状态管理是构建动态且可扩展的 React 应用程序的一个重要方面。虽然 React 提供了用于管理本地状态的强大工具,但随着应用程序变得越来越复杂,开发人员通常需要先进的解决方案来有效地处理全局和共享状态。在本文中,我们将探索 React 中的状态管理,重点关注 Context API 等内置选项和 Redux 等外部库。
React 中的状态管理是什么?
React 中的状态是指决定组件行为和渲染的数据。有效管理这些数据是维持可预测和无缝用户体验的关键。
React 通过 useState 和 useReducer 等钩子提供本地状态管理。然而,随着应用程序规模的扩大,诸如道具钻取(通过多个组件传递道具)和跨应用程序同步共享状态等挑战需要强大的状态管理解决方案。
React 内置的状态管理工具
1。 useState
useState 钩子是 Reactjs 管理功能组件中本地状态的最简单方法。它非常适合管理小型的、特定于组件的状态。
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onclick="{()"> setCount(count + 1)}>Increment</button> </div> ); }
2。使用Reducer
对于涉及多个状态转换的更复杂的状态逻辑,useReducer 是一个很好的选择。它通常被视为本地状态管理 Redux 的轻量级替代品。
import React, { useReducer } from 'react'; const reducer = (state, action) => { switch (action.type) { case 'increment': return { count: state.count + 1 }; case 'decrement': return { count: state.count - 1 }; default: return state; } }; function Counter() { const [state, dispatch] = useReducer(reducer, { count: 0 }); return ( <div> <p>Count: {state.count}</p> <button onclick="{()"> dispatch({ type: 'increment' })}>Increment</button> <button onclick="{()"> dispatch({ type: 'decrement' })}>Decrement</button> </div> ); }
3。上下文 API
Context API 允许您在整个组件树中全局共享状态,从而消除了 prop-drilling 的需要。
示例:使用 Context API 管理主题
import React, { createContext, useContext, useState } from 'react'; const ThemeContext = createContext(); function App() { const [theme, setTheme] = useState('light'); return ( <themecontext.provider value="{{" theme settheme> <header></header> </themecontext.provider> ); } function Header() { const { theme, setTheme } = useContext(ThemeContext); return ( <div> <p>While powerful, the Context API may not be the best choice for highly dynamic or large-scale applications due to performance concerns.</p> <p><strong>Redux: A Popular State Management Library</strong></p> <p><strong>What is Redux?</strong><br> Redux is a predictable state management library that helps manage global state. It uses a single store for the entire application and updates state via actions and reducers, ensuring a predictable state flow.</p> <p><strong>Key Concepts in Redux</strong></p> <ul> <li>Store: Centralized state container.</li> <li>Actions: Objects describing state changes.</li> <li>Reducers: Pure functions that specify how the state changes.</li> <li>Middleware: Handles side effects like API calls.</li> </ul> <p>Example: Simple Redux Flow<br> </p> <pre class="brush:php;toolbar:false">import { createStore } from 'redux'; // Reducer const counterReducer = (state = { count: 0 }, action) => { switch (action.type) { case 'increment': return { count: state.count + 1 }; case 'decrement': return { count: state.count - 1 }; default: return state; } }; // Store const store = createStore(counterReducer); // Dispatch Actions store.dispatch({ type: 'increment' }); console.log(store.getState()); // { count: 1 }
Redux 非常适合具有复杂状态逻辑的应用程序,但它的样板对于较小的项目来说可能是一个缺点。
何时使用每种解决方案
useState:最适合管理本地、简单的状态。
useReducer:非常适合单个组件内的复杂状态逻辑。
Context API:对于在较小的应用程序中全局共享状态很有用。
Redux:非常适合需要结构化和可预测状态管理的大型应用程序。
结论
状态管理对于构建可维护和可扩展的 React 应用程序至关重要。虽然 Reactjs 内置工具对于较小的应用程序来说已经足够了,但随着应用程序复杂性的增加,像 Redux 这样的库就变得不可或缺。了解每种方法的优势和用例可确保您为您的项目选择正确的解决方案。
您在 React 应用程序中更喜欢哪种状态管理解决方案?请在评论中告诉我们!
以上是React 中状态管理的作用:Redux、Context API 等指南的详细内容。更多信息请关注PHP中文网其他相关文章!
