collections 是 python 的内置模块,源码位于 Lib/collections/init.py ,该模块提供了通用的数据容器。
deque 容器对象
通过 from collections import deque 引入,创建 deque 容器对象时,可通过设置参数为 Iterable 对象(如 tuple,list,str)或 maxlen=x(int类型) or None 进行初始化。
deque 容器支持线程安全,通过 append 或 pop 对 deque 的两端进行插入或移除元素时,时间复杂度为 O(1)。与 list 对象相比,list 同样有相同的 api 实现相同的功能,但是对于 pop(0) 或 insert(0, x) 等对 list 的操作,时间复杂度为 O(n)。
如果在初始化 deque 时未声明 maxlen 或声明 maxlen=None,那么 deque 容器可以容纳任意多的元素,否则, deque 容器会被定义为有限长度的元素容器。
一旦容器中的元素个数达到设置的 maxlen,当有新的元素加入时,则会在加入元素一端的另一端排除相同个数的元素,这样可以保证当前 deque 中的元素全部是最新加入的元素。
deque 对象函数
append(x)
appendleft(x)
clear()
copy()
count(x):返回容器中值为 x 的元素个数
extend(iterable)
extendleft(iterable)
index(x):在容器中查到第一个值为 x 的元素索引,如果不存在,抛起 ValueError 异常
insert(idx, x)
pop()
popleft()
remove(x)
reverse():翻转容器中的元素,并返回 None
rotate(n)
deque 对象只读属性
maxlen
除了上述的对象函数外,由于 deque 对象也是 Iterable 对象,那么 len(deque);reversed(deque);copy.copy(deque);copy.deepcopy(deque) 等函数同样起作用,同样 in 操作符也在遍历 deque 操作时使用,切片操作 deque[-1] 也可以返回容器中最后一个元素。如果对容器中的随机元素进行操作的话,建议使用 list。
demo
获取文件中的 python 字符串所在的一行内容,和这行内容的前三行
from collections import deque def search(lines, pattern, maxlen): pre_lines = deque(maxlen=maxlen) for line in lines: if pattern in line: yield line, pre_lines pre_lines.append(line) if name == 'main': with(open('./test.txt')) as f: for line, pre_lines in search(f, 'python', 3): for pre_line in pre_lines: print(pre_line, end='') print(line)
输入文本文件内容为
c# c c++ javascript python java delphi python golang perl css html python
通过代码输出为
c c++ javascript python python java delphi python perl css html python
以上是Python之Collections内置模块详细说明的详细内容。更多信息请关注PHP中文网其他相关文章!
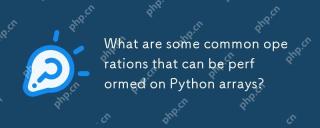
Pythonarrayssupportvariousoperations:1)Slicingextractssubsets,2)Appending/Extendingaddselements,3)Insertingplaceselementsatspecificpositions,4)Removingdeleteselements,5)Sorting/Reversingchangesorder,and6)Listcomprehensionscreatenewlistsbasedonexistin
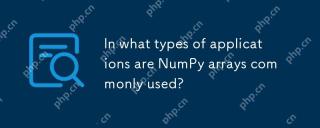
NumPyarraysareessentialforapplicationsrequiringefficientnumericalcomputationsanddatamanipulation.Theyarecrucialindatascience,machinelearning,physics,engineering,andfinanceduetotheirabilitytohandlelarge-scaledataefficiently.Forexample,infinancialanaly
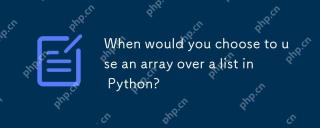
useanArray.ArarayoveralistinpythonwhendeAlingwithHomeSdata,performance-Caliticalcode,orinterFacingWithCcccode.1)同质性data:arrayssavememorywithtypedelements.2)绩效code-performance-clitionalcode-clitadialcode-critical-clitical-clitical-clitical-clitaine code:araysofferferbetterperperperformenterperformanceformanceformancefornalumericalicalialical.3)
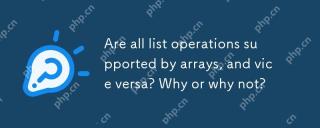
不,notalllistoperationsareSupportedByArrays,andviceversa.1)arraysdonotsupportdynamicoperationslikeappendorinsertwithoutresizing,wheremactssperformance.2)listssdonotguaranteeconeeconeconstanttanttanttanttanttanttanttanttimecomplecomecomecomplecomecomecomecomecomecomplecomectaccesslikearrikearraysodo。
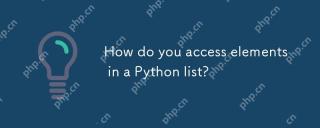
toAccesselementsInapythonlist,useIndIndexing,负索引,切片,口头化。1)indexingStartSat0.2)否定indexingAccessesessessessesfomtheend.3)slicingextractsportions.4)iterationerationUsistorationUsisturessoreTionsforloopsoreNumeratorseforeporloopsorenumerate.alwaysCheckListListListListlentePtotoVoidToavoIndexIndexIndexIndexIndexIndExerror。
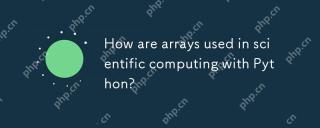
Arraysinpython,尤其是Vianumpy,ArecrucialInsCientificComputingfortheireftheireffertheireffertheirefferthe.1)Heasuedfornumerericalicerationalation,dataAnalysis和Machinelearning.2)Numpy'Simpy'Simpy'simplementIncressionSressirestrionsfasteroperoperoperationspasterationspasterationspasterationspasterationspasterationsthanpythonlists.3)inthanypythonlists.3)andAreseNableAblequick
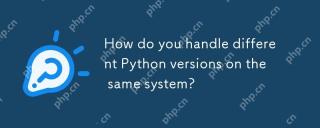
你可以通过使用pyenv、venv和Anaconda来管理不同的Python版本。1)使用pyenv管理多个Python版本:安装pyenv,设置全局和本地版本。2)使用venv创建虚拟环境以隔离项目依赖。3)使用Anaconda管理数据科学项目中的Python版本。4)保留系统Python用于系统级任务。通过这些工具和策略,你可以有效地管理不同版本的Python,确保项目顺利运行。
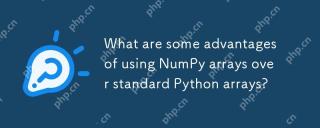
numpyarrayshaveseveraladagesoverandastardandpythonarrays:1)基于基于duetoc的iMplation,2)2)他们的aremoremoremorymorymoremorymoremorymoremorymoremoremory,尤其是WithlargedAtasets和3)效率化,效率化,矢量化函数函数函数函数构成和稳定性构成和稳定性的操作,制造


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。

Atom编辑器mac版下载
最流行的的开源编辑器
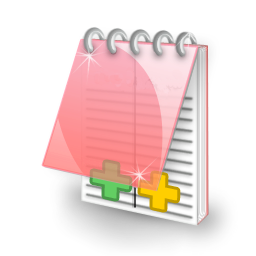
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

SublimeText3 英文版
推荐:为Win版本,支持代码提示!

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。