1、删除模型
1.1 使用delete删除模型
删除模型很简单,先获取要删除的模型实例,然后调用delete方法即可:
$post = Post::find(5); if($post->delete()){ echo '删除文章成功!'; }else{ echo '删除文章失败!'; }
该方法返回true或false。
1.2 使用destroy删除模型
当然如果已知要删除的模型id的话,可以用更简单的方法destroy直接删除:
$deleted = Post::destroy(5);
你也可以一次传入多个模型id删除多个模型:
$deleted = Post::destroy([1,2,3,4,5]);
调用destroy方法返回被删除的记录数。
1.3 使用查询构建器删除模型
既然前面提到Eloquent模型本身就是查询构建器,也可以使用查询构建器风格删除模型,比如我们要删除所有浏览数为0的文章,可以使用如下方式:
$deleted = Models\Post::where('views', 0)->delete();
返回结果为被删除的文章数。
2、软删除实现
上述删除方法都会将数据表记录从数据库删除,此外Eloquent模型还支持软删除。
所谓软删除指的是数据表记录并未真的从数据库删除,而是将表记录的标识状态标记为软删除,这样在查询的时候就可以加以过滤,让对应表记录看上去是被”删除“了。Laravel中使用了一个日期字段作为标识状态,这个日期字段可以自定义,这里我们使用deleted_at,如果对应模型被软删除,则deleted_at字段的值为删除时间,否则该值为空。
要让Eloquent模型支持软删除,还要做一些设置。首先在模型类中要使用SoftDeletestrait,该trait为软删除提供一系列相关方法,具体可参考源码Illuminate\Database\Eloquent\SoftDeletes,此外还要设置$date属性数组,将deleted_at置于其中:
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\SoftDeletes; class Post extends Model { use SoftDeletes; //设置表名 public $table = 'posts'; //设置主键 public $primaryKey = 'id'; //设置日期时间格式 public $dateFormat = 'U'; protected $guarded = ['id','views','user_id','updated_at','created_at']; protected $dates = ['delete_at']; }
然后对应的数据库posts中添加deleted_at列,我们使用迁移来实现,先执行Artisan命令:
php artisan make:migration alter_posts_deleted_at --table=posts
然后编辑生成的PHP文件如下:
<?php use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class AlterPostsDeletedAt extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::table('posts', function (Blueprint $table) { $table->softDeletes(); }); } ...//其它方法 }
然后运行:
php artisan migrate
这样posts中就有了deleted_at列。接下来,我们在控制器中编写测试代码:
$post = Post::find(6); $post->delete(); if($post->trashed()){ echo '软删除成功!'; dd($post); }else{ echo '软删除失败!'; }
那如果想要在查询结果中包含软删除的记录呢?可以使用SoftDeletes trait上的withTrashed方法:
$posts = Post::withTrashed()->get(); dd($posts);
有时候我们只想要查看被软删除的模型,这也有招,通过SoftDeletes上的onlyTrashed方法即可:
$posts = Post::onlyTrashed()->get(); dd($posts);
软删除恢复
有时候我们需要恢复被软删除的模型,可以使用SoftDeletes提供的restore方法:
恢复单个模型
$post = Post::find(6); $post->restore();
有点问题,ID为6的已经被软删除了,Post::find(6) 是查不到数据的,
应该
$post = Post::withTrashed()->find(6); $post->restore();
恢复多个模型
Post::withTrashed()->where('id','>',1)->restore();
恢复所有模型
Post::withTrashed()->restore();
恢复关联查询模型
$post = Post::find(6); $post->history()->restore();
强制删除
如果模型配置了软删除但我们确实要删除改模型对应数据库表记录,则可以使用SoftDeletes提供的forceDelete方法:
$post = Post::find(6); $post->forceDelete();
PHP中文网,大量的免费laravel入门教程,欢迎在线学习!
本文转自:https://blog.csdn.net/weixin_38112233/article/details/78574007
以上是一文了解laravel模型删除和软删除的详细内容。更多信息请关注PHP中文网其他相关文章!

协作文档编辑是分布式团队优化工作流程的有效工具。它通过实时协作和反馈循环提升沟通和项目进度,常用工具包括GoogleDocs、MicrosoftTeams和Notion。使用时需注意版本控制和学习曲线等挑战。

ThepreviousversionofLaravelissupportedwithbugfixesforsixmonthsandsecurityfixesforoneyearafteranewmajorversion'srelease.Understandingthissupporttimelineiscrucialforplanningupgrades,ensuringprojectstability,andleveragingnewfeaturesandsecurityenhancemen

Laravelcanbeeffectivelyusedforbothfrontendandbackenddevelopment.1)Backend:UtilizeLaravel'sEloquentORMforsimplifieddatabaseinteractions.2)Frontend:LeverageBladetemplatesforcleanHTMLandintegrateVue.jsfordynamicSPAs,ensuringseamlessfrontend-backendinteg

LaravelcanbeusedforfullstackDevelopment.1)BackendMasteryWithlaravel'sexpressiversyntaxAndFeaturesLikeElikeElikeEloquentormfordatabaseMemangement.2)FrontendIntIntegration usingbladebladynamichtegration bladynamichtmltmltemplates.3)增强fradeffordynamichtmltemplate)

答案:升级Laravel的最佳工具包括Laravel的UpgradeGuide、LaravelShift、Rector、Composer和LaravelPint。1.使用Laravel的UpgradeGuide作为升级路线图。2.利用LaravelShift自动化大部分升级工作,但需人工复查。3.通过Rector自动重构代码,需理解并可能自定义其规则。4.用Composer管理依赖,需注意可能的依赖冲突。5.运行LaravelPint保持代码风格一致性,但它不解决功能问题。

ToenhanceGaimentAndCohesionAmongDistributedTeamSbeyondzoom,实施策略:1)组织virtualCoffeebreaksForinfornformalChats,2)useassynchronoustoolslikeslikeslikeslikeslikeslikslackfornon worksdiscusions,3)介绍present cuctuceGamificationgamificeGamificationgamificationgamificationWithteamGameGameSorchallEngEsorChallEngEnsErchallEnges,and4)

Laravel10 IntroducesseveralbreakingChanges:1)Itrequiresphp8.1orhigher,2)TherOuteserviceProviderNowSabootMethodForloadingRoutes,3)thewithTimestAmpAmpAmps()MethodOneLoquentRectrationShipsissississisdeprected,and4))

tomaintainfocusandmotivationInremotework,createStructuredEnvorment,托管式构成,促进性,促进性通过socialescialactionsions andgoalsetting,维持工作劳动生平,维持且苏联核酸盐学。1)setupadeDedworkspadedworkspacepaceandstickeandsticketicktickticktoorine aroutine。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具
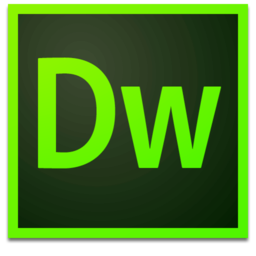
Dreamweaver Mac版
视觉化网页开发工具

SecLists
SecLists是最终安全测试人员的伙伴。它是一个包含各种类型列表的集合,这些列表在安全评估过程中经常使用,都在一个地方。SecLists通过方便地提供安全测试人员可能需要的所有列表,帮助提高安全测试的效率和生产力。列表类型包括用户名、密码、URL、模糊测试有效载荷、敏感数据模式、Web shell等等。测试人员只需将此存储库拉到新的测试机上,他就可以访问到所需的每种类型的列表。

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),

SublimeText3 英文版
推荐:为Win版本,支持代码提示!
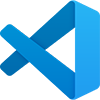
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器