现代应用程序需要可扩展性、可靠性和可维护性。在本指南中,我们将探索如何设计和实现微服务架构,以应对现实世界的挑战,同时保持卓越的运营。
基础:服务设计原则
让我们从指导我们架构的核心原则开始:
graph TD A[Service Design Principles] --> B[Single Responsibility] A --> C[Domain-Driven Design] A --> D[API First] A --> E[Event-Driven] A --> F[Infrastructure as Code]
建立弹性服务
这是使用 Go 构建结构良好的微服务的示例:
package main import ( "context" "log" "net/http" "os" "os/signal" "syscall" "time" "github.com/prometheus/client_golang/prometheus" "go.opentelemetry.io/otel" ) // Service configuration type Config struct { Port string ShutdownTimeout time.Duration DatabaseURL string } // Service represents our microservice type Service struct { server *http.Server logger *log.Logger config Config metrics *Metrics } // Metrics for monitoring type Metrics struct { requestDuration *prometheus.HistogramVec requestCount *prometheus.CounterVec errorCount *prometheus.CounterVec } func NewService(cfg Config) *Service { metrics := initializeMetrics() logger := initializeLogger() return &Service{ config: cfg, logger: logger, metrics: metrics, } } func (s *Service) Start() error { // Initialize OpenTelemetry shutdown := initializeTracing() defer shutdown() // Setup HTTP server router := s.setupRoutes() s.server = &http.Server{ Addr: ":" + s.config.Port, Handler: router, } // Graceful shutdown go s.handleShutdown() s.logger.Printf("Starting server on port %s", s.config.Port) return s.server.ListenAndServe() }
实施断路器
保护您的服务免受级联故障的影响:
type CircuitBreaker struct { failureThreshold uint32 resetTimeout time.Duration state uint32 failures uint32 lastFailure time.Time } func NewCircuitBreaker(threshold uint32, timeout time.Duration) *CircuitBreaker { return &CircuitBreaker{ failureThreshold: threshold, resetTimeout: timeout, } } func (cb *CircuitBreaker) Execute(fn func() error) error { if !cb.canExecute() { return errors.New("circuit breaker is open") } err := fn() if err != nil { cb.recordFailure() return err } cb.reset() return nil }
事件驱动的沟通
使用 Apache Kafka 进行可靠的事件流:
type EventProcessor struct { consumer *kafka.Consumer producer *kafka.Producer logger *log.Logger } func (ep *EventProcessor) ProcessEvents(ctx context.Context) error { for { select { case <h2> 基础设施即代码 </h2> <p>使用 Terraform 进行基础设施管理:<br> </p> <pre class="brush:php;toolbar:false"># Define the microservice infrastructure module "microservice" { source = "./modules/microservice" name = "user-service" container_port = 8080 replicas = 3 environment = { KAFKA_BROKERS = var.kafka_brokers DATABASE_URL = var.database_url LOG_LEVEL = "info" } # Configure auto-scaling autoscaling = { min_replicas = 2 max_replicas = 10 metrics = [ { type = "Resource" resource = { name = "cpu" target_average_utilization = 70 } } ] } } # Set up monitoring module "monitoring" { source = "./modules/monitoring" service_name = module.microservice.name alert_email = var.alert_email dashboard = { refresh_interval = "30s" time_range = "6h" } }
使用 OpenAPI 进行 API 设计
定义您的服务 API 合约:
openapi: 3.0.3 info: title: User Service API version: 1.0.0 description: User management microservice API paths: /users: post: summary: Create a new user operationId: createUser requestBody: required: true content: application/json: schema: $ref: '#/components/schemas/CreateUserRequest' responses: '201': description: User created successfully content: application/json: schema: $ref: '#/components/schemas/User' '400': $ref: '#/components/responses/BadRequest' '500': $ref: '#/components/responses/InternalError' components: schemas: User: type: object properties: id: type: string format: uuid email: type: string format: email created_at: type: string format: date-time required: - id - email - created_at
实施可观察性
设置全面监控:
