如何使用Java的加密API进行加密和解密?
Java在java.security
软件包及其子包中提供了一组强大的加密API。这些API允许开发人员执行各种加密操作,包括加密和解密。涉及的核心类是Cipher
, SecretKey
, SecretKeyFactory
和KeyGenerator
。这是如何使用它们进行对称加密的细分(使用AES):
1。密钥一代:
首先,您需要生成一个秘密密钥。该密钥对于加密和解密至关重要。以下代码片段演示了如何生成256位AES密钥:
<code class="java">import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; import javax.crypto.spec.SecretKeySpec; import java.security.NoSuchAlgorithmException; import java.security.SecureRandom; import java.util.Base64; public class AESEncryption { public static void main(String[] args) throws NoSuchAlgorithmException { // Generate a 256-bit AES key KeyGenerator keyGenerator = KeyGenerator.getInstance("AES"); keyGenerator.init(256, new SecureRandom()); SecretKey secretKey = keyGenerator.generateKey(); // ... (rest of the code for encryption and decryption) ... } }</code>
2。加密:
拥有密钥后,您可以使用Cipher
类来加密数据。以下代码显示了如何使用pkcs5padding的CBC模式使用AES加密字符串:
<code class="java">import javax.crypto.Cipher; import javax.crypto.NoSuchPaddingException; import javax.crypto.SecretKey; import javax.crypto.spec.IvParameterSpec; import java.security.InvalidAlgorithmParameterException; import java.security.InvalidKeyException; import java.security.NoSuchAlgorithmException; import java.util.Base64; import java.util.Arrays; // ... (previous code for key generation) ... byte[] iv = new byte[16]; // Initialization Vector (IV) - must be randomly generated new SecureRandom().nextBytes(iv); IvParameterSpec ivParameterSpec = new IvParameterSpec(iv); Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding"); cipher.init(Cipher.ENCRYPT_MODE, secretKey, ivParameterSpec); byte[] encryptedBytes = cipher.doFinal("This is my secret message".getBytes()); String encryptedString = Base64.getEncoder().encodeToString(iv) Base64.getEncoder().encodeToString(encryptedBytes); //Combine IV and encrypted data for later decryption System.out.println("Encrypted: " encryptedString); } }</code>
3。解密:
解密类似于加密,但是您使用Cipher.DECRYPT_MODE
。请记住使用相同的密钥,IV和算法参数:
<code class="java">// ... (previous code for key generation and encryption) ... String[] parts = encryptedString.split("\\s "); // Split the string into IV and encrypted data byte[] decodedIv = Base64.getDecoder().decode(parts[0]); byte[] decodedEncryptedBytes = Base64.getDecoder().decode(parts[1]); IvParameterSpec ivParameterSpecDec = new IvParameterSpec(decodedIv); Cipher decipher = Cipher.getInstance("AES/CBC/PKCS5Padding"); decipher.init(Cipher.DECRYPT_MODE, secretKey, ivParameterSpecDec); byte[] decryptedBytes = decipher.doFinal(decodedEncryptedBytes); System.out.println("Decrypted: " new String(decryptedBytes)); } }</code>
请记住在生产环境中适当处理异常。此示例提供了一个基本的例证。对于更复杂的方案,请考虑使用密钥库和其他安全性最佳实践。
使用Java密码学时,安全密钥管理的最佳实践是什么?
安全密钥管理在密码学中至关重要。损坏的钥匙使您的加密无用。以下是一些最佳实践:
-
使用强大的密钥生成:采用算法,例如具有足够长度的AE(至少256位)。使用诸如
SecureRandom
类的密码固定的随机数生成器(CSPRNG)。 - 钥匙存储:永远不要直接进入应用程序中的硬码密钥。使用Java加密体系结构(JCA)或专用硬件安全模块(HSM)提供的安全密钥库。密钥库提供了密码保护和密钥管理的机制。
- 钥匙旋转:定期旋转密钥以限制潜在折衷的影响。实施计划的密钥旋转过程。
- 访问控制:限制基于最低特权原则对键的访问。只有授权人员或系统才能访问钥匙。
- 钥匙破坏:当不再需要钥匙时,会安全地销毁它。多次覆盖关键数据是一种常见方法,但是HSM提供了更强大的关键破坏机制。
- 避免重复使用:切勿用于多种目的或跨不同应用程序重复使用相同的密钥。
- 使用密钥管理系统(KMS):对于企业级应用程序,请考虑使用专用的KMS,其提供高级功能,例如关键生命周期管理,审核以及与其他安全系统集成。
哪种Java加密算法最适合不同的安全需求?
