WebAssembly (WASM) 是一种基于堆栈的虚拟机的二进制指令格式,设计为高性能应用程序的便携式目标。在本文中,我们将探讨如何将简单的 C 程序编译为 WebAssembly,将其加载到 Web 浏览器中,并使用 JavaScript 与其交互。我们还将探索一些在开发容器环境之外使用 WASM 的有用工具和命令。
设置开发环境
为您的 WebAssembly 项目创建必要的文件夹结构和文件。
- 创建项目文件夹: 首先为您的项目创建一个新目录。在此文件夹中,您将添加必要的文件和配置。
mkdir wasm-web-example cd wasm-web-example
- 设置开发容器: 在 wasm-web-example 目录中,创建 .devcontainer 文件夹来存储 dev 容器配置文件。这些文件将设置一个安装了 Emscripten 的容器,用于将 C 代码编译成 WebAssembly。
在 .devcontainer 文件夹中,创建以下文件:
-
devcontainer.json
此文件将 VSCode 配置为使用具有必要扩展和环境设置的 Docker 容器。
{ "name": "Emscripten DevContainer", "build": { "dockerfile": "Dockerfile" }, "customizations": { "vscode": { "settings": { "terminal.integrated.shell.linux": "/bin/bash", "C_Cpp.default.configurationProvider": "ms-vscode.cmake-tools", "C_Cpp.default.intelliSenseMode": "gcc-x64" }, "extensions": [ "ms-vscode.cpptools", "ms-vscode.cmake-tools" ] } }, "postCreateCommand": "emcc --version" }
-
Dockerfile
Dockerfile 将设置 Emscripten 环境。这是该文件的内容:
# Use the official Emscripten image FROM emscripten/emsdk:3.1.74 # Set the working directory WORKDIR /workspace # Copy the source code into the container COPY . . # Install any additional packages if necessary (optional) # Ensure to clean up cache to minimize image size RUN apt-get update && \ apt-get install -y build-essential && \ apt-get clean && \ rm -rf /var/lib/apt/lists/*
- 创建 VSCode 设置: 在项目的根目录中,创建一个包含以下文件的 .vscode 文件夹:
-
c_cpp_properties.json
此文件配置 C IntelliSense 并包含项目的路径。
{ "configurations": [ { "name": "Linux", "includePath": [ "${workspaceFolder}/**", "/emsdk/upstream/emscripten/system/include" ], "defines": [], "compilerPath": "/usr/bin/gcc", "cStandard": "c17", "cppStandard": "gnu++17", "configurationProvider": "ms-vscode.cmake-tools" } ], "version": 4 }
-
settings.json
此文件包含语言关联的特定 VSCode 设置。
{ "files.associations": { "emscripten.h": "c" }, "[javascript]": { "editor.defaultFormatter": "vscode.typescript-language-features" }, "[typescript]": { "editor.defaultFormatter": "vscode.typescript-language-features" }, "[jsonc]": { "editor.defaultFormatter": "vscode.json-language-features" }, "[json]": { "editor.defaultFormatter": "vscode.json-language-features" }, "[html]": { "editor.defaultFormatter": "vscode.html-language-features" } }
- 创建 C、JavaScript 和 HTML 文件: 现在,为您的项目创建以下文件:
-
test.c
这个 C 文件包含将被编译为 WebAssembly 的简单函数。
// test.c int add(int lhs, int rhs) { return lhs + rhs; }
-
test.html
此 HTML 文件将使用 JavaScript 加载 WebAssembly 模块。
<meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>WebAssembly Example</title> <h1 id="WebAssembly-Example">WebAssembly Example</h1> <div> <li> <p><strong>test.js</strong><br><br> This JavaScript file will fetch the WebAssembly module and call the exported function.<br> </p> <pre class="brush:php;toolbar:false"> // test.js const wasmFile = 'test.wasm'; fetch(wasmFile) .then(response => response.arrayBuffer()) .then(bytes => WebAssembly.instantiate(bytes)) .then(({ instance }) => { const result = instance.exports.add(5, 3); // Call the WebAssembly function document.getElementById('output').textContent = `Result from WebAssembly: ${result}`; }) .catch(error => console.error('Error loading WebAssembly module:', error));
基础 C 程序:
文件 test.c 包含一个简单的函数 add,用于将两个整数相加。我们将使用 Emscripten 将此 C 函数编译为 WebAssembly。Emscripten 命令:
在 dev 容器内,打开终端(在 VSCode 中使用 cmd j)并运行以下 Emscripten 命令将 C 代码编译为 WebAssembly:
现在您已经设置了所有必要的文件和配置,您可以继续编译 WebAssembly 并与 WebAssembly 交互。
使用 Emscripten 将 C 代码编译为 WebAssembly
mkdir wasm-web-example cd wasm-web-example
此命令将生成 test.wasm(WebAssembly 二进制文件),并确保导出 add 函数以在 JavaScript 中使用。
在浏览器中加载 WebAssembly 并与之交互
HTML 设置:
文件 test.html 包含一个简单的 HTML 页面,该页面使用 JavaScript 加载 WebAssembly 二进制文件。JavaScript 设置:
JavaScript 文件 test.js 加载 test.wasm 文件并调用导出的 add 函数:
在 macOS 上使用外部工具
在开发容器之外,您可以运行几个有用的命令来在 Mac 上使用 WebAssembly。
- 安装wabt: wabt(WebAssembly 二进制工具包)提供了用于使用 WebAssembly 的实用程序,包括将 .wasm 文件转换为人类可读的 WAT(WebAssembly 文本)格式。通过 Homebrew 安装:
{ "name": "Emscripten DevContainer", "build": { "dockerfile": "Dockerfile" }, "customizations": { "vscode": { "settings": { "terminal.integrated.shell.linux": "/bin/bash", "C_Cpp.default.configurationProvider": "ms-vscode.cmake-tools", "C_Cpp.default.intelliSenseMode": "gcc-x64" }, "extensions": [ "ms-vscode.cpptools", "ms-vscode.cmake-tools" ] } }, "postCreateCommand": "emcc --version" }
- 将 WASM 转换为 WAT: 安装 wabt 后,您可以使用 wasm2wat 工具将 WebAssembly 二进制文件 (test.wasm) 转换为 WAT 格式:
# Use the official Emscripten image FROM emscripten/emsdk:3.1.74 # Set the working directory WORKDIR /workspace # Copy the source code into the container COPY . . # Install any additional packages if necessary (optional) # Ensure to clean up cache to minimize image size RUN apt-get update && \ apt-get install -y build-essential && \ apt-get clean && \ rm -rf /var/lib/apt/lists/*
- 提供 HTML 页面: 要查看与 WebAssembly 模块交互的 HTML 页面,可以使用 Python 的简单 HTTP 服务器:
{ "configurations": [ { "name": "Linux", "includePath": [ "${workspaceFolder}/**", "/emsdk/upstream/emscripten/system/include" ], "defines": [], "compilerPath": "/usr/bin/gcc", "cStandard": "c17", "cppStandard": "gnu++17", "configurationProvider": "ms-vscode.cmake-tools" } ], "version": 4 }
结论
通过执行以下步骤,您可以设置一个开发环境,将 C 代码编译为 WebAssembly,使用 JavaScript 与其交互,并转换生成的二进制文件以供检查。使用 wabt 和 Python 的 HTTP 服务器等外部工具简化了 macOS 系统上的 WebAssembly 模块的管理和探索。
以上是WebAssembly (WASM) 简介的详细内容。更多信息请关注PHP中文网其他相关文章!
