异步编程在 Python 开发中变得越来越重要。 随着 asyncio
现在成为标准库组件和许多兼容的第三方包,这种范例将继续存在。本教程演示如何使用 HTTPX
库进行异步 HTTP 请求 - 非阻塞代码的主要用例。
什么是非阻塞代码?
“异步”、“非阻塞”和“并发”等术语可能会令人困惑。 本质上:
- 异步例程可以在等待结果时“暂停”,从而允许其他例程同时执行。
- 这会创建并发执行的外观,即使可能不涉及真正的并行性。
异步代码避免阻塞,使其他代码能够在等待结果时运行。 asyncio
库为此提供了工具,并且 aiohttp
提供了专门的 HTTP 请求功能。 HTTP 请求非常适合异步性,因为它们涉及等待服务器响应,在此期间其他任务可以有效执行。
设置
确保您的 Python 环境已配置。 如果需要,请参阅虚拟环境指南(需要 Python 3.7)。 安装HTTPX
:
pip install httpx==0.18.2
使用 HTTPX 发出 HTTP 请求
此示例使用对 Pokémon API 的单个 GET 请求来获取 Mew(Pokémon #151)的数据:
import asyncio import httpx async def main(): url = 'https://pokeapi.co/api/v2/pokemon/151' async with httpx.AsyncClient() as client: response = await client.get(url) pokemon = response.json() print(pokemon['name']) asyncio.run(main())
async
指定一个协程; await
产生对事件循环的控制,在结果可用时恢复执行。
提出多个请求
当发出大量请求时,异步性的真正力量是显而易见的。此示例获取前 150 个 Pokémon 的数据:
import asyncio import httpx import time start_time = time.time() async def main(): async with httpx.AsyncClient() as client: for number in range(1, 151): url = f'https://pokeapi.co/api/v2/pokemon/{number}' response = await client.get(url) pokemon = response.json() print(pokemon['name']) asyncio.run(main()) print(f"--- {time.time() - start_time:.2f} seconds ---")
执行的时间。 将此与同步方法进行比较。
同步请求比较
同步等效项:
import httpx import time start_time = time.time() client = httpx.Client() for number in range(1, 151): url = f'https://pokeapi.co/api/v2/pokemon/{number}' response = client.get(url) pokemon = response.json() print(pokemon['name']) print(f"--- {time.time() - start_time:.2f} seconds ---")
注意运行时差异。 HTTPX
的连接池最大限度地减少了差异,但 asyncio 提供了进一步的优化。
高级异步技术
为了获得卓越的性能,请使用 asyncio.ensure_future
和 asyncio.gather
同时运行请求:
import asyncio import httpx import time start_time = time.time() async def fetch_pokemon(client, url): response = await client.get(url) return response.json()['name'] async def main(): async with httpx.AsyncClient() as client: tasks = [asyncio.ensure_future(fetch_pokemon(client, f'https://pokeapi.co/api/v2/pokemon/{number}')) for number in range(1, 151)] pokemon_names = await asyncio.gather(*tasks) for name in pokemon_names: print(name) asyncio.run(main()) print(f"--- {time.time() - start_time:.2f} seconds ---")
这通过并发运行请求显着减少了执行时间。 总时间接近最长单次请求的持续时间。
结论
使用 HTTPX
和异步编程可以显着提高多个 HTTP 请求的性能。本教程提供了 asyncio
的基本介绍;进一步探索其功能以增强您的 Python 项目。 考虑探索 aiohttp
来替代异步 HTTP 请求处理。
以上是Python 中使用 HTTPX 和 asyncio 的异步 HTTP 请求的详细内容。更多信息请关注PHP中文网其他相关文章!
