How do I use MongoDB's query language to retrieve data efficiently?
To use MongoDB's query language efficiently for data retrieval, you need to understand and apply the following concepts:
-
Basic Query Syntax: MongoDB uses a JSON-like syntax for querying data. For example, to find documents where the field
name
equals "John", you would use:db.collection.find({ name: "John" })
-
Operators: MongoDB provides a wide range of query operators such as
$eq
,$gt
,$lt
,$in
, and$or
. These allow for more complex and efficient queries. For instance, to find documents where the fieldage
is greater than 18 and less than 30, you could use:db.collection.find({ age: { $gt: 18, $lt: 30 } })
-
Projection: You can use projections to limit the amount of data returned from a query, reducing bandwidth and improving performance. For example, to retrieve only the
name
andemail
fields, you would use:db.collection.find({}, { name: 1, email: 1, _id: 0 })
-
Pagination: Efficiently handling large result sets involves using pagination. You can use
skip()
andlimit()
methods to retrieve results in manageable chunks:db.collection.find().skip(10).limit(10)
- Indexing: While not part of the query syntax itself, indexing is critical for efficient querying. MongoDB can use indexes to speed up queries by avoiding full collection scans. Always ensure that your queries can utilize indexes effectively.
By combining these elements, you can tailor your MongoDB queries to be as efficient as possible for your specific use cases.
What are the best practices for optimizing MongoDB queries to improve retrieval speed?
Optimizing MongoDB queries to enhance retrieval speed involves several best practices:
- Use Appropriate Indexes: Ensure that your queries can use indexes effectively. Indexes can drastically reduce the time required to retrieve data, especially for large collections.
-
Avoid Using
$or
: The$or
operator can be slow because MongoDB may not be able to use indexes efficiently for multiple conditions. Instead, use$in
where possible, or split the query into multiple indexed queries. -
Minimize the Use of
skip()
: Theskip()
method can be slow for large offsets. When paginating through large datasets, consider using range queries or a cursor-based pagination strategy. - Use Covered Queries: A covered query is one where all the fields in the query and the projection are covered by an index. This can significantly improve performance as MongoDB does not need to scan the document collection.
-
Limit and Sort Appropriately: Use
limit()
to constrain the number of documents returned andsort()
in conjunction with indexes to efficiently sort the results. - Regularly Analyze and Optimize: Use MongoDB’s profiling and explain tools to analyze queries and make necessary optimizations.
- Denormalization: In some cases, denormalizing your data can improve query performance by reducing the need for complex joins and lookups.
By implementing these best practices, you can significantly improve the speed and efficiency of your MongoDB queries.
How can I use indexes effectively in MongoDB to enhance query performance?
Using indexes effectively in MongoDB is key to enhancing query performance. Here are some strategies:
-
Create Indexes on Frequently Queried Fields: If you often query by certain fields, create indexes on these fields. For example, if you frequently search by
username
, you should create an index on theusername
field:db.collection.createIndex({ username: 1 })
-
Compound Indexes: Use compound indexes when your queries involve multiple fields. For example, if you commonly query by both
lastName
andfirstName
, a compound index would be beneficial:db.collection.createIndex({ lastName: 1, firstName: 1 })
-
Indexing for Sorting and Ranging: If you sort or use range queries on certain fields, index them to improve performance. For example, if you sort by
createdAt
, index this field:db.collection.createIndex({ createdAt: 1 })
- Sparse Indexes: Use sparse indexes for fields that are not present in every document. This can save space and improve performance for queries that filter on these fields.
-
Text Indexes: For full-text search capabilities, create text indexes on fields that contain text data:
