使用 JavaScript 处理引用的 CSV 字段中的逗号
在 JavaScript 中处理 CSV(逗号分隔值)字符串时,解析可以当数据可以在引用字段中包含逗号时,这将具有挑战性。以下是如何通过详细的解决方案有效处理这种情况:
用于验证和解析 CSV 字符串的正则表达式
确保输入字符串是有效的 CSV 字符串,我们定义一个验证正则表达式:
re_valid = r""" # Validate a CSV string having single, double or un-quoted values. ^ # Anchor to start of string. \s* # Allow whitespace before value. (?: # Group for value alternatives. '[^'\]*(?:\[\S\s][^'\]*)*' # Either Single quoted string, | "[^"\]*(?:\[\S\s][^"\]*)*" # or Double quoted string, | [^,'"\s\]*(?:\s+[^,'"\s\]+)* # or Non-comma, non-quote stuff. ) # End group of value alternatives. \s* # Allow whitespace after value. (?: # Zero or more additional values , # Values separated by a comma. \s* # Allow whitespace before value. (?: # Group for value alternatives. '[^'\]*(?:\[\S\s][^'\]*)*' # Either Single quoted string, | "[^"\]*(?:\[\S\s][^"\]*)*" # or Double quoted string, | [^,'"\s\]*(?:\s+[^,'"\s\]+)* # or Non-comma, non-quote stuff. ) # End group of value alternatives. \s* # Allow whitespace after value. )* # Zero or more additional values $ # Anchor to end of string. """
从经过验证的 CSV 中解析各个值字符串,我们使用以下正则表达式:
re_value = r""" # Match one value in valid CSV string. (?!\s*$) # Don't match empty last value. \s* # Strip whitespace before value. (?: # Group for value alternatives. '([^'\]*(?:\[\S\s][^'\]*)*)' # Either : Single quoted string, | "([^"\]*(?:\[\S\s][^"\]*)*)" # or : Double quoted string, | ([^,'"\s\]*(?:\s+[^,'"\s\]+)*) # or : Non-comma, non-quote stuff. ) # End group of value alternatives. \s* # Strip whitespace after value. (?:,|$) # Field ends on comma or EOS. """
CSV 解析函数
定义这些正则表达式后,我们可以实现一个解析 CSV 字符串的函数:
function CSVtoArray(text) { // Return NULL if input string is not well formed CSV string. if (!re_valid.test(text)) return null; var a = []; // Initialize array to receive values. text.replace(re_value, // "Walk" the string using replace with callback. function(m0, m1, m2, m3) { // Remove backslash from \' in single quoted values. if (m1 !== undefined) a.push(m1.replace(/\'/g, "'")); // Remove backslash from \" in double quoted values. else if (m2 !== undefined) a.push(m2.replace(/\"/g, '"')); else if (m3 !== undefined) a.push(m3); return ''; // Return empty string. }); // Handle special case of empty last value. if (/,\s*$/.test(text)) a.push(''); return a; }
示例用法
以下是输入 CSV 字符串及其相应解析输出的一些示例:
// Test string from original question let result = CSVtoArray("'string, duppi, du', 23, lala"); console.log(result); // ['string, duppi, du', '23', 'lala'] // Empty CSV string let result = CSVtoArray(""); console.log(result); // [] // CSV string with two empty values let result = CSVtoArray(","); console.log(result); // ['', ''] // Double quoted CSV string having single quoted values let result = CSVtoArray("'one','two with escaped \' single quote', 'three, with, commas'"); console.log(result); // ['one', 'two with escaped \' single quote', 'three, with, commas'] // Single quoted CSV string having double quoted values let result = CSVtoArray('"one","two with escaped \" double quote", "three, with, commas"'); console.log(result); // ['one', 'two with escaped " double quote', 'three, with, commas'] // CSV string with whitespace in and around empty and non-empty values let result = CSVtoArray(" one , 'two' , , ' four' ,, 'six ', ' seven ' , "); console.log(result); // ['one', 'two', '', 'four', '', 'six ', ' seven '] // Not valid let result = CSVtoArray("one, that's me!, escaped \, comma"); console.log(result); // null
此解决方案可确保准确解析 CSV 字符串,处理包含逗号的引用字段,同时坚持达到指定要求。
以上是JavaScript 如何有效解析引用字段中带有逗号的 CSV 字符串?的详细内容。更多信息请关注PHP中文网其他相关文章!
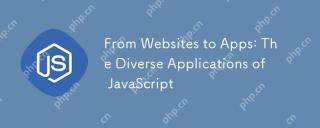
JavaScript在网站、移动应用、桌面应用和服务器端编程中均有广泛应用。1)在网站开发中,JavaScript与HTML、CSS一起操作DOM,实现动态效果,并支持如jQuery、React等框架。2)通过ReactNative和Ionic,JavaScript用于开发跨平台移动应用。3)Electron框架使JavaScript能构建桌面应用。4)Node.js让JavaScript在服务器端运行,支持高并发请求。
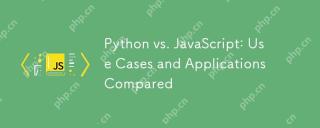
Python更适合数据科学和自动化,JavaScript更适合前端和全栈开发。1.Python在数据科学和机器学习中表现出色,使用NumPy、Pandas等库进行数据处理和建模。2.Python在自动化和脚本编写方面简洁高效。3.JavaScript在前端开发中不可或缺,用于构建动态网页和单页面应用。4.JavaScript通过Node.js在后端开发中发挥作用,支持全栈开发。
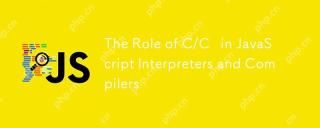
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。
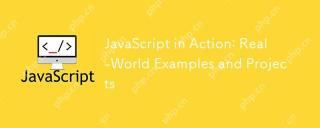
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
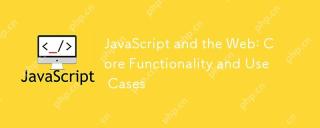
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
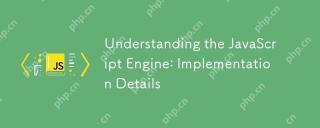
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。

Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
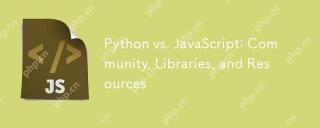
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具
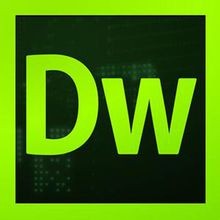
Dreamweaver CS6
视觉化网页开发工具

适用于 Eclipse 的 SAP NetWeaver 服务器适配器
将Eclipse与SAP NetWeaver应用服务器集成。

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。

禅工作室 13.0.1
功能强大的PHP集成开发环境

PhpStorm Mac 版本
最新(2018.2.1 )专业的PHP集成开发工具