我爱❤️ TypeScript。
特别是在经历了 JavaScript 臭名昭著的“无法访问未定义的值”错误之后。
然而,即使 TypeScript 很棒,仍然有搬起石头砸自己脚的方法。
在这篇文章中,我将分享 TypeScript 中的 5 个不良做法以及如何避免它们。
?下载我的免费 101 React Tips And Tricks Book,抢占先机。
1. 将错误声明为 Any 类型
例子
在下面的代码片段中,我们捕获错误然后将其声明为类型any。
async function asyncFunction() { try { const response = await doSomething(); return response; } catch (err: any) { toast(`Failed to do something: ${err.message}`); } }
为什么不好❌
不保证错误具有字符串类型的消息字段。
不幸的是,由于类型断言,代码让我们假设它确实如此。
代码可以在开发中使用特定的测试用例,但在生产中可能会严重损坏。
该怎么办呢✅
不要设置错误类型。默认情况下应该是未知的。
相反,您可以执行以下任一操作:
- 选项 1: 使用类型保护检查错误的类型是否正确。
async function asyncFunction() { try { const response = await doSomething(); return response; } catch (err) { const toastMessage = hasMessage(err) ? `Failed to do something: ${err.message}` : `Failed to do something`; toast(toastMessage); } } // We use a type guard to check first function hasMessage(value: unknown): value is { message: string } { return ( value != null && typeof value === "object" && "message" in value && typeof value.message === "string" ); } // You can also simply check if the error is an instance of Error const toastMessage = err instanceof Error ? `Failed to do something: ${err.message}` : `Failed to do something`;
- 选项 2(推荐): 不要对错误做出假设
不要对错误类型做出假设,而是显式处理每种类型并向用户提供适当的反馈。
如果无法确定具体的错误类型,最好显示完整的错误信息而不是部分详细信息。
有关错误处理的更多信息,请查看这个优秀的指南:编写更好的错误消息。
2. 具有多个相同类型的连续参数的函数
例子
export function greet( firstName: string, lastName: string, city: string, email: string ) { // Do something... }
为什么不好❌
- 您可能会意外地以错误的顺序传递参数:
// We inverted firstName and lastName, but TypeScript won't catch this greet("Curry", "Stephen", "LA", "stephen.curry@gmail.com")
- 很难理解每个参数代表什么,尤其是在代码审查期间,当在声明之前看到函数调用时
该怎么办 ✅
使用对象参数来阐明每个字段的用途并最大程度地减少错误风险。
export function greet(params: { firstName: string; lastName: string; city: string; email: string; }) { // Do something... }
3. 具有多个分支且无返回类型的函数
例子
async function asyncFunction() { try { const response = await doSomething(); return response; } catch (err: any) { toast(`Failed to do something: ${err.message}`); } }
为什么不好❌
添加新的 AnimalType 可能会导致返回结构错误的对象。
返回类型结构的更改可能会导致代码其他部分出现难以追踪的问题。
拼写错误可能会导致推断出不正确的类型。
该怎么办 ✅
显式指定函数的返回类型:
async function asyncFunction() { try { const response = await doSomething(); return response; } catch (err) { const toastMessage = hasMessage(err) ? `Failed to do something: ${err.message}` : `Failed to do something`; toast(toastMessage); } } // We use a type guard to check first function hasMessage(value: unknown): value is { message: string } { return ( value != null && typeof value === "object" && "message" in value && typeof value.message === "string" ); } // You can also simply check if the error is an instance of Error const toastMessage = err instanceof Error ? `Failed to do something: ${err.message}` : `Failed to do something`;
4. 添加不必要的类型而不是使用可选字段
例子
export function greet( firstName: string, lastName: string, city: string, email: string ) { // Do something... }
为什么不好❌
无法扩展:添加新字段需要创建多个新类型
使类型检查更加复杂,需要额外的类型保护
导致类型名称混乱且维护更加困难
该怎么办 ✅
使用可选字段来保持类型简单且易于维护:
// We inverted firstName and lastName, but TypeScript won't catch this greet("Curry", "Stephen", "LA", "stephen.curry@gmail.com")
5. 在不同的组件级别使属性可选
例子
disabled 属性在所有组件中都是可选的。
export function greet(params: { firstName: string; lastName: string; city: string; email: string; }) { // Do something... }
