.3)

ReactKeySareUniqueIdentifiers usedwhenrenderingListstoimprovereConciliation效率。1)heelPreactrackChangesInListItems,2)使用StableanDuniqueIdentifiersLikeItifiersLikeItemidSisRecumended,3)避免使用ArrayIndicesaskeyindicesaskeystopreventopReventOpReventSissUseSuseSuseWithReRefers和4)

独特的keysarecrucialinreactforoptimizingRendering和MaintainingComponentStateTegrity.1)useanaturalAlaluniqueIdentifierFromyourDataiFabable.2)ifnonaturalalientedifierexistsistsists,generateauniqueKeyniqueKeyKeyLiquekeyperaliqeyAliqueLiqueAlighatiSaliqueLiberaryLlikikeuuId.3)deversearrayIndiceSaskeyseSecialIndiceSeasseAsialIndiceAseAsialIndiceAsiall


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器
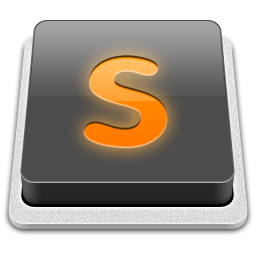
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

SecLists
SecLists是最终安全测试人员的伙伴。它是一个包含各种类型列表的集合,这些列表在安全评估过程中经常使用,都在一个地方。SecLists通过方便地提供安全测试人员可能需要的所有列表,帮助提高安全测试的效率和生产力。列表类型包括用户名、密码、URL、模糊测试有效载荷、敏感数据模式、Web shell等等。测试人员只需将此存储库拉到新的测试机上,他就可以访问到所需的每种类型的列表。

SublimeText3汉化版
中文版,非常好用
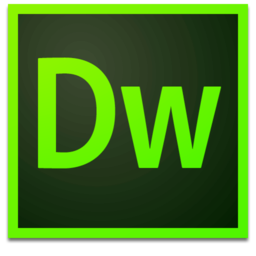
Dreamweaver Mac版
视觉化网页开发工具