LINQ OrderBy 参数的动态指定
在需要动态指定 LINQ OrderBy 操作中使用的字段的场景中,可以使用反射技术来实现。
示例:
假设存在一个学生列表 existingStudents
和一个表示要排序的字段的参数 param
。
List<student> existingStudents = new List<student> { ... }; string param = "City";
标准实现:
默认方法使用硬编码属性名称调用 OrderBy 进行排序:
List<student> orderedByAddress = existingStudents.OrderBy(c => c.Address).ToList();
动态实现:
使用反射,可以创建一个动态表达式:
public static IQueryable<T> OrderBy<T>(this IQueryable<T> source, string orderByProperty, bool desc) { // 确定排序表达式 string command = desc ? "OrderByDescending" : "OrderBy"; var type = typeof(T); var property = type.GetProperty(orderByProperty); var parameter = Expression.Parameter(type, "p"); var propertyAccess = Expression.MakeMemberAccess(parameter, property); var orderByExpression = Expression.Lambda(propertyAccess, parameter); // 构建动态查询表达式 var resultExpression = Expression.Call(typeof(Queryable), command, new Type[] { type, property.PropertyType }, source.Expression, Expression.Quote(orderByExpression)); return source.Provider.CreateQuery<T>(resultExpression); }
使用方法:
现在可以使用 OrderBy 扩展方法按任何属性动态排序:
List<student> orderbyAddress = existingStudents.OrderBy("City", true); // 降序 List<student> orderbyName = existingStudents.OrderBy("Name", false); // 升序
以上是如何在LINQ中动态指定OrderBy字段?的详细内容。更多信息请关注PHP中文网其他相关文章!
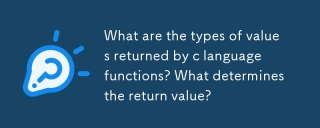
本文详细介绍了C函数返回类型,包括基本(int,float,char等),派生(数组,指针,结构)和void类型。 编译器通过函数声明和返回语句确定返回类型,执行

Gulc是一个高性能的C库,优先考虑最小开销,积极的内衬和编译器优化。 其设计非常适合高频交易和嵌入式系统等关键应用程序,其设计强调简单性,模型
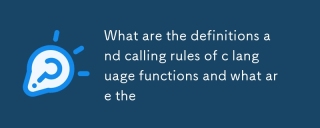
本文解释了C函数声明与定义,参数传递(按值和指针),返回值以及常见的陷阱,例如内存泄漏和类型不匹配。 它强调了声明对模块化和省份的重要性

本文详细介绍了字符串案例转换的C功能。 它可以通过ctype.h的toupper()和tolower()解释,并通过字符串迭代并处理零终端。 常见的陷阱,例如忘记ctype.h和修改字符串文字是
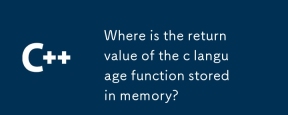
本文研究C函数返回值存储。 较小的返回值通常存储在寄存器中以备速度;较大的值可能会使用指针来记忆(堆栈或堆),影响寿命并需要手动内存管理。直接ACC
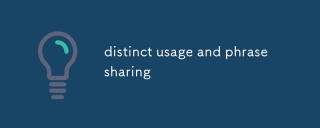
本文分析了形容词“独特”的多方面用途,探索其语法功能,常见的短语(例如,“不同于”,“完全不同”),以及在正式与非正式中的细微应用
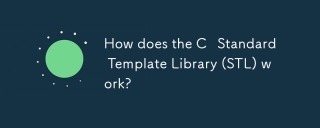
本文解释了C标准模板库(STL),重点关注其核心组件:容器,迭代器,算法和函子。 它详细介绍了这些如何交互以启用通用编程,提高代码效率和可读性t
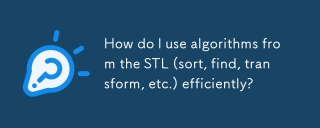
本文详细介绍了c中有效的STL算法用法。 它强调了数据结构选择(向量与列表),算法复杂性分析(例如,std :: sort vs. std vs. std :: partial_sort),迭代器用法和并行执行。 常见的陷阱


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。
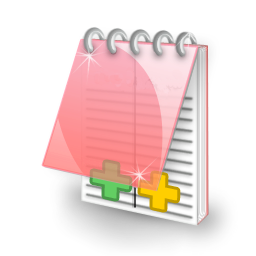
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能
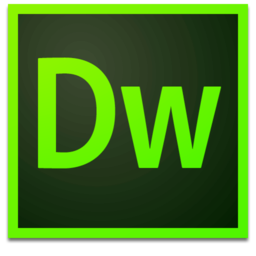
Dreamweaver Mac版
视觉化网页开发工具

记事本++7.3.1
好用且免费的代码编辑器
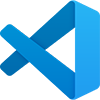
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器