为什么不好❌
- 很容易忘记传递禁用的属性,导致部分启用的表单
该怎么办 ✅
将共享字段设为必填对于内部组件。
这将确保正确的道具传递。这对于较低级别的组件尽早发现任何疏忽尤其重要。
在上面的示例中,所有内部组件现在都需要禁用。
function getAnimalDetails(animalType: "dog" | "cat" | "cow") { switch (animalType) { case "dog": return { name: "Dog", sound: "Woof" }; case "cat": return { name: "Cat", sound: "Meow" }; case "cow": return { name: "Cow", sound: "Moo" }; default: // This ensures TypeScript catches unhandled cases ((_: never) => {})(animalType); } }
注意:如果您正在为库设计组件,我不建议这样做,因为必填字段需要更多工作。
概括
TypeScript 很棒,但没有工具 ?️ 是完美的。
避免这 5 个错误将帮助您编写更干净、更安全、更易于维护的代码。
有关更多提示,请查看我的免费电子书,101 React Tips & Tricks。
?发现错误
?本周提示
这是一个包装?.
<script> // Detect dark theme var iframe = document.getElementById('tweet-1869351983934738523-882'); if (document.body.className.includes('dark-theme')) { iframe.src = "https://platform.twitter.com/embed/Tweet.html?id=1869351983934738523&theme=dark" } </script>发表评论?分享您所犯的 Typescript 错误。<script> // Detect dark theme var iframe = document.getElementById('tweet-1869050042931449902-927'); if (document.body.className.includes('dark-theme')) { iframe.src = "https://platform.twitter.com/embed/Tweet.html?id=1869050042931449902&theme=dark" } </script>别忘了加上“???”。
如果您正在学习 React,请免费下载我的 101 React Tips & Tricks 书。
如果您喜欢这样的文章,请加入我的免费时事通讯,FrontendJoy。
如果您想要每日提示,请在 X/Twitter 或 Bluesky 上找到我。
以上是✨ TypeScript 中的广告创意的详细内容。更多信息请关注PHP中文网其他相关文章!
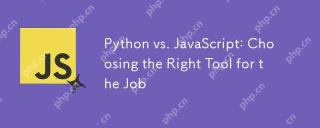
选择Python还是JavaScript取决于项目类型:1)数据科学和自动化任务选择Python;2)前端和全栈开发选择JavaScript。Python因其在数据处理和自动化方面的强大库而备受青睐,而JavaScript则因其在网页交互和全栈开发中的优势而不可或缺。
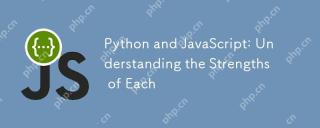
Python和JavaScript各有优势,选择取决于项目需求和个人偏好。1.Python易学,语法简洁,适用于数据科学和后端开发,但执行速度较慢。2.JavaScript在前端开发中无处不在,异步编程能力强,Node.js使其适用于全栈开发,但语法可能复杂且易出错。
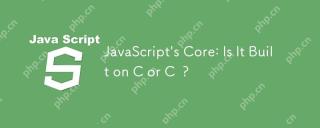
javascriptisnotbuiltoncorc; saninterpretedlanguagethatrunsonenginesoftenwritteninc.1)javascriptwasdesignedAsalightweight,解释edganguageforwebbrowsers.2)Enginesevolvedfromsimpleterterterpretpreterterterpretertestojitcompilerers,典型地提示。
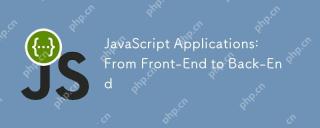
JavaScript可用于前端和后端开发。前端通过DOM操作增强用户体验,后端通过Node.js处理服务器任务。1.前端示例:改变网页文本内容。2.后端示例:创建Node.js服务器。
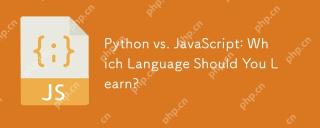
选择Python还是JavaScript应基于职业发展、学习曲线和生态系统:1)职业发展:Python适合数据科学和后端开发,JavaScript适合前端和全栈开发。2)学习曲线:Python语法简洁,适合初学者;JavaScript语法灵活。3)生态系统:Python有丰富的科学计算库,JavaScript有强大的前端框架。
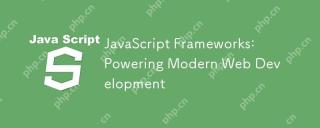
JavaScript框架的强大之处在于简化开发、提升用户体验和应用性能。选择框架时应考虑:1.项目规模和复杂度,2.团队经验,3.生态系统和社区支持。
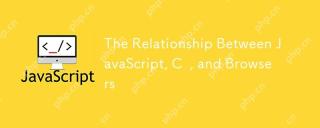
引言我知道你可能会觉得奇怪,JavaScript、C 和浏览器之间到底有什么关系?它们之间看似毫无关联,但实际上,它们在现代网络开发中扮演着非常重要的角色。今天我们就来深入探讨一下这三者之间的紧密联系。通过这篇文章,你将了解到JavaScript如何在浏览器中运行,C 在浏览器引擎中的作用,以及它们如何共同推动网页的渲染和交互。JavaScript与浏览器的关系我们都知道,JavaScript是前端开发的核心语言,它直接在浏览器中运行,让网页变得生动有趣。你是否曾经想过,为什么JavaScr
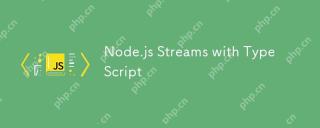
Node.js擅长于高效I/O,这在很大程度上要归功于流。 流媒体汇总处理数据,避免内存过载 - 大型文件,网络任务和实时应用程序的理想。将流与打字稿的类型安全结合起来创建POWE


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

SecLists
SecLists是最终安全测试人员的伙伴。它是一个包含各种类型列表的集合,这些列表在安全评估过程中经常使用,都在一个地方。SecLists通过方便地提供安全测试人员可能需要的所有列表,帮助提高安全测试的效率和生产力。列表类型包括用户名、密码、URL、模糊测试有效载荷、敏感数据模式、Web shell等等。测试人员只需将此存储库拉到新的测试机上,他就可以访问到所需的每种类型的列表。

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。

SublimeText3 Linux新版
SublimeText3 Linux最新版

SublimeText3 英文版
推荐:为Win版本,支持代码提示!

Atom编辑器mac版下载
最流行的的开源编辑器