C# 中的命名字符串格式化
在 Python 等编程语言中,您可以按名称而不是位置格式化字符串。例如,您可以有这样的字符串:
print '%(language)s has %(#)03d quote types.' % \ {'language': "Python", "#": 2}
这将打印“Python has 002 quote types.”
在 C# 中,没有用于格式化字符串的内置方法按名字。但是,有几种方法可以实现类似的结果。
使用字典
一种选择是使用字典将变量名称映射到其值。然后,您可以将字典传递给自定义字符串格式化方法,该方法使用变量名称来格式化字符串。
这是一个示例:
public static string FormatStringByName(string format, Dictionary<string object> values) { // Create a regular expression to match variable names in the format string. var regex = new Regex(@"\{(.*?)\}"); // Replace each variable name with its value from the dictionary. var formattedString = regex.Replace(format, match => values[match.Groups[1].Value].ToString()); return formattedString; }</string>
您可以像这样使用此方法:
var values = new Dictionary<string object> { { "some_variable", "hello" }, { "some_other_variable", "world" } }; string formattedString = FormatStringByName("{some_variable}: {some_other_variable}", values);</string>
使用字符串插值
中C# 6.0 及更高版本,您可以使用字符串插值按名称格式化字符串。字符串插值是一项功能,允许您将表达式直接嵌入到字符串中。
这里是一个示例:
string formattedString = $"{some_variable}: {some_other_variable}";
这将打印“hello: world”。
扩展方法
另一种选择是使用扩展方法来添加命名字符串格式化功能到字符串类。以下是您可以使用的扩展方法的示例:
public static class StringExtensions { public static string FormatByName(this string format, object values) { // Get the type of the values object. var type = values.GetType(); // Get the properties of the values object. var properties = type.GetProperties(); // Create a regular expression to match variable names in the format string. var regex = new Regex(@"\{(.*?)\}"); // Replace each variable name with its value from the values object. var formattedString = regex.Replace(format, match => { var property = properties.FirstOrDefault(p => p.Name == match.Groups[1].Value); if (property != null) { return property.GetValue(values).ToString(); } else { return match.Groups[1].Value; } }); return formattedString; } }
您可以像这样使用此扩展方法:
string formattedString = "{some_variable}: {some_other_variable}".FormatByName(new { some_variable = "hello", some_other_variable = "world" });
以上是如何在 C# 中实现命名字符串格式化?的详细内容。更多信息请关注PHP中文网其他相关文章!
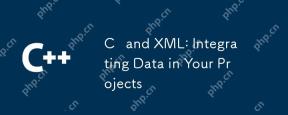
在C 项目中集成XML可以通过以下步骤实现:1)使用pugixml或TinyXML库解析和生成XML文件,2)选择DOM或SAX方法进行解析,3)处理嵌套节点和多级属性,4)使用调试技巧和最佳实践优化性能。
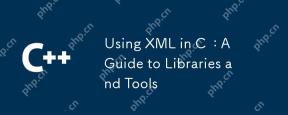
在C 中使用XML是因为它提供了结构化数据的便捷方式,尤其在配置文件、数据存储和网络通信中不可或缺。1)选择合适的库,如TinyXML、pugixml、RapidXML,根据项目需求决定。2)了解XML解析和生成的两种方式:DOM适合频繁访问和修改,SAX适用于大文件或流数据。3)优化性能时,TinyXML适合小文件,pugixml在内存和速度上表现好,RapidXML处理大文件优异。
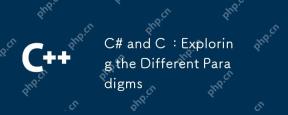
C#和C 的主要区别在于内存管理、多态性实现和性能优化。1)C#使用垃圾回收器自动管理内存,C 则需要手动管理。2)C#通过接口和虚方法实现多态性,C 使用虚函数和纯虚函数。3)C#的性能优化依赖于结构体和并行编程,C 则通过内联函数和多线程实现。
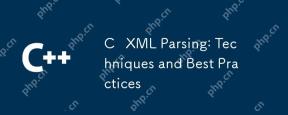
C 中解析XML数据可以使用DOM和SAX方法。1)DOM解析将XML加载到内存,适合小文件,但可能占用大量内存。2)SAX解析基于事件驱动,适用于大文件,但无法随机访问。选择合适的方法并优化代码可提高效率。
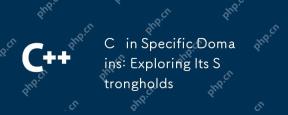
C 在游戏开发、嵌入式系统、金融交易和科学计算等领域中的应用广泛,原因在于其高性能和灵活性。1)在游戏开发中,C 用于高效图形渲染和实时计算。2)嵌入式系统中,C 的内存管理和硬件控制能力使其成为首选。3)金融交易领域,C 的高性能满足实时计算需求。4)科学计算中,C 的高效算法实现和数据处理能力得到充分体现。
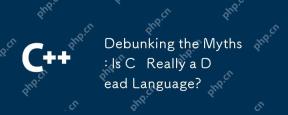
C 没有死,反而在许多关键领域蓬勃发展:1)游戏开发,2)系统编程,3)高性能计算,4)浏览器和网络应用,C 依然是主流选择,展现了其强大的生命力和应用场景。
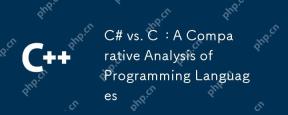
C#和C 的主要区别在于语法、内存管理和性能:1)C#语法现代,支持lambda和LINQ,C 保留C特性并支持模板。2)C#自动内存管理,C 需要手动管理。3)C 性能优于C#,但C#性能也在优化中。
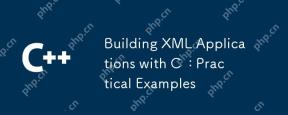
在C 中处理XML数据可以使用TinyXML、Pugixml或libxml2库。1)解析XML文件:使用DOM或SAX方法,DOM适合小文件,SAX适合大文件。2)生成XML文件:将数据结构转换为XML格式并写入文件。通过这些步骤,可以有效地管理和操作XML数据。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。
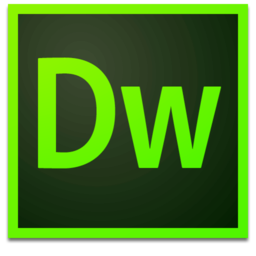
Dreamweaver Mac版
视觉化网页开发工具

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。
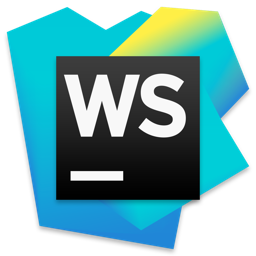
WebStorm Mac版
好用的JavaScript开发工具

禅工作室 13.0.1
功能强大的PHP集成开发环境