本教程为初学者演示了 Go 接口。我们将创建一个 ProofOfId
接口来定义身份证件(身份证、驾照、护照)的方法,以及一个用于列出国家/地区的 CountriesList
接口。 这说明了接口如何以多态性的形式发挥作用,取代其他语言中的继承。
项目设置
- 创建项目目录:
mkdir proof-of-identity-checker && cd proof-of-identity-checker
- 初始化 Go 模块:
go mod init <yourname>/proof-of-identity-checker</yourname>
(将<yourname></yourname>
替换为您的姓名或合适的标识符)。 - 在代码编辑器中打开目录。
定义接口 (interfaces.go
)
package main import "time" type ProofOfId interface { getExpDate() time.Time getName() string getObtentionDate() time.Time } type CountriesList interface { getCountries() []string }
ProofOfId
需要 getExpDate()
、getName()
和 getObtentionDate()
。 CountriesList
需要 getCountries()
。
基于接口的函数 (main.go
)
package main import "time" // IdentityVerification checks if a proof of ID is valid (date-based). func IdentityVerification(proof ProofOfId) bool { // ... (Date comparison logic would go here. See the provided link for details.) return proof.getExpDate().After(time.Now()) //Example: Check if expiration date is in the future. } // DisplayVisitedCountries prints a list of visited countries. func DisplayVisitedCountries(passport CountriesList) { countries := passport.getCountries() println("Visited countries:") for _, country := range countries { println(country) } }
实施身份证明文件类型
- 身份证 (
idcard.go
)
package main import "time" type IdCard struct { Name string ObtentionDate time.Time ExpDate time.Time } func (card IdCard) getName() string { return card.Name } func (card IdCard) getExpDate() time.Time { return card.ExpDate } func (card IdCard) getObtentionDate() time.Time { return card.ObtentionDate }
- 驾驶执照 (
driver-license.go
)
package main import "time" type DriverLicense struct { Name string ObtentionDate time.Time ExpDate time.Time Enterprise string } func (license DriverLicense) getName() string { return license.Name } func (license DriverLicense) getExpDate() time.Time { return license.ExpDate } func (license DriverLicense) getObtentionDate() time.Time { return license.ObtentionDate } func (license DriverLicense) getEnterprise() string { return license.Enterprise }
- 护照 (
passport.go
)
package main import "time" type Passport struct { Name string ObtentionDate time.Time ExpDate time.Time VisitedCountries []string } func (passport Passport) getName() string { return passport.Name } func (passport Passport) getExpDate() time.Time { return passport.ExpDate } func (passport Passport) getObtentionDate() time.Time { return passport.ObtentionDate } func (passport Passport) getCountries() []string { return passport.VisitedCountries }
测试(main.go
继续)
func main() { idCard := IdCard{ObtentionDate: time.Now().Add(24 * time.Hour), ExpDate: time.Now().Add(730 * 24 * time.Hour)} driverLicense := DriverLicense{ObtentionDate: time.Now().Add(-24 * time.Hour), ExpDate: time.Now().Add(365 * 24 * time.Hour)} passport := Passport{ObtentionDate: time.Now().Add(-24 * time.Hour), ExpDate: time.Now().Add(-1 * time.Hour), VisitedCountries: []string{"France", "Spain", "Belgium"}} println(IdentityVerification(idCard)) println(IdentityVerification(driverLicense)) println(IdentityVerification(passport)) DisplayVisitedCountries(passport) }
与go run .
结论
这个示例展示了 Go 接口如何通过定义对象之间的契约来实现灵活的代码,从而提高代码的可重用性和可维护性。 应参考提供的日期比较链接来完成 IdentityVerification
函数的日期检查逻辑。
以上是小Go接口示例:身份证证明的详细内容。更多信息请关注PHP中文网其他相关文章!
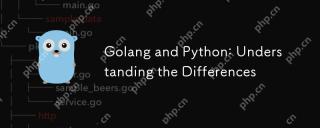
Golang和Python的主要区别在于并发模型、类型系统、性能和执行速度。1.Golang使用CSP模型,适用于高并发任务;Python依赖多线程和GIL,适合I/O密集型任务。2.Golang是静态类型,Python是动态类型。3.Golang编译型语言执行速度快,Python解释型语言开发速度快。
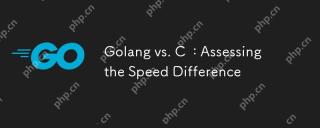
Golang通常比C 慢,但Golang在并发编程和开发效率上更具优势:1)Golang的垃圾回收和并发模型使其在高并发场景下表现出色;2)C 通过手动内存管理和硬件优化获得更高性能,但开发复杂度较高。
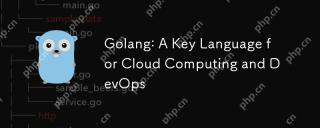
Golang在云计算和DevOps中的应用广泛,其优势在于简单性、高效性和并发编程能力。1)在云计算中,Golang通过goroutine和channel机制高效处理并发请求。2)在DevOps中,Golang的快速编译和跨平台特性使其成为自动化工具的首选。
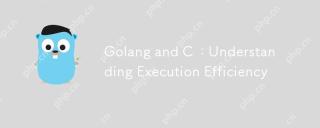
Golang和C 在执行效率上的表现各有优势。1)Golang通过goroutine和垃圾回收提高效率,但可能引入暂停时间。2)C 通过手动内存管理和优化实现高性能,但开发者需处理内存泄漏等问题。选择时需考虑项目需求和团队技术栈。
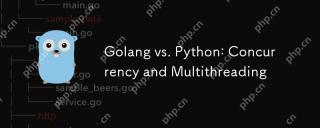
Golang更适合高并发任务,而Python在灵活性上更有优势。1.Golang通过goroutine和channel高效处理并发。2.Python依赖threading和asyncio,受GIL影响,但提供多种并发方式。选择应基于具体需求。
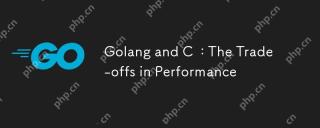
Golang和C 在性能上的差异主要体现在内存管理、编译优化和运行时效率等方面。1)Golang的垃圾回收机制方便但可能影响性能,2)C 的手动内存管理和编译器优化在递归计算中表现更为高效。
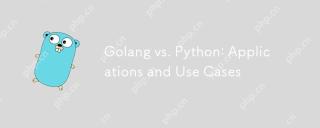
selectgolangforhighpperformanceandcorrency,ifealforBackendServicesSandNetwork程序; selectpypypythonforrapiddevelopment,dataScience和machinelearningDuetoitsverserverserverserversator versator anderticality andextility andextentensivelibraries。

Golang和Python各有优势:Golang适合高性能和并发编程,Python适用于数据科学和Web开发。 Golang以其并发模型和高效性能着称,Python则以简洁语法和丰富库生态系统着称。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具
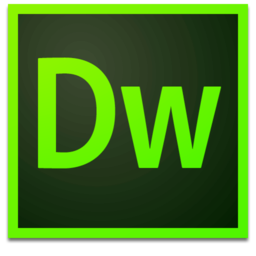
Dreamweaver Mac版
视觉化网页开发工具

PhpStorm Mac 版本
最新(2018.2.1 )专业的PHP集成开发工具

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。
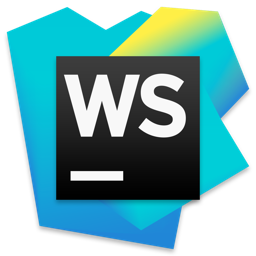
WebStorm Mac版
好用的JavaScript开发工具