使用 C# 计算列表中的出现次数
在 C# 中,有多种方法可用于有效计算列表中每个元素的出现次数。一种方法涉及利用 GroupBy 扩展方法:
using System; using System.Collections.Generic; using System.Linq; List<int> list = new List<int>() { 1, 2, 3, 4, 5, 2, 2, 2, 4, 4, 4, 1 }; // Group the list elements based on their values var groupedList = list.GroupBy(i => i); // Iterate over each group and count the occurrences foreach (var group in groupedList) { Console.WriteLine($"{group.Key}: {group.Count()}"); }</int></int>
在此示例中,GroupBy 方法采用 Func
或者,您可以使用字典
using System; using System.Collections.Generic; List<int> list = new List<int>() { 1, 2, 3, 4, 5, 2, 2, 2, 4, 4, 4, 1 }; // Create a dictionary to store element counts Dictionary<int int> counts = new Dictionary<int int>(); // Populate the dictionary with counts foreach (var item in list) { if (counts.ContainsKey(item)) { counts[item]++; } else { counts[item] = 1; } } // Iterate over the dictionary to print element counts foreach (var kvp in counts) { Console.WriteLine($"{kvp.Key}: {kvp.Value}"); }</int></int></int></int>
以上是如何有效地计算 C# 列表中元素出现的次数?的详细内容。更多信息请关注PHP中文网其他相关文章!
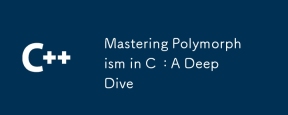
掌握C 中的多态性可以显着提高代码的灵活性和可维护性。 1)多态性允许不同类型的对象被视为同一基础类型的对象。 2)通过继承和虚拟函数实现运行时多态性。 3)多态性支持代码扩展而不修改现有类。 4)使用CRTP实现编译时多态性可提升性能。 5)智能指针有助于资源管理。 6)基类应有虚拟析构函数。 7)性能优化需先进行代码分析。
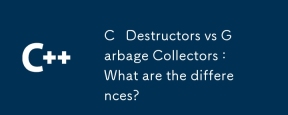
C DestructorSprovidePreciseControloverResourCemangement,whergarBageCollectorSautomateMoryManagementbutintroduceunPredicational.c Destructors:1)允许CustomCleanUpactionsWhenObextionsWhenObextSaredSaredEstRoyed,2)RorreasereSouresResiorSouresiorSourseResiorMeymemsmedwhenEbegtsGoOutofScop
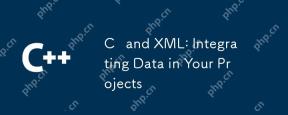
在C 项目中集成XML可以通过以下步骤实现:1)使用pugixml或TinyXML库解析和生成XML文件,2)选择DOM或SAX方法进行解析,3)处理嵌套节点和多级属性,4)使用调试技巧和最佳实践优化性能。
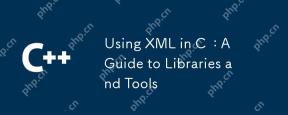
在C 中使用XML是因为它提供了结构化数据的便捷方式,尤其在配置文件、数据存储和网络通信中不可或缺。1)选择合适的库,如TinyXML、pugixml、RapidXML,根据项目需求决定。2)了解XML解析和生成的两种方式:DOM适合频繁访问和修改,SAX适用于大文件或流数据。3)优化性能时,TinyXML适合小文件,pugixml在内存和速度上表现好,RapidXML处理大文件优异。
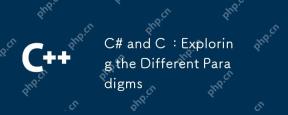
C#和C 的主要区别在于内存管理、多态性实现和性能优化。1)C#使用垃圾回收器自动管理内存,C 则需要手动管理。2)C#通过接口和虚方法实现多态性,C 使用虚函数和纯虚函数。3)C#的性能优化依赖于结构体和并行编程,C 则通过内联函数和多线程实现。
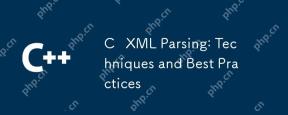
C 中解析XML数据可以使用DOM和SAX方法。1)DOM解析将XML加载到内存,适合小文件,但可能占用大量内存。2)SAX解析基于事件驱动,适用于大文件,但无法随机访问。选择合适的方法并优化代码可提高效率。
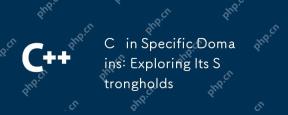
C 在游戏开发、嵌入式系统、金融交易和科学计算等领域中的应用广泛,原因在于其高性能和灵活性。1)在游戏开发中,C 用于高效图形渲染和实时计算。2)嵌入式系统中,C 的内存管理和硬件控制能力使其成为首选。3)金融交易领域,C 的高性能满足实时计算需求。4)科学计算中,C 的高效算法实现和数据处理能力得到充分体现。
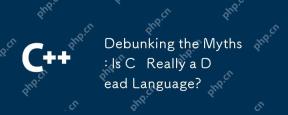
C 没有死,反而在许多关键领域蓬勃发展:1)游戏开发,2)系统编程,3)高性能计算,4)浏览器和网络应用,C 依然是主流选择,展现了其强大的生命力和应用场景。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

SublimeText3汉化版
中文版,非常好用
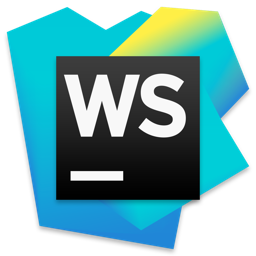
WebStorm Mac版
好用的JavaScript开发工具

禅工作室 13.0.1
功能强大的PHP集成开发环境

SublimeText3 Linux新版
SublimeText3 Linux最新版
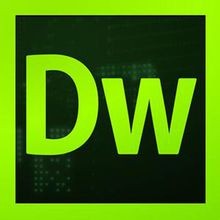
Dreamweaver CS6
视觉化网页开发工具