使用openai而无需函数
>在本节中,我们将使用GPT-3.5-Turbo模型生成响应,而无需呼叫,以查看我们是否获得一致的输出。
>在安装OpenAI Python API之前,您必须获得一个API键并将其设置在本地系统上。通过Python教程中的OpenAI API遵循GPT-3.5和GPT-4,以了解如何获取API键并进行设置。该教程还包括在DataCamp的DataCamp的AI启用数据笔记本中设置环境变量的示例。以获取进一步的帮助,请查看Datalab上的OpenAI函数拨打工作簿中的代码。
>使用以下方式将OpenAi Python API升级到V1
之后,使用API键启动OpenAI客户端。
pip install --upgrade openai -q>
>
import os from openai import OpenAI client = OpenAI( api_key=os.environ['OPENAI_API_KEY'], )注:OpenAI不再向新用户提供免费的积分,因此您必须购买它们才能使用API。
我们将编写一个随机的学生描述。您可以提出自己的文字,或者使用chatgpt为您生成一个。>
>在下一部分中,我们将编写一个提示,以从文本中提取学生信息并将输出返回为JSON对象。我们将在学生描述中提取名称,专业,学校,成绩和俱乐部。
>student_1_description = "David Nguyen is a sophomore majoring in computer science at Stanford University. He is Asian American and has a 3.8 GPA. David is known for his programming skills and is an active member of the university's Robotics Club. He hopes to pursue a career in artificial intelligence after graduating."
>将提示添加到OpenAI API聊天完成模块中以生成响应。
# A simple prompt to extract information from "student_description" in a JSON format. prompt1 = f''' Please extract the following information from the given text and return it as a JSON object: name major school grades club This is the body of text to extract the information from: {student_1_description} '''响应非常好。让我们将其转换为JSON,以更好地理解它。
>
# Generating response back from gpt-3.5-turbo openai_response = client.chat.completions.create( model = 'gpt-3.5-turbo', messages = [{'role': 'user', 'content': prompt_1}] ) openai_response.choices[0].message.content我们将使用“ JSON”库将文本转换为JSON对象。
最终结果非常完美。那么,为什么我们需要调用函数?
'{\n "name": "David Nguyen",\n "major": "computer science",\n "school": "Stanford University",\n "grades": "3.8 GPA",\n "club": "Robotics Club"\n}'>
>让我们尝试相同的提示,但使用其他学生描述。
import json # Loading the response as a JSON object json_response = json.loads(openai_response.choices[0].message.content) json_response
>我们将在提示中更改学生描述文本。
{'name': 'David Nguyen', 'major': 'computer science', 'school': 'Stanford University', 'grades': '3.8 GPA', 'club': 'Robotics Club'}
,并使用第二个提示来运行聊天完成功能。
student_2_description="Ravi Patel is a sophomore majoring in computer science at the University of Michigan. He is South Asian Indian American and has a 3.7 GPA. Ravi is an active member of the university's Chess Club and the South Asian Student Association. He hopes to pursue a career in software engineering after graduating."如您所见,这是不一致的。它没有返回一个俱乐部,而是返回了拉维(Ravi)加入的俱乐部名单。这也与第一个学生不同。
>
prompt2 = f''' Please extract the following information from the given text and return it as a JSON object: name major school grades club This is the body of text to extract the information from: {student_2_description} '''openai函数调用示例
为了解决此问题,我们现在将使用最近引入的功能呼叫的功能。创建一个自定义功能以在字典列表中添加必要的信息是至关重要的,以便OpenAI API了解其功能。
- >名称:写您最近创建的python函数名称。
- 描述:函数的功能。 >
- 参数:在“属性”中,我们将写入参数,类型和描述的名称。它将帮助Openai API确定我们正在寻找的世界。
note:确保您遵循正确的模式。通过阅读官方文档来了解有关函数调用的更多信息。>
接下来,我们将使用添加到“函数”参数中的自定义函数为两个学生描述生成响应。之后,我们将将文本响应转换为JSON对象并打印它。pip install --upgrade openai -q>
