Node.js 以其非阻塞、事件驱动的架构而闻名,擅长处理高并发,尤其是 I/O 密集型任务。 然而,CPU 密集型操作提出了一个挑战:如何防止它们阻塞主事件循环并影响性能? 解决方案在于工作线程。
本文深入研究 Node.js 工作线程,解释它们的功能,将它们与 C 和 Java 等语言中的线程进行对比,并说明它们在处理计算要求较高的任务中的用途。
了解 Node.js 工作线程
Node.js 本质上是在单线程环境中运行的; JavaScript 代码在单个线程(事件循环)上执行。这对于异步 I/O 来说是高效的,但它成为 CPU 密集型任务的瓶颈,例如大型数据集处理、复杂计算或密集的图像/视频操作。
worker_threads
模块通过在多个线程中并行执行 JavaScript 代码来解决此限制。这些线程卸载繁重的计算,保留主事件循环响应能力并提高整体应用程序性能。
工作线程如何工作
Node.js 工作线程是本机操作系统线程,由操作系统管理,就像传统多线程应用程序中的线程一样。 至关重要的是,它们在 Node.js 的单线程 JavaScript 模型中运行,维护内存隔离并通过消息传递进行通信。
考虑这个说明性示例:
const { Worker, isMainThread, parentPort } = require('worker_threads'); if (isMainThread) { // Main thread: Creates a worker const worker = new Worker(__filename); worker.on('message', (message) => { console.log('Message from worker:', message); }); worker.postMessage('Start processing'); } else { // Worker thread: Handles the task parentPort.on('message', (message) => { console.log('Received in worker:', message); const result = heavyComputation(40); parentPort.postMessage(result); }); } function heavyComputation(n) { // Simulates heavy computation (recursive Fibonacci) if (n <= 1) return n; return heavyComputation(n - 1) + heavyComputation(n - 2); }
在这里,主线程使用相同的脚本生成一个工作线程。工作线程执行计算密集型任务(计算斐波那契数)并使用 postMessage()
.
工作线程的主要特性:
- **真正的操作系统线程:**工作线程是真正的操作系统线程,独立运行,适合计算成本较高的操作。
- **隔离内存空间:** 工作线程拥有自己的隔离内存,增强数据完整性并最大限度地减少竞争条件风险。线程间通信依赖于消息传递。
- **非阻塞并发:**工作线程支持并发执行,确保主线程响应能力,同时处理 CPU 密集型任务。
工作线程的最佳用例
在以下情况下在 Node.js 中使用工作线程:
- 涉及 CPU 密集型任务: 密集计算、图像/视频处理或复杂数据操作等可能会阻塞事件循环的任务。
- 需要非阻塞并发:当计算必须在不妨碍事件循环管理其他异步 I/O 操作(例如处理 HTTP 请求)的能力的情况下进行时。
- 需要解决单线程瓶颈:在多核系统上,工作线程利用多个内核,分配计算负载并提高性能。
处理大型数据集(解析大量 CSV 文件、运行机器学习模型)从卸载到工作线程中获益匪浅。
使用工作线程模拟 CPU 密集型任务
让我们研究一下如何模拟 CPU 密集型任务并观察使用工作线程所带来的效率提升。
示例1:斐波那契数列计算
我们将利用简单的递归斐波那契算法(指数复杂度)来模拟繁重的计算。 (上一个示例中的 heavyComputation
函数演示了这一点。)
示例 2:对大数组进行排序
对大型数据集进行排序是另一个经典的 CPU 密集型任务。 我们可以通过对大量随机数进行排序来模拟这一点:
const { Worker, isMainThread, parentPort } = require('worker_threads'); if (isMainThread) { // Main thread: Creates a worker const worker = new Worker(__filename); worker.on('message', (message) => { console.log('Message from worker:', message); }); worker.postMessage('Start processing'); } else { // Worker thread: Handles the task parentPort.on('message', (message) => { console.log('Received in worker:', message); const result = heavyComputation(40); parentPort.postMessage(result); }); } function heavyComputation(n) { // Simulates heavy computation (recursive Fibonacci) if (n <= 1) return n; return heavyComputation(n - 1) + heavyComputation(n - 2); }
对一百万个数字进行排序非常耗时;工作线程可以在主线程保持响应的同时处理这个问题。
示例 3:素数生成
生成大范围内的素数是另一项计算量大的任务。一个简单(低效)的方法是:
function heavyComputation() { const arr = Array.from({ length: 1000000 }, () => Math.random()); arr.sort((a, b) => a - b); return arr[0]; // Return the smallest element for demonstration }
这需要检查每个数字,使其适合卸载到工作线程。
工作线程与其他语言中的线程
Node.js 工作线程与 C 或 Java 中的线程相比如何?
