在 .NET Framework 中实现并发 HashSet
简介:
.NET Framework 没有提供并发 HashSet
自定义线程安全实现:
一种方法是创建自定义的线程安全 HashSet
public class ConcurrentHashSet<T> { private readonly HashSet<T> _hashSet = new HashSet<T>(); private readonly object _syncRoot = new object(); public bool Add(T item) { lock (_syncRoot) { return _hashSet.Add(item); } } public bool Remove(T item) { lock (_syncRoot) { return _hashSet.Remove(item); } } // 其他操作可以类似地实现 }
使用 ConcurrentDictionary
另一种方法是利用 System.Collections.Concurrent 命名空间中的 ConcurrentDictionary
private ConcurrentDictionary<T, byte> _concurrentDictionary = new ConcurrentDictionary<T, byte>(); public bool Add(T item) { byte dummyValue = 0; return _concurrentDictionary.TryAdd(item, dummyValue); } public bool Remove(T item) { byte dummyValue; return _concurrentDictionary.TryRemove(item, out dummyValue); } // 其他操作可以类似地实现
注意事项:
在选择方法时,请考虑以下因素:
- 并发安全性:这两种方法都提供对底层数据结构的线程安全访问。
-
性能:在某些情况下,自定义实现可能比 ConcurrentDictionary
具有更好的性能。 -
简洁性:ConcurrentDictionary
提供了更简洁、更直接的实现。 -
HashSet
的适用性: ConcurrentDictionary不会继承自 HashSet ,因此某些 HashSet 特定的功能可能会丢失。
结论:
可以通过实现自定义线程安全包装器或使用 ConcurrentDictionary
以上是如何在.NET Framework中实现并发HashSet?的详细内容。更多信息请关注PHP中文网其他相关文章!
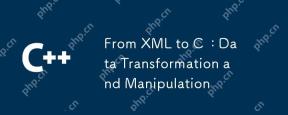
从XML转换到C 并进行数据操作可以通过以下步骤实现:1)使用tinyxml2库解析XML文件,2)将数据映射到C 的数据结构中,3)使用C 标准库如std::vector进行数据操作。通过这些步骤,可以高效地处理和操作从XML转换过来的数据。
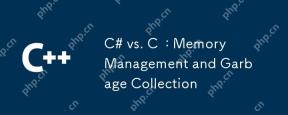
C#使用自动垃圾回收机制,而C 采用手动内存管理。1.C#的垃圾回收器自动管理内存,减少内存泄漏风险,但可能导致性能下降。2.C 提供灵活的内存控制,适合需要精细管理的应用,但需谨慎处理以避免内存泄漏。

C 在现代编程中仍然具有重要相关性。1)高性能和硬件直接操作能力使其在游戏开发、嵌入式系统和高性能计算等领域占据首选地位。2)丰富的编程范式和现代特性如智能指针和模板编程增强了其灵活性和效率,尽管学习曲线陡峭,但其强大功能使其在今天的编程生态中依然重要。
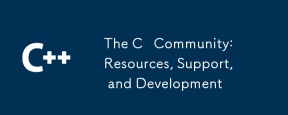
C 学习者和开发者可以从StackOverflow、Reddit的r/cpp社区、Coursera和edX的课程、GitHub上的开源项目、专业咨询服务以及CppCon等会议中获得资源和支持。1.StackOverflow提供技术问题的解答;2.Reddit的r/cpp社区分享最新资讯;3.Coursera和edX提供正式的C 课程;4.GitHub上的开源项目如LLVM和Boost提升技能;5.专业咨询服务如JetBrains和Perforce提供技术支持;6.CppCon等会议有助于职业
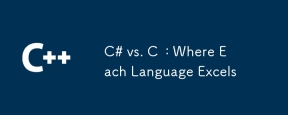
C#适合需要高开发效率和跨平台支持的项目,而C 适用于需要高性能和底层控制的应用。1)C#简化开发,提供垃圾回收和丰富类库,适合企业级应用。2)C 允许直接内存操作,适用于游戏开发和高性能计算。
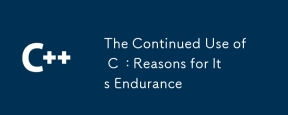
C 持续使用的理由包括其高性能、广泛应用和不断演进的特性。1)高效性能:通过直接操作内存和硬件,C 在系统编程和高性能计算中表现出色。2)广泛应用:在游戏开发、嵌入式系统等领域大放异彩。3)不断演进:自1983年发布以来,C 持续增加新特性,保持其竞争力。
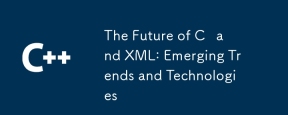
C 和XML的未来发展趋势分别为:1)C 将通过C 20和C 23标准引入模块、概念和协程等新特性,提升编程效率和安全性;2)XML将继续在数据交换和配置文件中占据重要地位,但会面临JSON和YAML的挑战,并朝着更简洁和易解析的方向发展,如XMLSchema1.1和XPath3.1的改进。
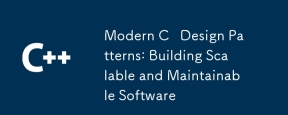
现代C 设计模式利用C 11及以后的新特性实现,帮助构建更灵活、高效的软件。1)使用lambda表达式和std::function简化观察者模式。2)通过移动语义和完美转发优化性能。3)智能指针确保类型安全和资源管理。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),
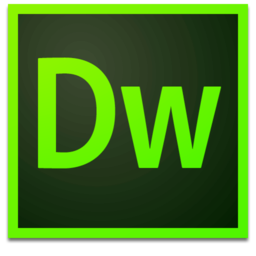
Dreamweaver Mac版
视觉化网页开发工具

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。

SublimeText3汉化版
中文版,非常好用

PhpStorm Mac 版本
最新(2018.2.1 )专业的PHP集成开发工具