Go 并发编程中的计数器同步:Mutex、缓冲通道和非缓冲通道
在 Go 语言中构建并发应用程序时,同步至关重要,以确保安全地访问共享数据。Mutex
和 Channel
是 Go 中用于同步的主要工具。
本文探讨了构建安全并发计数器的几种方法。虽然参考文章使用 Mutex
解决了这个问题,但我们还将探讨使用缓冲通道和非缓冲通道的替代方法。
问题描述
我们需要构建一个可以安全地并发使用的计数器。
计数器代码
package main type Counter struct { count int } func (c *Counter) Inc() { c.count++ } func (c *Counter) Value() int { return c.count }
为了确保代码的并发安全,让我们编写一些测试。
1. 使用 Mutex
Mutex
(互斥锁)是一种同步原语,它确保一次只有一个 goroutine 可以访问代码的关键部分。它提供了一种锁机制:当一个 goroutine 锁定 Mutex
时,其他试图锁定它的 goroutine 将被阻塞,直到 Mutex
被解锁。因此,当需要保护共享变量或资源免受竞争条件影响时,通常会使用它。
package main import ( "sync" "testing" ) func TestCounter(t *testing.T) { t.Run("using mutexes and wait groups", func(t *testing.T) { counter := Counter{} wantedCount := 1000 var wg sync.WaitGroup var mut sync.Mutex wg.Add(wantedCount) for i := 0; i < wantedCount; i++ { go func() { defer wg.Done() mut.Lock() counter.Inc() mut.Unlock() }() } wg.Wait() if counter.Value() != wantedCount { t.Errorf("got %d, want %d", counter.Value(), wantedCount) } }) }
代码使用了 sync.WaitGroup
来跟踪所有 goroutine 的完成情况,并使用 sync.Mutex
来防止多个 goroutine 同时访问共享计数器。
2. 使用缓冲通道
通道是 Go 允许 goroutine 安全通信的一种方式。它们能够在 goroutine 之间传输数据,并通过控制对所传递数据的访问来提供同步。
在本例中,我们将利用通道来阻塞 goroutine,并只允许一个 goroutine 访问共享数据。缓冲通道具有固定的容量,这意味着它们可以在阻塞发送方之前容纳预定义数量的元素。只有当缓冲区已满时,发送方才会被阻塞。
package main import ( "sync" "testing" ) func TestCounter(t *testing.T) { t.Run("using buffered channels and wait groups", func(t *testing.T) { counter := Counter{} wantedCount := 1000 var wg sync.WaitGroup wg.Add(wantedCount) ch := make(chan struct{}, 1) ch <- struct{}{} // 允许第一个 goroutine 开始 for i := 0; i < wantedCount; i++ { go func() { defer wg.Done() <-ch counter.Inc() ch <- struct{}{} }() } wg.Wait() if counter.Value() != wantedCount { t.Errorf("got %d, want %d", counter.Value(), wantedCount) } }) }
代码使用容量为 1 的缓冲通道,允许一次只有一个 goroutine 访问计数器。
3. 使用非缓冲通道
非缓冲通道没有缓冲区。它们会阻塞发送方,直到接收方准备好接收数据。这提供了严格的同步,其中数据一次一个地传递到 goroutine 之间。
package main import ( "sync" "testing" ) func TestCounter(t *testing.T) { t.Run("using unbuffered channels and wait groups", func(t *testing.T) { counter := Counter{} wantedCount := 1000 var wg sync.WaitGroup wg.Add(wantedCount) ch := make(chan struct{}) go func() { for i := 0; i < wantedCount; i++ { ch <- struct{}{} } close(ch) }() for range ch { counter.Inc() wg.Done() } if counter.Value() != wantedCount { t.Errorf("got %d, want %d", counter.Value(), wantedCount) } }) }
代码使用非缓冲通道,确保一次只有一个 goroutine 访问计数器。
4. 使用缓冲通道,不使用 WaitGroup
我们还可以使用缓冲通道而不使用 WaitGroup
,例如使用无限循环或另一个通道来跟踪 goroutine 的完成情况。
结论
本文探讨了在 Go 中构建安全并发计数器的不同方法。掌握这些工具以及何时使用它们是编写高效且安全的并发 Go 程序的关键。
参考资源
本文受《Learn Go with tests》中同步章节的启发。
希望本文对您有所帮助!
以上是Go 并发:互斥体与通道的示例的详细内容。更多信息请关注PHP中文网其他相关文章!
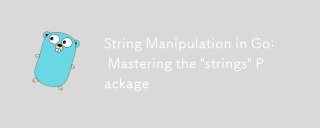
掌握Go语言中的strings包可以提高文本处理能力和开发效率。1)使用Contains函数检查子字符串,2)用Index函数查找子字符串位置,3)Join函数高效拼接字符串切片,4)Replace函数替换子字符串。注意避免常见错误,如未检查空字符串和大字符串操作性能问题。
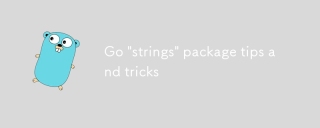
你应该关心Go语言中的strings包,因为它能简化字符串操作,使代码更清晰高效。1)使用strings.Join高效拼接字符串;2)用strings.Fields按空白符分割字符串;3)通过strings.Index和strings.LastIndex查找子串位置;4)用strings.ReplaceAll进行字符串替换;5)利用strings.Builder进行高效字符串拼接;6)始终验证输入以避免意外结果。
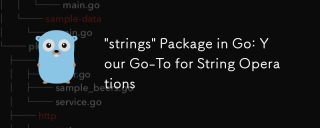
thestringspackageingoisesential forefficientstringManipulation.1)itoffersSimpleyetpoperfulfunctionsFortaskSlikeCheckingSslingSubstringsStringStringsStringsandStringsN.2)ithandhishiCodeDewell,withFunctionsLikestrings.fieldsfieldsfieldsfordsforeflikester.fieldsfordsforwhitespace-fieldsforwhitespace-separatedvalues.3)3)
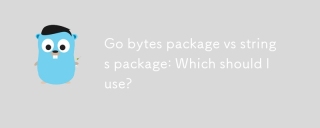
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
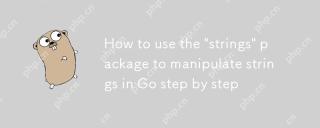
Go的strings包提供了多种字符串操作功能。1)使用strings.Contains检查子字符串。2)用strings.Split将字符串分割成子字符串切片。3)通过strings.Join合并字符串。4)用strings.TrimSpace或strings.Trim去除字符串首尾的空白或指定字符。5)用strings.ReplaceAll替换所有指定子字符串。6)使用strings.HasPrefix或strings.HasSuffix检查字符串的前缀或后缀。
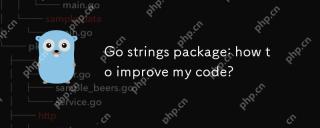
使用Go语言的strings包可以提升代码质量。1)使用strings.Join()优雅地连接字符串数组,避免性能开销。2)结合strings.Split()和strings.Contains()处理文本,注意大小写敏感问题。3)避免滥用strings.Replace(),考虑使用正则表达式进行大量替换。4)使用strings.Builder提高频繁拼接字符串的性能。
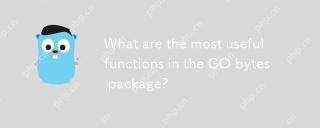
Go的bytes包提供了多种实用的函数来处理字节切片。1.bytes.Contains用于检查字节切片是否包含特定序列。2.bytes.Split用于将字节切片分割成smallerpieces。3.bytes.Join用于将多个字节切片连接成一个。4.bytes.TrimSpace用于去除字节切片的前后空白。5.bytes.Equal用于比较两个字节切片是否相等。6.bytes.Index用于查找子切片在largerslice中的起始索引。
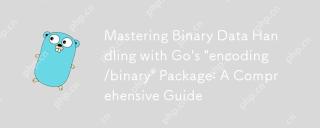
theEncoding/binarypackageingoisesenebecapeitProvidesAstandArdArdArdArdArdArdArdArdAndWriteBinaryData,确保Cross-cross-platformCompatibilitiational and handhandlingdifferentendenness.itoffersfunctionslikeread,写下,写,dearte,readuvarint,andwriteuvarint,andWriteuvarIntforPreciseControloverBinary


