构建公平的彩票应用:C#泛型列表的随机排序
开发彩票应用的关键在于确保列表元素的随机排序。本文探讨在C#中实现泛型列表<list></list>
随机排序的最佳方法。
Fisher-Yates洗牌算法
Fisher-Yates洗牌算法是高效打乱泛型列表的一种方法。该算法通过扩展方法操作IList
接口:
private static Random rng = new Random(); public static void Shuffle<T>(this IList<T> list) { int n = list.Count; while (n > 1) { n--; int k = rng.Next(n + 1); T value = list[k]; list[k] = list[n]; list[n] = value; } }
使用方法:
只需在任何IList
集合上调用此方法:
List<Product> products = GetProducts(); products.Shuffle();
随机数生成器的考量
System.Random
类虽然方便,但其随机性可能不足。为了提高随机性,建议使用System.Security.Cryptography
中的随机数生成器:
using System.Security.Cryptography; ... public static void Shuffle<T>(this IList<T> list) { RNGCryptoServiceProvider provider = new RNGCryptoServiceProvider(); int n = list.Count; while (n > 1) { byte[] box = new byte[1]; do provider.GetBytes(box); while (!(box[0] < 251)); // Ensure a valid range int k = (int)(box[0] / 251.0 * (n + 1)); // Scale to the list size T value = list[k]; list[k] = list[n]; list[n] = value; } }
线程安全改进
为了避免多线程环境下的潜在问题,可以改进线程安全性:
using System; using System.Collections.Generic; using System.Threading; ... static class MyExtensions { public static void Shuffle<T>(this IList<T> list) { int n = list.Count; while (n > 1) { n--; int k = ThreadSafeRandom.ThisThreadsRandom.Next(n + 1); T value = list[k]; list[k] = list[n]; list[n] = value; } } }
这确保每个线程使用其自身的随机数生成器,避免冲突。
通过这些方法,您可以有效地随机排序泛型列表中的元素,从而创建真正随机的彩票抽奖应用。
以上是我如何有效地将彩票应用程序C#中的通用列表随机化?的详细内容。更多信息请关注PHP中文网其他相关文章!
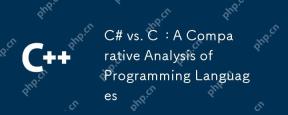
C#和C 的主要区别在于语法、内存管理和性能:1)C#语法现代,支持lambda和LINQ,C 保留C特性并支持模板。2)C#自动内存管理,C 需要手动管理。3)C 性能优于C#,但C#性能也在优化中。
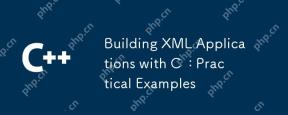
在C 中处理XML数据可以使用TinyXML、Pugixml或libxml2库。1)解析XML文件:使用DOM或SAX方法,DOM适合小文件,SAX适合大文件。2)生成XML文件:将数据结构转换为XML格式并写入文件。通过这些步骤,可以有效地管理和操作XML数据。
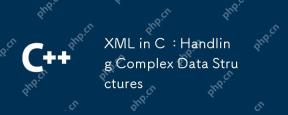
在C 中处理XML数据结构可以使用TinyXML或pugixml库。1)使用pugixml库解析和生成XML文件。2)处理复杂的嵌套XML元素,如书籍信息。3)优化XML处理代码,建议使用高效库和流式解析。通过这些步骤,可以高效处理XML数据。
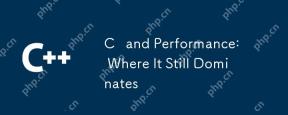
C 在性能优化方面仍然占据主导地位,因为其低级内存管理和高效执行能力使其在游戏开发、金融交易系统和嵌入式系统中不可或缺。具体表现为:1)在游戏开发中,C 的低级内存管理和高效执行能力使得它成为游戏引擎开发的首选语言;2)在金融交易系统中,C 的性能优势确保了极低的延迟和高吞吐量;3)在嵌入式系统中,C 的低级内存管理和高效执行能力使得它在资源有限的环境中非常受欢迎。
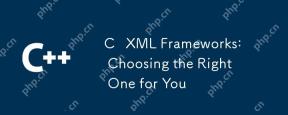
C XML框架的选择应基于项目需求。1)TinyXML适合资源受限环境,2)pugixml适用于高性能需求,3)Xerces-C 支持复杂的XMLSchema验证,选择时需考虑性能、易用性和许可证。
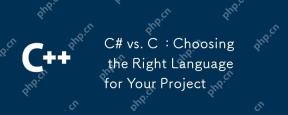
C#适合需要开发效率和类型安全的项目,而C 适合需要高性能和硬件控制的项目。 1)C#提供垃圾回收和LINQ,适用于企业应用和Windows开发。 2)C 以高性能和底层控制着称,广泛用于游戏和系统编程。
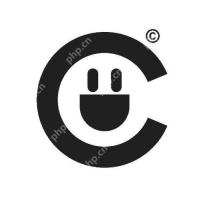
C 代码优化可以通过以下策略实现:1.手动管理内存以优化使用;2.编写符合编译器优化规则的代码;3.选择合适的算法和数据结构;4.使用内联函数减少调用开销;5.应用模板元编程在编译时优化;6.避免不必要的拷贝,使用移动语义和引用参数;7.正确使用const帮助编译器优化;8.选择合适的数据结构,如std::vector。
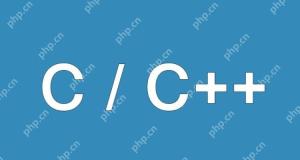
C 中的volatile关键字用于告知编译器变量值可能在代码控制之外被改变,因此不能对其进行优化。1)它常用于读取可能被硬件或中断服务程序修改的变量,如传感器状态。2)volatile不能保证多线程安全,应使用互斥锁或原子操作。3)使用volatile可能导致性能slight下降,但确保程序正确性。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具
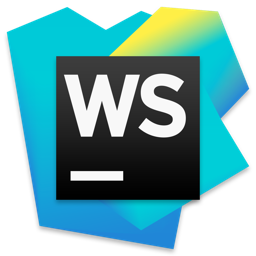
WebStorm Mac版
好用的JavaScript开发工具

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。
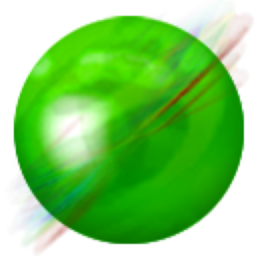
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境

禅工作室 13.0.1
功能强大的PHP集成开发环境
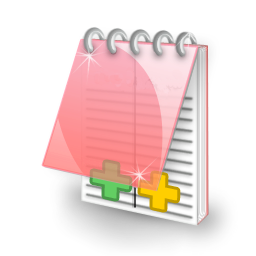
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能