如我们所见,我们获得了统一的输出。我们甚至在数字而不是字符串中获得成绩。一致的输出对于创建没有错误的AI应用程序至关重要。
>import os from openai import OpenAI client = OpenAI( api_key=os.environ['OPENAI_API_KEY'], )
多个自定义功能
student_1_description = "David Nguyen is a sophomore majoring in computer science at Stanford University. He is Asian American and has a 3.8 GPA. David is known for his programming skills and is an active member of the university's Robotics Club. He hopes to pursue a career in artificial intelligence after graduating.">您可以在聊天完成功能中添加多个自定义功能。在本节中,我们将看到OpenAI API的神奇功能,以及它如何自动选择正确的函数并返回正确的参数。
。 在字典的python列表中,我们将添加另一个称为“ extract_school_info”的功能,该功能将帮助我们从文本中提取大学信息。 为了实现这一目标,您必须添加另一个具有名称,描述和参数的函数的字典。
>我们将使用Chatgpt生成“ Stanford University”描述来测试我们的功能。
>创建学生和学校描述列表,并通过OpenAI聊天完成功能将其传递以生成响应。确保您提供了更新的自定义功能。
># A simple prompt to extract information from "student_description" in a JSON format. prompt1 = f''' Please extract the following information from the given text and return it as a JSON object: name major school grades club This is the body of text to extract the information from: {student_1_description} '''
> GPT-3.5-Turbo模型已自动为不同的描述类型选择了正确的功能。我们为学生和学校提供了完美的JSON输出。
># Generating response back from gpt-3.5-turbo openai_response = client.chat.completions.create( model = 'gpt-3.5-turbo', messages = [{'role': 'user', 'content': prompt_1}] ) openai_response.choices[0].message.content
我们甚至可以使用“ extract_school_info”函数生成休息的名称。
'{\n "name": "David Nguyen",\n "major": "computer science",\n "school": "Stanford University",\n "grades": "3.8 GPA",\n "club": "Robotics Club"\n}'
import json # Loading the response as a JSON object json_response = json.loads(openai_response.choices[0].message.content) json_response
>函数调用的应用
在本节中,我们将构建一个稳定的文本摘要,该摘要将以某种方式汇总学校和学生信息。
首先,我们将创建两个python函数,即extract_student_info和extract_school_info,从函数调用中获取参数并返回汇总的字符串。
pip install --upgrade openai -q
- 创建Python列表,该列表由学生描述,随机提示和学校描述组成。添加随机提示以验证自动函数调用机械师。
- 我们将使用“描述”列表中的每个文本生成响应。 >
- 如果使用了函数调用,我们将获得函数的名称,并基于它,将相关参数应用于函数。否则,返回正常响应。 >
- 打印所有三个样本的输出。
import os from openai import OpenAI client = OpenAI( api_key=os.environ['OPENAI_API_KEY'], )
- 示例#1 :GPT模型选择了“ extract_student_info”,我们得到了有关学生的简短摘要。 >
- >样本#2 :GPT模型尚未选择任何功能,并将提示视为常规问题,结果,我们得到了亚伯拉罕·林肯的传记。
- >样本#3 :GPT模型选择了“ extract_school_info”,我们得到了有关斯坦福大学的简短摘要。
student_1_description = "David Nguyen is a sophomore majoring in computer science at Stanford University. He is Asian American and has a 3.8 GPA. David is known for his programming skills and is an active member of the university's Robotics Club. He hopes to pursue a career in artificial intelligence after graduating."在本教程中,我们了解了Openai的功能调用。我们还学习了如何使用它来生成一致的输出,创建多个功能并构建可靠的文本摘要。
>如果您想了解有关OpenAi API的更多信息,请考虑使用OpenAI API课程,并在Python备忘单中使用OpenAi API来创建您的第一个AI驱动项目。
>>
是否可以与外部API或数据库一起使用OpenAI函数来使用?
>
>>如果模型的功能调用与任何定义的函数不匹配,会发生什么?
>
如果模型的函数调用与已定义的函数或所提供的架构不匹配,则该函数调用未触发,并且该模型将输入视为基于标准文本的提示,返回基于文本的基于典型的基于文本的响应。这确保了处理各种输入类型的灵活性。
赚取顶级AI认证证明您可以有效,负责任地使用AI。获得认证,被录用以上是OpenAI函数调用教程:生成结构化输出的详细内容。更多信息请关注PHP中文网其他相关文章!