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具
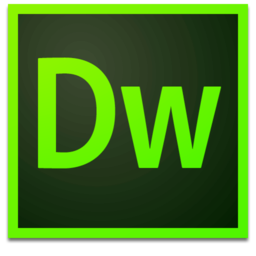
Dreamweaver Mac版
视觉化网页开发工具
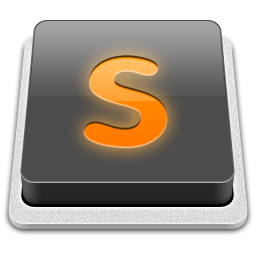
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
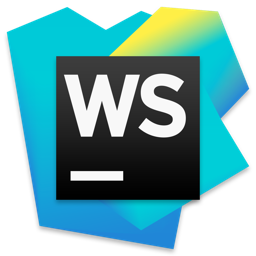
WebStorm Mac版
好用的JavaScript开发工具

Atom编辑器mac版下载
最流行的的开源编辑器

DVWA
Damn Vulnerable Web App (DVWA) 是一个PHP/MySQL的Web应用程序,非常容易受到攻击。它的主要目标是成为安全专业人员在合法环境中测试自己的技能和工具的辅助工具,帮助Web开发人员更好地理解保护Web应用程序的过程,并帮助教师/学生在课堂环境中教授/学习Web应用程序安全。DVWA的目标是通过简单直接的界面练习一些最常见的Web漏洞,难度各不相同。请注意,该软件中