首先需要确认GD库是否正常,如果是合成图片,请确保把分解的图片放在frames的文件夹里面。
GIFEncoder.class.php 类
<? Class GIFEncoder { var $GIF = "GIF89a"; /* GIF header 6 bytes */ var $VER = "GIFEncoder V2.06"; /* Encoder version */ var $BUF = Array ( ); var $LOP = 0; var $DIS = 2; var $COL = -1; var $IMG = -1; var $ERR = Array ( 'ERR00' =>"Does not supported function for only one image!", 'ERR01' =>"Source is not a GIF image!", 'ERR02' =>"Unintelligible flag ", 'ERR03' =>"Could not make animation from animated GIF source", ); /* ::::::::::::::::::::::::::::::::::::::::::::::::::: :: :: GIFEncoder... :: */ function GIFEncoder ( $GIF_src, $GIF_dly, $GIF_lop, $GIF_dis, $GIF_red, $GIF_grn, $GIF_blu, $GIF_mod ) { if ( ! is_array ( $GIF_src ) && ! is_array ( $GIF_tim ) ) { printf ( "%s: %s", $this->VER, $this->ERR [ 'ERR00' ] ); exit ( 0 ); } $this->LOP = ( $GIF_lop > -1 ) ? $GIF_lop : 0; $this->DIS = ( $GIF_dis > -1 ) ? ( ( $GIF_dis < 3 ) ? $GIF_dis : 3 ) : 2; $this->COL = ( $GIF_red > -1 && $GIF_grn > -1 && $GIF_blu > -1 ) ? ( $GIF_red | ( $GIF_grn << 8 ) | ( $GIF_blu << 16 ) ) : -1; for ( $i = 0; $i < count ( $GIF_src ); $i++ ) { if ( strToLower ( $GIF_mod ) == "url" ) { $this->BUF [ ] = fread ( fopen ( $GIF_src [ $i ], "rb" ), filesize ( $GIF_src [ $i ] ) ); } else if ( strToLower ( $GIF_mod ) == "bin" ) { $this->BUF [ ] = $GIF_src [ $i ]; } else { printf ( "%s: %s ( %s )!", $this->VER, $this->ERR [ 'ERR02' ], $GIF_mod ); exit ( 0 ); } if ( substr ( $this->BUF [ $i ], 0, 6 ) != "GIF87a" && substr ( $this->BUF [ $i ], 0, 6 ) != "GIF89a" ) { printf ( "%s: %d %s", $this->VER, $i, $this->ERR [ 'ERR01' ] ); exit ( 0 ); } for ( $j = ( 13 + 3 * ( 2 << ( ord ( $this->BUF [ $i ] { 10 } ) & 0x07 ) ) ), $k = TRUE; $k; $j++ ) { switch ( $this->BUF [ $i ] { $j } ) { case "!": if ( ( substr ( $this->BUF [ $i ], ( $j + 3 ), 8 ) ) == "NETSCAPE" ) { printf ( "%s: %s ( %s source )!", $this->VER, $this->ERR [ 'ERR03' ], ( $i + 1 ) ); exit ( 0 ); } break; case ";": $k = FALSE; break; } } } GIFEncoder::GIFAddHeader ( ); for ( $i = 0; $i < count ( $this->BUF ); $i++ ) { GIFEncoder::GIFAddFrames ( $i, $GIF_dly [ $i ] ); } GIFEncoder::GIFAddFooter ( ); } /* ::::::::::::::::::::::::::::::::::::::::::::::::::: :: :: GIFAddHeader... :: */ function GIFAddHeader ( ) { $cmap = 0; if ( ord ( $this->BUF [ 0 ] { 10 } ) & 0x80 ) { $cmap = 3 * ( 2 << ( ord ( $this->BUF [ 0 ] { 10 } ) & 0x07 ) ); $this->GIF .= substr ( $this->BUF [ 0 ], 6, 7 ); $this->GIF .= substr ( $this->BUF [ 0 ], 13, $cmap ); $this->GIF .= "!