使用 PostgreSQL 在单个查询中批量更新多行
问题:
如何在 PostgreSQL 中使用单个 SQL 语句同时更新多行去吗?
答案:
要使用单个查询在 PostgreSQL 中实现批量更新,建议使用派生表。以下示例演示了如何使用派生表更新多行:
UPDATE t SET column_a = v.column_a, column_b = v.column_b FROM (VALUES (1, 'FINISH', 1234), (2, 'UNFINISH', 3124) ) v(id, column_a, column_b) WHERE v.id = t.id;
Go 实现:
以下 Go 代码演示了执行批量更新查询:
import ( "context" "database/sql" "fmt" _ "github.com/lib/pq" // PostgreSQL driver ) func main() { // Open a database connection db, err := sql.Open("postgres", "user=postgres password=mypassword host=localhost port=5432 database=mydatabase") if err != nil { panic(err) } defer db.Close() // Create the derived table derivedTableQuery := ` CREATE TEMP TABLE BulkUpdate AS SELECT 1 AS id, 'FINISH' AS column_a, 1234 AS column_b UNION ALL SELECT 2, 'UNFINISH', 3124 ` if _, err := db.Exec(derivedTableQuery); err != nil { panic(err) } // Execute the bulk update query bulkUpdateQuery := ` UPDATE t SET column_a = v.column_a, column_b = v.column_b FROM BulkUpdate v WHERE v.id = t.id ` // Create a context for the query execution ctx := context.Background() // Execute the bulk update query result, err := db.ExecContext(ctx, bulkUpdateQuery) if err != nil { panic(err) } // Retrieve the number of rows affected rowsAffected, err := result.RowsAffected() if err != nil { panic(err) } // Print the number of rows affected fmt.Printf("%d rows updated\n", rowsAffected) }
以上是如何使用 Go 在 PostgreSQL 中执行批量更新?的详细内容。更多信息请关注PHP中文网其他相关文章!
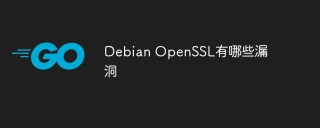
OpenSSL,作为广泛应用于安全通信的开源库,提供了加密算法、密钥和证书管理等功能。然而,其历史版本中存在一些已知安全漏洞,其中一些危害极大。本文将重点介绍Debian系统中OpenSSL的常见漏洞及应对措施。DebianOpenSSL已知漏洞:OpenSSL曾出现过多个严重漏洞,例如:心脏出血漏洞(CVE-2014-0160):该漏洞影响OpenSSL1.0.1至1.0.1f以及1.0.2至1.0.2beta版本。攻击者可利用此漏洞未经授权读取服务器上的敏感信息,包括加密密钥等。
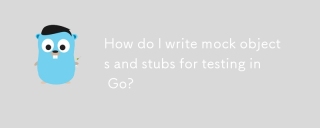
本文演示了创建模拟和存根进行单元测试。 它强调使用接口,提供模拟实现的示例,并讨论最佳实践,例如保持模拟集中并使用断言库。 文章
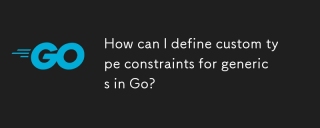
本文探讨了GO的仿制药自定义类型约束。 它详细介绍了界面如何定义通用功能的最低类型要求,从而改善了类型的安全性和代码可重复使用性。 本文还讨论了局限性和最佳实践
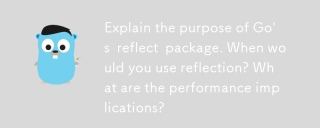
本文讨论了GO的反思软件包,用于运行时操作代码,对序列化,通用编程等有益。它警告性能成本,例如较慢的执行和更高的内存使用,建议明智的使用和最佳
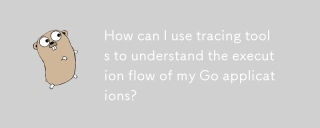
本文使用跟踪工具探讨了GO应用程序执行流。 它讨论了手册和自动仪器技术,比较诸如Jaeger,Zipkin和Opentelemetry之类的工具,并突出显示有效的数据可视化
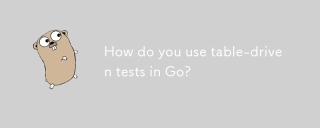
本文讨论了GO中使用表驱动的测试,该方法使用测试用例表来测试具有多个输入和结果的功能。它突出了诸如提高的可读性,降低重复,可伸缩性,一致性和A


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器
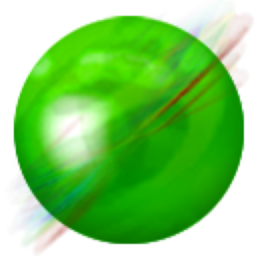
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),

SecLists
SecLists是最终安全测试人员的伙伴。它是一个包含各种类型列表的集合,这些列表在安全评估过程中经常使用,都在一个地方。SecLists通过方便地提供安全测试人员可能需要的所有列表,帮助提高安全测试的效率和生产力。列表类型包括用户名、密码、URL、模糊测试有效载荷、敏感数据模式、Web shell等等。测试人员只需将此存储库拉到新的测试机上,他就可以访问到所需的每种类型的列表。