Golang 是一种非常流行的编程语言,由 Google 开发,因其高效率和卓越的性能而备受开发者喜爱。虽然 Golang 受到许多开发者的青睐,但对于使用 Golang 来实现 SOAP 的开发者而言,相关的指导和资源相对较少。在这篇文章中,我们将介绍 Golang 如何实现 SOAP 的过程。
首先,让我们来简单了解一下 SOAP。SOAP (Simple Object Access Protocol) 是基于 XML 的协议,用于在 Web 应用程序之间交换信息。SOAP 一般与 WSDL (Web Services Description Language) 和 UDDI (Universal Description, Discovery, and Integration) 一起使用。Web 服务一般包含以下内容:一个以 SOAP 为协议的请求和响应消息,用于发送和接收数据;以及一个 WSDL 文件,其中包含有关可用 Web 服务的信息。
要在 Golang 中实现 SOAP,我们需要使用相应的 Go 库。目前较为流行的有以下两种:
- Go-SOAP:一个相对较新的库,用于解析和构建 SOAP 协议。它支持 SOAP 1.1 和 SOAP 1.2,并实现了 WSDL 1.1 契约的一部分。它还支持 HTTP 和 HTTPS 传输,使用了 Go 语言的默认 HTTP 客户端进行 API 请求。
- Gosoap:一个较为老旧的库,支持 SOAP 1.1 和 SOAP 1.2 (附加标准),但不支持 WSDL。它还支持 HTTP 和 HTTPS 传输,使用了 Go 语言的默认 HTTP 客户端进行 API 请求。
在这篇文章中,我们将介绍如何使用 Go-SOAP 库来实现 SOAP 协议。首先,我们需要在 Go 项目中添加 go-soap 包。可以使用以下指令进行安装:
go get -u github.com/tiaguinho/go-soap
然后,我们需要定义 SOAP Web 服务的请求。请求将基于 XML 进行构建,以下是一个示例请求:
<soapenv:Envelope xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:yt="urn:youtube"> <soapenv:Header/> <soapenv:Body> <yt:GetVideoInformation> <yt:VideoID>xxxxxxxxxxx</yt:VideoID> </yt:GetVideoInformation> </soapenv:Body> </soapenv:Envelope>
上述示例中,请求的名称为 GetVideoInformation,参数值为 VideoID = xxxxxxxxxxx。
接下来,我们需要定义 SOAP Web 服务的响应,这同样是 XML 格式,以下是示例响应:
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:yt="urn:youtube"> <soapenv:Header/> <soapenv:Body> <yt:GetVideoInformationResponse> <yt:Title>Title of the Video</yt:Title> <yt:Description>Multiline Description</yt:Description> </yt:GetVideoInformationResponse> </soapenv:Body> </soapenv:Envelope>
在结构中,需要包含 SOAP Web 服务的地址、函数名称、请求和响应格式。以下是代码示例:
import ( "net/url" "github.com/tiaguinho/go-soap" ) // SOAP 请求体 type GetVideoInformationRequestEnvelope struct { SOAPEnv string `xml:"xmlns:soapenv,attr"` XSI string `xml:"xmlns:xsi,attr"` Yt string `xml:"xmlns:yt,attr"` Body GetVideoInformationRequestBody } // SOAP 请求部分 type GetVideoInformationRequestBody struct { GetVideoInformation YoutubeRequest } // Youtube Request type YoutubeRequest struct { VideoID string } // SOAP 响应体 type GetVideoInformationResponseEnvelope struct { SOAPEnv string `xml:"xmlns:soapenv,attr"` Yt string `xml:"xmlns:yt,attr"` Body GetVideoInformationResponseBody } // SOAP 响应部分 type GetVideoInformationResponseBody struct { GetVideoInformationResponse YoutubeResponse } // Youtube Response type YoutubeResponse struct { Title string `xml:"Title"` Description string `xml:"Description"` } func main() { // 服务地址 soapURL, _ := url.Parse("http://example.com/soap") soapClient := soap.NewClient(soapURL) // 函数名称 soapRequest, err := soapClient.NewRequest("http://www.youtube.com", "GetVideoInformation") if err != nil { log.Fatalln(err) } // 填写请求信息 soapRequest.Body = &GetVideoInformationRequestEnvelope{ XSI: "http://www.w3.org/2001/XMLSchema-instance", SOAPEnv: "http://schemas.xmlsoap.org/soap/envelope/", Yt: "urn:youtube", Body: GetVideoInformationRequestBody{ GetVideoInformation: YoutubeRequest{ VideoID: "xxxxxxxxxxx", }, }, } // 发送请求 soapResponse, err := soapClient.Do(soapRequest) if err != nil { log.Fatalln(err) } // 解析响应数据 var result GetVideoInformationResponseEnvelope if err := soapResponse.Unmarshal(&result); err != nil { log.Fatalln(err) } // 打印结果 fmt.Println("Title:", result.Body.GetVideoInformationResponse.Title) fmt.Println("Description:", result.Body.GetVideoInformationResponse.Description) }
在现代的 Web 开发中,SOAP 已经被 REST 和 JSON 所取代,但在某些特定场景下,SOAP 协议仍然在使用。如果您正在寻找一种使用 Golang 实现 SOAP 的方法,上述示例将帮助您入门。Golang 的高效性和并发模型使其成为用于 Web 服务的强大工具,这使开发人员更加便捷和高效地完成工作。享受 Golang 的乐趣吧!
