本文探讨了 Java 的 Swing 工具包。一个使用 JFrame、JDialog 和 JApplet 等组件构建图形用户界面 (GUI) 的库,这些组件充当基本的顶级容器。它还演示了如何使用 Swing 组件创建简单的联系表单。
Swing 是一个用于创建简单的图形用户界面 (GUI) 的 Java 库。它是一个 GUI 小部件工具包,提供用于构建 GUI 的组件集合。 (Oracle 文档,n.d.a)。 Swing 组件完全用 Java 编程语言编写。共有三个通常有用的顶级容器类:JFrame、JDialog 和 JApplet(Oracle 文档,n.d. b)。
顶级容器:
JFrame 是一个带有标题和边框的顶级窗口。
(Oracle 文档,n.d.b,如何制作框架(主 Windows)。
JDialog 窗口是一个独立的子窗口,除了主 Swing 应用程序窗口之外,临时会注意到它。
(Oracle 文档,n.d.b,如何创建对话框)
“JApplet,Java applet 是一种特殊的 Java 程序,支持 Java 技术的浏览器可以从互联网下载并运行。小程序通常嵌入网页内并在浏览器上下文中运行。小程序必须是小程序的子类。小程序类。 Applet 类提供了 applet 和浏览器环境之间的标准接口”(Oracle 文档,n.d.c)。
每个使用 Swing 组件的程序都至少包含一个顶级容器。这个顶级容器充当包含层次结构的根,它包含容器内的所有 Swing 组件(Oracle 文档,n.d.b)。
通常,具有基于 Swing 的 GUI 的独立应用程序将至少有一个以 JFrame 作为根的包含层次结构。例如,如果应用程序具有一个主窗口和两个对话框,则它将具有三个包含层次结构,每个层次结构都有自己的顶级容器。主窗口将有一个 JFrame 作为其根,而每个对话框将有一个 JDialog 作为其根。基于 Swing 的 applet 还至少有一个包含层次结构,其中一个以 JApplet 对象为根。例如,显示对话框的小程序将具有两个包含层次结构。浏览器窗口中的组件属于以 JApplet 对象为根的包含层次结构,而对话框属于以 JDialog 对象为根的包含层次结构。
JComponent 类:
除了顶级容器之外,所有以“J”开头的 Swing 组件都派生自 JComponent 类。例如,JPanel、JScrollPane、JButton 和 JTable 都继承自 JComponent。但是,JFrame 和 JDialog 则不然,因为它们是顶级容器(Oracle 文档、n.d.b、JComponent 类)
框架和面板之间的差异:
框架:
JFrame 是一个顶级容器,代表一个带有标题、边框和按钮的窗口。
它通常用作应用程序的主窗口。
一个JFrame可以包含多个组件,包括JPanel、JScrollPane、JButton、JTable等
面板:
JPanel 是一个通用容器,用于将窗口中的一组组件分组在一起。
它没有标题栏或关闭按钮等窗口装饰。
JPanel 通常用于组织和管理 JFrame 内的布局。
每个使用 Swing 组件的程序都至少包含一个顶级容器。这个顶级容器充当包含层次结构的根,它包含容器内的所有 Swing 组件(Oracle 文档,n.d.b)。
通常,具有基于 Swing 的 GUI 的独立应用程序将至少有一个以 JFrame 作为根的包含层次结构。例如,如果应用程序具有一个主窗口和两个对话框,则它将具有三个包含层次结构,每个层次结构都有自己的顶级容器。主窗口将以 JFrame 作为其根,而每个对话框将以 JDialog 作为其根。
基于 Swing 的 applet 还至少有一个包含层次结构,其中一个以 JApplet 对象为根。例如,显示对话框的小程序将具有两个包含层次结构。浏览器窗口中的组件属于以 JApplet 对象为根的包含层次结构,而对话框属于以 JDialog 对象为根的包含层次结构。
下面的示例包括 JFrame 和 JPanel,以及使用 GridBagLayout 的其他组件,例如按钮、文本字段和标签。此外,它还使用 JDialog、JOptionPane 组件和 Dialog 窗口组件显示消息。这是一个使用 Swing 组件的简单图形用户界面 (GUI) 联系表单。
//--- Abstract Window Toolkit (AWT) // Provides layout manager for arranging components in five regions: // north, south, east, west, and center. import java.awt.BorderLayout; // Grid layout - Specifies constraints for components that are laid out using the GridBagLayout. import java.awt.GridBagConstraints; // Grid - layout manager that aligns components vertically and horizontally, // without requiring the components to be of the same size. import java.awt.GridBagLayout; // Gird padding - Specifies the space (padding) between components and their borders. import java.awt.Insets; // Button - Provides the capability to handle action events like button clicks. import java.awt.event.ActionEvent; // Button event - Allows handling of action events, such as button clicks. import java.awt.event.ActionListener; //--- swing GUI // Button - Provides a button component that can trigger actions when clicked. import javax.swing.JButton; // Frame - Provides a window with decorations // such as a title, border, and buttons for closing and minimizing. import javax.swing.JFrame; // Labels - Provides a display area for a short text string or an image, or both. import javax.swing.JLabel; // Submition Message - Provides standard dialog boxes such as message, input, and confirmation dialogs. import javax.swing.JOptionPane; // Panel - Provides a generic container for grouping components together. import javax.swing.JPanel; // Scroll user message - Provides to the a scrollable view of a lightweight component. import javax.swing.JScrollPane; // User message - Provides a multi-line area to display/edit plain text. import javax.swing.JTextArea; // Name & Email - Provides a single-line text field for user input. import javax.swing.JTextField; /** * This class generates a