\377\13NETSCAPE2.0\3\1" . GIFEncoder::GIFWord ( $this->LOP ) . "\0"; } } /* ::::::::::::::::::::::::::::::::::::::::::::::::::: :: :: GIFAddFrames... :: */ function GIFAddFrames ( $i, $d ) { $Locals_str = 13 + 3 * ( 2 << ( ord ( $this->BUF [ $i ] { 10 } ) & 0x07 ) ); $Locals_end = strlen ( $this->BUF [ $i ] ) - $Locals_str - 1; $Locals_tmp = substr ( $this->BUF [ $i ], $Locals_str, $Locals_end ); $Global_len = 2 << ( ord ( $this->BUF [ 0 ] { 10 } ) & 0x07 ); $Locals_len = 2 << ( ord ( $this->BUF [ $i ] { 10 } ) & 0x07 ); $Global_rgb = substr ( $this->BUF [ 0 ], 13, 3 * ( 2 << ( ord ( $this->BUF [ 0 ] { 10 } ) & 0x07 ) ) ); $Locals_rgb = substr ( $this->BUF [ $i ], 13, 3 * ( 2 << ( ord ( $this->BUF [ $i ] { 10 } ) & 0x07 ) ) ); $Locals_ext = "!\xF9\x04" . chr ( ( $this->DIS << 2 ) + 0 ) . chr ( ( $d >> 0 ) & 0xFF ) . chr ( ( $d >> 8 ) & 0xFF ) . "\x0\x0"; if ( $this->COL > -1 && ord ( $this->BUF [ $i ] { 10 } ) & 0x80 ) { for ( $j = 0; $j < ( 2 << ( ord ( $this->BUF [ $i ] { 10 } ) & 0x07 ) ); $j++ ) { if ( ord ( $Locals_rgb { 3 * $j + 0 } ) == ( $this->COL >> 0 ) & 0xFF && ord ( $Locals_rgb { 3 * $j + 1 } ) == ( $this->COL >> 8 ) & 0xFF && ord ( $Locals_rgb { 3 * $j + 2 } ) == ( $this->COL >> 16 ) & 0xFF ) { $Locals_ext = "!\xF9\x04" . chr ( ( $this->DIS << 2 ) + 1 ) . chr ( ( $d >> 0 ) & 0xFF ) . chr ( ( $d >> 8 ) & 0xFF ) . chr ( $j ) . "\x0"; break; } } } switch ( $Locals_tmp { 0 } ) { case "!": $Locals_img = substr ( $Locals_tmp, 8, 10 ); $Locals_tmp = substr ( $Locals_tmp, 18, strlen ( $Locals_tmp ) - 18 ); break; case ",": $Locals_img = substr ( $Locals_tmp, 0, 10 ); $Locals_tmp = substr ( $Locals_tmp, 10, strlen ( $Locals_tmp ) - 10 ); break; } if ( ord ( $this->BUF [ $i ] { 10 } ) & 0x80 && $this->IMG > -1 ) { if ( $Global_len == $Locals_len ) { if ( GIFEncoder::GIFBlockCompare ( $Global_rgb, $Locals_rgb, $Global_len ) ) { $this->GIF .= ( $Locals_ext . $Locals_img . $Locals_tmp ); } else { $byte = ord ( $Locals_img { 9 } ); $byte |= 0x80; $byte &= 0xF8; $byte |= ( ord ( $this->BUF [ 0 ] { 10 } ) & 0x07 ); $Locals_img { 9 } = chr ( $byte ); $this->GIF .= ( $Locals_ext . $Locals_img . $Locals_rgb . $Locals_tmp ); } } else { $byte = ord ( $Locals_img { 9 } ); $byte |= 0x80; $byte &= 0xF8; $byte |= ( ord ( $this->BUF [ $i ] { 10 } ) & 0x07 ); $Locals_img { 9 } = chr ( $byte ); $this->GIF .= ( $Locals_ext . $Locals_img . $Locals_rgb . $Locals_tmp ); } } else { $this->GIF .= ( $Locals_ext . $Locals_img . $Locals_tmp ); } $this->IMG = 1; } /* ::::::::::::::::::::::::::::::::::::::::::::::::::: :: :: GIFAddFooter... :: */ function GIFAddFooter ( ) { $this->GIF .