以上是Golang如何实现SOAP的过程的详细内容。更多信息请关注PHP中文网其他相关文章!
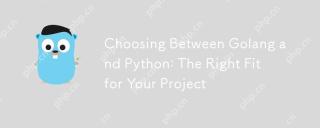
golangisidealforperformance-Critical-clitageAppations and ConcurrentPrompromming,而毛皮刺激性,快速播种和可及性。1)forhigh-porformanceneeds,pelectgolangduetoitsefefsefefseffifeficefsefeflicefsiveficefsiveandconcurrencyfeatures.2)fordataa-fordataa-fordata-fordata-driventriventriventriventriventrivendissp pynonnononesp
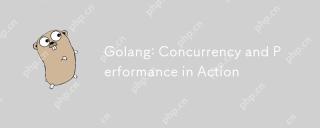
Golang通过goroutine和channel实现高效并发:1.goroutine是轻量级线程,使用go关键字启动;2.channel用于goroutine间安全通信,避免竞态条件;3.使用示例展示了基本和高级用法;4.常见错误包括死锁和数据竞争,可用gorun-race检测;5.性能优化建议减少channel使用,合理设置goroutine数量,使用sync.Pool管理内存。
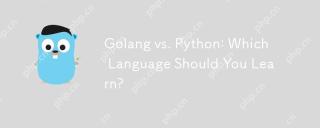
Golang更适合系统编程和高并发应用,Python更适合数据科学和快速开发。1)Golang由Google开发,静态类型,强调简洁性和高效性,适合高并发场景。2)Python由GuidovanRossum创造,动态类型,语法简洁,应用广泛,适合初学者和数据处理。
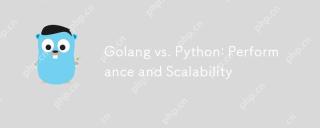
Golang在性能和可扩展性方面优于Python。1)Golang的编译型特性和高效并发模型使其在高并发场景下表现出色。2)Python作为解释型语言,执行速度较慢,但通过工具如Cython可优化性能。
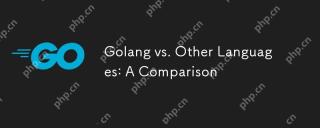
Go语言在并发编程、性能、学习曲线等方面有独特优势:1.并发编程通过goroutine和channel实现,轻量高效。2.编译速度快,运行性能接近C语言。3.语法简洁,学习曲线平缓,生态系统丰富。
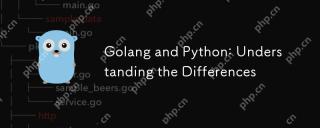
Golang和Python的主要区别在于并发模型、类型系统、性能和执行速度。1.Golang使用CSP模型,适用于高并发任务;Python依赖多线程和GIL,适合I/O密集型任务。2.Golang是静态类型,Python是动态类型。3.Golang编译型语言执行速度快,Python解释型语言开发速度快。
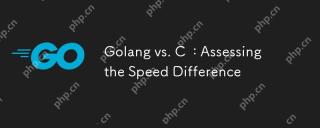
Golang通常比C 慢,但Golang在并发编程和开发效率上更具优势:1)Golang的垃圾回收和并发模型使其在高并发场景下表现出色;2)C 通过手动内存管理和硬件优化获得更高性能,但开发复杂度较高。
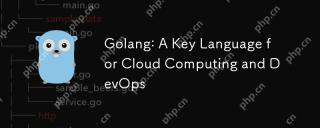
Golang在云计算和DevOps中的应用广泛,其优势在于简单性、高效性和并发编程能力。1)在云计算中,Golang通过goroutine和channel机制高效处理并发请求。2)在DevOps中,Golang的快速编译和跨平台特性使其成为自动化工具的首选。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具
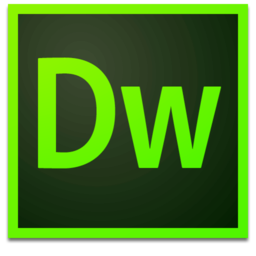
Dreamweaver Mac版
视觉化网页开发工具

记事本++7.3.1
好用且免费的代码编辑器

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。