此代码片段演示了如何在表单控件中显示实时命令输出。让我们对其进行改进,使其更加清晰和准确。
改进的解释和代码:
核心功能涉及异步执行命令并使用每行输出更新表单的文本框。 原始代码的主要问题在于其对线程同步的处理。 Invoke
会阻塞,直到 UI 线程完成更新,这可能会导致延迟。 BeginInvoke
更好,因为它将更新排队并立即返回。然而,即使是 BeginInvoke
也并不完全适合这里。 更强大的方法使用专用的 SynchronizationContext
.
以下是改进方法和代码的详细说明:
-
异步命令执行:命令执行应该是异步的,以防止阻塞 UI 线程。 我们将使用
async
和await
。 -
SynchronizationContext: 这可以确保 UI 更新发生在正确的线程上,即使命令输出是从后台线程接收的。
-
错误处理:代码应包含错误处理,以便在命令执行期间妥善管理潜在的异常。
-
更清晰的变量名称:更具描述性的变量名称增强了可读性。
改进的 C# 代码:
private async void btnExecute_Click(object sender, EventArgs e) { // Get currently selected tab page and controls var tabPage = tcExecControl.SelectedTab; var commandTextBox = (TextBox)tabPage.Controls[0]; // Assuming command is in the first control var argumentsTextBox = (TextBox)tabPage.Controls[1]; // Assuming arguments are in the second control var outputTextBox = (TextBox)tabPage.Controls[2]; // Assuming output textbox is the third control string command = commandTextBox.Text; string arguments = argumentsTextBox.Text; try { // Capture the SynchronizationContext for UI thread updates var uiContext = SynchronizationContext.Current; // Asynchronously execute the command await Task.Run(() => { using (var process = new Process()) { process.StartInfo.FileName = command; process.StartInfo.Arguments = arguments; process.StartInfo.UseShellExecute = false; process.StartInfo.RedirectStandardOutput = true; process.StartInfo.RedirectStandardError = true; // Redirect error stream as well process.Start(); // Read output line by line string line; while ((line = process.StandardOutput.ReadLine()) != null) { // Update the textbox on the UI thread uiContext.Post(_ => outputTextBox.AppendText(line + Environment.NewLine), null); } // Read and display error output (if any) string errorLine; while ((errorLine = process.StandardError.ReadLine()) != null) { uiContext.Post(_ => outputTextBox.AppendText("Error: " + errorLine + Environment.NewLine), null); } process.WaitForExit(); } }); } catch (Exception ex) { outputTextBox.AppendText($"An error occurred: {ex.Message}{Environment.NewLine}"); } }
修改后的代码更加健壮、高效和可读。它处理错误,使用异步操作,并使用 SynchronizationContext
正确更新 UI 线程。请记住在代码文件的顶部添加 using System.Threading;
和 using System.Threading.Tasks;
。 另外,请确保在表单的文本框中正确指定命令及其参数。
以上是如何在表单控件中显示实时命令输出?的详细内容。更多信息请关注PHP中文网其他相关文章!
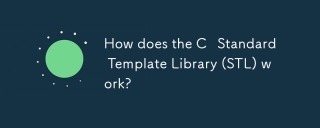
本文解释了C标准模板库(STL),重点关注其核心组件:容器,迭代器,算法和函子。 它详细介绍了这些如何交互以启用通用编程,提高代码效率和可读性t
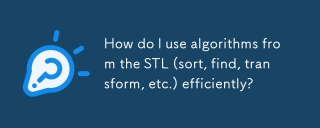
本文详细介绍了c中有效的STL算法用法。 它强调了数据结构选择(向量与列表),算法复杂性分析(例如,std :: sort vs. std vs. std :: partial_sort),迭代器用法和并行执行。 常见的陷阱
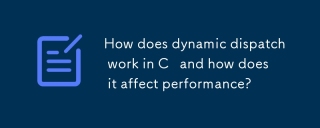
本文讨论了C中的动态调度,其性能成本和优化策略。它突出了动态调度会影响性能并将其与静态调度进行比较的场景,强调性能和之间的权衡
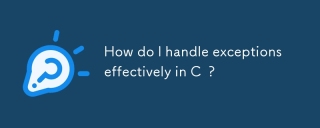
本文详细介绍了C中的有效异常处理,涵盖了尝试,捕捉和投掷机制。 它强调了诸如RAII之类的最佳实践,避免了不必要的捕获块,并为强大的代码登录例外。 该文章还解决了Perf
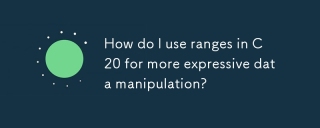
C 20范围通过表现力,合成性和效率增强数据操作。它们简化了复杂的转换并集成到现有代码库中,以提高性能和可维护性。
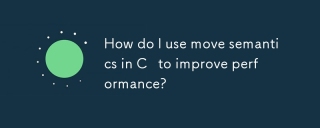
本文讨论了使用C中的移动语义来通过避免不必要的复制来提高性能。它涵盖了使用std :: Move的实施移动构造函数和任务运算符,并确定了关键方案和陷阱以有效
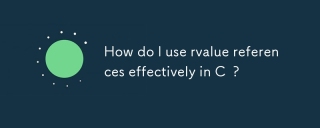
文章讨论了在C中有效使用RVALUE参考,以进行移动语义,完美的转发和资源管理,重点介绍最佳实践和性能改进。(159个字符)


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

PhpStorm Mac 版本
最新(2018.2.1 )专业的PHP集成开发工具
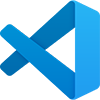
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器
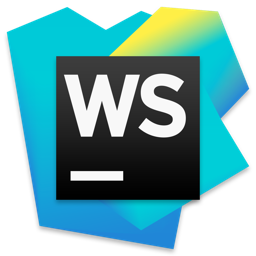
WebStorm Mac版
好用的JavaScript开发工具
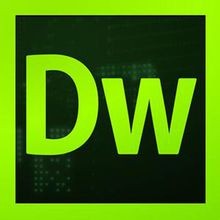
Dreamweaver CS6
视觉化网页开发工具
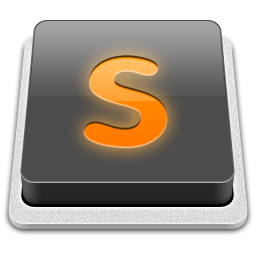
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)