db.collection.createIndex({ description: "text" })
-
Monitor and Adjust Indexes: Regularly use the
explain()
method to see how queries are using indexes and adjust them based on performance metrics. For instance:db.collection.find({ username: "john" }).explain()
By strategically planning and maintaining your indexes, you can greatly enhance the performance of your MongoDB queries.
What tools or methods can I use to analyze and troubleshoot slow MongoDB queries?
To analyze and troubleshoot slow MongoDB queries, you can utilize the following tools and methods:
-
MongoDB Profiler: MongoDB’s built-in profiler can log slow queries, which helps identify performance bottlenecks. You can enable the profiler to capture queries that exceed a certain execution time threshold:
db.setProfilingLevel(2, { slowms: 100 })
-
Explain() Method: The
explain()
method provides detailed information about the query execution plan, including index usage and execution time. Use it to analyze how your queries are being processed:db.collection.find({ field: "value" }).explain()
- MongoDB Compass: This GUI tool offers visual query performance analysis, showing execution statistics and index usage, which can be particularly helpful for developers who prefer a graphical interface.
- MongoDB Atlas Performance Advisor: If you're using MongoDB Atlas, the Performance Advisor can automatically analyze your queries and provide recommendations for index creation and optimization.
- Database Profiler and Logs: Regularly review the MongoDB server logs to identify and troubleshoot slow operations. You can configure MongoDB to log queries that exceed certain time thresholds.
- Third-Party Monitoring Tools: Tools like Datadog, New Relic, and Prometheus can monitor MongoDB performance and help identify slow queries in real-time.
-
Query Plan Cache: MongoDB caches query plans, which can help optimize repeated queries. Use the
planCacheListPlans
command to review cached plans:db.collection.getPlanCache().listPlans()
By leveraging these tools and methods, you can effectively analyze and troubleshoot slow MongoDB queries, ensuring optimal database performance.
以上是如何使用MongoDB的查询语言有效地检索数据?的详细内容。更多信息请关注PHP中文网其他相关文章!
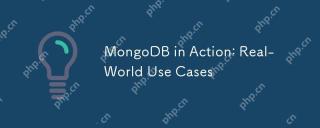
MongoDB在实际项目中的用法包括:1)文档存储,2)复杂的聚合操作,3)性能优化和最佳实践。具体来说,MongoDB的文档模型支持灵活的数据结构,适合处理用户生成内容;聚合框架可用于分析用户行为;性能优化可以通过索引优化、分片和缓存实现,最佳实践包括文档设计、数据迁移和监控维护。
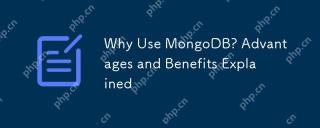
MongoDB是一个开源的NoSQL数据库,采用文档模型存储数据。其优势包括:1.灵活的数据模型,支持JSON格式存储,适用于快速迭代开发;2.横向扩展和高可用性,通过分片实现负载均衡;3.丰富的查询语言,支持复杂查询和聚合操作;4.性能和优化,通过索引和内存映射文件系统提升数据访问速度;5.生态系统和社区支持,提供多种驱动程序和活跃的社区帮助。
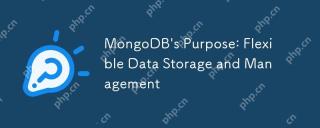
MongoDB的灵活性体现在:1)能存储任意结构的数据,2)使用BSON格式,3)支持复杂查询和聚合操作。这种灵活性使其在处理多变数据结构时表现出色,是现代应用开发的强大工具。
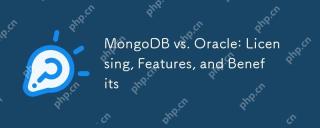
MongoDB适合处理大规模非结构化数据,采用开源许可证;Oracle适合复杂商业事务,采用商业许可证。1.MongoDB提供灵活的文档模型和横向扩展能力,适合大数据处理。2.Oracle提供强大的ACID事务支持和企业级功能,适合复杂分析工作负载。选择时需考虑数据类型、预算和技术资源。
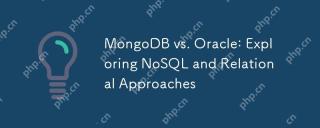
在不同的应用场景下,选择MongoDB还是Oracle取决于具体需求:1)如果需要处理大量非结构化数据且对数据一致性要求不高,选择MongoDB;2)如果需要严格的数据一致性和复杂查询,选择Oracle。
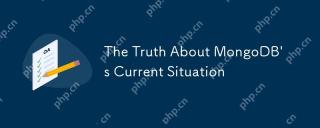
MongoDB当前的表现取决于具体的使用场景和需求。1)在电商平台中,MongoDB适合存储商品信息和用户数据,但处理订单时可能面临一致性问题。2)在内容管理系统中,MongoDB便于存储文章和评论,但处理大量数据时需使用分片技术。
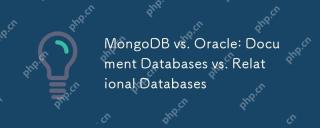
引言在现代数据管理的世界里,选择合适的数据库系统对于任何项目来说都是至关重要的。我们常常会面临一个选择:是选择MongoDB这种文档型数据库,还是选择Oracle这种关系型数据库?今天我将带你深入探讨MongoDB和Oracle之间的差异,帮助你理解它们的优劣势,并分享我在实际项目中使用它们的经验。本文将会带你从基础知识开始,逐步深入到这两类数据库的核心特性、使用场景和性能表现。无论你是刚入门的数据管理者,还是有经验的数据库管理员,读完这篇文章,你将对如何在项目中选择和使用MongoDB或Ora
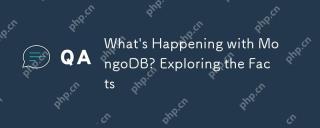
MongoDB仍然是一个强大的数据库解决方案。 1)它以灵活性和可扩展性着称,适合存储复杂数据结构。 2)通过合理索引和查询优化,可以提升其性能。 3)使用聚合框架和分片技术,可以进一步优化和扩展MongoDB的应用。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

SecLists
SecLists是最终安全测试人员的伙伴。它是一个包含各种类型列表的集合,这些列表在安全评估过程中经常使用,都在一个地方。SecLists通过方便地提供安全测试人员可能需要的所有列表,帮助提高安全测试的效率和生产力。列表类型包括用户名、密码、URL、模糊测试有效载荷、敏感数据模式、Web shell等等。测试人员只需将此存储库拉到新的测试机上,他就可以访问到所需的每种类型的列表。
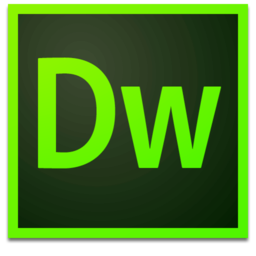
Dreamweaver Mac版
视觉化网页开发工具

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。

SublimeText3 英文版
推荐:为Win版本,支持代码提示!
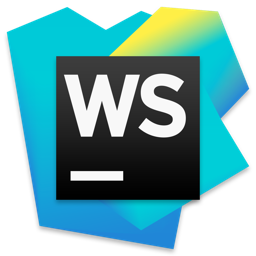
WebStorm Mac版
好用的JavaScript开发工具