介绍
有效管理配置是构建可扩展和可维护软件的基石。在 Go 中,Viper 包?作为管理应用程序配置的强大解决方案而脱颖而出。通过支持多种文件格式、环境变量和无缝解组到结构,Viper 简化了现代应用程序的配置管理。
在本博客中,我们将介绍如何使用 Viper 加载和管理来自不同来源的配置,将它们映射到 Go 结构体,以及动态集成环境变量。
??设置 Viper:
让我们深入了解 Viper 在 Go 应用程序中的实际实现。在本指南中,我们将使用一个简单的应用程序配置示例,其中包含 YAML 文件和环境变量。
第 1 步:安装 Viper 软件包
首先在您的项目中安装 Viper:
go get github.com/spf13/viper
第 2 步:创建配置文件
在项目的根目录中创建一个 config.yaml 文件。该文件将定义您的应用程序的默认配置:
app: name: "MyApp" port: 8080 namespace: "myapp" owner: "John Doe"
?在 Go 中实现 Viper
以下是如何在应用程序中使用 Viper。以下是 main.go 中的示例代码:
package main import ( "fmt" "log" "strings" "github.com/spf13/viper" ) type AppConfig struct { App struct { Name string `mapstructure:"name"` Port int `mapstructure:"port"` } `mapstructure:"app"` NS string `mapstructure:"namespace"` Owner string `mapstructure:"owner"` } func main() { // Set up viper to read the config.yaml file viper.SetConfigName("config") // Config file name without extension viper.SetConfigType("yaml") // Config file type viper.AddConfigPath(".") // Look for the config file in the current directory /* AutomaticEnv will check for an environment variable any time a viper.Get request is made. It will apply the following rules. It will check for an environment variable with a name matching the key uppercased and prefixed with the EnvPrefix if set. */ viper.AutomaticEnv() viper.SetEnvPrefix("env") // will be uppercased automatically viper.SetEnvKeyReplacer(strings.NewReplacer(".", "_")) // this is useful e.g. want to use . in Get() calls, but environmental variables to use _ delimiters (e.g. app.port -> APP_PORT) // Read the config file err := viper.ReadInConfig() if err != nil { log.Fatalf("Error reading config file, %s", err) } // Set up environment variable mappings if necessary /* BindEnv takes one or more parameters. The first parameter is the key name, the rest are the name of the environment variables to bind to this key. If more than one are provided, they will take precedence in the specified order. The name of the environment variable is case sensitive. If the ENV variable name is not provided, then Viper will automatically assume that the ENV variable matches the following format: prefix + "_" + the key name in ALL CAPS. When you explicitly provide the ENV variable name (the second parameter), it does not automatically add the prefix. For example if the second parameter is "id", Viper will look for the ENV variable "ID". */ viper.BindEnv("app.name", "APP_NAME") // Bind the app.name key to the APP_NAME environment variable // Get the values, using env variables if present appName := viper.GetString("app.name") namespace := viper.GetString("namespace") // AutomaticEnv will look for an environment variable called `ENV_NAMESPACE` ( prefix + "_" + key in ALL CAPS) appPort := viper.GetInt("app.port") // AutomaticEnv will look for an environment variable called `ENV_APP_PORT` ( prefix + "_" + key in ALL CAPS with _ delimiters) // Output the configuration values fmt.Printf("App Name: %s\n", appName) fmt.Printf("Namespace: %s\n", namespace) fmt.Printf("App Port: %d\n", appPort) // Create an instance of AppConfig var config AppConfig // Unmarshal the config file into the AppConfig struct err = viper.Unmarshal(&config) if err != nil { log.Fatalf("Unable to decode into struct, %v", err) } // Output the configuration values fmt.Printf("Config: %v\n", config) }
使用环境变量?
要动态集成环境变量,请创建一个包含以下内容的 .env 文件:
export APP_NAME="MyCustomApp" export ENV_NAMESPACE="go-viper" export ENV_APP_PORT=9090
运行命令加载环境变量:
source .env
在代码中,Viper的AutomaticEnv和SetEnvKeyReplacer方法允许您将嵌套配置键(如app.port)映射到环境变量(如APP_PORT)。其工作原理如下:
- 带有 SetEnvPrefix 的前缀:
viper.SetEnvPrefix("env") 行确保所有环境变量查找都以 ENV_ 为前缀。例如:
- app.port 变为 ENV_APP_PORT
- 命名空间变为 ENV_NAMESPACE
- 使用 SetEnvKeyReplacer 进行密钥替换: SetEnvKeyReplacer(strings.NewReplacer(".", "_")) 替换 .键名称中包含 _,因此像 app.port 这样的嵌套键可以直接映射到环境变量。
通过结合这两种方法,您可以使用环境变量无缝覆盖特定的配置值。
?运行示例
使用以下命令运行应用程序:
go get github.com/spf13/viper
预期输出:
app: name: "MyApp" port: 8080 namespace: "myapp" owner: "John Doe"
最佳实践?
- 对敏感数据使用环境变量:避免在配置文件中存储机密。使用环境变量或秘密管理工具。
- 设置默认值: 使用 viper.SetDefault("key", value) 确保您的应用程序具有合理的默认值。
- 验证配置: 加载配置后,验证它们以防止运行时错误。
- 保持配置井然有序: 为了清晰起见,将相关配置分组在一起并使用嵌套结构。
?结论
通过利用 Viper,您可以简化 Go 应用程序中的配置管理。它集成多个源的灵活性、动态环境变量支持以及对结构的解组使其成为开发人员不可或缺的工具。
开始在您的下一个项目中使用 Viper 并体验无忧的配置管理。快乐编码! ?
以上是Go with Viper 配置管理指南的详细内容。更多信息请关注PHP中文网其他相关文章!
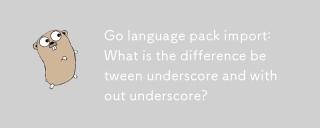
本文解释了GO的软件包导入机制:命名imports(例如导入“ fmt”)和空白导入(例如导入_ fmt; fmt;)。 命名导入使包装内容可访问,而空白导入仅执行t

