检查用户输入的整数有效性
在提供的 C 代码中,目标是开发一个从用户读取两个整数的程序并对它们执行基本的数学运算。然而,一个重要的考虑因素出现了:如何确保用户输入有效的整数。
要检查输入是否是整数,我们可以使用 cin.fail() 函数。如果输入有效,则返回 false;如果输入无效或包含非数字字符,则返回 true。
检查有效整数
以下代码片段演示了如何检查用户输入的两个整数的有效性:
int firstvariable, secondvariable; cin >> firstvariable; if (cin.fail()) { // Not an integer; handle appropriately } cin >> secondvariable; if (cin.fail()) { // Not an integer; handle appropriately }
如果输入无效,则需要进行错误处理。这可能涉及显示消息、清除输入流以及重新提示用户输入正确的整数。
处理无效输入
确保连续输入,直到输入一个有效的整数,我们可以实现一个循环,直到输入通过有效性检查:
while (cin.fail()) { // Clear the input stream cin.clear(); // Ignore the invalid input cin.ignore(256, '\n'); // Re-prompt the user for a valid integer cout > firstvariable; }
在此循环,清除输入流,忽略无效输入,并提示用户输入有效整数。
示例
以下代码提供了完整的检查有效整数输入并处理无效输入的示例:
#include <iostream> using namespace std; int main() { int firstvariable, secondvariable; cout > firstvariable; while (cin.fail()) { cin.clear(); cin.ignore(256, '\n'); cout > firstvariable; } cin >> secondvariable; while (cin.fail()) { cin.clear(); cin.ignore(256, '\n'); cout > secondvariable; } // Perform mathematical operations on the valid integers return 0; }</iostream>
以上是如何确保 C 中整数输入有效?的详细内容。更多信息请关注PHP中文网其他相关文章!
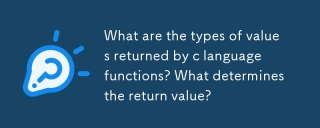
本文详细介绍了C函数返回类型,包括基本(int,float,char等),派生(数组,指针,结构)和void类型。 编译器通过函数声明和返回语句确定返回类型,执行

Gulc是一个高性能的C库,优先考虑最小开销,积极的内衬和编译器优化。 其设计非常适合高频交易和嵌入式系统等关键应用程序,其设计强调简单性,模型
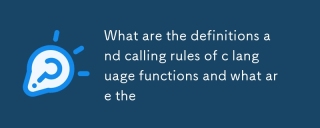
本文解释了C函数声明与定义,参数传递(按值和指针),返回值以及常见的陷阱,例如内存泄漏和类型不匹配。 它强调了声明对模块化和省份的重要性

本文详细介绍了字符串案例转换的C功能。 它可以通过ctype.h的toupper()和tolower()解释,并通过字符串迭代并处理零终端。 常见的陷阱,例如忘记ctype.h和修改字符串文字是
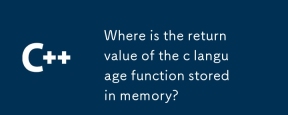
本文研究C函数返回值存储。 较小的返回值通常存储在寄存器中以备速度;较大的值可能会使用指针来记忆(堆栈或堆),影响寿命并需要手动内存管理。直接ACC
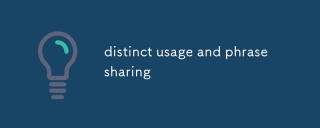
本文分析了形容词“独特”的多方面用途,探索其语法功能,常见的短语(例如,“不同于”,“完全不同”),以及在正式与非正式中的细微应用
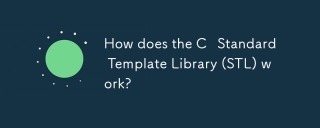
本文解释了C标准模板库(STL),重点关注其核心组件:容器,迭代器,算法和函子。 它详细介绍了这些如何交互以启用通用编程,提高代码效率和可读性t
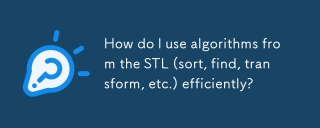
本文详细介绍了c中有效的STL算法用法。 它强调了数据结构选择(向量与列表),算法复杂性分析(例如,std :: sort vs. std vs. std :: partial_sort),迭代器用法和并行执行。 常见的陷阱


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。
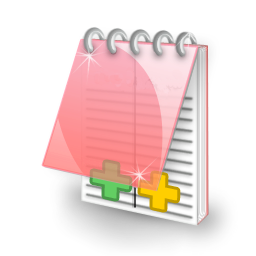
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能
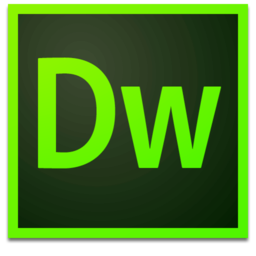
Dreamweaver Mac版
视觉化网页开发工具

记事本++7.3.1
好用且免费的代码编辑器
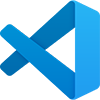
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器