算法的选择取决于您的特定安全需求和约束。这是一个简短的概述:
-
对称加密(用于机密性):
- AE(高级加密标准):广泛认为是大多数应用程序的最安全,最有效的对称算法。使用256位密钥以获得最大的安全性。
- CHACHA20:现代的溪流密码提供强大的安全性和性能,尤其是在资源有限的系统上。
-
非对称加密(用于机密性和数字签名):
- RSA:一种用于数字签名和密钥交换的广泛使用算法。但是,它在计算上比对称算法昂贵。使用至少2048位的关键尺寸。
- ECC(椭圆曲线密码学):提供具有较小钥匙尺寸的RSA的可比安全性,使其在资源约束环境中更有效。
-
哈希(用于完整性和身份验证):
- SHA-256/SHA-512:提供碰撞阻力的安全哈希算法。 SHA-512提供的安全性略高,但计算上更昂贵。
- HMAC(基于哈希的消息身份验证代码):提供消息身份验证和完整性。结合使用SHA-256或SHA-512等强大的哈希功能。
-
数字签名(用于身份验证和非纠正):
- RSA和ECDA(椭圆曲线数字签名算法):两者都广泛用于创建数字签名。 ECDSA通常比RSA更有效。
请记住,始终使用系统可以有效处理并与最新的安全咨询有关的最强算法。
在Java中实施加密和解密时,是否有任何共同的陷阱可以避免?
几个常见的陷阱可以削弱您的加密实施的安全性:
- 不正确的IV处理:使用非随机或重复使用的IV与CBC模式中AES(例如AES)这样的块iv大大降低了安全性。始终为每个加密操作生成一个密码固定的随机iv。
- 弱或硬编码键:无需直接在代码中的硬码键。使用安全的密钥库,并遵循密钥管理最佳实践。
- 填充不当:使用错误或不安全的填充方案可能会导致诸如填充甲骨文攻击之类的漏洞。使用公认的填充方案,例如PKCS5PADDING或PKCS7PADDING。
- 算法滥用:选择不适当的算法或错误地使用它会严重损害安全性。仔细考虑应用程序的安全要求,然后选择适当的算法和操作方式。
- 密钥长度不足:使用太短的密钥长度使您的加密很容易受到蛮力攻击。始终为选定的算法使用推荐的密钥长度。
- 忽略异常处理:正确处理异常对于安全和稳健的加密至关重要。无法处理异常会导致漏洞或数据丢失。
- 数据不当:在加密之前未能对数据进行消毒,可能会导致注射攻击。加密前适当地对数据进行了适当的消毒。
-
不安全的随机数生成:使用弱随机数生成器可以削弱密钥和IV的安全性。始终使用csprng之类的
SecureRandom
。
通过仔细考虑这些陷阱并遵循最佳实践,您可以显着提高Java加密实现的安全性。请记住,安全性是一个持续的过程,并且对最新的安全咨询和最佳实践进行更新至关重要。
以上是如何使用Java的加密API进行加密和解密?的详细内容。更多信息请关注PHP中文网其他相关文章!
