随着PHP版本升级,一些函数已经废弃,例如加密和解密函数,在PHP7.1之前,通过mcrypt_decrypt()和mcrypt_encrypt()实现解密和加密,在PHP7.1之后通过openssl_decrypt()和openssl_encrypt()实现解密和加密。在此,通过封装类,将两种实现的方式实现
两种方式的加密解密步骤:
解密时返回原始数据(数组—->加密—->加密串—->解密—->原始数组 || JSON字符串—->加密—->加密串—->解密—->原始JSON字符串 || 字符串—->加密—->加密串—->解密—->原始字符串)
PHP7.1以下使用
类方法
该类中需要注意的是密钥: 算法所对应的 key 长度:AES-128, 192 and 256 的加密 key 的长度分别为 16, 24 和 32 位
<?php namespace aes; class AesT { private $_bit = MCRYPT_RIJNDAEL_256; private $_type = MCRYPT_MODE_CBC; private $_key = 'ThisIsYourAesKey'; // 密钥 必须16位 24位 32位 private $_use_base64 = true; private $_iv_size = null; private $_iv = null; /** * @param string $_key 密钥 * @param int $_bit 默认使用128字节 支持256、192、128 * @param string $_type 加密解密方式 默认为ecb 支持cfb、cbc、nofb、ofb、stream、ecb * @param boolean $_use_base64 默认使用base64二次加密 */ public function __construct($_key = '', $_bit = 128, $_type = 'ecb', $_use_base64 = true) { // 加密字节 if (192 === $_bit) { $this->_bit = MCRYPT_RIJNDAEL_192; } elseif (128 === $_bit) { $this->_bit = MCRYPT_RIJNDAEL_128; } else { $this->_bit = MCRYPT_RIJNDAEL_256; } // 加密方法 if ('cfb' === $_type) { $this->_type = MCRYPT_MODE_CFB; } elseif ('cbc' === $_type) { $this->_type = MCRYPT_MODE_CBC; } elseif ('nofb' === $_type) { $this->_type = MCRYPT_MODE_NOFB; } elseif ('ofb' === $_type) { $this->_type = MCRYPT_MODE_OFB; } elseif ('stream' === $_type) { $this->_type = MCRYPT_MODE_STREAM; } else { $this->_type = MCRYPT_MODE_ECB; } // 密钥 if (!empty($_key)) { $this->_key = $_key; } // 是否使用base64 $this->_use_base64 = $_use_base64; $this->_iv_size = mcrypt_get_iv_size($this->_bit, $this->_type); $this->_iv = mcrypt_create_iv($this->_iv_size, MCRYPT_RAND); } /** * 加密 * @param string $string 待加密字符串 * @return string */ public function encode($string) { // if (MCRYPT_MODE_ECB === $this->_type) { $encodeString = mcrypt_encrypt($this->_bit, $this->_key, json_encode($string), $this->_type); } else { $encodeString = mcrypt_encrypt($this->_bit, $this->_key, json_encode($string), $this->_type, $this->_iv); } if ($this->_use_base64) { $encodeString = base64_encode($encodeString); } return $encodeString; } /** * 解密 * @param string $string 待解密字符串 * @return string */ public function decode($string) { if ($this->_use_base64) { $string = base64_decode($string); } if (MCRYPT_MODE_ECB === $this->_type) { $decodeString = mcrypt_decrypt($this->_bit, $this->_key, $string, $this->_type); } else { $decodeString = mcrypt_decrypt($this->_bit, $this->_key, $string, $this->_type, $this->_iv); } return $decodeString; } }
使用
<?php use aes\AesT; class Test{ public function index() { $data1 = ['status' => '1', 'info' => 'success', 'data' => [['id' => 1, 'name' => '大房间', '2' => '小房间']]]; //$data2 = '{"status":"1","info":"success","data":[{"id":1,"name":"\u5927\u623f\u95f4","2":"\u5c0f\u623f\u95f4"}]}'; //$data3 = 'PHP AES cbc模式 pkcs7 128加密解密'; $objT = new AesT(); $resT = $objT->encode($data1);//加密数据 var_dump($resT); var_dump($objT->decode($resT));//解密 } }
PHP7.1以上使用
类方法
AES-128-CBC, AES-192-CBC和AES-256-CBC 的加密 key 的长度分别为 16, 24 和 32 位
openssl_encrypt()的四个参数中,需要注意的是第四个参数:
0 : 自动对明文进行 padding, 返回的数据经过 base64 编码.
