C# 中父进程终止对子进程终止的影响
在软件开发中,处理父进程终止时子进程的行为对于维护系统稳定性至关重要。当多个子进程由父进程生成时,确保它们与父进程一起终止非常重要。本文讨论了一个常见问题:使用 System.Diagnostics.Process
类生成的子进程即使在主应用程序崩溃或通过任务管理器强制终止时仍然存在。
作业对象:解决父子进程层次结构的方案
为了建立子进程和父进程之间的依赖关系,可以使用称为“作业对象”的功能。作业对象允许在进程之间创建层次关系,其中父作业对象的终止也会导致所有关联的子进程终止。
以下代码演示了如何使用作业对象来管理子进程依赖关系:
using System; using System.Runtime.InteropServices; using System.Diagnostics; public class Job : IDisposable { [DllImport("kernel32.dll", CharSet = CharSet.Unicode)] static extern IntPtr CreateJobObject(IntPtr a, string lpName); [DllImport("kernel32.dll")] static extern bool SetInformationJobObject(IntPtr hJob, JobObjectInfoType infoType, IntPtr lpJobObjectInfo, uint cbJobObjectInfoLength); [DllImport("kernel32.dll", SetLastError = true)] static extern bool AssignProcessToJobObject(IntPtr job, IntPtr process); private IntPtr _handle; private bool _disposed = false; public Job() { _handle = CreateJobObject(IntPtr.Zero, null); JOBOBJECT_BASIC_LIMIT_INFORMATION info = new JOBOBJECT_BASIC_LIMIT_INFORMATION(); info.LimitFlags = 0x2000; // JOB_OBJECT_LIMIT_KILL_ON_JOB_CLOSE JOBOBJECT_EXTENDED_LIMIT_INFORMATION extendedInfo = new JOBOBJECT_EXTENDED_LIMIT_INFORMATION(); extendedInfo.BasicLimitInformation = info; int length = Marshal.SizeOf(typeof(JOBOBJECT_EXTENDED_LIMIT_INFORMATION)); IntPtr extendedInfoPtr = Marshal.AllocHGlobal(length); Marshal.StructureToPtr(extendedInfo, extendedInfoPtr, false); if (!SetInformationJobObject(_handle, JobObjectInfoType.ExtendedLimitInformation, extendedInfoPtr, (uint)length)) throw new Exception($"无法设置信息。错误:{Marshal.GetLastWin32Error()}"); Marshal.FreeHGlobal(extendedInfoPtr); } public void AddProcess(Process process) { if (!AssignProcessToJobObject(_handle, process.Handle)) throw new Exception($"无法将进程分配到作业对象。错误:{Marshal.GetLastWin32Error()}"); } public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } protected virtual void Dispose(bool disposing) { if (_disposed) return; if (disposing) { } Close(); _disposed = true; } public void Close() { if (_handle != IntPtr.Zero) { Win32.CloseHandle(_handle); _handle = IntPtr.Zero; } } } internal static class Win32 { [DllImport("kernel32.dll")] internal static extern bool CloseHandle(IntPtr hObject); } // 必要的结构体定义 (根据需要补充完整) enum JobObjectInfoType { ExtendedLimitInformation = 9 } [StructLayout(LayoutKind.Sequential)] internal struct IO_COUNTERS { public UInt64 ReadOperationCount; public UInt64 WriteOperationCount; public UInt64 OtherOperationCount; public UInt64 ReadTransferCount; public UInt64 WriteTransferCount; public UInt64 OtherTransferCount; } [StructLayout(LayoutKind.Sequential)] internal struct JOBOBJECT_BASIC_LIMIT_INFORMATION { public Int64 PerProcessUserTimeLimit; public Int64 PerJobUserTimeLimit; public UInt32 LimitFlags; public UIntPtr MinimumWorkingSetSize; public UIntPtr MaximumWorkingSetSize; public UInt32 ActiveProcessLimit; public UInt32 Affinity; public UInt32 PriorityClass; public UInt32 SchedulingClass; } [StructLayout(LayoutKind.Sequential)] internal struct JOBOBJECT_EXTENDED_LIMIT_INFORMATION { public JOBOBJECT_BASIC_LIMIT_INFORMATION BasicLimitInformation; public IO_COUNTERS IoInfo; public UInt64 ProcessMemoryLimit; public UInt64 JobMemoryLimit; public UInt64 PeakProcessMemoryUsed; public UInt64 PeakJobMemoryUsed; }
在创建子进程后,调用 AddProcess()
方法将其与已建立的作业对象关联。 注意代码中添加了必要的结构体定义和IDisposable
接口的实现,以及对资源的正确释放。
以上是当父程进程以C#结束时,如何确保子进程终止?的详细内容。更多信息请关注PHP中文网其他相关文章!