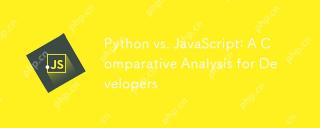
Python和JavaScript的主要区别在于类型系统和应用场景。1.Python使用动态类型,适合科学计算和数据分析。2.JavaScript采用弱类型,广泛用于前端和全栈开发。两者在异步编程和性能优化上各有优势,选择时应根据项目需求决定。
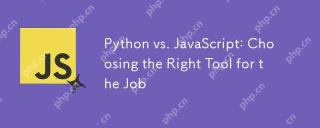
选择Python还是JavaScript取决于项目类型:1)数据科学和自动化任务选择Python;2)前端和全栈开发选择JavaScript。Python因其在数据处理和自动化方面的强大库而备受青睐,而JavaScript则因其在网页交互和全栈开发中的优势而不可或缺。
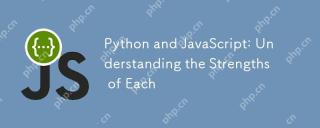
Python和JavaScript各有优势,选择取决于项目需求和个人偏好。1.Python易学,语法简洁,适用于数据科学和后端开发,但执行速度较慢。2.JavaScript在前端开发中无处不在,异步编程能力强,Node.js使其适用于全栈开发,但语法可能复杂且易出错。
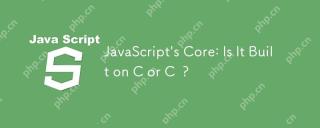
javascriptisnotbuiltoncorc; saninterpretedlanguagethatrunsonenginesoftenwritteninc.1)javascriptwasdesignedAsalightweight,解释edganguageforwebbrowsers.2)Enginesevolvedfromsimpleterterterpretpreterterterpretertestojitcompilerers,典型地提示。
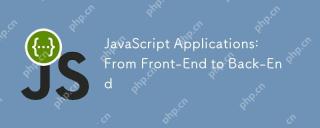
JavaScript可用于前端和后端开发。前端通过DOM操作增强用户体验,后端通过Node.js处理服务器任务。1.前端示例:改变网页文本内容。2.后端示例:创建Node.js服务器。
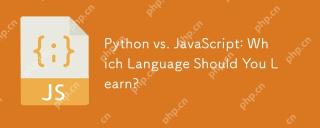
选择Python还是JavaScript应基于职业发展、学习曲线和生态系统:1)职业发展:Python适合数据科学和后端开发,JavaScript适合前端和全栈开发。2)学习曲线:Python语法简洁,适合初学者;JavaScript语法灵活。3)生态系统:Python有丰富的科学计算库,JavaScript有强大的前端框架。
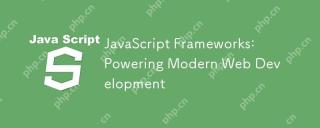
JavaScript框架的强大之处在于简化开发、提升用户体验和应用性能。选择框架时应考虑:1.项目规模和复杂度,2.团队经验,3.生态系统和社区支持。
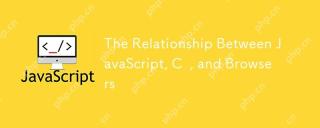
引言我知道你可能会觉得奇怪,JavaScript、C 和浏览器之间到底有什么关系?它们之间看似毫无关联,但实际上,它们在现代网络开发中扮演着非常重要的角色。今天我们就来深入探讨一下这三者之间的紧密联系。通过这篇文章,你将了解到JavaScript如何在浏览器中运行,C 在浏览器引擎中的作用,以及它们如何共同推动网页的渲染和交互。JavaScript与浏览器的关系我们都知道,JavaScript是前端开发的核心语言,它直接在浏览器中运行,让网页变得生动有趣。你是否曾经想过,为什么JavaScr


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

SublimeText3汉化版
中文版,非常好用
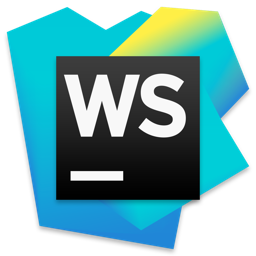
WebStorm Mac版
好用的JavaScript开发工具
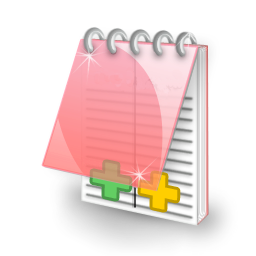
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

DVWA
Damn Vulnerable Web App (DVWA) 是一个PHP/MySQL的Web应用程序,非常容易受到攻击。它的主要目标是成为安全专业人员在合法环境中测试自己的技能和工具的辅助工具,帮助Web开发人员更好地理解保护Web应用程序的过程,并帮助教师/学生在课堂环境中教授/学习Web应用程序安全。DVWA的目标是通过简单直接的界面练习一些最常见的Web漏洞,难度各不相同。请注意,该软件中

禅工作室 13.0.1
功能强大的PHP集成开发环境