1 : OPENSSL_RAW_DATA, 自动对明文进行 padding, 但返回的结果未经过 base64 编码.
2 : OPENSSL_ZERO_PADDING, 自动对明文进行 0 填充, 返回的结果经过 base64 编码
<?php namespace aes; class Aes { public static $key = ''; public static $iv = ''; public static $method = ''; public function __construct() { //加密key self::$key = md5('AeSNJkBfhHmJqLzHL', true); //保证偏移量为16位 self::$iv = md5('HfgUdCliBjKjuRfa', true); //加密方式 # AES-128-CBC AES-192-CBC AES-256-CBC self::$method = 'AES-128-CBC'; } /** * @desc 加密 * @param $data * @return false|string */ public function aesEn($data) { return base64_encode(openssl_encrypt(json_encode($data), self::$method, self::$key, OPENSSL_RAW_DATA, self::$iv)); } /** * @desc 解密 * @param $data * @return false|string */ public function aesDe($data) { $tmpData = openssl_decrypt(base64_decode($data), self::$method, self::$key, OPENSSL_RAW_DATA, self::$iv); return is_null(json_decode($tmpData)) ? $tmpData : json_decode($tmpData, true); } }
使用
<?php use aes\Aes; class Test { public function aes() { $obj = new Aes(); $data = ['status' => '1', 'info' => 'success', 'data' => [['id' => 1, 'name' => '大房间', '2' => '小房间']]]; //$data = '{"status":"1","info":"success","data":[{"id":1,"name":"\u5927\u623f\u95f4","2":"\u5c0f\u623f\u95f4"}]}'; //$data = 'PHP AES cbc模式 pkcs7 128加密解密'; $res = $obj->aesEn($data);//加密数据 var_dump($res); var_dump($obj->aesDe($res));//解密 } }
使用该加密解密类时,如果将两个类整合到一个类中,需要通过PHP_VERSION获取当前PHP版本
以上是数据加密解密的详细内容。更多信息请关注PHP中文网其他相关文章!
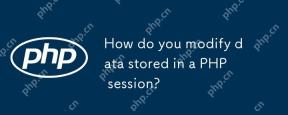
tomodifyDataNaphPsession,startTheSessionWithSession_start(),然后使用$ _sessionToset,修改,orremovevariables.1)startThesession.2)setthesession.2)使用$ _session.3)setormodifysessessvariables.3)emovervariableswithunset()
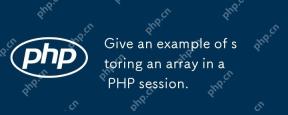
在PHP会话中可以存储数组。1.启动会话,使用session_start()。2.创建数组并存储在$_SESSION中。3.通过$_SESSION检索数组。4.优化会话数据以提升性能。
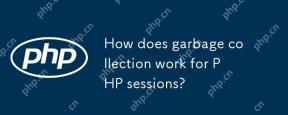
PHP会话垃圾回收通过概率机制触发,清理过期会话数据。1)配置文件中设置触发概率和会话生命周期;2)可使用cron任务优化高负载应用;3)需平衡垃圾回收频率与性能,避免数据丢失。
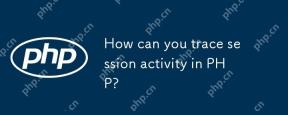
PHP中追踪用户会话活动通过会话管理实现。1)使用session_start()启动会话。2)通过$_SESSION数组存储和访问数据。3)调用session_destroy()结束会话。会话追踪用于用户行为分析、安全监控和性能优化。
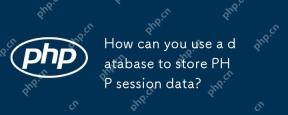
利用数据库存储PHP会话数据可以提高性能和可扩展性。1)配置MySQL存储会话数据:在php.ini或PHP代码中设置会话处理器。2)实现自定义会话处理器:定义open、close、read、write等函数与数据库交互。3)优化和最佳实践:使用索引、缓存、数据压缩和分布式存储来提升性能。
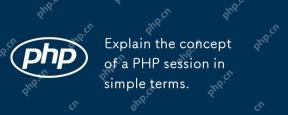
phpsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIdStoredInacookie.here'showtomanageThemeffectionaly:1)startAsessionWithSessionwwithSession_start()和stordoredAtain $ _session.2)
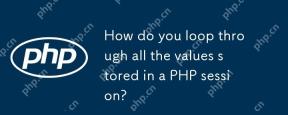
在PHP中,遍历会话数据可以通过以下步骤实现:1.使用session_start()启动会话。2.通过foreach循环遍历$_SESSION数组中的所有键值对。3.处理复杂数据结构时,使用is_array()或is_object()函数,并用print_r()输出详细信息。4.优化遍历时,可采用分页处理,避免一次性处理大量数据。这将帮助你在实际项目中更有效地管理和使用PHP会话数据。
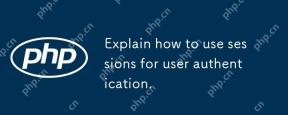
会话通过服务器端的状态管理机制实现用户认证。1)会话创建并生成唯一ID,2)ID通过cookies传递,3)服务器存储并通过ID访问会话数据,4)实现用户认证和状态管理,提升应用安全性和用户体验。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。

SublimeText3汉化版
中文版,非常好用
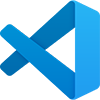
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器

Atom编辑器mac版下载
最流行的的开源编辑器

SublimeText3 英文版
推荐:为Win版本,支持代码提示!