>如何在PHP 7中使用多态性? 这主要是通过接口和抽象类来实现的。
使用接口:一个接口定义了类必须遵守的合同。 它指定方法签名而不提供实现。 然后,类
实现接口,为方法提供了自己的具体实现。
// Define an interface interface Shape { public function getArea(); } // Implement the interface in different classes class Circle implements Shape { private $radius; public function __construct($radius) { $this->radius = $radius; } public function getArea() { return pi() * $this->radius * $this->radius; } } class Square implements Shape { private $side; public function __construct($side) { $this->side = $side; } public function getArea() { return $this->side * $this->side; } } // Using polymorphism $shapes = [new Circle(5), new Square(4)]; foreach ($shapes as $shape) { echo "Area: " . $shape->getArea() . PHP_EOL; }在此示例中,
>和Circle
被视为Square
>对象。 Shape
循环通过包含两种类型的数组迭代,每个类型都在每种类型上调用foreach
getArea()
摘要类与接口相似,但可以为某些方法提供默认实现。 它们不能直接实例化;子类必须扩展它们并为任何抽象方法提供实现。
// Define an abstract class abstract class Animal { public function speak() { echo "Generic animal sound" . PHP_EOL; } abstract public function move(); } // Extend the abstract class class Dog extends Animal { public function move() { echo "Dog is running" . PHP_EOL; } } class Bird extends Animal { public function move() { echo "Bird is flying" . PHP_EOL; } } // Using polymorphism $animals = [new Dog(), new Bird()]; foreach ($animals as $animal) { $animal->speak(); $animal->move(); }和
> Dog
从Bird
继承,并提供其对Animal
方法的具体实现。 该方法在抽象类中具有默认实现,但是如果需要的话,子类可以覆盖它。move()
>speak()
>在PHP 7应用中使用多态性的应用有什么实际好处?
- 在PHP 7中使用多态性的实际好处,没有PHP 7的范围,而无需添加php 7>
- 代码可重用性:
- 改进的设计: 多态性会导致更模块化和结构良好的设计。 它鼓励关注点的分离并促进更清洁的体系结构。
- 可检验性: 多态性使单位测试更加容易。您可以轻松模拟或存根界面或抽象类,简化测试过程。
- 可维护性: 更改对一个类的更改不太可能影响应用程序的其他部分。 这降低了引入错误并使维护更加容易的风险。
>
>多态性如何改善PHP 7项目中的代码可维护性和可扩展性?- >松散的耦合:
多态性降低了类之间的依赖性。 代码没有直接与特定类交互,而是与接口或抽象类交互。 这意味着一个类中的变化不太可能在系统的其余部分中旋转。 open/封闭的原理: - 可以在不修改使用接口或抽象类的现有代码的情况下添加新类。 这遵守实体设计原理的开放/封闭原理。 >更轻松的重构:
- 由于松散的耦合,因此简化了重构。 只要维护接口或抽象类合同,您就可以修改或替换实现,而无需影响系统的其他部分。 >简化的调试:
- ,由于模块化,指出错误的源源变得更加容易。 变化的影响是本地化的,更易于跟踪。 >您能否提供PHP 7中的多态性示例,这些示例在不同的方案中证明了它的使用?
saceario 1:database互动:
>Database
connect()
query()
disconnect()
MySQLDatabase
PostgreSQLDatabase
Database
,和
。 您的应用程序代码可以使用PaymentGateway
>接口与数据库进行交互,而不管实际使用的数据库系统如何。 切换数据库仅需要更改混凝土类的实例。processPayment()
StripePaymentGateway
PayPalPaymentGateway
PaymentGateway
方案2:付款处理:
>您可能有不同的付款网关(Stripe,PayPal)。使用
之类的方法创建一个接口。 例如Logger
和log()
之类的实现将处理每个网关的细节。 您的购物车应用程序可以使用FileLogger
>接口,使您可以轻松地添加新的付款选项而不更改核心功能。 混凝土类,例如DatabaseLogger
>,EmailLogger
,Logger
将处理特定的日志记录方法。您的应用程序可以使用
>
>这些示例表明了多态性如何通过将应用程序逻辑从特定实现中解除应用程序来促进灵活性,可维护性和可扩展性。 这会导致更清洁,更健壮且易于维护的PHP 7应用程序。以上是如何在PHP 7中使用多态性?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。
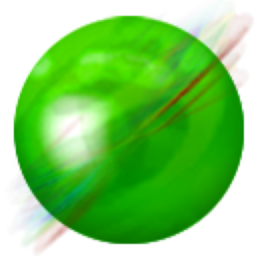
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境
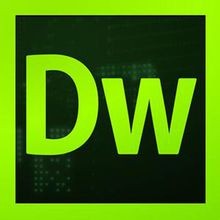
Dreamweaver CS6
视觉化网页开发工具

SublimeText3 英文版
推荐:为Win版本,支持代码提示!

SublimeText3 Linux新版
SublimeText3 Linux最新版