![无法使用chatgpt!解释可以立即测试的原因和解决方案[最新2025]](https://img.php.cn/upload/article/001/242/473/174717025174979.jpg?x-oss-process=image/resize,p_40)
ChatGPT无法访问?本文提供多种实用解决方案!许多用户在日常使用ChatGPT时,可能会遇到无法访问或响应缓慢等问题。本文将根据不同情况,逐步指导您解决这些问题。 ChatGPT无法访问的原因及初步排查 首先,我们需要确定问题是出在OpenAI服务器端,还是用户自身网络或设备问题。 请按照以下步骤进行排查: 步骤1:检查OpenAI官方状态 访问OpenAI Status页面 (status.openai.com),查看ChatGPT服务是否正常运行。如果显示红色或黄色警报,则表示Open
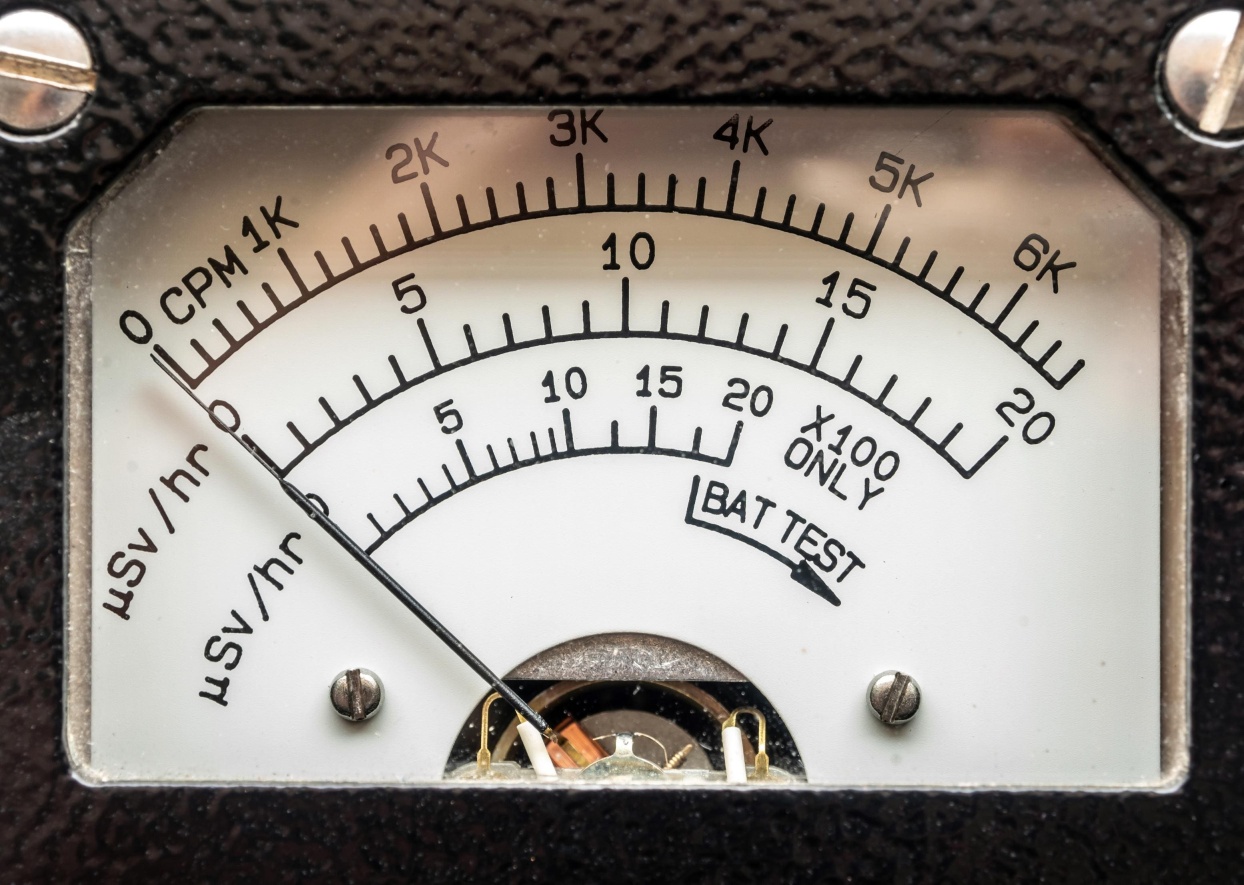
2025年5月10日,麻省理工学院物理学家Max Tegmark告诉《卫报》,AI实验室应在释放人工超级智能之前模仿Oppenheimer的三位一体测试演算。 “我的评估是'康普顿常数',这是一场比赛的可能性

AI音乐创作技术日新月异,本文将以ChatGPT等AI模型为例,详细讲解如何利用AI辅助音乐创作,并辅以实际案例进行说明。我们将分别介绍如何通过SunoAI、Hugging Face上的AI jukebox以及Python的Music21库进行音乐创作。 通过这些技术,每个人都能轻松创作原创音乐。但需注意,AI生成内容的版权问题不容忽视,使用时务必谨慎。 让我们一起探索AI在音乐领域的无限可能! OpenAI最新AI代理“OpenAI Deep Research”介绍: [ChatGPT]Ope