= ";"; } /* ::::::::::::::::::::::::::::::::::::::::::::::::::: :: :: GIFBlockCompare... :: */ function GIFBlockCompare ( $GlobalBlock, $LocalBlock, $Len ) { for ( $i = 0; $i < $Len; $i++ ) { if ( $GlobalBlock { 3 * $i + 0 } != $LocalBlock { 3 * $i + 0 } || $GlobalBlock { 3 * $i + 1 } != $LocalBlock { 3 * $i + 1 } || $GlobalBlock { 3 * $i + 2 } != $LocalBlock { 3 * $i + 2 } ) { return ( 0 ); } } return ( 1 ); } /* ::::::::::::::::::::::::::::::::::::::::::::::::::: :: :: GIFWord... :: */ function GIFWord ( $int ) { return ( chr ( $int & 0xFF ) . chr ( ( $int >> 8 ) & 0xFF ) ); } /* ::::::::::::::::::::::::::::::::::::::::::::::::::: :: :: GetAnimation... :: */ function GetAnimation ( ) { return ( $this->GIF ); } } ?>
为大家分享两个实例供大家参考:
实例 1 合成gif动画:
<?php include "GIFEncoder.class.php"; /* Build a frames array from sources... */ if ( $dh = opendir ( "frames/" ) ) { while ( false !== ( $dat = readdir ( $dh ) ) ) { if ( $dat != "." && $dat != ".." ) { $frames [ ] = "frames/$dat"; $framed [ ] = 5; } } closedir ( $dh ); } /* GIFEncoder constructor: ======================= image_stream = new GIFEncoder ( URL or Binary data 'Sources' int 'Delay times' int 'Animation loops' int 'Disposal' int 'Transparent red, green, blue colors' int 'Source type' ); */ $gif = new GIFEncoder ( $frames, $framed, 0, 2, 0, 0, 0, "url" ); /* Possibles outputs: ================== Output as GIF for browsers : - Header ( 'Content-type:image/gif' ); Output as GIF for browsers with filename: - Header ( 'Content-disposition:Attachment;filename=myanimation.gif'); Output as file to store into a specified file: - FWrite ( FOpen ( "myanimation.gif", "wb" ), $gif->GetAnimation ( ) ); */ Header ( 'Content-type:image/gif' ); echo $gif->GetAnimation ( ); ?>
实例 2 创建gif动画:
<?php include "GIFEncoder.class.php"; ob_start(); $board_width = 60; $board_height = 60; $pad_width = 5; $pad_height = 15; $ball_size = 5; $game_width = $board_width - $pad_width*2 - $ball_size; $game_height = $board_height-$ball_size; $x = 0; $y = rand(0,$game_height); $xv = rand(1,10); $yv = rand(1,10); $pt[] = array($x,$y); do{ $x += $xv; $y += $yv; if($x > $game_width){ $xv = -1*$xv; $x = $game_width - ($x-$game_width); }elseif($x < 0){ $xv = -1*$xv; $x = abs($x); } if($y>$game_height){ $yv = -1*$yv; $y = $game_height - ($y - $game_height); }elseif($y<0){ $yv = -1*$yv; $y = abs($y); } $pt[] = array($x,$y); }while($x!=$pt[0][0]||$y!