Node.js Worker Threads | C /Java Threads |
---|---|
No shared memory; communication uses message passing. | Threads typically share memory, simplifying data sharing but increasing the risk of race conditions. |
Each worker has its own independent event loop. | Threads run concurrently, each with its own execution flow, sharing a common memory space. |
Communication is via message passing (`postMessage()` and event listeners). | Communication is via shared memory, variables, or synchronization methods (mutexes, semaphores). |
More restrictive but safer for concurrency due to isolation and message passing. | Easier for shared memory access but more prone to deadlocks or race conditions. |
Ideal for offloading CPU-intensive tasks non-blockingly. | Best for tasks requiring frequent shared memory interaction and parallel execution in memory-intensive applications. |
记忆共享与交流:
在 C 和 Java 中,线程通常共享内存,允许直接变量访问。这很有效,但如果多个线程同时修改相同的数据,则会带来竞争条件风险。同步(互斥体、信号量)通常是必要的,这会导致代码复杂。
Node.js 工作线程通过使用消息传递来避免这种情况,从而增强并发应用程序的安全性。 虽然限制性更大,但这种方法可以缓解常见的多线程编程问题。
结论
Node.js 工作线程提供了一种强大的机制,可以在不阻塞主事件循环的情况下处理 CPU 密集型任务。 它们支持并行执行,提高计算要求较高的操作的效率。
与 C 或 Java 中的线程相比,Node.js 工作线程通过强制内存隔离和消息传递通信提供了更简单、更安全的模型。这使得它们更容易在卸载任务对于性能和响应能力至关重要的应用程序中使用。 无论是构建 Web 服务器、执行数据分析还是处理大型数据集,工作线程都可以显着提高性能。
以上是了解 Node.js 中的工作线程:深入探讨的详细内容。更多信息请关注PHP中文网其他相关文章!
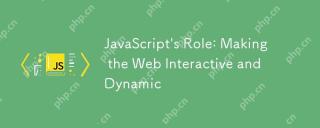
JavaScript是现代网站的核心,因为它增强了网页的交互性和动态性。1)它允许在不刷新页面的情况下改变内容,2)通过DOMAPI操作网页,3)支持复杂的交互效果如动画和拖放,4)优化性能和最佳实践提高用户体验。
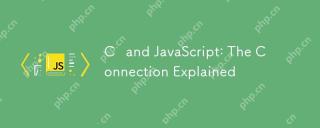
C 和JavaScript通过WebAssembly实现互操作性。1)C 代码编译成WebAssembly模块,引入到JavaScript环境中,增强计算能力。2)在游戏开发中,C 处理物理引擎和图形渲染,JavaScript负责游戏逻辑和用户界面。
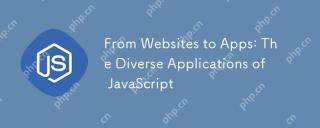
JavaScript在网站、移动应用、桌面应用和服务器端编程中均有广泛应用。1)在网站开发中,JavaScript与HTML、CSS一起操作DOM,实现动态效果,并支持如jQuery、React等框架。2)通过ReactNative和Ionic,JavaScript用于开发跨平台移动应用。3)Electron框架使JavaScript能构建桌面应用。4)Node.js让JavaScript在服务器端运行,支持高并发请求。
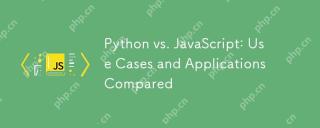
Python更适合数据科学和自动化,JavaScript更适合前端和全栈开发。1.Python在数据科学和机器学习中表现出色,使用NumPy、Pandas等库进行数据处理和建模。2.Python在自动化和脚本编写方面简洁高效。3.JavaScript在前端开发中不可或缺,用于构建动态网页和单页面应用。4.JavaScript通过Node.js在后端开发中发挥作用,支持全栈开发。
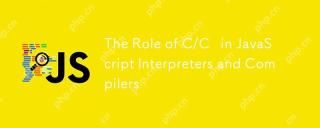
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。
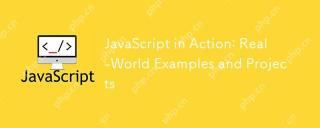
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
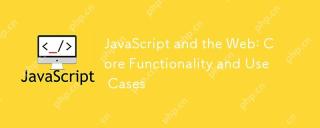
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
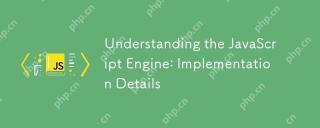
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具
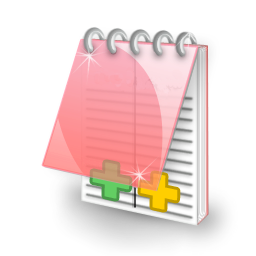
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用
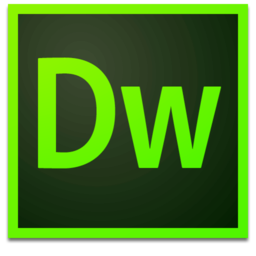
Dreamweaver Mac版
视觉化网页开发工具

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。