ChatGPT-4的出现,极大地拓展了AI应用的可能性。相较于GPT-3.5,ChatGPT-4有了显着提升,它具备强大的语境理解能力,还能识别和生成图像,堪称万能的AI助手。在提高商业效率、辅助创作等诸多领域,它都展现出巨大的潜力。然而,与此同时,我们也必须注意其使用上的注意事项。 本文将详细解读ChatGPT-4的特性,并介绍针对不同场景的有效使用方法。文中包含充分利用最新AI技术的技巧,敬请参考。 OpenAI发布的最新AI代理,“OpenAI Deep Research”详情请点击下方链
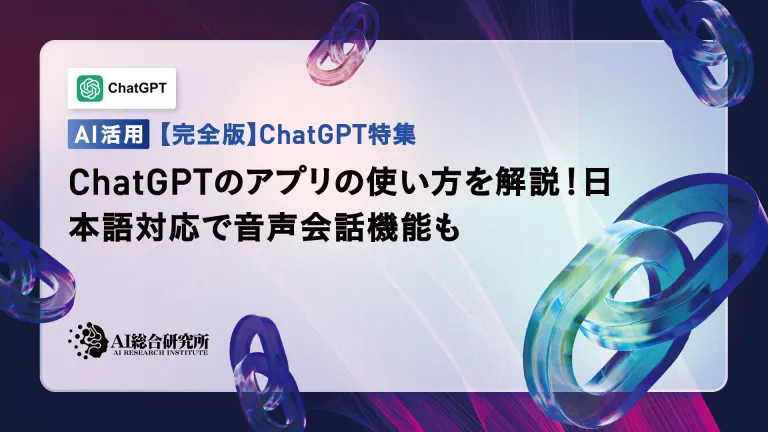
CHATGPT应用程序:与AI助手释放您的创造力!初学者指南 ChatGpt应用程序是一位创新的AI助手,可处理各种任务,包括写作,翻译和答案。它是一种具有无限可能性的工具,可用于创意活动和信息收集。 在本文中,我们将以一种易于理解的方式解释初学者,从如何安装chatgpt智能手机应用程序到语音输入功能和插件等应用程序所独有的功能,以及在使用该应用时要牢记的要点。我们还将仔细研究插件限制和设备对设备配置同步
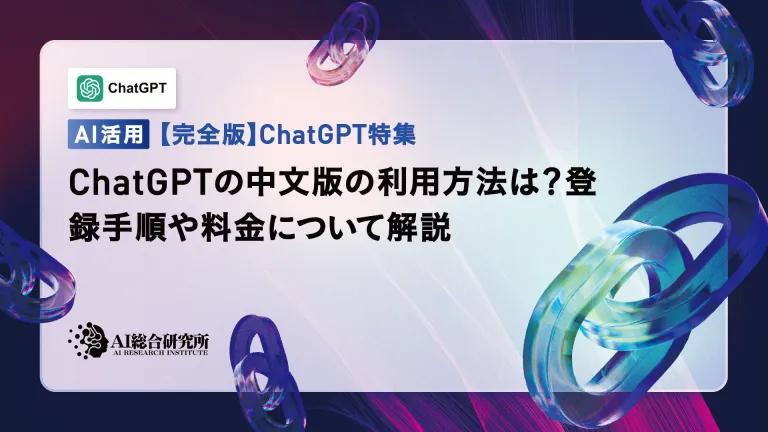
ChatGPT中文版:解锁中文AI对话新体验 ChatGPT风靡全球,您知道它也提供中文版本吗?这款强大的AI工具不仅支持日常对话,还能处理专业内容,并兼容简体中文和繁体中文。无论是中国地区的使用者,还是正在学习中文的朋友,都能从中受益。 本文将详细介绍ChatGPT中文版的使用方法,包括账户设置、中文提示词输入、过滤器的使用、以及不同套餐的选择,并分析潜在风险及应对策略。此外,我们还将对比ChatGPT中文版和其他中文AI工具,帮助您更好地了解其优势和应用场景。 OpenAI最新发布的AI智能