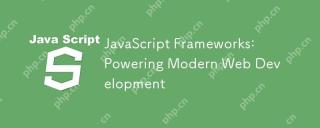
JavaScript框架的强大之处在于简化开发、提升用户体验和应用性能。选择框架时应考虑:1.项目规模和复杂度,2.团队经验,3.生态系统和社区支持。
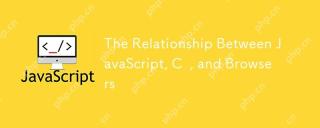
引言我知道你可能会觉得奇怪,JavaScript、C 和浏览器之间到底有什么关系?它们之间看似毫无关联,但实际上,它们在现代网络开发中扮演着非常重要的角色。今天我们就来深入探讨一下这三者之间的紧密联系。通过这篇文章,你将了解到JavaScript如何在浏览器中运行,C 在浏览器引擎中的作用,以及它们如何共同推动网页的渲染和交互。JavaScript与浏览器的关系我们都知道,JavaScript是前端开发的核心语言,它直接在浏览器中运行,让网页变得生动有趣。你是否曾经想过,为什么JavaScr
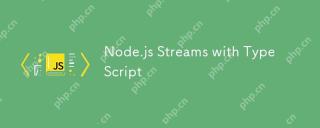
Node.js擅长于高效I/O,这在很大程度上要归功于流。 流媒体汇总处理数据,避免内存过载 - 大型文件,网络任务和实时应用程序的理想。将流与打字稿的类型安全结合起来创建POWE
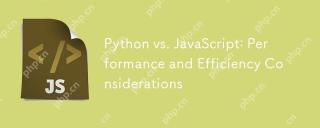
Python和JavaScript在性能和效率方面的差异主要体现在:1)Python作为解释型语言,运行速度较慢,但开发效率高,适合快速原型开发;2)JavaScript在浏览器中受限于单线程,但在Node.js中可利用多线程和异步I/O提升性能,两者在实际项目中各有优势。
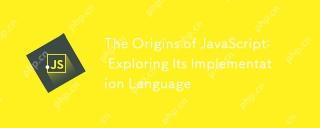
JavaScript起源于1995年,由布兰登·艾克创造,实现语言为C语言。1.C语言为JavaScript提供了高性能和系统级编程能力。2.JavaScript的内存管理和性能优化依赖于C语言。3.C语言的跨平台特性帮助JavaScript在不同操作系统上高效运行。
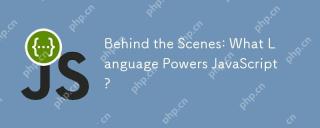
JavaScript在浏览器和Node.js环境中运行,依赖JavaScript引擎解析和执行代码。1)解析阶段生成抽象语法树(AST);2)编译阶段将AST转换为字节码或机器码;3)执行阶段执行编译后的代码。
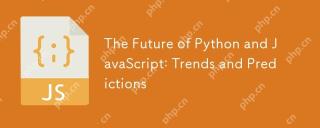
Python和JavaScript的未来趋势包括:1.Python将巩固在科学计算和AI领域的地位,2.JavaScript将推动Web技术发展,3.跨平台开发将成为热门,4.性能优化将是重点。两者都将继续在各自领域扩展应用场景,并在性能上有更多突破。
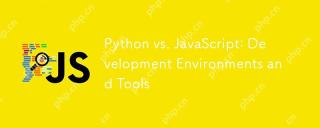
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具
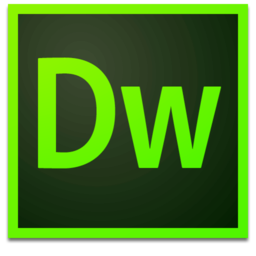
Dreamweaver Mac版
视觉化网页开发工具
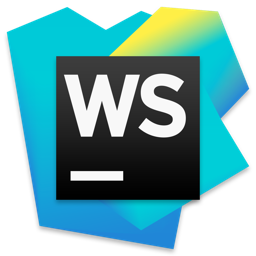
WebStorm Mac版
好用的JavaScript开发工具

MinGW - 适用于 Windows 的极简 GNU
这个项目正在迁移到osdn.net/projects/mingw的过程中,你可以继续在那里关注我们。MinGW:GNU编译器集合(GCC)的本地Windows移植版本,可自由分发的导入库和用于构建本地Windows应用程序的头文件;包括对MSVC运行时的扩展,以支持C99功能。MinGW的所有软件都可以在64位Windows平台上运行。
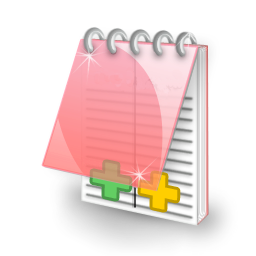
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。