simple contact form. The form includes fields for the * user's name, email, and message, and a submit button to submit the form. * * @author Alejandro Ricciardi * @version 1.0 * @date 06/16/2024 */ public class contactForm { /** * The main method to create and display the contact form. * * @param args Command line arguments */ public static void main(String[] args) { /*------------ | Frame | ------------*/ // ---- Initializes frame // Creates the main application frame JFrame frame = new JFrame("Contact Form"); frame.setSize(400, 300); // Set the size of the frame // Close the application when the frame is closed frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setLayout(new BorderLayout()); // Use BorderLayout for the frame /*------------ | Panel | ------------*/ // ---- Initializes panel // Create a panel with GridBagLayout JPanel panel = new JPanel(new GridBagLayout()); GridBagConstraints gridForm = new GridBagConstraints(); gridForm.insets = new Insets(5, 5, 5, 5); // Add padding around components // ---- Creates and adds grid components to the panel // -- Name // Adds "Name" label JLabel nameLabel = new JLabel("Name:"); gridForm.gridx = 0; // Position at column 0 gridForm.gridy = 0; // Position at row 0 panel.add(nameLabel, gridForm); // Add text field for name input JTextField nameField = new JTextField(20); gridForm.gridx = 1; // Position at column 1 gridForm.gridy = 0; // Position at row 0 panel.add(nameField, gridForm); // -- Email // Add "Email" label JLabel emailLabel = new JLabel("Email:"); gridForm.gridx = 0; // Position at column 0 gridForm.gridy = 1; // Position at row 1 panel.add(emailLabel, gridForm); // Adds text field for email input JTextField emailField = new JTextField(20); gridForm.gridx = 1; // Position at column 1 gridForm.gridy = 1; // Position at row 1 panel.add(emailField, gridForm); // Adds "Message" label JLabel messageLabel = new JLabel("Message:"); gridForm.gridx = 0; // Position at column 0 gridForm.gridy = 2; // Position at row 2 panel.add(messageLabel, gridForm); // -- Message // Adds text area for message input with a scroll pane JTextArea messageArea = new JTextArea(5, 20); JScrollPane scrollPane = new JScrollPane(messageArea); gridForm.gridx = 1; // Position at column 1 gridForm.gridy = 2; // Position at row 2 panel.add(scrollPane, gridForm); // Adds "Submit" button JButton submitButton = new JButton("Submit"); gridForm.gridx = 1; // Position at column 1 gridForm.gridy = 3; // Position at row 3 panel.add(submitButton, gridForm); // Adds the panel to the frame's center frame.add(panel, BorderLayout.CENTER); // Make the frame visible frame.setVisible(true); /*------------ | JDialog | ------------*/ // Add action listener to the submit button ActionListener submitBtnClicked = new ActionListener() { @Override public void actionPerformed(ActionEvent e) { // Display a message dialog when the submit button is clicked JOptionPane.showMessageDialog(frame, "Message was sent!"); } }; submitButton.addActionListener(submitBtnClicked); } }
如果您有兴趣了解有关对话窗口的更多信息,以下视频介绍了如何实现 JDialog JOptionPane 消息。
总而言之,Java 的 Swing 工具包提供了一组组件,使开发人员能够创建用户友好的、可视化结构化的 GUI。该库使用 JFrame、JDialog 和 JApplet 等顶级容器,以及 JPanel 和 JOptionPane 等基本元素。
参考文献:
Oracle 文档。 (n.d.a)。 摇摆。甲骨文。 https://docs.oracle.com/javase/8/docs/technotes/guides/swing/
Oracle 文档。 (n.d.b)。使用顶级容器。 Java™ 教程。甲骨文。 https://docs.oracle.com/javase/tutorial/uiswing/components/toplevel.html
Oracle 文档。 (n.d.c)。 Java 小程序。 Java™ 教程。甲骨文。 https://docs.oracle.com/javase/tutorial/deployment/applet/index.html
最初由 Alex.omegapy 于 2024 年 11 月 3 日在 Medium 上发布。
以上是使用 Swing 组件创建 Java GUI的详细内容。更多信息请关注PHP中文网其他相关文章!