# Prometheus configuration scrape_configs: - job_name: 'microservices' kubernetes_sd_configs: - role: pod relabel_configs: - source_labels: [__meta_kubernetes_pod_annotation_prometheus_io_scrape] action: keep regex: true # Grafana dashboard { "dashboard": { "panels": [ { "title": "Request Rate", "type": "graph", "datasource": "Prometheus", "targets": [ { "expr": "rate(http_requests_total{service=\"user-service\"}[5m])", "legendFormat": "{{method}} {{path}}" } ] }, { "title": "Error Rate", "type": "graph", "datasource": "Prometheus", "targets": [ { "expr": "rate(http_errors_total{service=\"user-service\"}[5m])", "legendFormat": "{{status_code}}" } ] } ] } }
部署策略
实施零停机部署:
apiVersion: apps/v1 kind: Deployment metadata: name: user-service spec: replicas: 3 strategy: type: RollingUpdate rollingUpdate: maxSurge: 1 maxUnavailable: 0 template: spec: containers: - name: user-service image: user-service:1.0.0 ports: - containerPort: 8080 readinessProbe: httpGet: path: /health port: 8080 initialDelaySeconds: 5 periodSeconds: 10 livenessProbe: httpGet: path: /health port: 8080 initialDelaySeconds: 15 periodSeconds: 20
生产最佳实践
- 实施适当的健康检查和就绪探测
- 使用带有相关 ID 的结构化日志记录
- 通过指数退避实施适当的重试策略
- 使用断路器进行外部依赖
- 实施适当的速率限制
- 监控关键指标并发出警报
- 使用适当的秘密管理
- 实施适当的备份和灾难恢复
结论
构建弹性微服务需要仔细考虑许多因素。关键是:
- 为失败而设计
- 实现适当的可观察性
- 使用基础设施即代码
- 实施正确的测试策略
- 使用正确的部署策略
- 有效监控和警报
您在构建微服务时遇到了哪些挑战?在下面的评论中分享您的经验!
以上是设计弹性微服务:云架构实用指南的详细内容。更多信息请关注PHP中文网其他相关文章!
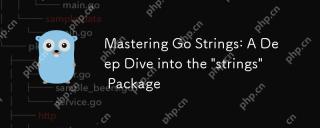
你应该关心Go语言中的"strings"包,因为它提供了处理文本数据的工具,从基本的字符串拼接到高级的正则表达式匹配。1)"strings"包提供了高效的字符串操作,如Join函数用于拼接字符串,避免性能问题。2)它包含高级功能,如ContainsAny函数,用于检查字符串是否包含特定字符集。3)Replace函数用于替换字符串中的子串,需注意替换顺序和大小写敏感性。4)Split函数可以根据分隔符拆分字符串,常用于正则表达式处理。5)使用时需考虑性能,如
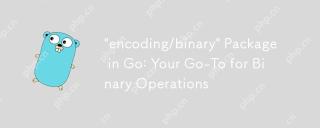
“编码/二进制”软件包interingoisentialForHandlingBinaryData,oferingToolSforreDingingAndWritingBinaryDataEfficely.1)Itsupportsbothlittle-endianandBig-endianBig-endianbyteorders,CompialforOss-System-System-System-compatibility.2)
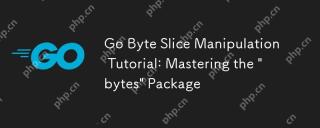
掌握Go语言中的bytes包有助于提高代码的效率和优雅性。1)bytes包对于解析二进制数据、处理网络协议和内存管理至关重要。2)使用bytes.Buffer可以逐步构建字节切片。3)bytes包提供了搜索、替换和分割字节切片的功能。4)bytes.Reader类型适用于从字节切片读取数据,特别是在I/O操作中。5)bytes包与Go的垃圾回收器协同工作,提高了大数据处理的效率。
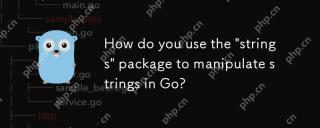
你可以使用Go语言中的"strings"包来操纵字符串。1)使用strings.TrimSpace去除字符串两端的空白字符。2)用strings.Split将字符串按指定分隔符拆分成切片。3)通过strings.Join将字符串切片合并成一个字符串。4)用strings.Contains检查字符串是否包含特定子串。5)利用strings.ReplaceAll进行全局替换。注意使用时要考虑性能和潜在的陷阱。
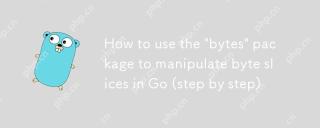
ThebytespackageinGoishighlyeffectiveforbyteslicemanipulation,offeringfunctionsforsearching,splitting,joining,andbuffering.1)Usebytes.Containstosearchforbytesequences.2)bytes.Splithelpsbreakdownbyteslicesusingdelimiters.3)bytes.Joinreconstructsbytesli
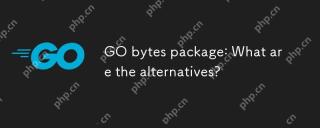
thealternativestogo'sbytespackageincageincludethestringspackage,bufiopackage和customstructs.1)thestringspackagecanbeusedforbytemanipulationforbytemanipulationbybyconvertingbytestostostostostostrings.2))
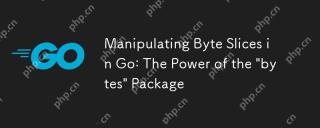
“字节”包装封装forefforeflyManipulatingByteslices,CocialforbinaryData,网络交易和andfilei/o.itoffersfunctionslikeIndexForsearching,BufferForhandLinglaRgedLargedLargedAtaTasets,ReaderForsimulatingStreamReadReadImreAmreadReamReadinging,以及Joineffiter和Joineffiter和Joineffore
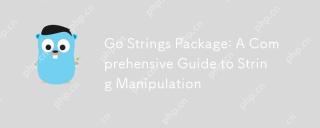
go'sstringspackageIscialforficientficientsTringManipulation,uperingToolSlikestrings.split(),strings.join(),strings.replaceall(),andStrings.contains.contains.contains.contains.contains.contains.split.split(split()strings.split()dividesStringoSubSubStrings; 2)strings.joins.joins.joinsillise.joinsinelline joinsiline joinsinelline; 3);


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

SecLists
SecLists是最终安全测试人员的伙伴。它是一个包含各种类型列表的集合,这些列表在安全评估过程中经常使用,都在一个地方。SecLists通过方便地提供安全测试人员可能需要的所有列表,帮助提高安全测试的效率和生产力。列表类型包括用户名、密码、URL、模糊测试有效载荷、敏感数据模式、Web shell等等。测试人员只需将此存储库拉到新的测试机上,他就可以访问到所需的每种类型的列表。
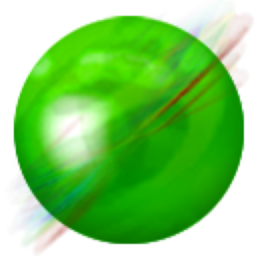
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。

SublimeText3 Linux新版
SublimeText3 Linux最新版