React 中的 CSS-in-JS
CSS-in-JS 是一种将 CSS 样式写入 JavaScript 文件内的技术。它允许开发人员使用 JavaScript 语法编写 CSS 规则,提供更加动态和模块化的方法来设计 React 应用程序。随着基于组件的架构的兴起,这种技术越来越受欢迎,其中样式的范围仅限于单个组件而不是全局样式表。
在 React 中,CSS-in-JS 使样式能够与其所属的组件紧密联系在一起,确保样式易于维护和扩展。有几个流行的库实现了这种技术,例如 Styled Components、Emotion 和 JSS。
CSS-in-JS 的主要优点
作用域样式:CSS-in-JS 确保样式作用于各个组件,消除了全局 CSS 冲突的可能性。这使得应用程序更易于维护,因为样式在其组件内是隔离的。
动态样式:通过 CSS-in-JS,可以轻松使用 JavaScript 变量、道具和状态来根据组件逻辑动态更改样式。例如,您可以根据按钮的状态更改按钮的颜色。
基于组件的样式:样式与组件逻辑一起编写,使其更易于管理,尤其是在大型应用程序中。这允许更加模块化和可维护的代码库。
自动供应商前缀:许多 CSS-in-JS 库,例如 Styled Components 和 Emotion,会自动处理供应商前缀,确保跨浏览器兼容性。
主题:CSS-in-JS 可以更轻松地创建全局主题。您可以在顶层定义配色方案、版式和其他共享样式,并将它们动态注入到组件中。
流行的 CSS-in-JS 库
1. 样式组件
样式组件 是最流行的 CSS-in-JS 库之一。它允许您在 JavaScript 文件中编写实际的 CSS 代码,但其范围仅限于各个组件。
示例:使用样式化组件
npm install styled-components
import React from 'react'; import styled from 'styled-components'; // Create a styled component const Button = styled.button` background-color: ${(props) => (props.primary ? 'blue' : 'gray')}; color: white; padding: 10px 20px; border: none; border-radius: 5px; cursor: pointer; &:hover { opacity: 0.8; } `; const App = () => { return ( <div> <button primary>Primary Button</button> <button>Secondary Button</button> </div> ); }; export default App;
说明:
- styled.button 是 Styled Components 提供的一个函数,允许您为按钮组件定义样式。
- 样式的范围仅限于按钮组件,这意味着它不会影响页面上的任何其他按钮。
- 主要道具用于动态更改按钮的背景颜色。
2. 情感
Emotion 是另一个流行的 CSS-in-JS 库,它提供了强大的样式解决方案。它与样式组件类似,但具有一些附加功能,例如性能优化和对服务器端渲染的更好支持。
示例:使用情感
npm install @emotion/react @emotion/styled
/** @jsxImportSource @emotion/react */ import { css } from '@emotion/react'; import styled from '@emotion/styled'; const buttonStyle = (primary) => css` background-color: ${primary ? 'blue' : 'gray'}; color: white; padding: 10px 20px; border: none; border-radius: 5px; cursor: pointer; &:hover { opacity: 0.8; } `; const Button = styled.button` ${props => buttonStyle(props.primary)} `; const App = () => { return ( <div> <button primary>Primary Button</button> <button>Secondary Button</button> </div> ); }; export default App;
说明:
- Emotion 中的 css 函数用于创建样式定义,可以通过 styled API 将其应用于组件。
- Emotion 允许您使用模板文字和 JavaScript 对象编写样式。
3. JSS(JavaScript 样式表)
JSS 是另一个 CSS-in-JS 库,它允许使用 JavaScript 来编写 CSS。它通过自定义主题和更高级的样式逻辑等功能提供对样式的更精细的控制。
示例:使用 JSS
npm install jss react-jss
import React from 'react'; import { createUseStyles } from 'react-jss'; const useStyles = createUseStyles({ button: { backgroundColor: (props) => (props.primary ? 'blue' : 'gray'), color: 'white', padding: '10px 20px', border: 'none', borderRadius: '5px', cursor: 'pointer', '&:hover': { opacity: 0.8, }, }, }); const Button = (props) => { const classes = useStyles(props); return <button classname="{classes.button}">{props.children}</button>; }; const App = () => { return ( <div> <button primary>Primary Button</button> <button>Secondary Button</button> </div> ); }; export default App;