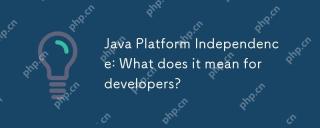
Java'splatFormIndependecemeansDeveloperScanWriteCeandeCeandOnanyDeviceWithouTrecompOlding.thisAcachivedThroughThroughTheroughThejavavirtualmachine(JVM),WhaterslatesbyTecodeDecodeOdeIntComenthendions,允许univerniverSaliversalComplatibilityAcrossplatss.allospplats.s.howevss.howev
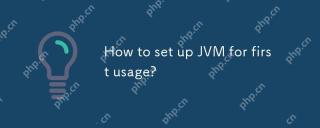
要设置JVM,需按以下步骤进行:1)下载并安装JDK,2)设置环境变量,3)验证安装,4)设置IDE,5)测试运行程序。设置JVM不仅仅是让其工作,还包括优化内存分配、垃圾收集、性能调优和错误处理,以确保最佳运行效果。
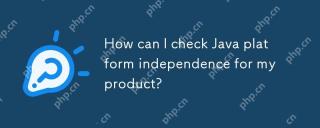
toensurejavaplatFormIntence,lofterTheSeSteps:1)compileAndRunyOpplicationOnmultPlatFormSusiseDifferenToSandjvmversions.2)upureizeci/cdppipipelinelikeinkinslikejenkinsorgithikejenkinsorgithikejenkinsorgithikejenkinsorgithike forautomatecross-plateftestesteftestesting.3)
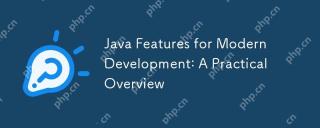
javastandsoutsoutinmoderndevelopmentduetoitsrobustfeatureslikelambdaexpressions,streams,andenhanced concurrencysupport.1)lambdaexpressionssimplifyfunctional promprogientsmangional programmanging,makencodemoreconciseandable.2)
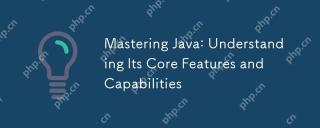
Java的核心特点包括平台独立性、面向对象设计和丰富的标准库。1)面向对象设计通过多态等特性使得代码更加灵活和可维护。2)垃圾回收机制解放了开发者的内存管理负担,但需要优化以避免性能问题。3)标准库提供了从集合到网络的强大工具,但应谨慎选择数据结构以保持代码简洁。
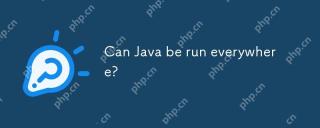
Yes,Javacanruneverywhereduetoits"WriteOnce,RunAnywhere"philosophy.1)Javacodeiscompiledintoplatform-independentbytecode.2)TheJavaVirtualMachine(JVM)interpretsorcompilesthisbytecodeintomachine-specificinstructionsatruntime,allowingthesameJava
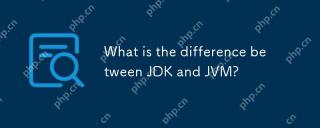
jdkincludestoolsfordevelveping and compilingjavacode,whilejvmrunsthecompiledbytecode.1)jdkcontainsjre,编译器和授权。2)
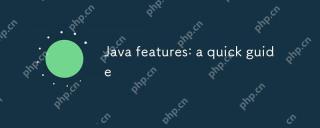
Java的关键特性包括:1)面向对象设计,2)平台独立性,3)垃圾回收机制,4)丰富的库和框架,5)并发支持,6)异常处理,7)持续演进。Java的这些特性使其成为开发高效、可维护软件的强大工具。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境
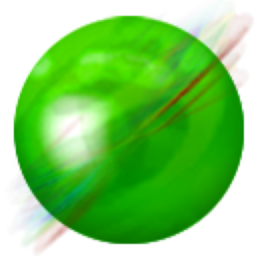
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境
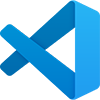
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器
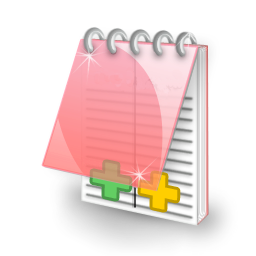
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能