=$pt[0][1]); $i = 0; while(isset($pt[$i])){ $image = imagecreate($board_width,$board_height); imagecolorallocate($image, 0,0,0); $color = imagecolorallocate($image, 255,255,255); $color2 = imagecolorallocate($image, 255,0,0); if($pt[$i][1] + $pad_height < $board_width){ imagefilledrectangle($image,0,$pt[$i][1],$pad_width, $pt[$i][1]+$pad_height,$color); }else{ imagefilledrectangle($image,0,$board_width-$pad_height,$pad_width, $board_width,$color); } imagefilledrectangle($image,$board_width-$pad_width,0,$board_width, $board_height,$color2); imagefilledrectangle($image,$pad_width+$pt[$i][0], $ball_size+$pt[$i][1]-$ball_size, $pad_width+$pt[$i][0]+$ball_size, $ball_size+$pt[$i][1],$color); //imagesetpixel($image,$pt[$i][0],$pt[$i][1],$color); imagegif($image); imagedestroy($image); $imagedata[] = ob_get_contents(); ob_clean(); ++$i; } $gif = new GIFEncoder( $imagedata, 100, 0, 2, 0, 0, 1, "bin" ); Header ('Content-type:image/gif'); echo $gif->GetAnimation(); ?>
以上就是教大家如何利用php合成或是创建gif动画,希望大家仔细研究分享的两个实例,有所收获。
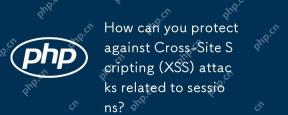
要保护应用免受与会话相关的XSS攻击,需采取以下措施:1.设置HttpOnly和Secure标志保护会话cookie。2.对所有用户输入进行输出编码。3.实施内容安全策略(CSP)限制脚本来源。通过这些策略,可以有效防护会话相关的XSS攻击,确保用户数据安全。
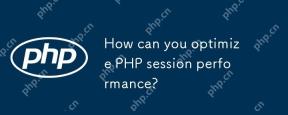
优化PHP会话性能的方法包括:1.延迟会话启动,2.使用数据库存储会话,3.压缩会话数据,4.管理会话生命周期,5.实现会话共享。这些策略能显着提升应用在高并发环境下的效率。
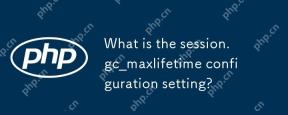
thesession.gc_maxlifetimesettinginphpdeterminesthelifespanofsessiondata,setInSeconds.1)它'sconfiguredinphp.iniorviaini_set().2)abalanceIsiseededeedeedeedeedeedeedto to to avoidperformance andununununununexpectedLogOgouts.3)

在PHP中,可以使用session_name()函数配置会话名称。具体步骤如下:1.使用session_name()函数设置会话名称,例如session_name("my_session")。2.在设置会话名称后,调用session_start()启动会话。配置会话名称可以避免多应用间的会话数据冲突,并增强安全性,但需注意会话名称的唯一性、安全性、长度和设置时机。
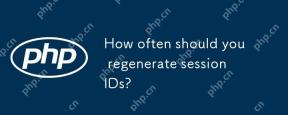
会话ID应在登录时、敏感操作前和每30分钟定期重新生成。1.登录时重新生成会话ID可防会话固定攻击。2.敏感操作前重新生成提高安全性。3.定期重新生成降低长期利用风险,但需权衡用户体验。
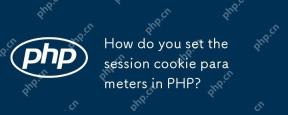
在PHP中设置会话cookie参数可以通过session_set_cookie_params()函数实现。1)使用该函数设置参数,如过期时间、路径、域名、安全标志等;2)调用session_start()使参数生效;3)根据需求动态调整参数,如用户登录状态;4)注意设置secure和httponly标志以提升安全性。
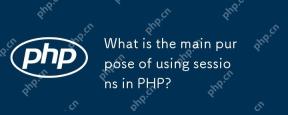
在PHP中使用会话的主要目的是维护用户在不同页面之间的状态。1)会话通过session_start()函数启动,创建唯一会话ID并存储在用户cookie中。2)会话数据保存在服务器上,允许在不同请求间传递数据,如登录状态和购物车内容。
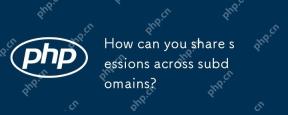
如何在子域名间共享会话?通过设置通用域名的会话cookie实现。1.在服务器端设置会话cookie的域为.example.com。2.选择合适的会话存储方式,如内存、数据库或分布式缓存。3.通过cookie传递会话ID,服务器根据ID检索和更新会话数据。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具
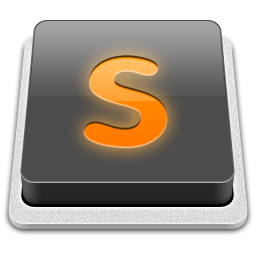
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。

Atom编辑器mac版下载
最流行的的开源编辑器
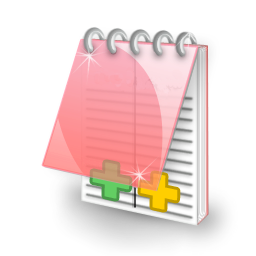
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

SecLists
SecLists是最终安全测试人员的伙伴。它是一个包含各种类型列表的集合,这些列表在安全评估过程中经常使用,都在一个地方。SecLists通过方便地提供安全测试人员可能需要的所有列表,帮助提高安全测试的效率和生产力。列表类型包括用户名、密码、URL、模糊测试有效载荷、敏感数据模式、Web shell等等。测试人员只需将此存储库拉到新的测试机上,他就可以访问到所需的每种类型的列表。