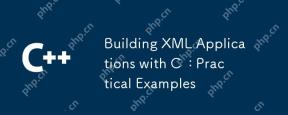
在C 中处理XML数据可以使用TinyXML、Pugixml或libxml2库。1)解析XML文件:使用DOM或SAX方法,DOM适合小文件,SAX适合大文件。2)生成XML文件:将数据结构转换为XML格式并写入文件。通过这些步骤,可以有效地管理和操作XML数据。
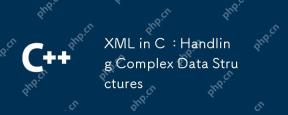
在C 中处理XML数据结构可以使用TinyXML或pugixml库。1)使用pugixml库解析和生成XML文件。2)处理复杂的嵌套XML元素,如书籍信息。3)优化XML处理代码,建议使用高效库和流式解析。通过这些步骤,可以高效处理XML数据。
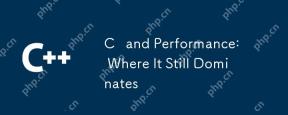
C 在性能优化方面仍然占据主导地位,因为其低级内存管理和高效执行能力使其在游戏开发、金融交易系统和嵌入式系统中不可或缺。具体表现为:1)在游戏开发中,C 的低级内存管理和高效执行能力使得它成为游戏引擎开发的首选语言;2)在金融交易系统中,C 的性能优势确保了极低的延迟和高吞吐量;3)在嵌入式系统中,C 的低级内存管理和高效执行能力使得它在资源有限的环境中非常受欢迎。
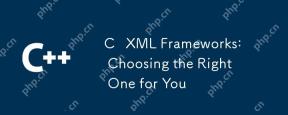
C XML框架的选择应基于项目需求。1)TinyXML适合资源受限环境,2)pugixml适用于高性能需求,3)Xerces-C 支持复杂的XMLSchema验证,选择时需考虑性能、易用性和许可证。
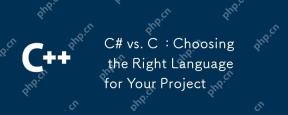
C#适合需要开发效率和类型安全的项目,而C 适合需要高性能和硬件控制的项目。 1)C#提供垃圾回收和LINQ,适用于企业应用和Windows开发。 2)C 以高性能和底层控制着称,广泛用于游戏和系统编程。
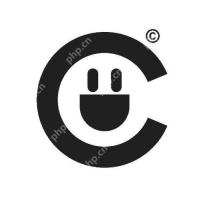
C 代码优化可以通过以下策略实现:1.手动管理内存以优化使用;2.编写符合编译器优化规则的代码;3.选择合适的算法和数据结构;4.使用内联函数减少调用开销;5.应用模板元编程在编译时优化;6.避免不必要的拷贝,使用移动语义和引用参数;7.正确使用const帮助编译器优化;8.选择合适的数据结构,如std::vector。
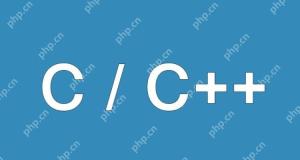
C 中的volatile关键字用于告知编译器变量值可能在代码控制之外被改变,因此不能对其进行优化。1)它常用于读取可能被硬件或中断服务程序修改的变量,如传感器状态。2)volatile不能保证多线程安全,应使用互斥锁或原子操作。3)使用volatile可能导致性能slight下降,但确保程序正确性。
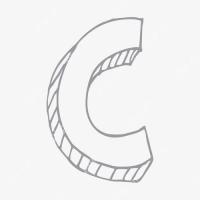
在C 中测量线程性能可以使用标准库中的计时工具、性能分析工具和自定义计时器。1.使用库测量执行时间。2.使用gprof进行性能分析,步骤包括编译时添加-pg选项、运行程序生成gmon.out文件、生成性能报告。3.使用Valgrind的Callgrind模块进行更详细的分析,步骤包括运行程序生成callgrind.out文件、使用kcachegrind查看结果。4.自定义计时器可灵活测量特定代码段的执行时间。这些方法帮助全面了解线程性能,并优化代码。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3 Linux新版
SublimeText3 Linux最新版
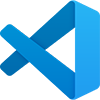
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),