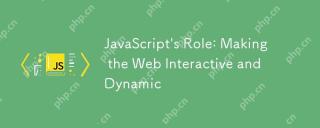
JavaScript是现代网站的核心,因为它增强了网页的交互性和动态性。1)它允许在不刷新页面的情况下改变内容,2)通过DOMAPI操作网页,3)支持复杂的交互效果如动画和拖放,4)优化性能和最佳实践提高用户体验。
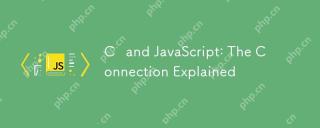
C 和JavaScript通过WebAssembly实现互操作性。1)C 代码编译成WebAssembly模块,引入到JavaScript环境中,增强计算能力。2)在游戏开发中,C 处理物理引擎和图形渲染,JavaScript负责游戏逻辑和用户界面。
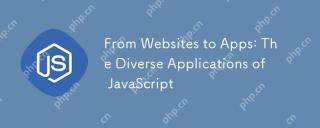
JavaScript在网站、移动应用、桌面应用和服务器端编程中均有广泛应用。1)在网站开发中,JavaScript与HTML、CSS一起操作DOM,实现动态效果,并支持如jQuery、React等框架。2)通过ReactNative和Ionic,JavaScript用于开发跨平台移动应用。3)Electron框架使JavaScript能构建桌面应用。4)Node.js让JavaScript在服务器端运行,支持高并发请求。
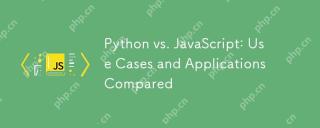
Python更适合数据科学和自动化,JavaScript更适合前端和全栈开发。1.Python在数据科学和机器学习中表现出色,使用NumPy、Pandas等库进行数据处理和建模。2.Python在自动化和脚本编写方面简洁高效。3.JavaScript在前端开发中不可或缺,用于构建动态网页和单页面应用。4.JavaScript通过Node.js在后端开发中发挥作用,支持全栈开发。
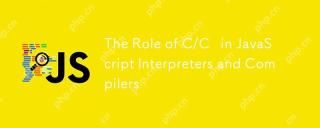
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。
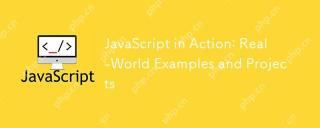
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
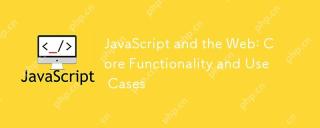
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
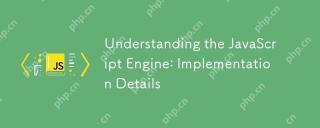
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

SecLists
SecLists是最终安全测试人员的伙伴。它是一个包含各种类型列表的集合,这些列表在安全评估过程中经常使用,都在一个地方。SecLists通过方便地提供安全测试人员可能需要的所有列表,帮助提高安全测试的效率和生产力。列表类型包括用户名、密码、URL、模糊测试有效载荷、敏感数据模式、Web shell等等。测试人员只需将此存储库拉到新的测试机上,他就可以访问到所需的每种类型的列表。

PhpStorm Mac 版本
最新(2018.2.1 )专业的PHP集成开发工具
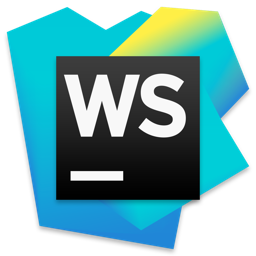
WebStorm Mac版
好用的JavaScript开发工具

记事本++7.3.1
好用且免费的代码编辑器

DVWA
Damn Vulnerable Web App (DVWA) 是一个PHP/MySQL的Web应用程序,非常容易受到攻击。它的主要目标是成为安全专业人员在合法环境中测试自己的技能和工具的辅助工具,帮助Web开发人员更好地理解保护Web应用程序的过程,并帮助教师/学生在课堂环境中教授/学习Web应用程序安全。DVWA的目标是通过简单直接的界面练习一些最常见的Web漏洞,难度各不相同。请注意,该软件中