本文解释了Beego的NewFlash()函数,用于Web应用程序中的页间数据传输。 它专注于使用newflash()在控制器之间显示临时消息(成功,错误,警告),并利用会话机制。 Lima
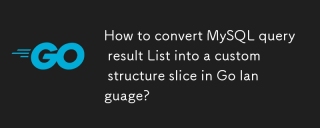
本文详细介绍了MySQL查询结果的有效转换为GO结构切片。 它强调使用数据库/SQL的扫描方法来最佳性能,避免手动解析。 使用DB标签和Robus的结构现场映射的最佳实践
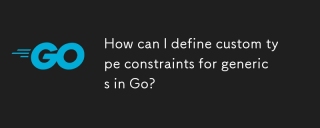
本文探讨了GO的仿制药自定义类型约束。 它详细介绍了界面如何定义通用功能的最低类型要求,从而改善了类型的安全性和代码可重复使用性。 本文还讨论了局限性和最佳实践
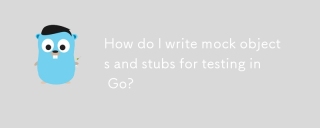
本文演示了创建模拟和存根进行单元测试。 它强调使用接口,提供模拟实现的示例,并讨论最佳实践,例如保持模拟集中并使用断言库。 文章

本文详细介绍了在GO中详细介绍有效的文件,将OS.WriteFile(适用于小文件)与OS.openfile和缓冲写入(最佳大型文件)进行比较。 它强调了使用延迟并检查特定错误的可靠错误处理。
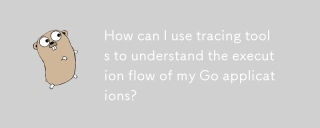
本文使用跟踪工具探讨了GO应用程序执行流。 它讨论了手册和自动仪器技术,比较诸如Jaeger,Zipkin和Opentelemetry之类的工具,并突出显示有效的数据可视化


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

PhpStorm Mac 版本
最新(2018.2.1 )专业的PHP集成开发工具
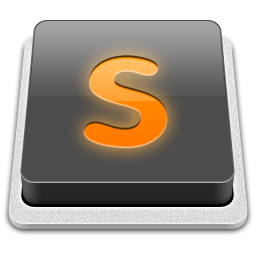
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

mPDF
mPDF是一个PHP库,可以从UTF-8编码的HTML生成PDF文件。原作者Ian Back编写mPDF以从他的网站上“即时”输出PDF文件,并处理不同的语言。与原始脚本如HTML2FPDF相比,它的速度较慢,并且在使用Unicode字体时生成的文件较大,但支持CSS样式等,并进行了大量增强。支持几乎所有语言,包括RTL(阿拉伯语和希伯来语)和CJK(中日韩)。支持嵌套的块级元素(如P、DIV),

记事本++7.3.1
好用且免费的代码编辑器

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。