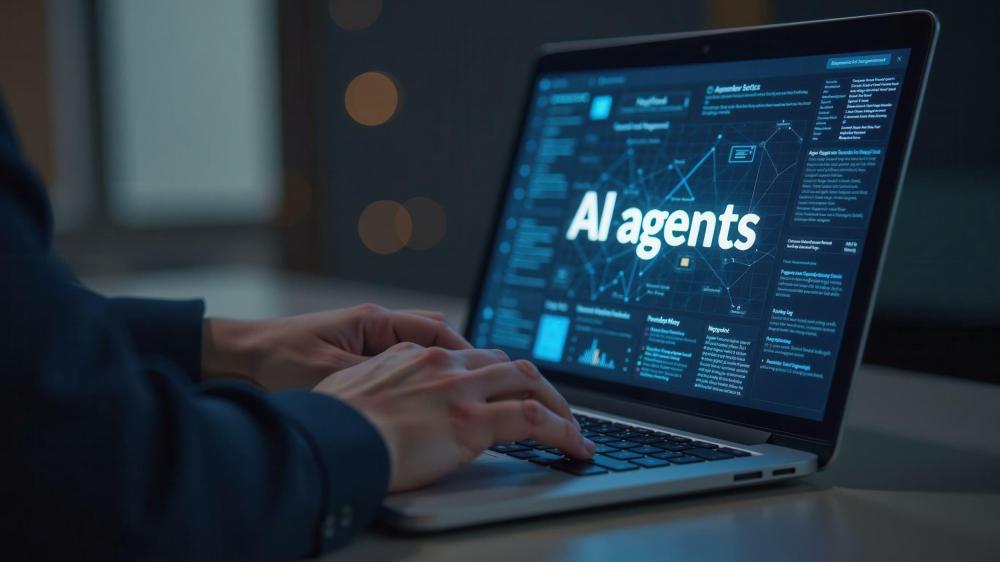
这些可以将其视为生成AI领域的下一个飞跃,这为我们提供了Chatgpt和其他大型语言模型聊天机器人。他们可以代表我们采取行动,而不是简单地回答问题或产生信息
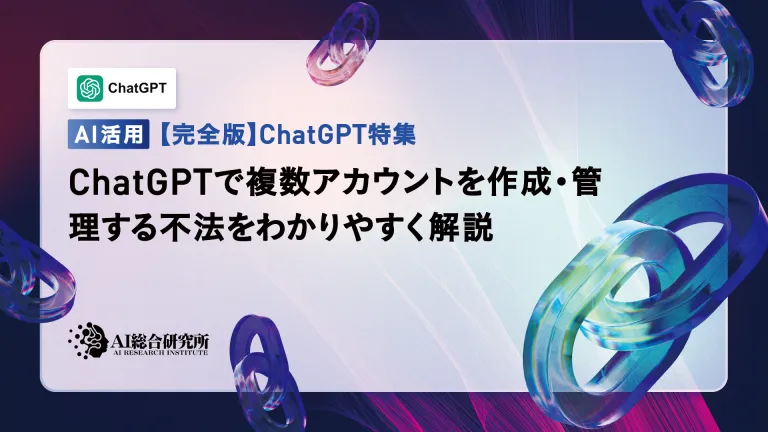
使用chatgpt有效的多个帐户管理技术|关于如何使用商业和私人生活的详尽解释! Chatgpt在各种情况下都使用,但是有些人可能担心管理多个帐户。本文将详细解释如何为ChatGpt创建多个帐户,使用时该怎么做以及如何安全有效地操作它。我们还介绍了重要的一点,例如业务和私人使用差异,并遵守OpenAI的使用条款,并提供指南,以帮助您安全地利用多个帐户。 Openai


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

禅工作室 13.0.1
功能强大的PHP集成开发环境

SublimeText3 Linux新版
SublimeText3 Linux最新版

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。

DVWA
Damn Vulnerable Web App (DVWA) 是一个PHP/MySQL的Web应用程序,非常容易受到攻击。它的主要目标是成为安全专业人员在合法环境中测试自己的技能和工具的辅助工具,帮助Web开发人员更好地理解保护Web应用程序的过程,并帮助教师/学生在课堂环境中教授/学习Web应用程序安全。DVWA的目标是通过简单直接的界面练习一些最常见的Web漏洞,难度各不相同。请注意,该软件中