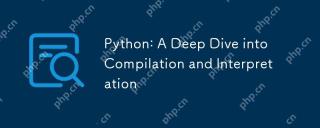
pythonisehybridmodelofcompilationand interpretation:1)thepythoninterspretercompilesourcececodeintoplatform- interpententbybytecode.2)thepytythonvirtualmachine(pvm)thenexecuteCutestestestesteSteSteSteSteSteSthisByTecode,BelancingEaseofuseWithPerformance。
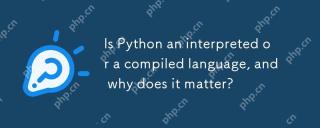
pythonisbothinterpretedAndCompiled.1)它的compiledTobyTecodeForportabilityAcrosplatforms.2)bytecodeisthenInterpreted,允许fordingfordforderynamictynamictymictymictymictyandrapiddefupment,尽管Ititmaybeslowerthananeflowerthanancompiledcompiledlanguages。
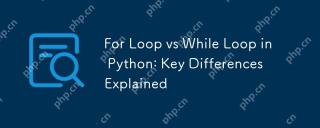
在您的知识之际,而foroopsareideal insinAdvance中,而WhileLoopSareBetterForsituations则youneedtoloopuntilaconditionismet
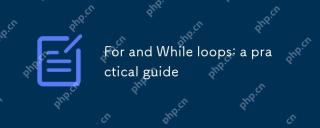
ForboopSareSusedwhenthentheneMberofiterationsiskNownInAdvance,而WhileLoopSareSareDestrationsDepportonAcondition.1)ForloopSareIdealForiteratingOverSequencesLikelistSorarrays.2)whileLeleLooleSuitableApeableableableableableableforscenarioscenarioswhereTheLeTheLeTheLeTeLoopContinusunuesuntilaspecificiccificcificCondond
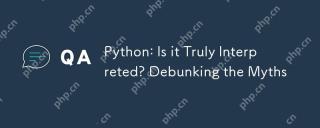
pythonisnotpuroly interpred; itosisehybridablectofbytecodecompilationandruntimeinterpretation.1)PythonCompiLessourceceCeceDintobyTecode,whitsthenexecececected bytybytybythepythepythepythonvirtirtualmachine(pvm).2)
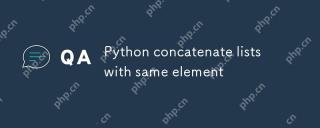
concateNateListsinpythonwithTheSamelements,使用:1)operatototakeepduplicates,2)asettoremavelemavphicates,or3)listCompreanspearensionforcontroloverduplicates,每个methodhasdhasdifferentperferentperferentperforentperforentperforentperfortenceandordormplications。
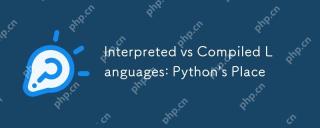
pythonisanterpretedlanguage,offeringosofuseandflexibilitybutfacingperformancelanceLimitationsInCricapplications.1)drightingedlanguageslikeLikeLikeLikeLikeLikeLikeLikeThonexecuteline-by-line,允许ImmediaMediaMediaMediaMediaMediateFeedBackAndBackAndRapidPrototypiD.2)compiledLanguagesLanguagesLagagesLikagesLikec/c thresst
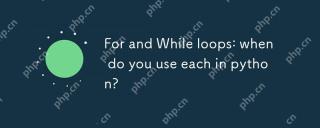
Useforloopswhenthenumberofiterationsisknowninadvance,andwhileloopswheniterationsdependonacondition.1)Forloopsareidealforsequenceslikelistsorranges.2)Whileloopssuitscenarioswheretheloopcontinuesuntilaspecificconditionismet,usefulforuserinputsoralgorit


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

SublimeText3 英文版
推荐:为Win版本,支持代码提示!
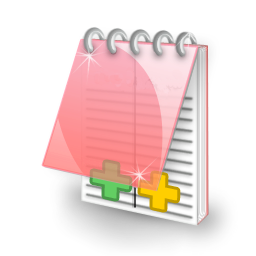
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能
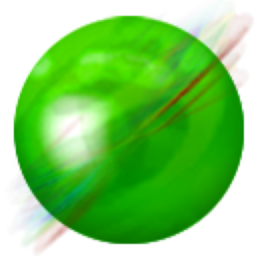
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。
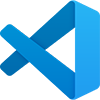
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器