在本指南创建内存缓存的基础上,我们将进一步介绍可配置的数据持久性。通过利用适配器和策略模式,我们将设计一个可扩展的系统,将存储机制与缓存逻辑分离,从而允许根据需要无缝集成数据库或服务。
愿景:像 ORM 一样解耦
目标是使缓存可扩展而不改变其核心逻辑。受 ORM 系统的启发,我们的方法涉及共享 API 抽象。这使得存储(例如 localStorage、IndexedDB 甚至远程数据库)能够以最少的代码更改互换工作。
存储适配器基类
这是为任何持久性系统定义 API 的抽象类:
export abstract class StorageAdapter { abstract connect(): Promise<void>; abstract add(key: string, value: unknown): Promise<void>; abstract get(key: string): Promise<unknown null>; abstract getAll(): Promise<record unknown>>; abstract delete(key: string): Promise<void>; abstract clear(): Promise<void>; } </void></void></record></unknown></void></void>
任何存储解决方案都必须扩展此基类,以确保交互的一致性。例如,这是 IndexedDB 的实现:
示例:IndexedDB 适配器
此适配器实现 StorageAdapter 接口以将缓存数据保存在 IndexedDB 存储中。
import { StorageAdapter } from './storage_adapter'; /** * IndexedDBAdapter is an implementation of the StorageAdapter * interface designed to provide a persistent storage mechanism * using IndexedDB. This adapter can be reused for other cache * implementations or extended for similar use cases, ensuring * flexibility and scalability. */ export class IndexedDBAdapter extends StorageAdapter { private readonly dbName: string; private readonly storeName: string; private db: IDBDatabase | null = null; /** * Initializes the adapter with the specified database and store * names. Defaults are provided to make it easy to set up without * additional configuration. */ constructor(dbName: string = 'cacheDB', storeName: string = 'cacheStore') { super(); this.dbName = dbName; this.storeName = storeName; } /** * Connects to the IndexedDB database and initializes it if * necessary. This asynchronous method ensures that the database * and object store are available before any other operations. * It uses the `onupgradeneeded` event to handle schema creation * or updates, making it a robust solution for versioning. */ async connect(): Promise<void> { return await new Promise((resolve, reject) => { const request = indexedDB.open(this.dbName, 1); request.onupgradeneeded = (event) => { const db = (event.target as IDBOpenDBRequest).result; if (!db.objectStoreNames.contains(this.storeName)) { db.createObjectStore(this.storeName, { keyPath: 'key' }); } }; request.onsuccess = (event) => { this.db = (event.target as IDBOpenDBRequest).result; resolve(); }; request.onerror = () => reject(request.error); }); } /** * Adds or updates a key-value pair in the store. This method is * asynchronous to ensure compatibility with the non-blocking * nature of IndexedDB and to prevent UI thread blocking. Using * the `put` method ensures idempotency: the operation will * insert or replace the entry. */ async add(key: string, value: unknown): Promise<void> { await this._withTransaction('readwrite', (store) => store.put({ key, value })); } /** * Retrieves the value associated with a key. If the key does not * exist, null is returned. This method is designed to integrate * seamlessly with caching mechanisms, enabling fast lookups. */ async get(key: string): Promise<unknown null> { return await this._withTransaction('readonly', (store) => this._promisifyRequest(store.get(key)).then((result) => result ? (result as { key: string; value: unknown }).value : null ) ); } /** * Fetches all key-value pairs from the store. Returns an object * mapping keys to their values, making it suitable for bulk * operations or syncing with in-memory caches. */ async getAll(): Promise<record unknown>> { return await this._withTransaction('readonly', (store) => this._promisifyRequest(store.getAll()).then((results) => results.reduce((acc: Record<string unknown>, item: { key: string; value: unknown }) => { acc[item.key] = item.value; return acc; }, {}) ) ); } /** * Deletes a key-value pair by its key. This method is crucial * for managing cache size and removing expired entries. The * `readwrite` mode is used to ensure proper deletion. */ async delete(key: string): Promise<void> { await this._withTransaction('readwrite', (store) => store.delete(key)); } /** * Clears all entries from the store. This method is ideal for * scenarios where the entire cache needs to be invalidated, such * as during application updates or environment resets. */ async clear(): Promise<void> { await this._withTransaction('readwrite', (store) => store.clear()); } /** * Handles transactions in a reusable way. Ensures the database * is connected and abstracts the transaction logic. By * centralizing transaction handling, this method reduces * boilerplate code and ensures consistency across all operations. */ private async _withTransaction<t>( mode: IDBTransactionMode, callback: (store: IDBObjectStore) => IDBRequest | Promise<t> ): Promise<t> { if (!this.db) throw new Error('IndexedDB is not connected'); const transaction = this.db.transaction([this.storeName], mode); const store = transaction.objectStore(this.storeName); const result = callback(store); return result instanceof IDBRequest ? await this._promisifyRequest(result) : await result; } /** * Converts IndexedDB request events into Promises, allowing for * cleaner and more modern asynchronous handling. This is * essential for making IndexedDB operations fit seamlessly into * the Promise-based architecture of JavaScript applications. */ private async _promisifyRequest<t>(request: IDBRequest): Promise<t> { return await new Promise((resolve, reject) => { request.onsuccess = () => resolve(request.result as T); request.onerror = () => reject(request.error); }); } } </t></t></t></t></t></void></void></string></record></unknown></void></void>
将适配器集成到缓存中
缓存接受可选的 StorageAdapter。如果提供,它会初始化数据库连接,将数据加载到内存中,并使缓存和存储保持同步。
private constructor(capacity: number, storageAdapter?: StorageAdapter) { this.capacity = capacity; this.storageAdapter = storageAdapter; if (this.storageAdapter) { this.storageAdapter.connect().catch((error) => { throw new Error(error); }); this.storageAdapter.getAll().then((data) => { for (const key in data) { this.put(key, data[key] as T); } }).catch((error) => { throw new Error(error); }); } this.hash = new Map(); this.head = this.tail = undefined; this.hitCount = this.missCount = this.evictionCount = 0; }
为什么选择适配器和策略模式?
使用适配器模式:
- 将缓存与特定存储机制解耦。 确保新存储后端的
- 可扩展性。
- 启用持久层的运行时选择。
- 通过模拟不同的适配器来简化测试。
- 抽象 API: 使缓存逻辑与存储详细信息无关。
- 单例缓存: 确保共享状态一致性。
- 异步初始化:避免在设置过程中阻塞操作。
- 延迟加载:仅在提供存储适配器时加载持久数据。
这种设计很稳健,但仍有增强的空间:
- 优化同步逻辑以获得更好的性能。
- 使用 Redis 或 SQLite 等其他适配器进行实验。
尝试一下! ?
如果您想测试实际缓存,可以使用 npm 包:adev-lru。您还可以在 GitHub 上探索完整的源代码:adev-lru 存储库。我欢迎任何建议、建设性反馈或贡献,以使其变得更好! ?
编码愉快! ?
以上是通过可配置的数据持久性增强 LRU 缓存的详细内容。更多信息请关注PHP中文网其他相关文章!
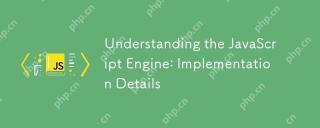
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。

Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
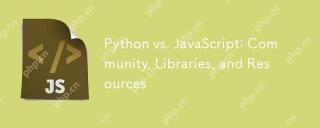
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
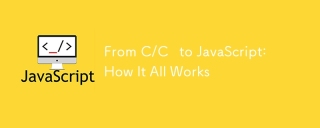
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
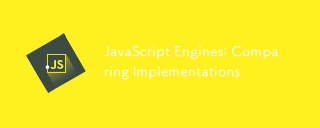
不同JavaScript引擎在解析和执行JavaScript代码时,效果会有所不同,因为每个引擎的实现原理和优化策略各有差异。1.词法分析:将源码转换为词法单元。2.语法分析:生成抽象语法树。3.优化和编译:通过JIT编译器生成机器码。4.执行:运行机器码。V8引擎通过即时编译和隐藏类优化,SpiderMonkey使用类型推断系统,导致在相同代码上的性能表现不同。
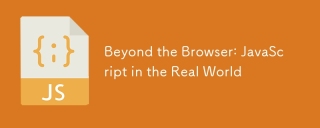
JavaScript在现实世界中的应用包括服务器端编程、移动应用开发和物联网控制:1.通过Node.js实现服务器端编程,适用于高并发请求处理。2.通过ReactNative进行移动应用开发,支持跨平台部署。3.通过Johnny-Five库用于物联网设备控制,适用于硬件交互。
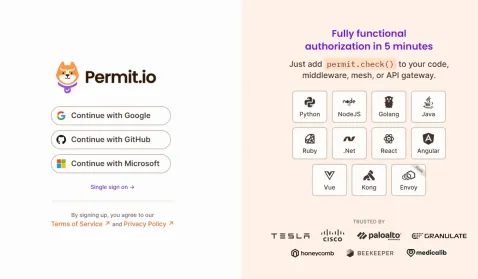
我使用您的日常技术工具构建了功能性的多租户SaaS应用程序(一个Edtech应用程序),您可以做同样的事情。 首先,什么是多租户SaaS应用程序? 多租户SaaS应用程序可让您从唱歌中为多个客户提供服务
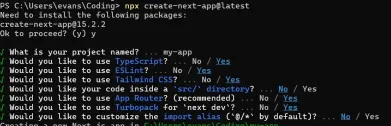
本文展示了与许可证确保的后端的前端集成,并使用Next.js构建功能性Edtech SaaS应用程序。 前端获取用户权限以控制UI的可见性并确保API要求遵守角色库


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。

禅工作室 13.0.1
功能强大的PHP集成开发环境

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。
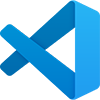
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器
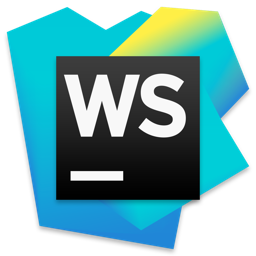
WebStorm Mac版
好用的JavaScript开发工具