JavaScript 中的 DOM 操作
DOM 操作 是现代 Web 开发的一个基本方面,它允许开发人员动态修改网页的内容、结构和样式。文档对象模型 (DOM) 以树状格式表示网页的 HTML 结构。
1.什么是 DOM?
DOM 是浏览器提供的接口,允许 JavaScript 与 HTML 和 CSS 交互。它将页面表示为节点树,其中每个元素、属性或文本都是一个节点。
例子:
对于以下 HTML:
<div> <p>The DOM structure looks like this:</p> <ul> <li>Document <ul> <li>HTML</li> <li>Body <ul> <li>Div (id="container")</li> <li>Paragraph ("Hello, World!")</li> </ul> </li> </ul> </li> </ul> <hr> <h3> <strong>2. Selecting DOM Elements</strong> </h3> <h4> <strong>A. Using getElementById</strong> </h4> <p>Select an element by its ID.<br> </p> <pre class="brush:php;toolbar:false">const container = document.getElementById("container"); console.log(container); // Output: <div> <h4> <strong>B. Using querySelector</strong> </h4> <p>Select the first matching element.<br> </p> <pre class="brush:php;toolbar:false">const paragraph = document.querySelector("p"); console.log(paragraph); // Output: <p>Hello, World!</p>
C.使用 querySelectorAll
选择所有匹配的元素作为 NodeList。
const allParagraphs = document.querySelectorAll("p"); console.log(allParagraphs); // Output: NodeList of <p> elements </p>
3.修改 DOM 元素
A.更改内容
使用textContent或innerHTML属性来修改内容。
const paragraph = document.querySelector("p"); paragraph.textContent = "Hello, JavaScript!"; // Changes <p>Hello, World!</p> to <p>Hello, JavaScript!</p>
B.更改属性
使用 setAttribute 或直接属性赋值。
const image = document.querySelector("img"); image.setAttribute("src", "new-image.jpg"); image.alt = "A descriptive text";
C.改变风格
修改样式属性以应用内联样式。
const container = document.getElementById("container"); container.style.backgroundColor = "lightblue"; container.style.padding = "20px";
4.添加和删除元素
A.创建新元素
使用createElement方法。
const newParagraph = document.createElement("p"); newParagraph.textContent = "This is a new paragraph."; document.body.appendChild(newParagraph);
B.删除元素
使用remove方法或removeChild。
const paragraph = document.querySelector("p"); paragraph.remove(); // Removes the paragraph from the DOM
5.向元素添加事件监听器
您可以使用事件侦听器向元素添加交互性。
const button = document.createElement("button"); button.textContent = "Click Me"; button.addEventListener("click", function() { alert("Button clicked!"); }); document.body.appendChild(button);
6.遍历 DOM
浏览父元素、子元素和兄弟元素。
A.家长和孩子
const child = document.querySelector("p"); const parent = child.parentElement; console.log(parent); // Output: Parent element of <p> const children = parent.children; console.log(children); // Output: HTMLCollection of child elements </p>
B.兄弟姐妹
const sibling = child.nextElementSibling; console.log(sibling); // Output: Next sibling element
7.性能优化
- 使用 documentFragment 进行批量更新: 通过对 DOM 更新进行分组,最大限度地减少回流和重绘。
const fragment = document.createDocumentFragment(); for (let i = 0; i
最小化直接 DOM 操作:
缓存元素并批量修改。使用虚拟 DOM 库:
对于复杂的应用程序,请考虑像 React 或 Vue 这样的库。
8.实际示例:待办事项列表
<div> <p>The DOM structure looks like this:</p> <ul> <li>Document <ul> <li>HTML</li> <li>Body <ul> <li>Div (id="container")</li> <li>Paragraph ("Hello, World!")</li> </ul> </li> </ul> </li> </ul> <hr> <h3> <strong>2. Selecting DOM Elements</strong> </h3> <h4> <strong>A. Using getElementById</strong> </h4> <p>Select an element by its ID.<br> </p> <pre class="brush:php;toolbar:false">const container = document.getElementById("container"); console.log(container); // Output: <div> <h4> <strong>B. Using querySelector</strong> </h4> <p>Select the first matching element.<br> </p> <pre class="brush:php;toolbar:false">const paragraph = document.querySelector("p"); console.log(paragraph); // Output: <p>Hello, World!</p>
9.总结
- DOM Manipulation 允许开发者动态修改网页。
- 使用 getElementById、querySelector 和 createElement 等方法进行有效操作。
- 最小化直接 DOM 操作以获得更好的性能。
掌握 DOM 操作对于创建动态、交互式和用户友好的 Web 应用程序至关重要。
嗨,我是 Abhay Singh Kathayat!
我是一名全栈开发人员,拥有前端和后端技术方面的专业知识。我使用各种编程语言和框架来构建高效、可扩展且用户友好的应用程序。
请随时通过我的商务电子邮件与我联系:kaashshorts28@gmail.com。
以上是掌握 JavaScript 中的 DOM 操作:综合指南的详细内容。更多信息请关注PHP中文网其他相关文章!
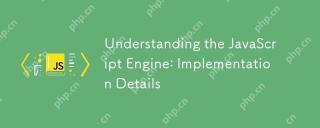
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。

Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
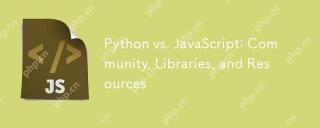
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
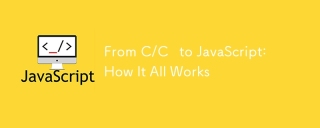
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
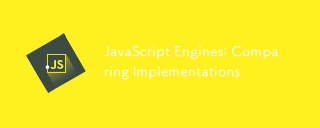
不同JavaScript引擎在解析和执行JavaScript代码时,效果会有所不同,因为每个引擎的实现原理和优化策略各有差异。1.词法分析:将源码转换为词法单元。2.语法分析:生成抽象语法树。3.优化和编译:通过JIT编译器生成机器码。4.执行:运行机器码。V8引擎通过即时编译和隐藏类优化,SpiderMonkey使用类型推断系统,导致在相同代码上的性能表现不同。
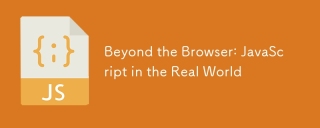
JavaScript在现实世界中的应用包括服务器端编程、移动应用开发和物联网控制:1.通过Node.js实现服务器端编程,适用于高并发请求处理。2.通过ReactNative进行移动应用开发,支持跨平台部署。3.通过Johnny-Five库用于物联网设备控制,适用于硬件交互。
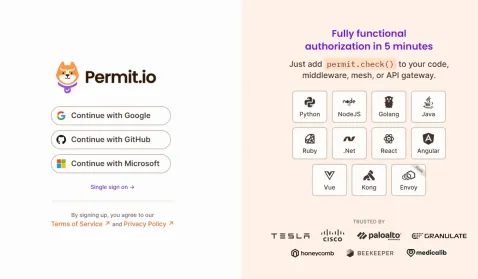
我使用您的日常技术工具构建了功能性的多租户SaaS应用程序(一个Edtech应用程序),您可以做同样的事情。 首先,什么是多租户SaaS应用程序? 多租户SaaS应用程序可让您从唱歌中为多个客户提供服务
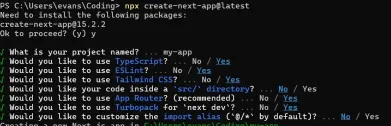
本文展示了与许可证确保的后端的前端集成,并使用Next.js构建功能性Edtech SaaS应用程序。 前端获取用户权限以控制UI的可见性并确保API要求遵守角色库


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。
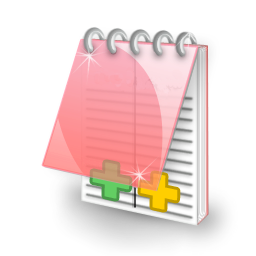
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

SublimeText3汉化版
中文版,非常好用

SublimeText3 Linux新版
SublimeText3 Linux最新版

禅工作室 13.0.1
功能强大的PHP集成开发环境