说明:
- createUseStyles是react-jss提供的一个生成样式的hook。
- useStyles 钩子接收 props 并返回按钮的类名。
- 此方法还允许基于传递给组件的 props 进行动态样式。
CSS-in-JS 的挑战
虽然 CSS-in-JS 有很多优点,但它也面临着一系列挑战:
- 性能开销:CSS-in-JS 库可能会带来性能开销,特别是对于具有多种动态样式的大型应用程序。
- 增加捆绑包大小:由于样式与 JavaScript 代码捆绑在一起,因此可能会增加 JavaScript 捆绑包的大小。
- 学习曲线:对于习惯传统 CSS 或基于预处理器的工作流程(如 Sass)的开发人员来说,过渡到 CSS-in-JS 可能需要一个学习曲线。
- 关注点分离:一些开发者认为将样式和逻辑组合在同一个文件中违反了关注点分离原则。
CSS-in-JS 的最佳实践
- 使用主题:利用 CSS-in-JS 库提供的主题功能来管理应用程序的全局样式(例如颜色、字体)。
- 保持样式作用域:使用 styled-components 或 JSS 将样式保持在其所属组件的作用域内,防止全局样式相互干扰。
- 限制动态样式:谨慎使用动态样式,因为过多的动态样式可能会对性能产生负面影响。
- 使用适当的工具:像Babel这样的工具可以帮助优化CSS-in-JS库的性能,例如通过缩小生成的CSS。
结论
CSS-in-JS 是一种设计 React 应用程序的现代方法,它将 JavaScript 和 CSS 的力量结合在一起。通过使用 Styled Components、Emotion 或 JSS 等库,您可以在 JavaScript 文件中编写样式,从而提高代码库的模块化、性能和可维护性。然而,平衡 CSS-in-JS 的使用和潜在的性能考虑非常重要,特别是在大型应用程序中。
以上是CSS-in-JS:React 应用程序的现代样式的详细内容。更多信息请关注PHP中文网其他相关文章!
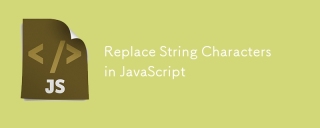
JavaScript字符串替换方法详解及常见问题解答 本文将探讨两种在JavaScript中替换字符串字符的方法:在JavaScript代码内部替换和在网页HTML内部替换。 在JavaScript代码内部替换字符串 最直接的方法是使用replace()方法: str = str.replace("find","replace"); 该方法仅替换第一个匹配项。要替换所有匹配项,需使用正则表达式并添加全局标志g: str = str.replace(/fi
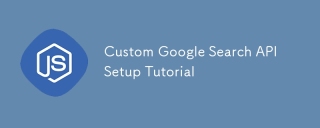
本教程向您展示了如何将自定义的Google搜索API集成到您的博客或网站中,提供了比标准WordPress主题搜索功能更精致的搜索体验。 令人惊讶的是简单!您将能够将搜索限制为Y
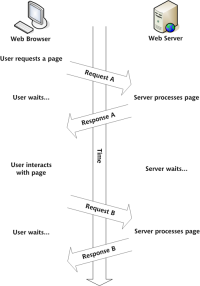
因此,在这里,您准备好了解所有称为Ajax的东西。但是,到底是什么? AJAX一词是指用于创建动态,交互式Web内容的一系列宽松的技术。 Ajax一词,最初由Jesse J创造
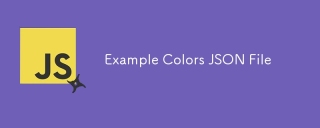
本文系列在2017年中期进行了最新信息和新示例。 在此JSON示例中,我们将研究如何使用JSON格式将简单值存储在文件中。 使用键值对符号,我们可以存储任何类型的
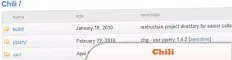
增强您的代码演示:开发人员的10个语法荧光笔 在您的网站或博客上共享代码片段是开发人员的常见实践。 选择合适的语法荧光笔可以显着提高可读性和视觉吸引力。 t
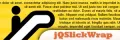
利用轻松的网页布局:8个基本插件 jQuery大大简化了网页布局。 本文重点介绍了简化该过程的八个功能强大的JQuery插件,对于手动网站创建特别有用
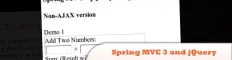
本文介绍了关于JavaScript和JQuery模型视图控制器(MVC)框架的10多个教程的精选选择,非常适合在新的一年中提高您的网络开发技能。 这些教程涵盖了来自Foundatio的一系列主题
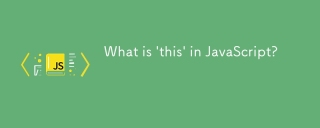
核心要点 JavaScript 中的 this 通常指代“拥有”该方法的对象,但具体取决于函数的调用方式。 没有当前对象时,this 指代全局对象。在 Web 浏览器中,它由 window 表示。 调用函数时,this 保持全局对象;但调用对象构造函数或其任何方法时,this 指代对象的实例。 可以使用 call()、apply() 和 bind() 等方法更改 this 的上下文。这些方法使用给定的 this 值和参数调用函数。 JavaScript 是一门优秀的编程语言。几年前,这句话可


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具
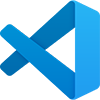
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器

SublimeText3 Linux新版
SublimeText3 Linux最新版

记事本++7.3.1
好用且免费的代码编辑器
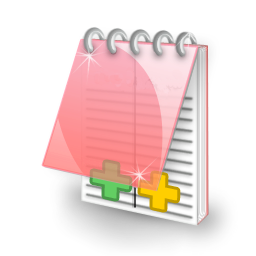
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

禅工作室 13.0.1
功能强大的PHP集成开发环境